Java Create XML File from Database |
Java Create XML File from Database ตัวอย่างนี้จะเป็นการเขียน Java เพื่อ Create สร้างไฟล์ให้อยู่ในรูปแบบ XML โดยจะทำการอ่านข้อมูลที่อยู่ใน Database ด้วยการ Query ข้อมูลจาก Table และหลังจากที่ได้ข้อมูลจาก Table แล้วก็จะใช้การ Loop เพื่อสร้างเอกสารให้อยู่ในรูปแบบของ XML
Example การเขียน Java เพื่อสร้าง XML ไฟล์ ซึ่งอ่านค่ามาจาก Database
MySQL Database
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- dump ตาราง `customer`
--
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
โครงสร้างของ MySQL Database
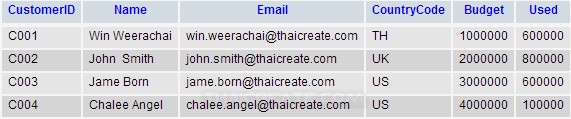
MyClass.java
package com.java.myapp;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.transform.OutputKeys;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import org.w3c.dom.Attr;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import java.io.File;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class MyClass {
public static void main(String[] args) {
try {
// Connect Database
Connection connect = null;
Statement s = null;
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
s = connect.createStatement();
String sql = "SELECT * FROM customer ORDER BY CustomerID ASC";
ResultSet rec = s.executeQuery(sql);
String strPath = "C:\\java\\myData.xml";
DocumentBuilderFactory documentFactory = DocumentBuilderFactory
.newInstance();
DocumentBuilder documentBuilder = documentFactory
.newDocumentBuilder();
// define root elements
Document doc = documentBuilder.newDocument();
Element ele = doc.createElement("customer");
doc.appendChild(ele);
int iRows = 0;
while((rec!=null) && (rec.next()))
{
++iRows;
// Row
Element sRows = doc.createElement("Rows");
ele.appendChild(sRows);
// Attributes
Attr sAttrID = doc.createAttribute("id");
sAttrID.setValue(String.valueOf(iRows));
sRows.setAttributeNode(sAttrID);
// CustomerID
Element sCustomerID = doc.createElement("CustomerID");
sCustomerID.appendChild(doc.createTextNode(rec.getString("CustomerID")));
sRows.appendChild(sCustomerID);
// Name
Element sName = doc.createElement("Name");
sName.appendChild(doc.createTextNode(rec.getString("Name")));
sRows.appendChild(sName);
// Email
Element sEmail = doc.createElement("Email");
sEmail.appendChild(doc.createTextNode(rec.getString("Email")));
sRows.appendChild(sEmail);
// CountryCode
Element sCountryCode = doc.createElement("CountryCode");
sCountryCode.appendChild(doc.createTextNode(rec.getString("CountryCode")));
sRows.appendChild(sCountryCode);
// Budget
Element sBudget = doc.createElement("Budget");
sBudget.appendChild(doc.createTextNode(rec.getString("Budget")));
sRows.appendChild(sBudget);
// Used
Element sUsed = doc.createElement("Used");
sUsed.appendChild(doc.createTextNode(rec.getString("Used")));
sRows.appendChild(sUsed);
}
// creating and writing to xml file
TransformerFactory tff = TransformerFactory
.newInstance();
Transformer tf = tff.newTransformer();
DOMSource domSource = new DOMSource(doc);
StreamResult streamResult = new StreamResult(new File(strPath));
tf.setOutputProperty(
"{http://xml.apache.org/xslt}indent-amount", "2");
tf.setOutputProperty(OutputKeys.INDENT, "yes");
tf.transform(domSource, streamResult);
System.out.println("XML file created!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<customer>
<Rows id="1">
<CustomerID>C001</CustomerID>
<Name>Win Weerachai</Name>
<Email>[email protected]</Email>
<CountryCode>TH</CountryCode>
<Budget>1000000</Budget>
<Used>600000</Used>
</Rows>
<Rows id="2">
<CustomerID>C002</CustomerID>
<Name>John Smith</Name>
<Email>[email protected]</Email>
<CountryCode>UK</CountryCode>
<Budget>2000000</Budget>
<Used>800000</Used>
</Rows>
<Rows id="3">
<CustomerID>C003</CustomerID>
<Name>Jame Born</Name>
<Email>[email protected]</Email>
<CountryCode>US</CountryCode>
<Budget>3000000</Budget>
<Used>600000</Used>
</Rows>
<Rows id="4">
<CustomerID>C004</CustomerID>
<Name>Chalee Angel</Name>
<Email>[email protected]</Email>
<CountryCode>US</CountryCode>
<Budget>4000000</Budget>
<Used>100000</Used>
</Rows>
</customer>

|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2013-05-26 11:50:09 /
2013-08-19 10:26:58 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|