.fadeTo() - Effects , jQuery |
.fadeTo() เป็นการใช้ Effects กับ .fadeTo() ใช้ในการปรับหรือแสดงความชัดของ element ที่อ้างถึง ในรูปแบบต่าง ๆ
Syntax
.fadeTo( duration, opacity, [callback] )
.fadeTo( duration, opacity, [easing,] [callback] )
Example 1 ตัวอย่างการใช้งาน .fadeTo()
EffectsfadeTo1.html
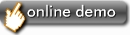
<html>
<head>
<title>ThaiCreate.Com jQuery Tutorials</title>
<script type="text/javascript" src="jquery-1.6.4.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$("p:first").click(function () {
$(this).fadeTo("slow", 0.33);
});
});
</script>
</head>
<body>
<p>
Click this paragraph to see it fade.
</p>
<p>
Compare to this one that won't fade.
</p>
</body>
</html>
Screenshot
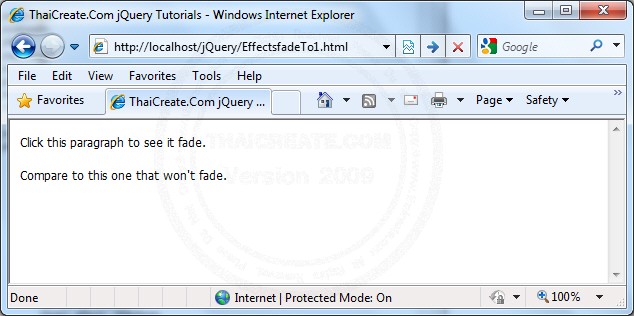
คำอธิบาย (ภาษาไทย)
จากตัวอย่างเป็นการใช้ Effects กับ .fadeTo() ในการจัดการกับ element ที่อ้างถึง และเทคนิคการใช้ fadeTo
Example 2 ตัวอย่างการใช้งาน .fadeTo()
EffectsfadeTo2.html
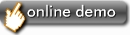
<html>
<head>
<title>ThaiCreate.Com jQuery Tutorials</title>
<style>
p { width:80px; margin:0; padding:5px; }
div { width:40px; height:40px; position:absolute; }
div#one { top:0; left:0; background:#f00; }
div#two { top:20px; left:20px; background:#0f0; }
div#three { top:40px; left:40px; background:#00f; }
</style>
<script type="text/javascript" src="jquery-1.6.4.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$("div").click(function () {
$(this).fadeTo("fast", Math.random());
});
});
</script>
</head>
<body>
<p>And this is the library that John built...</p>
<div id="one"></div>
<div id="two"></div>
<div id="three"></div>
</body>
</html>
Screenshot
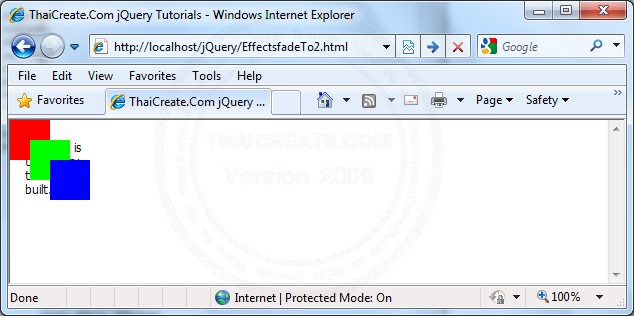
คำอธิบาย (ภาษาไทย)
จากตัวอย่างเป็นการใช้ Effects กับ .fadeTo() ในการจัดการกับ element ที่อ้างถึง และ การใช้ fadeTo ในรูปแบบต่าง ๆ ให้คลิกที่ Demo เพื่อดูผลลัพธ์
Example 3 ตัวอย่างการใช้งาน .fadeTo()
EffectsfadeTo3.html
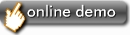
<html>
<head>
<title>ThaiCreate.Com jQuery Tutorials</title>
<style>
div, p { width:80px; height:40px; top:0; margin:0;
position:absolute; padding-top:8px; }
p { background:#fcc; text-align:center; }
div { background:blue; }
</style>
<script type="text/javascript" src="jquery-1.6.4.js"></script>
<script type="text/javascript">
$(document).ready(function(){
var getPos = function (n) {
return (Math.floor(n) * 90) + "px";
};
$("p").each(function (n) {
var r = Math.floor(Math.random() * 3);
var tmp = $(this).text();
$(this).text($("p:eq(" + r + ")").text());
$("p:eq(" + r + ")").text(tmp);
$(this).css("left", getPos(n));
});
$("div").each(function (n) {
$(this).css("left", getPos(n));
})
.css("cursor", "pointer")
.click(function () {
$(this).fadeTo(250, 0.25, function () {
$(this).css("cursor", "")
.prev().css({"font-weight": "bolder",
"font-style": "italic"});
});
});
});
</script>
</head>
<body>
<p>Wrong</p>
<div></div>
<p>Wrong</p>
<div></div>
<p>Right!</p>
<div></div>
</body>
</html>
Screenshot
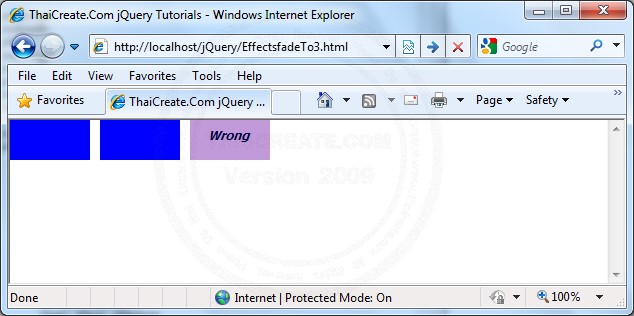
คำอธิบาย (ภาษาไทย)
จากตัวอย่างเป็นการใช้ Effects กับ .fadeTo() ในการจัดการกับ element ที่อ้างถึง รวมทั้งตัวอย่างการใช้ fadeTo ในรูปแบบจ่าง ๆ

ลิ้งค์ที่ควรศึกษา
Go to : jQuery Selectors : jQuery Selectors and Element
Go to : jQuery Effects : jQuery and Effects
เกี่ยวกับบทความ
ส่วนหนึ่งของบทความได้เรียบเรียงและแปลจากเว็บไซต์ jQuery.Com โค้ดตัวอย่างคำสั่งนี้อยู่ภายใต้สัญญาอนุญาตของ GFDL สามารถนำโค้ดและคำสั่งใช้งานได้ฟรี สงวนลิขสิทธิ์เฉพาะคำอธิบายภาษาไทย
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2011-09-22 21:43:28 /
2017-03-19 14:06:53 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|