How to use : Java GUI Login Username and Password via JDialog |
How to use : Java GUI Login Username and Password via JDialog บทความนี้จะเป็นตัวอย่างการเขียน Java GUI ทำระบบ Authentication ด้วย Username และ Password โดยหลักการของเราก็คือ ครั้งจากที่เปิด Application ครั้งแรกจะมี Dialog แจ้งให้กรอก Username และ Password ซึ่งจะใช้ Database ของ MySQL เป็นตัวจัดเก็บข้อมูลของผู้ใช้ และเมื่อผู้ใช้กรอกข้อมูลถูกต้องโปรแกรมจะยอมให้เทำการเข้าสู่หน้าแรกของ โปรแกรม
How to use : Java GUI Login Username and Password via JDialog
ในตัวอย่างนี้จะเลือกใช้ Database ของ MySQL แต่ในกรณีที่จะใช้ร่วมกับ Database อื่น ๆ ก็สามารถทำได้ง่าย ๆ เพียงแค่เปลี่ยน Connector และ Connection String เท่านั้น สามารถอ่านเพิ่มเติมได้ที่บทความนี้
Java Connect to MySQL Database (JDBC)
โครงสร้างของ MySQL และ Table

CREATE TABLE `member` (
`UserID` int(4) unsigned zerofill NOT NULL auto_increment,
`Username` varchar(20) NOT NULL,
`Password` varchar(20) NOT NULL,
`Email` varchar(150) NOT NULL,
`Name` varchar(250) NOT NULL,
PRIMARY KEY (`UserID`),
UNIQUE KEY `User` (`Username`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
--
-- dump ตาราง `member`
--
INSERT INTO `member` VALUES (0001, 'weerachai', 'weerachai@1', '[email protected]', 'Weerachai Nukitram');
INSERT INTO `member` VALUES (0002, 'surachai', 'surachai@2', '[email protected]', 'Surachai Sirisart');
INSERT INTO `member` VALUES (0003, 'adisorn', 'adisorn@3', '[email protected]', 'Adisorn Bonsong');
คำสั่งของ SQL ที่สามารถนำไปรันบน Query เพื่อสร้าง Table และ Rows ได้ทันที
Example การเขียน Java GUI เพื่อสร้างระบบ Login Username และ Password
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.swing.JComponent;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
import java.awt.Font;
public class MyForm extends JFrame {
public static String userName;
public static String passWord;
static JLabel lblWelcome;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm form = new MyForm();
form.setVisible(true);
}
});
}
public MyForm() {
// Create Form Frame
super("ThaiCreate.Com Tutorial");
setSize(679, 385);
setLocation(500, 280);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
getContentPane().setLayout(null);
// Label Welcome
