How to use : Java GUI JTable - Update data from all Cell in JTable |
How to use : Java GUI JTable - Update data from all Cell in JTable ตัวอย่างการเขียน Java GUI กับ JTable เพื่อทำการอ่านข้อมูลจาก Database มาแสดงบน JTable และการสร้างระบบการแก้ไข Update ข้อมูลจาก Cell ของ JTable ไปยัง Database ซึ่งปกติแล้วใน JTable เราจะสามารถทำการแก้ไขข้อมูลบน Cell ได้ทันที และเราจะนำข้อมูลที่ผู้ใช้ทำการแก้ไขนี้ Update ลงไปใน Database
How to use : Java GUI JTable - Update data all Cell in JTable
Syntax
for(int i=0 ; i<table.getRowCount(); i++)
{
String CustomerID = table.getValueAt(i, 0).toString();
String Name = table.getValueAt(i, 1).toString();
String Email = table.getValueAt(i, 2).toString();
String CountryCode = table.getValueAt(i, 3).toString();
String Budget = table.getValueAt(i, 4).toString();
String Used = table.getValueAt(i, 5).toString();
String sql = "UPDATE customer " +
"SET Name = '" + Name + "' " +
", Email = '" + Email + "' " +
", CountryCode = '" + CountryCode + "' " +
", Budget = '" + Budget + "' " +
", Used = '" + Used + "' " +
" WHERE CustomerID = '"+CustomerID+"' ";
s.execute(sql);
}
ในตัวอย่างนี้จะเลือกใช้ Database ของ MySQL แต่ในกรณีที่จะใช้ร่วมกับ Database อื่น ๆ ก็สามารถทำได้ง่าย ๆ เพียงแค่เปลี่ยน Connector และ Connection String เท่านั้น และการแสดงข้อมูลจะใช้ JTable ของ Java Swing สามารถอ่านเพิ่มเติมได้ที่บทความนี้
Java Connect to MySQL Database (JDBC)
โครงสร้างของ MySQL และ Table
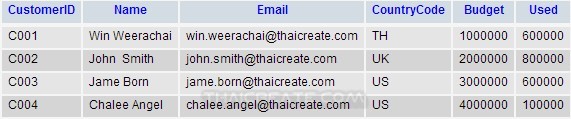
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- dump ตาราง `customer`
--
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
คำสั่งของ SQL ที่สามารถนำไปรันบน Query เพื่อสร้าง Table และ Rows ได้ทันที
Example การสร้าง Java GUI กับ JTable และการ Update ข้อมูลใน Database จากการแก้ไขบน Cell
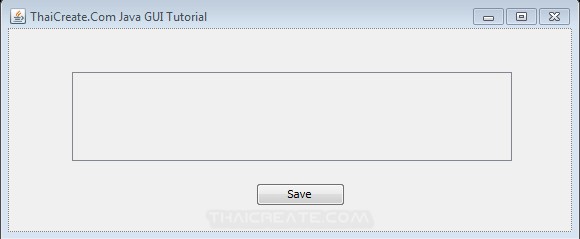
MyForm.java
package com.java.myapp;
import java.awt.Color;
import java.awt.Component;
import java.awt.EventQueue;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import javax.swing.JOptionPane;
import javax.swing.JFrame;
import javax.swing.JTable;
import javax.swing.JScrollPane;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableCellRenderer;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class MyForm extends JFrame {
private JTable table;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm frame = new MyForm();
frame.setVisible(true);
}
});
}
/**
* Create the frame.
