Example : JSP Insert data to Database (Java) |
Example : JSP Insert data to Database (Java) ตัวอย่างการเขียน JSP เพื่อติดต่อกับ Database และตัวอย่างการ Insert ข้อมูลจาก JSP ลงใน Database โดยในขั้นแรกจะใช้การออกแบบ Form เพื่อรับค่า Input ค่าต่าง ๆ จาก User และหลังจากที่ User ทำการ Submit ข้อมูลเหล่านั้นแล้ว เราก็จะใช้ JSP อ่านข้อมูลจาก Input แล้วค่อยนำไป Insert ใน Database
Example ตัวอย่างการเขียน JSP เพื่อ ทำการ Insert เพิ่มข้อมูลลงใน Database
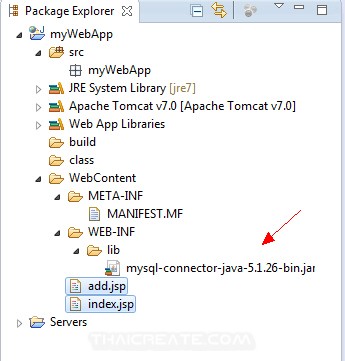
เนื่องจากใช้ Database ของ MySQL จึงเลือกใช้ MySQL Connector ด้วยการเรียกไฟล์ jar
mysql
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- dump ตาราง `customer`
--
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
โครงสร้างของ Table และ Data
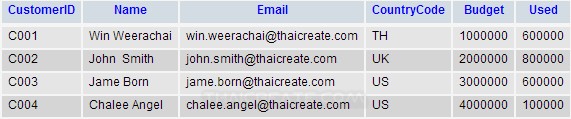
ฐานข้อมูลของ MySQL
index.jsp
<%@ page import="java.sql.ResultSet" %>
<%@ page import="java.sql.SQLException" %>
<%@ page import="java.sql.Statement" %>
<%@ page import="java.sql.Connection" %>
<%@ page import="java.sql.DriverManager" %>
<html>
<head>
<title>ThaiCreate.Com JSP Tutorial</title>
</head>
<body>
<form name="frmAdd" method="post" action="add.jsp">
Add Form
<table width="374" border="1">
<tr>
<th width="140">
<div align="left">CustomerID </div></th>
<td width="272"><input type="text" name="txtCustomerID" size="5"></td>
</tr>
<tr>
<th width="140">
<div align="left">Name </div></th>
<td><input type="text" name="txtName" size="20"></td>
</tr>
<tr>
<th width="140">
<div align="left">Email </div></th>
<td><input type="text" name="txtEmail" size="20"></td>
</tr>
<tr>
<th width="140">
<div align="left">CountryCode </div></th>
<td><input type="text" name="txtCountryCode" size="2"></td>
</tr>
<tr>
<th width="140">
<div align="left">Budget </div></th>
<td><input type="text" name="txtBudget" size="5"></td>
</tr>
<tr>
<th width="140">
<div align="left">Used </div></th>
<td><input type="text" name="txtUsed" size="5"></td>
</tr>
</table>
<input type="submit" value="Save">
</form>
</body>
</html>
add.jsp
<%@ page import="java.sql.ResultSet" %>
<%@ page import="java.sql.SQLException" %>
<%@ page import="java.sql.Statement" %>
<%@ page import="java.sql.Connection" %>
<%@ page import="java.sql.DriverManager" %>
<html>
<head>
<title>ThaiCreate.Com JSP Tutorial</title>
</head>
<body>
<%
String CusID = "";
if(request.getParameter("CustomerID") != null) {
CusID = request.getParameter("CustomerID");
}
Connection connect = null;
Statement s = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
s = connect.createStatement();
String strCustomerID = request.getParameter("txtCustomerID");
String strName = request.getParameter("txtName");
String strEmail = request.getParameter("txtEmail");
String strCountryCode = request.getParameter("txtCountryCode");
float intBudget = Float.valueOf(request.getParameter("txtBudget"));
float intUsed = Float.valueOf(request.getParameter("txtUsed"));
String sql = "INSERT INTO customer " +
"(CustomerID,Name,Email,CountryCode,Budget,Used) " +
"VALUES ('" + strCustomerID + "','" + strName + "' " +
",'" + strEmail + "','" + strCountryCode + "'" +
",'" + intBudget + "','" + intUsed + "') ";
s.execute(sql);
out.println("Record Inserted Successfully");
} catch (Exception e) {
// TODO Auto-generated catch block
out.println(e.getMessage());
e.printStackTrace();
}
try {
if(s!=null){
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
out.println(e.getMessage());
e.printStackTrace();
}
%>
</body>
</html>

Output
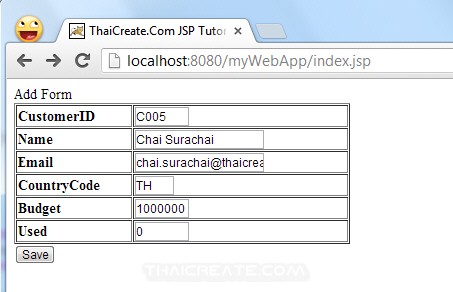
Form สำหรับรับข้อมูล
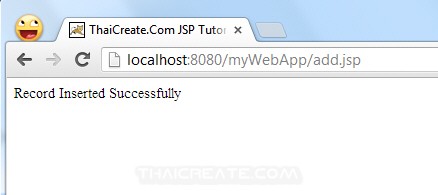
แสดงสถานะการ Insert
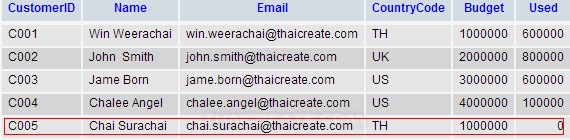
เมื่อกลับไปดูที่ Database ข้อมูลถูกเพิ่มเรียบร้อยแล้ว
อ่านเพิ่มเติมเกี่ยวกับ JSP และการติดต่อกับ Database ต่าง ๆ
|