Java MySQL Database and PreparedStatement/Parameters Query (JDBC) |
Java MySQL Database and PreparedStatement/Parameters Query (JDBC) การนำ PreparedStatement ในการเขียนโปรแกรมด้วยภาษา Java กับ MySQL มาช่วยในการเขียน SQL Statement จะช่วยให้การจัดการกับ Query นั้นมีประสิทธิภาพสูงขึ้นมาก เพราะ PreparedStatement เป็นการสร้าง Object ของ SQL Statement ขึ้นมา 1 ชุด ที่สามารถนำไปใช้งานได้หลายครั้ง อีกทั้งยังสามารถใช้ตรวจสอบความถูกต้องต้องข้อมูลได้ด้วย เช่นในแต่ล่ะ Parameters สามารถกำหนดชนิด Data Type ของข้อมูลได้ เช่น เป็นแบบ INTEGER ก็ใช้ setInt เป็นแบบ STRING ก็ใช้ setString และในความสามารถของการทำงานจะมี Performance ดีกว่าการเขียน Query ธรรมดา ทั้งในด้านความเร็ว ด้าน Secure ที่ช่วยป้องกัน SQL Injection อัตโนมัติ
PreparedStatement pstmt = con.prepareStatement("INSERT INTO EMPLOYEES
(ID,SALARY) VALUES (?,?)");
pstmt.setInt(1, 101)
pstmt.setBigDecimal(2, 110592)
รูปแบบการเขียน PreparedStatement บนภาษา Java จะใช้เครื่องหมาย ? เป็นตำแหน่งของ Parameters โดยสามารถกำหนด Parameters ตามตำแหน่งโดยเริ่มจาก 1 ซึ่งรองรับ Data Type ต่าง ๆ เช่น
setNull ( index, null )
setBoolean ( index, boolean )
setByte ( index, byte )
setDate ( index, date )
setTime ( index, time )
setInt ( index, int )
setLong ( index, long )
setShort ( index, short )
setFloat ( index, float )
setDouble ( index, double )
setString ( index, string )
setObject ( index, object )
การนำ PreparedStatement มาใช้งานหลายครั้ง
PreparedStatement pstmt = con.prepareStatement("INSERT INTO EMPLOYEES
(ID,SALARY) VALUES (?,?)");
// Query 1
pstmt.setInt(1, 101)
pstmt.setBigDecimal(2, 110592)
pstmt.executeUpdate();
// Query 2
pstmt.setInt(1, 102)
pstmt.setBigDecimal(2, 150592)
pstmt.executeUpdate();
จากตัวอย่างจะเห้นว่า Object ของ PreparedStatement สามารถนำมาใช้งานได้หลาย ๆ ครั้ง
พื้นฐาน Java Connect to MySQL Database (JDBC)
Syntax for INSERT / UPDATE / DELETE
PreparedStatement pre = connect.prepareStatement(sql);
สร้างชนิด Object ของ PreparedStatement เพื่อไว้สำหรับเก็บสร้าง Query และ Parameters
String sql = "INSERT INTO customer " +
"(CustomerID,Name,Email,CountryCode,Budget,Used) " +
"VALUES (?,?,?,?,?,?) ";
pre.setString(1, "C005");
pre.setString(2, "Chai Surachai");
pre.setString(3, "[email protected]");
pre.setString(4, "TH");
pre.setFloat(5, 1000000);
pre.setFloat(6, 0);
สร้างชุดคำสั่งของ SQL และการเพิ่ม Parameters ในแต่ล่ะตำแหน่ง และ ในแต่ล่ะชนิดของข้อมูล
pre.executeUpdate();
Execute เพื่อ Query ตัว PreparedStatement
Syntax for SELECT
PreparedStatement pre = connect.prepareStatement(sql);
สร้างชนิด Object ของ PreparedStatement เพื่อไว้สำหรับเก็บสร้าง Query และ Parameters
String sql = "SELECT * FROM customer " +
"WHERE CountryCode = ? ORDER BY CustomerID ASC";
pre = connect.prepareStatement(sql);
pre.setString(1, "TH");
สร้างชุดคำสั่งของ SQL และการเพิ่ม Parameters ในแต่ล่ะตำแหน่ง และ ในแต่ล่ะชนิดของข้อมูล
ResultSet rec = pre.executeQuery();
Execute เพื่อ Query ตัว PreparedStatement ซึ่งจะได้เป็น ResultSet สามารถที่จะนำ Result ที่ได้ไปใช้ได้ต่อ
รูปแบบการ Query ด้วยการ PreparedStatement ในภาษา Java กับ Databases ของ MySQL
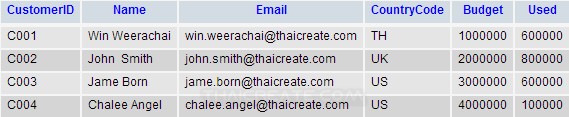
Example 1 ตัวอย่างการเขียน Query แบบ PreparedStatement เพื่อ SELECT ข้อมูล MySQL
MyClass.java
package com.java.myapp;
import java.sql.PreparedStatement;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
public class MyClass {
public static void main(String[] args) {
Connection connect = null;
PreparedStatement pre = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
