Java Swing and File Chooser (JFileChooser) - Example |
Java Swing and File Chooser (JFileChooser) - Swing Example สำหรับ File Chooser หรือ JFileChooser (javax.swing.JFileChooser) จัดอยู่ในกลุ่มของ Swing Windows ใช้สร้าง Input Dialog แบบ File Chooser เพื่อทำการ Browse เลือกไฟล์จาก Path ต่าง ๆ ที่อยู่ในเครื่อง Computer โดยสามารถกำหนด Filter ประเภทของไฟล์ได้ เช่น เลือกเป็นแบบ Folder หรือเป็นไฟล์ หรือจะ Filter เฉาะนามสกุลต่าง ๆ ที่ต้องการ
Java Swing and File Chooser (JFileChooser) - Swing Example
Syntax
// File Chooser
JFileChooser fileopen = new JFileChooser();
FileFilter filter = new FileNameExtensionFilter("Text/CSV file", "txt", "csv");
fileopen.addChoosableFileFilter(filter);
// get Selected File
if (ret == JFileChooser.APPROVE_OPTION) {
lblResult.setText(fileopen.getSelectedFile().toString());
}
Controls Icon Tools

Example 1 ตัวอย่างการสร้าง File Chooser ด้วย JFileChooser และการแสดง Path ของไฟล์ที่เลือก Browse
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.filechooser.FileFilter;
import javax.swing.filechooser.FileNameExtensionFilter;
public class MyForm extends JFrame {
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm form = new MyForm();
form.setVisible(true);
}
});
}
public MyForm() {
// Create Form Frame
super("ThaiCreate.Com Java GUI Tutorial");
setSize(450, 300);
setLocation(500, 280);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
getContentPane().setLayout(null);
// Label Result
final JLabel lblResult = new JLabel("Result",JLabel.CENTER);
lblResult.setBounds(26, 54, 370, 14);
getContentPane().add(lblResult);
// Create Button Open JFileChooser
JButton btnButton = new JButton("Open File Chooser");
btnButton.setBounds(159, 94, 121, 23);
btnButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JFileChooser fileopen = new JFileChooser();
FileFilter filter = new FileNameExtensionFilter("Text/CSV file", "txt", "csv");
fileopen.addChoosableFileFilter(filter);
int ret = fileopen.showDialog(null, "Choose file");
if (ret == JFileChooser.APPROVE_OPTION) {
lblResult.setText(fileopen.getSelectedFile().toString());
}
}
});
getContentPane().add(btnButton);
}
}
Output
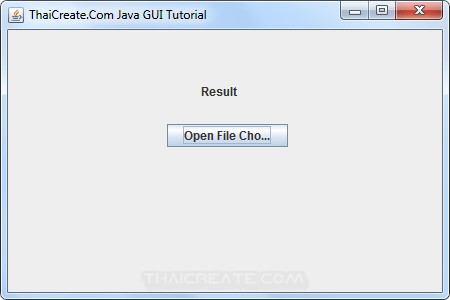
คลิกที่ Button เพื่อเปิด JFileChooser
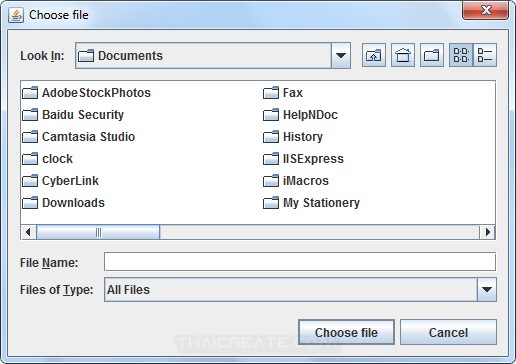
แสดง File Chooser Dialog เพื่อ Browse ไฟล์
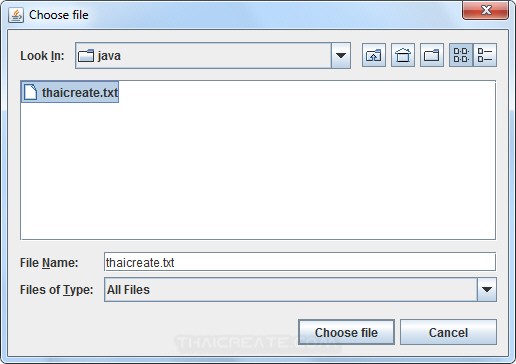
Browse เลือกไฟล์ที่ต้องการ
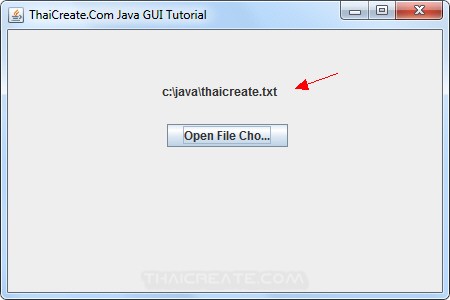
แสดง Path ของไฟล์ใน Label

Example 2 การสร้าง File Chooser และการอ่าน Text File จากที่ได้ Browse จาก File Chooser Dialog
