Java Example PDF Report from Database |
Java Example PDF Report from Database ตัวอย่างนี้จะเป็นการเขียน Java เพื่อ Create สร้างไฟล์ให้อยู่ในรูปแบบ PDF โดยจะทำการอ่านข้อมูลที่อยู่ใน Database ด้วยการ Query ข้อมูลจาก Table และหลังจากที่ได้ข้อมูลจาก Table แล้วก็จะใช้การ Loop เพื่อสร้างเอกสารให้อยู่ในรูปแบบของไฟล์ PDF ซึ่งเหมาะกับไว้การทำพวก Report
Example การเขียน Java เพื่อสร้าง PDF ไฟล์ ซึ่งอ่านค่ามาจาก Database
MySQL Database
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- dump ตาราง `customer`
--
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
โครงสร้างของ MySQL Database
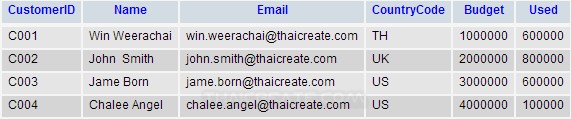
MyClass.java
package com.java.myapp;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.PDPage;
import org.apache.pdfbox.pdmodel.edit.PDPageContentStream;
import org.apache.pdfbox.pdmodel.font.PDFont;
import org.apache.pdfbox.pdmodel.font.PDType1Font;
public class MyClass {
public static String[][] append(String[][] a, String[][] b) {
String[][] result = new String[a.length + b.length][];
System.arraycopy(a, 0, result, 0, a.length);
System.arraycopy(b, 0, result, a.length, b.length);
return result;
}
public static void main(String[] args) {
Connection connect = null;
Statement s = null;
String[][] content = {{"CustomerID","Name", "Email",
"CountryCode", "Budget", "Used"}} ;
try {
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
s = connect.createStatement();
String sql = "SELECT * FROM customer ORDER BY CustomerID ASC";
ResultSet rec = s.executeQuery(sql);
while((rec!=null) && (rec.next()))
{
String[][] data = {{rec.getString("CustomerID")
,rec.getString("Name")
,rec.getString("Email")
,rec.getString("CountryCode")
,rec.getString("Budget")
,rec.getString("Used")}};
content = append(content,data);
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Close
try {
if(connect != null){
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
try {
PDDocument doc = new PDDocument();
PDPage page = new PDPage();
doc.addPage( page );
PDPageContentStream contentStream =
new PDPageContentStream(doc, page);
// Create a new font object selecting one of the PDF base fonts
PDFont font = PDType1Font.HELVETICA_BOLD;
// Define a text content stream using the selected font, moving the cursor and drawing the text "Hello World"
contentStream.beginText();
contentStream.setFont( font, 12 );
contentStream.moveTextPositionByAmount( 30, 700 );
contentStream.drawString( "Customer Report" );
contentStream.endText();
drawTable(page, contentStream, 690, 30, content);
contentStream.close();
doc.save("test.pdf" );
// Save the results and ensure that the document is properly closed:
doc.save( "C:\\java\\myPDF.pdf");
doc.close();
System.out.println("PDF Created Done.");
} catch (Exception e) {
e.printStackTrace();
}
}
public static void drawTable(PDPage page,
PDPageContentStream contentStream, float y, float margin,
String[][] content) throws IOException {
final int rows = content.length;
final int cols = content[0].length;
final float rowHeight = 20f;
final float tableWidth = page.findMediaBox().getWidth() - (2 * margin);
final float tableHeight = rowHeight * rows;
final float colWidth = tableWidth / (float) cols;
final float cellMargin = 2f;
// draw the rows
float nexty = y;
for (int i = 0; i <= rows; i++) {
contentStream.drawLine(margin, nexty, margin + tableWidth, nexty);
nexty -= rowHeight;
}
// draw the columns
float nextx = margin;
for (int i = 0; i <= cols; i++) {
contentStream.drawLine(nextx, y, nextx, y - tableHeight);
nextx += colWidth;
}
// now add the text
contentStream.setFont(PDType1Font.HELVETICA_BOLD, 6);
float textx = margin + cellMargin;
float texty = y - 15;
for (int i = 0; i < content.length; i++) {
for (int j = 0; j < content[i].length; j++) {
String text = content[i][j];
contentStream.beginText();
contentStream.moveTextPositionByAmount(textx, texty);
contentStream.drawString(text);
contentStream.endText();
textx += colWidth;
}
texty -= rowHeight;
textx = margin + cellMargin;
}
}
}

Output
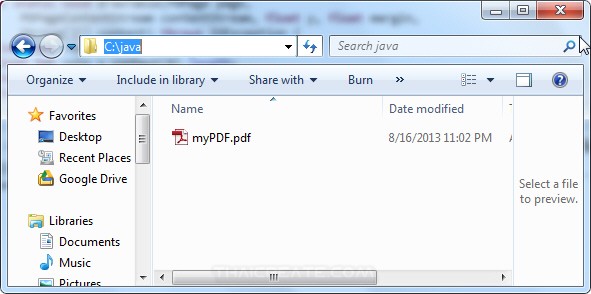
ไฟล์ PDF ถูกสร้างแล้ว
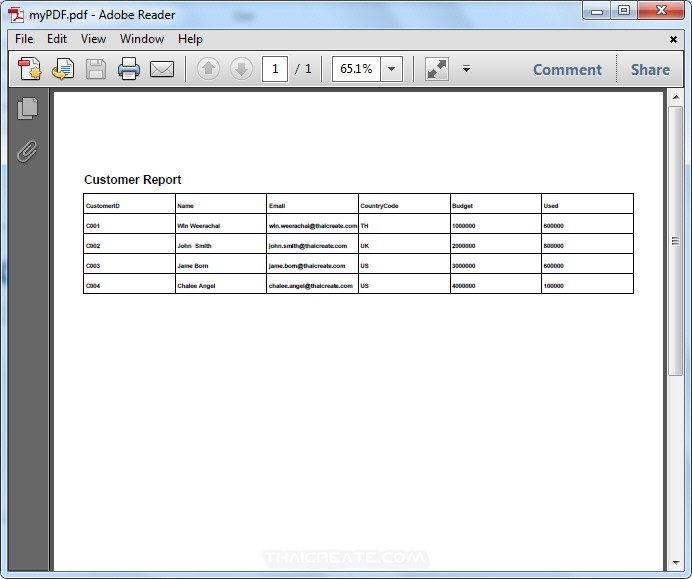
ตัวอย่าง Report
|