*/
public MyForm() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 580, 242);
setTitle("ThaiCreate.Com Java GUI Tutorial");
getContentPane().setLayout(null);
// ScrollPane
JScrollPane scrollPane = new JScrollPane();
scrollPane.setBounds(64, 44, 440, 89);
getContentPane().add(scrollPane);
// Table
table = new JTable(){
public boolean isCellEditable(int rowIndex, int colIndex) {
if(colIndex==0) {
return false; // Disallow Column 0
} else {
return true; // Allow the editing
}
}
@Override
public Component prepareRenderer(TableCellRenderer renderer, int row, int column) {
Component comp = super.prepareRenderer(renderer, row, column);
if (column==0) {
comp.setBackground(Color.lightGray);
} else {
comp.setBackground(Color.white);
}
return comp;
}
};
scrollPane.setViewportView(table);
// Update
JButton btnSave = new JButton("Save");
btnSave.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
UpdateData(); // UpdateData
}
});
btnSave.setBounds(248, 155, 89, 23);
getContentPane().add(btnSave);
// Windows Loaded
addWindowListener(new WindowAdapter() {
@Override
public void windowOpened(WindowEvent e) {
PopulateData(); // PopulateData
}
});
}
// Connection
private Connection ConnectDB() {
try {
Class.forName("com.mysql.jdbc.Driver");
return DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
} catch (ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
// PopulateData
private void PopulateData()
{
// Clear Table
table.setModel(new DefaultTableModel());
// Model for Table
DefaultTableModel model = (DefaultTableModel)table.getModel();
model.addColumn("CustomerID");
model.addColumn("Name");
model.addColumn("Email");
model.addColumn("CountryCode");
model.addColumn("Budget");
model.addColumn("Used");
Connection connect = ConnectDB();
Statement s = null;
try {
s = connect.createStatement();
String sql = "SELECT * FROM customer ORDER BY CustomerID ASC";
ResultSet rec = s.executeQuery(sql);
int row = 0;
while((rec!=null) && (rec.next()))
{
model.addRow(new Object[0]);
model.setValueAt(rec.getString("CustomerID"), row, 0);
model.setValueAt(rec.getString("Name"), row, 1);
model.setValueAt(rec.getString("Email"), row, 2);
model.setValueAt(rec.getString("CountryCode"), row, 3);
model.setValueAt(rec.getFloat("Budget"), row, 4);
model.setValueAt(rec.getFloat("Used"), row, 5);
row++;
}
rec.close();
} catch (Exception e) {
// TODO Auto-generated catch block
JOptionPane.showMessageDialog(null, e.getMessage());
e.printStackTrace();
}
try {
if(s != null) {
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
// UpdateData
private void UpdateData()
{
Connection connect = ConnectDB();
Statement s = null;
try {
s = connect.createStatement();
for(int i=0 ; i<table.getRowCount(); i++)
{
String CustomerID = table.getValueAt(i, 0).toString();
String Name = table.getValueAt(i, 1).toString();
String Email = table.getValueAt(i, 2).toString();
String CountryCode = table.getValueAt(i, 3).toString();
String Budget = table.getValueAt(i, 4).toString();
String Used = table.getValueAt(i, 5).toString();
String sql = "UPDATE customer " +
"SET Name = '" + Name + "' " +
", Email = '" + Email + "' " +
", CountryCode = '" + CountryCode + "' " +
", Budget = '" + Budget + "' " +
", Used = '" + Used + "' " +
" WHERE CustomerID = '"+CustomerID+"' ";
s.execute(sql);
}
JOptionPane.showMessageDialog(null, "Record Update Successfully");
PopulateData(); // Reload Data
} catch (Exception e) {
// TODO Auto-generated catch block
JOptionPane.showMessageDialog(null, e.getMessage());
e.printStackTrace();
}
try {
if(s != null) {
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
}
}
Output
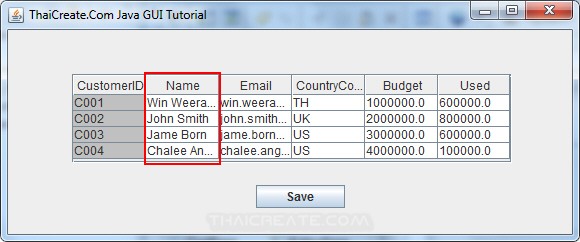
แสดง Frame และ JTable ใน Column แรกเราจะไม่ให้แก้ไขเพราะเป็น Key ของ Table
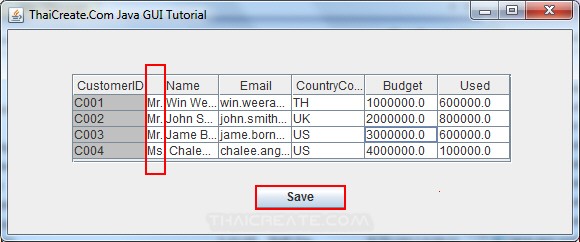
ทดสอบการแก้ไขข้อมูล
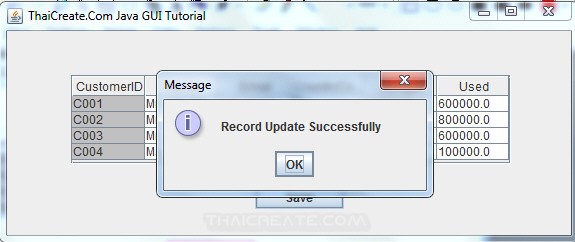
แก้ไข Update ข้อมูลเรียบร้อย
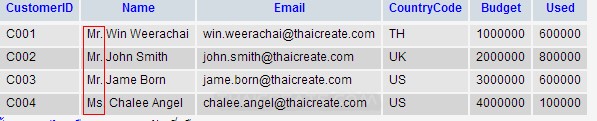
ข้อมูลใน Database ถูก Update
อ่านเพิ่มเติม : Java Table (JTable) - Swing Example
กรณีที่ใช้ร่วมกับ Database อื่น ๆ สามารถดูวิธีการใช้ Connector และ Connection String ได้ที่นี่
Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2013-09-10 10:11:24 /
2017-03-27 22:04:57 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|