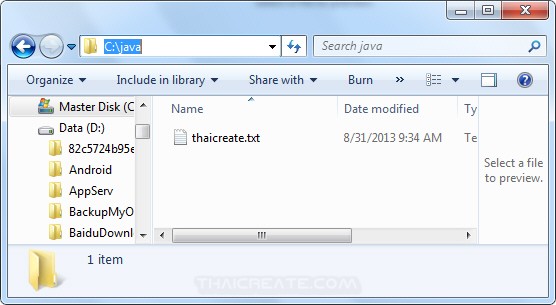
ทดสอบสร้าง Text File แบบง่าย ๆ
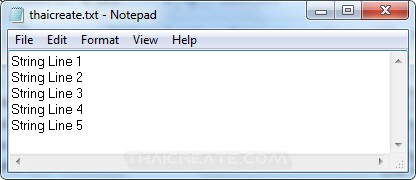
thaicreate.txt
String Line 1
String Line 2
String Line 3
String Line 4
String Line 5
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JScrollPane;
import javax.swing.JTextPane;
import javax.swing.filechooser.FileFilter;
import javax.swing.filechooser.FileNameExtensionFilter;
import javax.swing.text.BadLocationException;
import javax.swing.text.StyledDocument;
public class MyForm extends JFrame {
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm form = new MyForm();
form.setVisible(true);
}
});
}
public MyForm() {
// Create Form Frame
super("ThaiCreate.Com Java GUI Tutorial");
setSize(450, 300);
setLocation(500, 280);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
getContentPane().setLayout(null);
// Label Result
final JLabel lblResult = new JLabel("Result",JLabel.CENTER);
lblResult.setBounds(22, 22, 370, 14);
getContentPane().add(lblResult);
// EditPane
JTextPane textPane = new JTextPane();
// ScrollPane
JScrollPane scroll = new JScrollPane(textPane);
scroll.setBounds(124, 98, 162, 67);
getContentPane().add(scroll);
// Create txtPane Style
final StyledDocument doc = textPane.getStyledDocument();
// Create Button Open JFileChooser
JButton btnButton = new JButton("Open File Chooser");
btnButton.setBounds(147, 47, 135, 23);
btnButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JFileChooser fileopen = new JFileChooser();
FileFilter filter = new FileNameExtensionFilter("Text/CSV file", "txt", "csv");
fileopen.addChoosableFileFilter(filter);
int ret = fileopen.showDialog(null, "Choose file");
if (ret == JFileChooser.APPROVE_OPTION) {
// Read Text file
File file = fileopen.getSelectedFile();
try {
BufferedReader br = new BufferedReader(new FileReader(file));
String line;
while ((line = br.readLine()) != null) {
//System.out.println(line);
doc.insertString(doc.getLength(), line + "\n", null );
}
br.close();
} catch (IOException | BadLocationException ex) {
// TODO Auto-generated catch block
ex.printStackTrace();
}
lblResult.setText(fileopen.getSelectedFile().toString());
}
}
});
getContentPane().add(btnButton);
}
}
Output
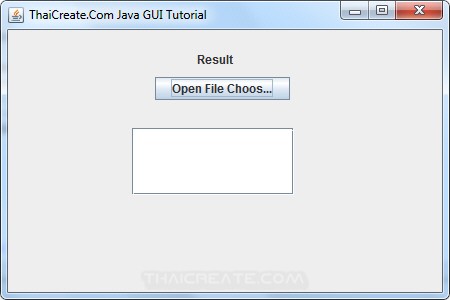
คลิกที่ Button เพื่อ Browse เลือกไฟล์
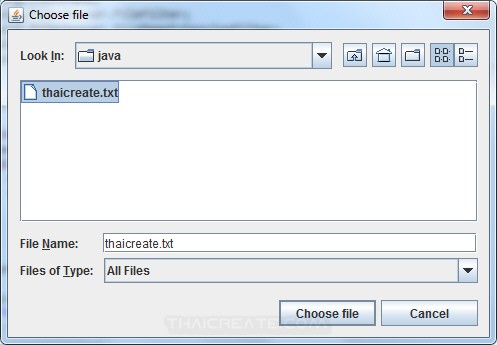
เลือกไฟล์ thaicreate.txt ที่ได้สร้างไว้
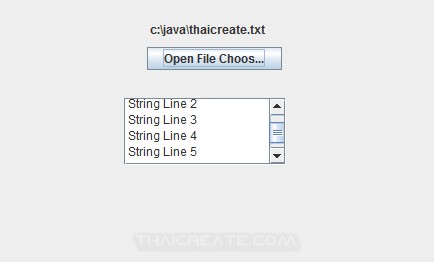
โปรแกรมจะอ่านไฟล์มาแสดงใน Text Pane (JTextPane)
Example 3 ตัวอย่างการสร้าง File Chooser เพื่อ Browse เลือกไฟล์ CSV จากนั้นนำข้อมูลที่ได้มาแสดงใน JTable
