Java Read and Get CSV file |
Java Read and Get CSV file บทความแรกจะเป็นการเขียน Java เพื่ออ่าน CSV ไฟล์ โดยจะมี 2 ตัวอย่าง คือ ตัวอย่างแรกจะใช้การอ่านข้อมูลเหมือนกับ Text file เพียงแต่เมื่อได้ข้อมูลแต่ล่ะ Line จะใช้การ Split ด้วยเครื่องหมายคอมมา (,) เพื่อแบ่งข้อมูลแต่ล่ะ Column จากนั้นใช้การแสดงผลออกทางหน้าจอ ส่วนตัวอย่างที่ 2 จะมีการใช้ Library ไฟล์ jar เข้ามาช่วยในการอ่านไฟล์ CSV
thaicreate.csv
C001,Win Weerachai,[email protected],TH,1000000,600000
C002,John Smith,[email protected],UK,2000000,800000
C003,Jame Born,[email protected],US,3000000,600000
C004,Chalee Angel,[email protected],US,4000000,100000
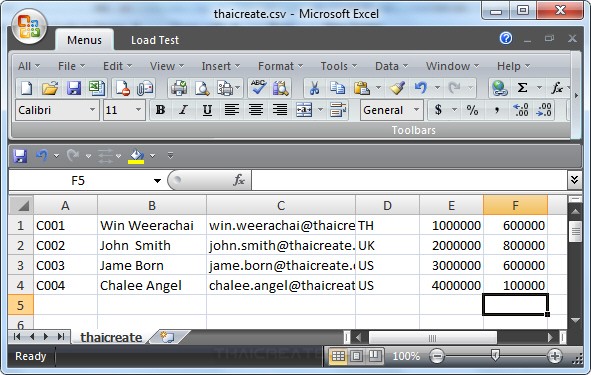
ไฟล์ CSV เมื่อเปิดผ่านโปรแกรม Excel
Example 1 การอ่านโดยใช้ java.io เหมือนกับการอ่าน Text file
MyClass.java
package com.java.myapp;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class MyClass {
public static void main(String[] args) {
String path = "C:\\java\\thaicreate.csv";
File file = new File(path);
try {
BufferedReader br = new BufferedReader(new FileReader(file));
String line;
while ((line = br.readLine()) != null) {
String[] arr = line.split(",");
System.out.print(arr[0]);
System.out.print(" - ");
System.out.print(arr[1]);
System.out.print(" - ");
System.out.print(arr[2]);
System.out.print(" - ");
System.out.print(arr[3]);
System.out.print(" - ");
System.out.print(arr[4]);
System.out.print(" - ");
System.out.print(arr[5]);
System.out.println("");
}
br.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
Output
Example 2 การอ่านโดยใช้ Library ไฟล์ .jar ของ opencsv โดยในขั้นแรกจะต้องทำการ Download ตัว Library และนำมา Import เข้ามาใน Project ซะก่อนถึงจะใช้งานได้