lblWelcome = new JLabel("lblWelcome",JLabel.CENTER);
lblWelcome.setFont(new Font("Tahoma", Font.PLAIN, 20));
lblWelcome.setBounds(168, 153, 336, 25);
getContentPane().add(lblWelcome);
// When Frame Loaded
addWindowListener(new WindowAdapter() {
@Override
public void windowOpened(WindowEvent e) {
LoginDialog();
}
});
}
public static Boolean getLogin() {
Connection connect = null;
PreparedStatement pre = null;
Boolean status = false;
try {
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
String sql = " SELECT * FROM member " +
" WHERE Username = ? " +
" AND Password = ? ";
pre = connect.prepareStatement(sql);
pre.setString(1, userName);
pre.setString(2, passWord);
ResultSet rec = pre.executeQuery();
if(rec.next()) {
lblWelcome.setText("Welcome : " + rec.getString("Name"));
status = true;
} else {
JOptionPane.showMessageDialog(null, "Incorrect Username/Password");
}
} catch (Exception e) {
// TODO Auto-generated catch block
JOptionPane.showMessageDialog(null, e.getMessage());
e.printStackTrace();
}
try {
if(pre != null) {
pre.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
return status;
}
public static void LoginDialog() {
JLabel title = new JLabel("Login Username and Password");
JTextField username = new JTextField();
JPasswordField password = new JPasswordField();
final JComponent[] inputs = new JComponent[] {
title,
new JLabel("Username"),
username,
new JLabel("Password"),
password
};
JOptionPane.showMessageDialog(null, inputs, "Login", JOptionPane.PLAIN_MESSAGE);
userName = username.getText();
passWord = new String(password.getPassword());
// Check Login
if(!getLogin())
{
LoginDialog();
}
}
}

Output
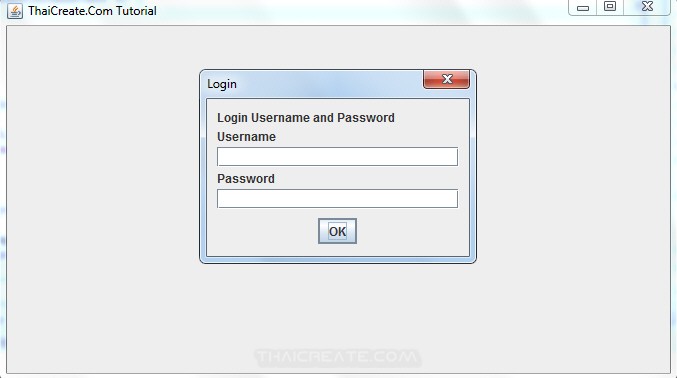
แสดงหน้า Frame หลักและมี Dialog ขึ้นให้กรอก Username และ Password
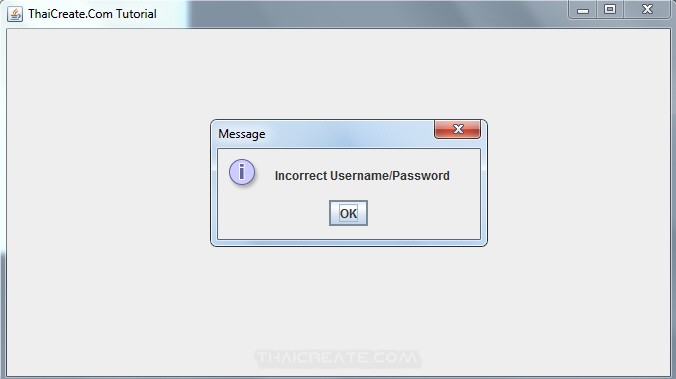
กรณีที่ Login ไม่ถูกต้อง
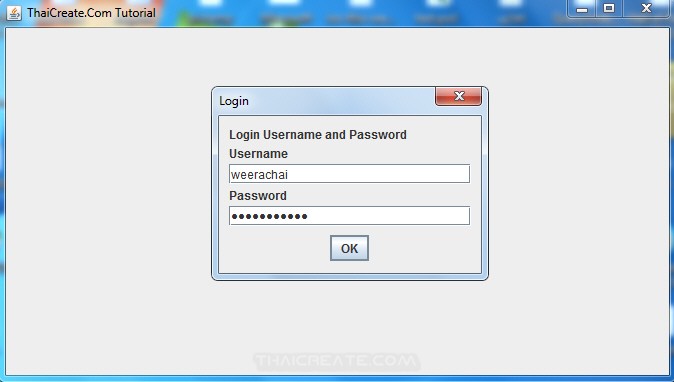
ทดสอบกรอกข้อมูล Username และ Password ให้ถูกต้อง
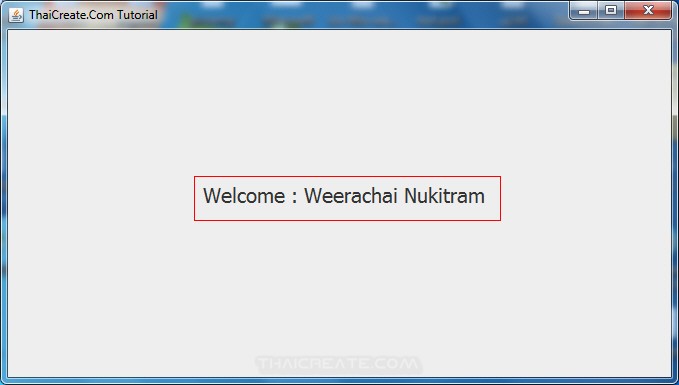
เข้าสู่หน้าจอ Application เรียบร้อยแล้ว
กรณีที่ใช้ร่วมกับ Database อื่น ๆ สามารถดูวิธีการใช้ Connector และ Connection String ได้ที่นี่
Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2013-09-10 10:04:08 /
2017-03-27 21:52:46 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|