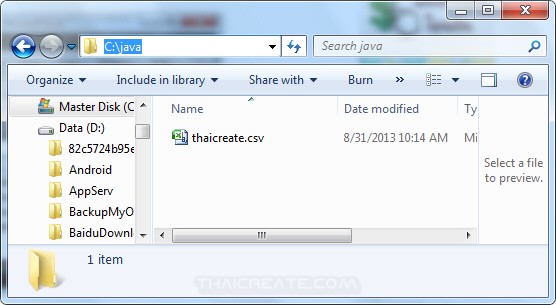
ไฟล์ CSV ที่อยู่ในเครื่อง
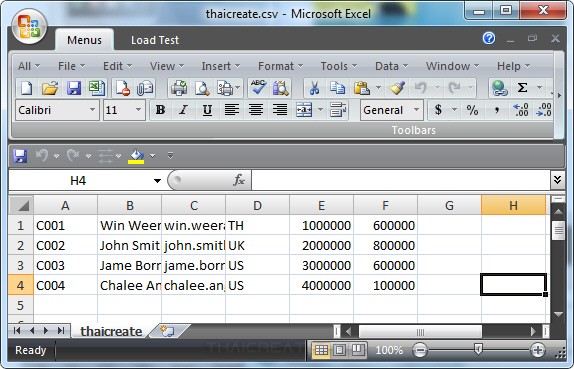
thaicreate.csv
C001,Win Weerachai,[email protected],TH,1000000,600000
C002,John Smith,[email protected],UK,2000000,800000
C003,Jame Born,[email protected],US,3000000,600000
C004,Chalee Angel,[email protected],US,4000000,100000
ไฟล์ CSV
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.filechooser.FileFilter;
import javax.swing.filechooser.FileNameExtensionFilter;
import javax.swing.table.AbstractTableModel;
public class MyForm extends JFrame {
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm form = new MyForm();
form.setVisible(true);
}
});
}
public MyForm() {
// Create Form Frame
super("ThaiCreate.Com Java GUI Tutorial");
setSize(668, 345);
setLocation(500, 280);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
getContentPane().setLayout(null);
// Label Result
final JLabel lblResult = new JLabel("Result", JLabel.CENTER);
lblResult.setBounds(150, 22, 370, 14);
getContentPane().add(lblResult);
// Data Source
final CustomModel model = new CustomModel();
// Table
JTable table = new JTable(model);
getContentPane().add(table);
// ScrollPane
JScrollPane scroll = new JScrollPane(table);
scroll.setBounds(84, 98, 506, 79);
getContentPane().add(scroll);
// Create Button Open JFileChooser
JButton btnButton = new JButton("Open File Chooser");
btnButton.setBounds(268, 47, 135, 23);
btnButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JFileChooser fileopen = new JFileChooser();
FileFilter filter = new FileNameExtensionFilter(
"Text/CSV file", "txt", "csv");
fileopen.addChoosableFileFilter(filter);
int ret = fileopen.showDialog(null, "Choose file");
if (ret == JFileChooser.APPROVE_OPTION) {
// Read Text file
File file = fileopen.getSelectedFile();
try {
BufferedReader br = new BufferedReader(new FileReader(
file));
String line;
while ((line = br.readLine()) != null) {
String[] arr = line.split(",");
model.addRow(arr[0],arr[1],arr[2],arr[3],arr[4],arr[5]);
}
br.close();
} catch (IOException ex) {
// TODO Auto-generated catch block
ex.printStackTrace();
}
lblResult.setText(fileopen.getSelectedFile().toString());
}
}
});
getContentPane().add(btnButton);
}
// **** Model ****//
class CustomModel extends AbstractTableModel {
List<Customer> dataRow;
String[] columnHeader = { "CustomerID", "Name", "Email",
"CountryCode", "Budget", "Used" };
int id = 0;
public CustomModel() {
dataRow = new ArrayList<Customer>();
}
@Override
