Example : JSP Search data from Database (Java) |
Example : JSP Search data from Database (Java) ตัวอย่างการเขียน JSP ติดต่อกับ Database และทำการค้นหา (Search) ข้อมูลจาก Database จากนั้นหลังจากที่ได้ผลลัพธ์แล้วจะทำการ Loop ข้อมูลใน HTML Table เพื่อแสดงผลลัพธ์ออกทางหน้าจอของไฟล์ JSP
Example ตัวอย่างการเขียน JSP เพื่อค้นหา (Search) ข้อมูลจาก Database มาแสดง
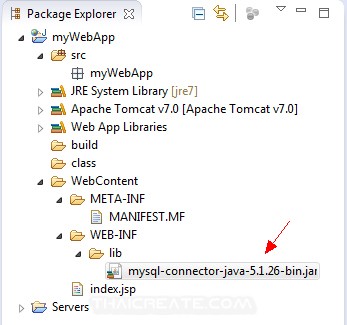
เนื่องจากใช้ Database ของ MySQL จึงเลือกใช้ MySQL Connector ด้วยการเรียกไฟล์ jar
mysql
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- dump ตาราง `customer`
--
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
โครงสร้างของ Table และ Data
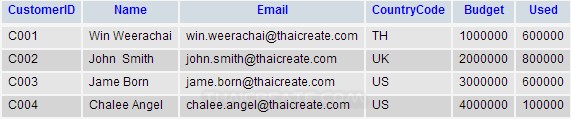
ฐานข้อมูลของ MySQL
index.jsp
<%@ page import="java.sql.ResultSet" %>
<%@ page import="java.sql.SQLException" %>
<%@ page import="java.sql.Statement" %>
<%@ page import="java.sql.Connection" %>
<%@ page import="java.sql.DriverManager" %>
<html>
<head>
<title>ThaiCreate.Com JSP Tutorial</title>
</head>
<body>
<%
String keyword = "";
if(request.getParameter("txtKeyword") != null) {
keyword = request.getParameter("txtKeyword");
}
%>
<form name="frmSearch" method="get" action="index.jsp">
<table width="599" border="1">
<tr>
<th>Keyword
<input name="txtKeyword" type="text" id="txtKeyword" value="<%=keyword%>">
<input type="submit" value="Search"></th>
</tr>
</table>
</form>
<%
Connection connect = null;
Statement s = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
s = connect.createStatement();
String sql = "SELECT * FROM customer WHERE Name like '%" + keyword + "%' " +
" ORDER BY CustomerID ASC";
System.out.println(sql);
ResultSet rec = s.executeQuery(sql);
%>
<table width="600" border="1">
<tr>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="98"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="59"> <div align="center">Budget </div></th>
<th width="71"> <div align="center">Used </div></th>
</tr>
<%while((rec!=null) && (rec.next())) { %>
<tr>
<td><div align="center"><%=rec.getString("CustomerID")%></div></td>
<td><%=rec.getString("Name")%></td>
<td><%=rec.getString("Email")%></td>
<td><div align="center"><%=rec.getString("CountryCode")%></div></td>
<td align="right"><%=rec.getFloat("Budget")%></td>
<td align="right"><%=rec.getFloat("Used")%></td>
</tr>
<%}%>
</table>
<%
} catch (Exception e) {
// TODO Auto-generated catch block
out.println(e.getMessage());
e.printStackTrace();
}
try {
if(s!=null){
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
out.println(e.getMessage());
e.printStackTrace();
}
%>
</body>
</html>
Output
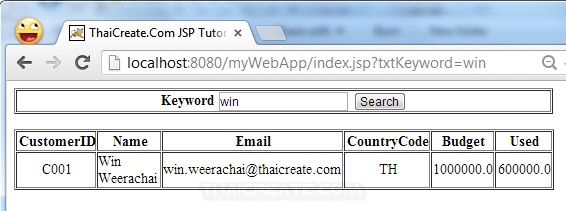
อ่านเพิ่มเติมเกี่ยวกับ JSP และการติดต่อกับ Database ต่าง ๆ

|