Dpwnload opencsv
การ Add Jar Library ใช้งาน External Library บน Eclipse IDE ในการเขียน Java
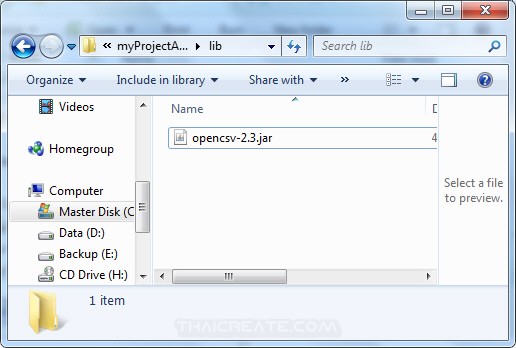
หลังจากที่ได้ไฟล์มาแล้วให้ Copy ไปไว้ในโฟเดอร์ lib ของโปรเจค (ถ้าไม่มีให้สร้างมาใหม่)
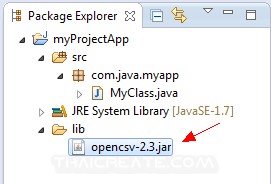
ไฟล์ jar อยู่ในโฟเดอร์ lib

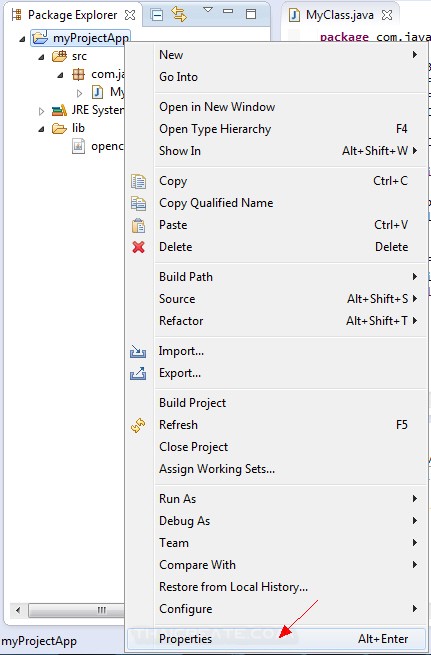
คลิกขวาที่ Project เลือก Properties
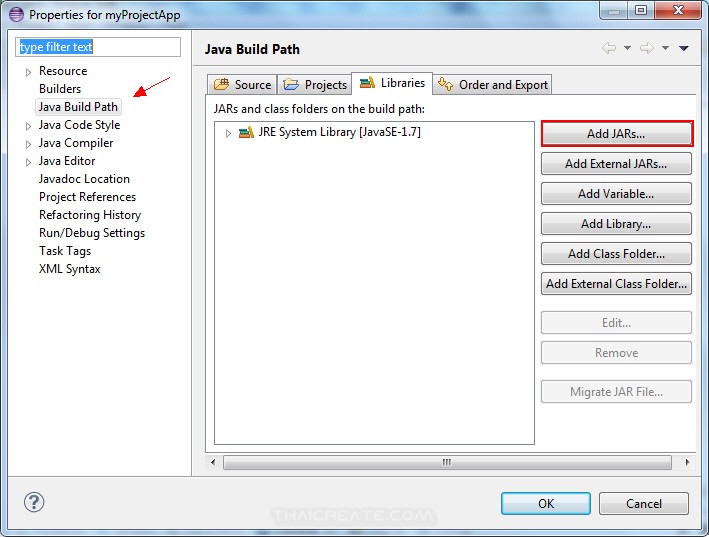
ในส่วนของ Java Build Path ให้เลือก Add JARs...
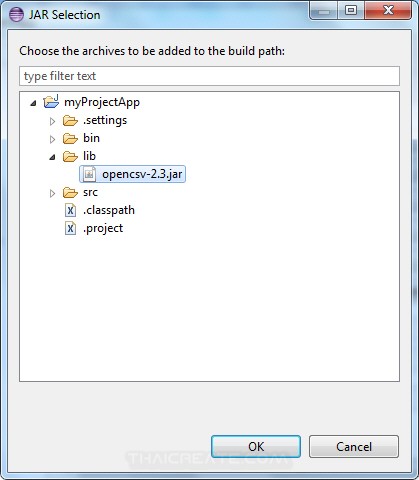
เลือกไฟล์ jar ที่ต้องการ
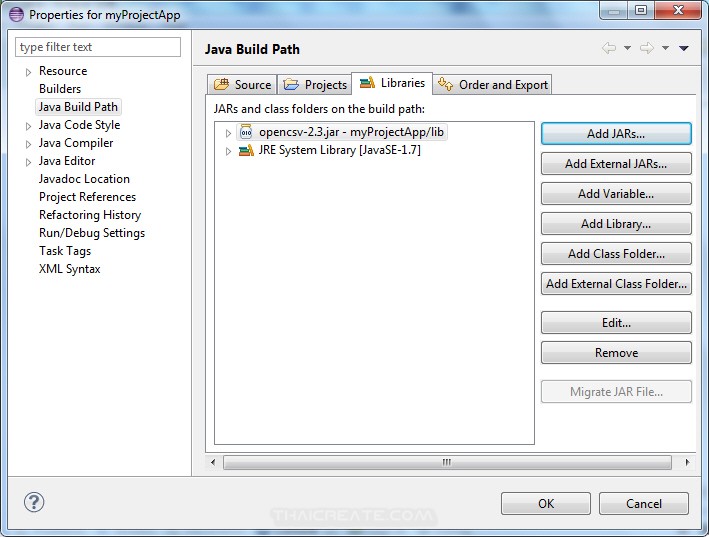
ถูก Add เข้ามาใน Project เรียบร้อยแล้ว จากนั้นเราก็สามารถเรียกใช้งาน Library ของ jar ได้แล้ว
MyClass.java
package com.java.myapp;
import java.io.FileReader;
import java.io.IOException;
import au.com.bytecode.opencsv.CSVReader;
public class MyClass {
public static void main(String[] args) {
String path = "C:\\java\\thaicreate.csv";
try {
CSVReader reader = new CSVReader(new FileReader(path));
String [] nextLine;
while ((nextLine = reader.readNext()) != null) {
System.out.print(nextLine[0]);
System.out.print(" - ");
System.out.print(nextLine[1]);
System.out.print(" - ");
System.out.print(nextLine[2]);
System.out.print(" - ");
System.out.print(nextLine[3]);
System.out.print(" - ");
System.out.print(nextLine[4]);
System.out.print(" - ");
System.out.print(nextLine[5]);
System.out.println("");
}
reader.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
Output
สิ่งที่ได้จากการเรียกใช้งาน Library ของไฟล์ jar ซึ่งก็ไม่ค่อยแตกต่างเท่าไหร่นัก
.
|