public String getColumnName(int column) {
return columnHeader[column];
}
@Override
public int getColumnCount() {
return columnHeader.length;
}
@Override
public int getRowCount() {
return dataRow.size();
}
@Override
public Object getValueAt(int rowIndex, int columnIndex) {
Customer customer = dataRow.get(rowIndex);
switch (columnIndex) {
case 0:
return customer.getCustomerID();
case 1:
return customer.getName();
case 2:
return customer.getEmail();
case 3:
return customer.getCountryCode();
case 4:
return customer.getBudget();
case 5:
return customer.getUsed();
default:
return null;
}
}
public void addRow(String CustomerID, String Name, String Email,
String CountryCode, String Budget, String Used) {
dataRow.add(new Customer(CustomerID, Name, Email, CountryCode,
Budget, Used));
int rowCount = getRowCount();
fireTableRowsInserted(rowCount, rowCount);
}
}
}
// **** Customer Class ****//
class Customer {
private String CustomerID;
private String Name;
private String Email;
private String CountryCode;
private String Budget;
private String Used;
public Customer(String sCustomerID, String sName, String sEmail,
String sCountryCode, String sBudget, String sUsed) {
this.CustomerID = sCustomerID;
this.Name = sName;
this.Email = sEmail;
this.CountryCode = sCountryCode;
this.Budget = sBudget;
this.Used = sUsed;
}
// CustomerID
public String getCustomerID() {
return CustomerID;
}
public void setCustomerID(String tCustomerID) {
this.CustomerID = tCustomerID;
}
// Name
public String getName() {
return Name;
}
public void setName(String tName) {
this.Name = tName;
}
// Email
public String getEmail() {
return Email;
}
public void setEmail(String tEmail) {
this.Email = tEmail;
}
// CountryCode
public String getCountryCode() {
return CountryCode;
}
public void setCountryCode(String tCountryCode) {
this.CountryCode = tCountryCode;
}
// Budget
public String getBudget() {
return Budget;
}
public void setBudget(String tBudget) {
this.Budget = tBudget;
}
// Used
public String getUsed() {
return Used;
}
public void setUsed(String tUsed) {
this.Used = tUsed;
}
}
Output
สำหรับตัวอย่างการแสดงใน Table สามารถอ่านเพิ่มเติมได้จากบทความของ JTable
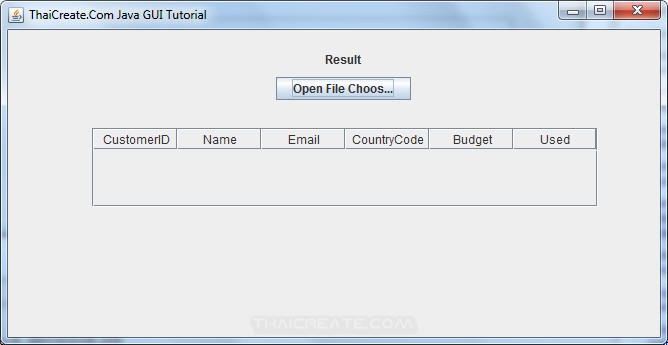
แสดง Frame และ JTable ให้คลิกที่ Button เพื่อเปิด File Chooser
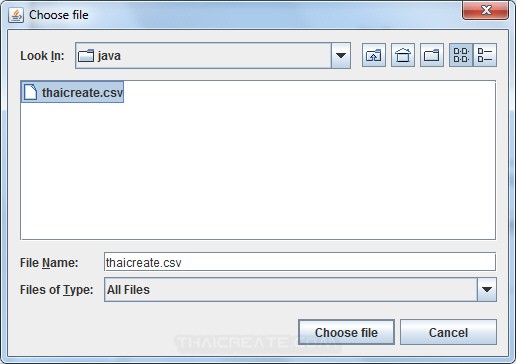
ทำการ File Chooser และ Browse เลือกไฟล์ CSV ที่ได้สร้างไว้
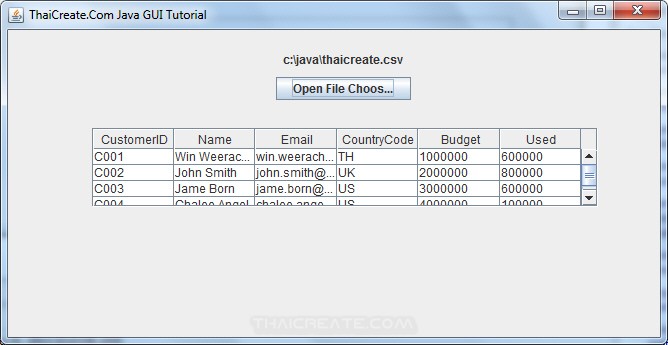
หลังจากที่คลิก Open ใน File Chooser จะได้ไฟล์ CSV มาแสดงข้อมูลที่ JTable
Property & Method (Others Related) |
|