String sql = "SELECT * FROM customer " +
"WHERE CountryCode = ? ORDER BY CustomerID ASC";
pre = connect.prepareStatement(sql);
pre.setString(1, "TH");
ResultSet rec = pre.executeQuery();
while((rec!=null) && (rec.next()))
{
System.out.print(rec.getString("CustomerID"));
System.out.print(" - ");
System.out.print(rec.getString("Name"));
System.out.print(" - ");
System.out.print(rec.getString("Email"));
System.out.print(" - ");
System.out.print(rec.getString("CountryCode"));
System.out.print(" - ");
System.out.print(rec.getFloat("Budget"));
System.out.print(" - ");
System.out.print(rec.getFloat("Used"));
System.out.println("");
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
try {
pre.close();
connect.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}

Example 2 ตัวอย่างการเขียน Query แบบ PreparedStatement เพื่อ INSERT ข้อมูล MySQL
MyClass.java
package com.java.myapp;
import java.sql.PreparedStatement;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class MyClass {
public static void main(String[] args) {
Connection connect = null;
PreparedStatement pre = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
String sql = "INSERT INTO customer " +
"(CustomerID,Name,Email,CountryCode,Budget,Used) " +
"VALUES (?,?,?,?,?,?) ";
pre = connect.prepareStatement(sql);
pre.setString(1, "C005");
pre.setString(2, "Chai Surachai");
pre.setString(3, "[email protected]");
pre.setString(4, "TH");
pre.setFloat(5, 1000000);
pre.setFloat(6, 0);
pre.executeUpdate();
System.out.println("Record Inserted Successfully");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Close
try {
if(connect != null){
pre.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
Example 3 ตัวอย่างการเขียน Query แบบ PreparedStatement เพื่อ UPDATE ข้อมูล MySQL
MyClass.java
package com.java.myapp;
import java.sql.PreparedStatement;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class MyClass {
public static void main(String[] args) {
Connection connect = null;
PreparedStatement pre = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
String sql = "UPDATE customer " +
"SET Budget = ? " +
" WHERE CustomerID = ? ";
pre = connect.prepareStatement(sql);
pre.setFloat(1, 5000000);
pre.setString(2, "C005");
pre.executeUpdate();
System.out.println("Record Update Successfully");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Close
try {
if(connect != null){
pre.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
Example 4 ตัวอย่างการเขียน Query แบบ PreparedStatement เพื่อ DELETE ข้อมูล MySQL
MyClass.java
package com.java.myapp;
import java.sql.PreparedStatement;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class MyClass {
public static void main(String[] args) {
Connection connect = null;
PreparedStatement pre = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
String sql = "DELETE FROM customer " +
" WHERE CustomerID = ? ";
pre = connect.prepareStatement(sql);
pre.setString(1, "C005");
pre.executeUpdate();
System.out.println("Record Delete Successfully");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Close
try {
if(connect != null){
pre.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
อ่านเพิ่มเติม
Java Parameters Query for Select (prepareStatement)
Java Parameters Query for Insert (prepareStatement)
Java Parameters Query for Update (prepareStatement)
Java Parameters Query for Delete (prepareStatement)
|