Java and JSON Parser from URL Web Site (PHP/MySQL) |
Java and JSON Parser from URL Web Site (PHP/MySQL) ตัวอย่างนี้จะเป็นการ Apply การเขียน Java เพื่อทำการอ่านค่า JSON ที่อยู่ในรูปแบบของ URL จากฝั่งของ Server โดยในฝั่งของ Server จะทำงานด้วย PHP กับ MySQL Database และจะใช้ PHP อ่านแปลงค่าที่ได้จาก MySQL มาอยู่ในรูปแบบของ JSON จากนั้น Java จะใช้การอ่าน URL นั้น ๆ และนำค่า JSON ที่ได้มาใช้งาน
Example การเขียน Java เพื่ออ่านค่า JSON จาก URL ซึ่งได้มาจาก PHP และ MySQL Database
MySQL Database
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- dump ตาราง `customer`
--
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
โครงสร้างของ MySQL Database
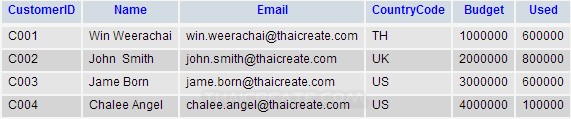
โครงสร้างของ MySQL Database
json.php ไฟล์ php สำหรับสร้าง JSON
<?php
$objConnect = mysql_connect("localhost","root","root") or die(mysql_error());
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM customer ";
$objQuery = mysql_query($strSQL) or die (mysql_error());
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
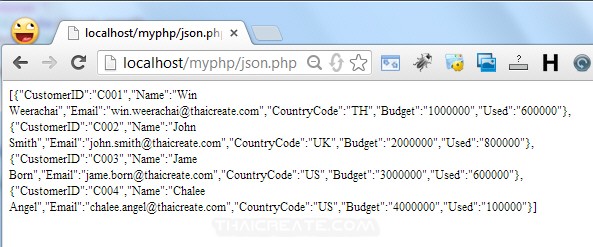
เมื่อเรียกไฟล์ PHP และ JSON Code ที่ได้
สร้างไฟล์ Java และเขียน Code เพื่ออ่านค่า JSON จาก URL
MyClass.java
package com.java.myapp;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import org.json.JSONArray;
import org.json.JSONObject;
public class MyClass {
public static void main(String[] args) {
URL url;
try {
url = new URL("http://localhost/myphp/json.php");
BufferedReader in = new BufferedReader(
new InputStreamReader(url.openStream()));
StringBuilder str = new StringBuilder();
String line;
while ((line = in.readLine()) != null)
str.append(line);
in.close();
// System.out.println(str);
/*** Write ***/
JSONArray data = new JSONArray(str.toString());
ArrayList<HashMap<String, String>> myArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("CustomerID", c.getString("CustomerID"));
map.put("Name", c.getString("Name"));
map.put("Email", c.getString("Email"));
map.put("CountryCode", c.getString("CountryCode"));
map.put("Budget", c.getString("Budget"));
map.put("Used", c.getString("Used"));
myArrList.add(map);
}
/*** Write Outout ***/
for (int i = 0; i < myArrList.size(); i++) {
String sCustomerID = myArrList.get(i).get("CustomerID").toString();
String sName = myArrList.get(i).get("Name").toString();
String sEmail = myArrList.get(i).get("Email").toString();
String sCountryCode = myArrList.get(i).get("CountryCode").toString();
String sBudget = myArrList.get(i).get("Budget").toString();
String sUsed = myArrList.get(i).get("Used").toString();
System.out.println("CustomerID = " + sCustomerID);
System.out.println("Name = " + sName);
System.out.println("Email = " + sEmail);
System.out.println("CountryCode = " + sCountryCode);
System.out.println("Budget = " + sBudget);
System.out.println("Used = " + sUsed);
System.out.println("=========================");
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
Output
CustomerID = C001
Name = Win Weerachai
Email = [email protected]
CountryCode = TH
Budget = 1000000
Used = 600000
=========================
CustomerID = C002
Name = John Smith
Email = [email protected]
CountryCode = UK
Budget = 2000000
Used = 800000
=========================
CustomerID = C003
Name = Jame Born
Email = [email protected]
CountryCode = US
Budget = 3000000
Used = 600000
=========================
CustomerID = C004
Name = Chalee Angel
Email = [email protected]
CountryCode = US
Budget = 4000000
Used = 100000
=========================

|