Java Text Pane (JTextPane) - Swing Example |
Java Text Pane (JTextPane) - Swing Example สำหรับ Text Pane หรือ JTextPane (javax.swing.JTextPane) จัดอยู่ในกลุ่มของ Component ใช้สำหรับสร้างกล่องข้อความแบบกราฟฟิก สามารถแทรกและปรับแต่งขนาดรูปแบบของ String หรือจะแทรกเป็นรูปภาพ และอื่น ๆ ก็ได้เช่นเดียวกัน
Java Text Pane(JTextPane) - Swing Example
Syntax
JTextPane textPane = new JTextPane();
textPane.setText( "String String" );
Controls Icon Tools

Example 1 การสร้าง Text Pane ด้วย JTextPane แบบง่าย ๆ
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JTextPane;
public class MyForm extends JFrame {
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm frame = new MyForm();
frame.setVisible(true);
}
});
}
/**
* Create the frame.
*/
public MyForm() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 362, 249);
setTitle("ThaiCreate.Com Java GUI Tutorial");
getContentPane().setLayout(null);
// Text Pane
JTextPane textPane = new JTextPane();
textPane.setBounds(78, 34, 114, 137);
textPane.setText( "String String" );
getContentPane().add(textPane);
}
}
Output
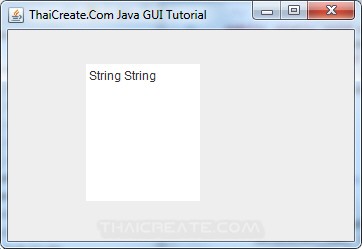
แสดง Text Pane แบบง่าย ๆ

Example 2 การสร้าง Text Pane ด้วย JTextPane ใส่ Style เช่น สี และแทรกรูปภาพ
MyForm.java
package com.java.myapp;
import java.awt.Color;
import java.awt.EventQueue;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JTextPane;
import javax.swing.text.SimpleAttributeSet;
import javax.swing.text.StyleConstants;
import javax.swing.text.StyledDocument;
public class MyForm extends JFrame {
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm frame = new MyForm();
frame.setVisible(true);
}
});
}
/**
* Create the frame.
*/
public MyForm() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 362, 249);
setTitle("ThaiCreate.Com Java GUI Tutorial");
getContentPane().setLayout(null);
JTextPane textPane = new JTextPane();
textPane.setBounds(78, 34, 114, 137);
textPane.setText( "String Of Line 1" );
StyledDocument doc = textPane.getStyledDocument();
// Define a style1
SimpleAttributeSet style1 = new SimpleAttributeSet();
StyleConstants.setForeground(style1, Color.RED);
StyleConstants.setBackground(style1, Color.YELLOW);
StyleConstants.setBold(style1, true);
SimpleAttributeSet style2 = new SimpleAttributeSet();
StyleConstants.setForeground(style2, Color.GREEN);
// Add some text
try
{
doc.insertString(doc.getLength(), "\nString Of Line 2", null );
doc.insertString(doc.getLength(), "\nString Of Line 3", style1);
doc.insertString(doc.getLength(), "\nString Of Line 4", style2);
doc.insertString(doc.getLength(), "\n", null );
}
catch(Exception e) { System.out.println(e); }
textPane.insertIcon (new ImageIcon(getClass().getResource("save.gif")));
getContentPane().add(textPane);
}
}
Output
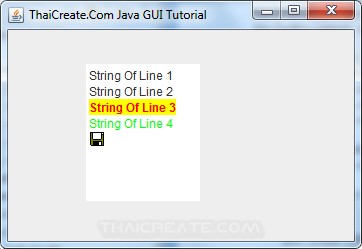
แสดง Text Pane แบบกราฟฟิก เช่น สี และสามารถแทรกรูปภาพได้
Example 3
MyForm.java การสร้าง Text Pane ด้วย JTextPane แบบมี Scroll Pane
package com.java.myapp;
import java.awt.Color;
import java.awt.EventQueue;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTextPane;
import javax.swing.text.SimpleAttributeSet;
import javax.swing.text.StyleConstants;
import javax.swing.text.StyledDocument;
public class MyForm extends JFrame {
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm frame = new MyForm();
frame.setVisible(true);
}
});
}
/**
* Create the frame.
*/
public MyForm() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 362, 249);
setTitle("ThaiCreate.Com Java GUI Tutorial");
getContentPane().setLayout(null);
// ScrollPane
JScrollPane scroll = new JScrollPane();
scroll.setBounds(100, 52, 130, 50);
// TextPane
JTextPane textPane = new JTextPane();
textPane.setText( "String Of Line 1" );
StyledDocument doc = textPane.getStyledDocument();
// Define a style1
SimpleAttributeSet style1 = new SimpleAttributeSet();
StyleConstants.setForeground(style1, Color.RED);
StyleConstants.setBackground(style1, Color.YELLOW);
StyleConstants.setBold(style1, true);
// Define a style2
SimpleAttributeSet style2 = new SimpleAttributeSet();
StyleConstants.setForeground(style2, Color.GREEN);
// Add some text
try
{
doc.insertString(doc.getLength(), "\nString Of Line 2", null );
doc.insertString(doc.getLength(), "\nString Of Line 3", style1);
doc.insertString(doc.getLength(), "\nString Of Line 4", style2);
doc.insertString(doc.getLength(), "\n", null );
}
catch(Exception e) { System.out.println(e); }
textPane.insertIcon (new ImageIcon(getClass().getResource("save.gif")));
scroll.setViewportView(textPane);
getContentPane().add(scroll);
}
}

Output
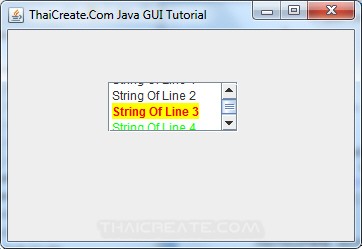
แสดง Text Pane แบบมี Scroll Pane
Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2013-09-03 22:06:17 /
2017-03-27 21:05:32 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|