Android and Asynchronous Http Client - com.loopj : (HTTP LIB:Library) |
Android and Asynchronous Http Client - com.loopj : (HTTP LIB:Library) ยังมีอีก Library อีกหลายตัวที่น่าสนใจในการเขียน Android App เชื่อมต่อกับ Http หรือข้อมูลในรูปแบบของ Web Server กับ Client โดยในบทความจะแนะนำ Asynchronous Http Client เป็นไลบรารี่ตัวนี้ค่อนข้างจะทำงานได้ดีในระดับหนึ่งถึงดีมาก ความสามารถพื้นฐานรองรับการการรับ-ส่งข้อมูลได้เกือบทุกรูปแบบไม่น่าจะเป็นในรูปแบบ Get และ Post สามารถส่งได้ทั้งที่เป็น String , Multipart File , Streaming และอื่น ๆ อีกหลายฟีเจอร์ สั่งความสามารถพิเศษที่น่าจะสนใจ คือจะใช้ Gson ทั้งการ รับและส่ง ได้เช่นเดียวกัน
Android and Asynchronous Http Client - com.loopj
ในการเขียน Android App ช่วงหลัง ๆ ผมจึงแนะนำให้ใช้ Library มาเข้าจัดการกับเรื่องเหล่านี้แทน ซึ่งในปัจจุบันมี Library หลายตัวมาก ที่เข้ามาจัดการกับ Http โดยเฉพาะ รองรับการทำงานเกือบทุกรูปแบบ ไม่ว่าจะเป็น get, post การรับส่ง string , files หรือแม้แต่ json ก็ยังถูกนำมารวมใช้งานกับ library นี้ด้วย โดยที่เราไม่จำเป็นจะต้องใช้การ parser ค่ามันอีก เพราะมันจะแปลงมาเป็น json ให้เลย ทั้ง ไป-กลับ และ function ต่าง ๆ ที่อยู่ใน Library เราจะมั่นใจได้ว่ามันจะไม่ถูก deprecated แน่นอน เพราะถ้ามีการ deprecated เราก็จะสามารถอัพเดดเวอร์ชั่นใหม่ ๆ ได้ตลอด โดยที่ไม่จำเป็นจะต้องมาแก้ไข Code ใหม่
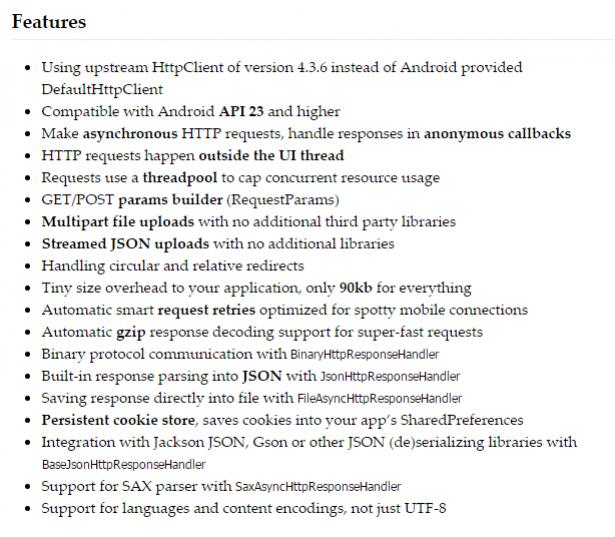
สำหรับตัวอย่างและ Code นี้รองรับการเขียนทั้งบนโปรแกรม Eclipse และ Android Studio
Download OkHttp
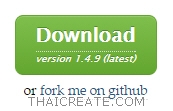
การติดตั้งสามารถดาวน์โหลดไฟล์ .JAR แล้วนำไป Import ลงใน Eclipse หรือ Android Studio

ในการใช้งาน Asynchronous Http Client จะต้องใช้ Library ของ httpclient [HttpClient Android Library (cz.msebera.android.httpclient)]
กรณีใช้บน Android Studio เพิ่ม build.gradle
compile 'com.loopj.android:android-async-http:1.4.9'
ใน AndroidManifest.xml เพิ่ม Permission สำหรับการเชื่อมต่อกับ Internet ดังนี้
<uses-permission android:name="android.permission.INTERNET" />
ขั้นตอนการเรียกใช้
Example 1 : การเรียก URL ผ่าน get
Syntax
AsyncHttpClient client = new AsyncHttpClient();
client.get("https://www.google.com", new AsyncHttpResponseHandler() {
@Override
public void onStart() {
// called before request is started
}
@Override
public void onSuccess(int statusCode, Header[] headers, byte[] response) {
// called when response HTTP status is "200 OK"
}
@Override
public void onFailure(int statusCode, Header[] headers, byte[] errorResponse, Throwable e) {
// called when response HTTP status is "4XX" (eg. 401, 403, 404)
}
@Override
public void onRetry(int retryNo) {
// called when request is retried
}
});
getString.php : ตัวอย่างไฟล์ PHP ที่อยู่บน Server (www.thaicreate.com/android/getString.php)
<?php
echo date("Y-m-d H:i:s");
?>

activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/txtResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="50dp"
android:gravity="center"
android:text="Result" />
</TableLayout>
MainActivity.java
package com.myapp;
import android.os.Bundle;
import java.io.UnsupportedEncodingException;
import com.loopj.android.http.*;
import android.app.Activity;
import android.widget.TextView;
import cz.msebera.android.httpclient.Header;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
final TextView txtResult = (TextView) findViewById(R.id.txtResult);
AsyncHttpClient client = new AsyncHttpClient();
client.get("https://www.thaicreate.com/android/getString.php", new AsyncHttpResponseHandler() {
@Override
public void onStart() {
// called before request is started
}
@Override
public void onSuccess(int statusCode, Header[] headers, byte[] response) {
// called when response HTTP status is "200 OK"
String result = null;
try {
result = new String(response, "UTF-8");
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
txtResult.setText(result);
}
@Override
public void onFailure(int statusCode, Header[] headers, byte[] errorResponse, Throwable e) {
// called when response HTTP status is "4XX" (eg. 401, 403, 404)
}
@Override
public void onRetry(int retryNo) {
// called when request is retried
}
});
}
}

Example 2 : การเรียก URL และส่งค่า Parameters ผ่าน post
Syntax
AsyncHttpClient client = new AsyncHttpClient();
RequestParams params = new RequestParams();
params.put("key", "value");
params.put("more", "data");
client.post("https://www.google.com", params, new AsyncHttpResponseHandler() {
@Override
public void onStart() {
// called before request is started
}
@Override
public void onSuccess(int statusCode, Header[] headers, byte[] response) {
// called when response HTTP status is "200 OK"
}
@Override
public void onFailure(int statusCode, Header[] headers, byte[] errorResponse, Throwable e) {
// called when response HTTP status is "4XX" (eg. 401, 403, 404)
}
@Override
public void onRetry(int retryNo) {
// called when request is retried
}
});
postString.php : ตัวอย่างไฟล์ PHP ที่อยู่บน Server (www.thaicreate.com/android/postString.php)
<?php
echo "Sawatdee : ".$_POST["sName"]." ".$_POST["sLastName"];
?>
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/txtResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="107dp"
android:text="Result" />
</RelativeLayout>
MainActivity.java
package com.myapp;
import android.os.Bundle;
import java.io.UnsupportedEncodingException;
import com.loopj.android.http.*;
import android.app.Activity;
import android.widget.TextView;
import cz.msebera.android.httpclient.Header;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
final TextView txtResult = (TextView) findViewById(R.id.txtResult);
AsyncHttpClient client = new AsyncHttpClient();
RequestParams params = new RequestParams();
params.put("sName", "Weerachai");
params.put("sLastName", "Nukitram");
client.post("https://www.thaicreate.com/android/postString.php",params, new AsyncHttpResponseHandler() {
@Override
public void onStart() {
// called before request is started
}
@Override
public void onSuccess(int statusCode, Header[] headers, byte[] response) {
// called when response HTTP status is "200 OK"
String result = null;
try {
result = new String(response, "UTF-8");
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
txtResult.setText(result);
}
@Override
public void onFailure(int statusCode, Header[] headers, byte[] errorResponse, Throwable e) {
// called when response HTTP status is "4XX" (eg. 401, 403, 404)
}
@Override
public void onRetry(int retryNo) {
// called when request is retried
}
});
}
}
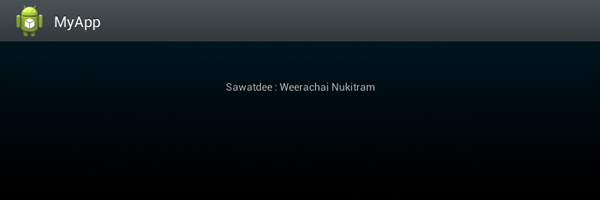
Example 3 : การส่งค่า Post ไปยัง Web Server พร้อมกับ multipart/form-data
AsyncHttpClient client = new AsyncHttpClient();
File myFile = new File("/path/to/file.png");
RequestParams params = new RequestParams();
try {
params.put("uploadFile", myFile);
} catch (FileNotFoundException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
params.put("sName", "Weerachai");
params.put("sLastName", "Nukitram");
ตัวอย่างการใช้งานต่าง ๆ
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2015-11-22 13:03:13 /
2017-03-26 21:30:02 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|