Android AsyncTask and ProgressBar |
Android AsyncTask and ProgressBar สำหรับ AsyncTask เป็นการทำงานในรูปแบบของ Thread และ Background Process คือจะทำงานอยู่เบื้องหลัง และสามารถนำผลลัพธ์ที่ได้แสดงผลในส่วนของ UI (User Interface) ถ้าจะให้เข้าใจง่าย ๆ ก็คือในขณะที่โปรแกรมกำลังทำงานอยู่ จะใช้ AsyncTask ทำงานอยู่เบื้องหลัง และเมื่อทำงานเสร็จสิ้นก็จะแสดงผลลัพธ์ที่ได้ทางหน้าจอของ Screen ข้อดีของการใช้ AsyncTask ที่เห็นได้ชัดคือในขณะที่โปรแกรมกำลังทำงาน หน้าจอของโปรแกรมจะไม่ค้าง ซึ่ง AsyncTask จะนิยมใช้งานร่วมกับ ProgressBar
ภาพประกอบแสดงการใช้งาน AsyncTask ร่วมกับ ProgressBar ในขณะที่กำลังโหลดข้อมูลจาก Server โดยจะสังเกตุว่าโปรแกรมจะไม่ค้าง ในขณะที่ ProgressBar กำลังทำงาน
AsyncTask Syntax
public class CommandInAsync extends AsyncTask<String, Void, Void> {
@Override
protected void onPreExecute() {
super.onPreExecute();
}
@Override
protected Void doInBackground(String... params) {
// TODO Auto-generated method stub
return null;
}
@Override
protected void onPostExecute(Void unused) {
// TODO Auto-generated method stub
}
}
Run AsyncTask
new CommandInAsync().execute();
จาก Code ของ Java เป็นการประกาศ Class แบบ AsyncTask และการสั่งให้ AsyncTask ทำงาน โดยใน AsyncTask มี Method ที่เป็น Override หลัก ๆ อยู่ 3 ตัวคือ
- onPreExecute() // เป็น Method แรกสุดที่ทำงานหลังจากที่ได้เรียกสั่งให้ AsyncTask ทำงาน
- doInBackground() // เป็น Method ที่ทำงานต่าง ๆ ของ AsyncTask โดยคำสั่งต่าง ๆ จะเก็บไว้ใน Method นี้
- onPostExecute() // เป็น Method สุดท้่ายเมื่อ AsyncTask ทำงานเสร็จสิ้น
ในการเขียนโปรแกรม Android ติดต่อกับ Server เพื่อจะดึงข้อมูลในรูปแบบต่าง ๆ การใช้ AsyncTask กับ ProgressBar นั้นจะมีความจำเป็นและสำคัญอย่างยิ่ง เพราะจะเป็นการแสดงผลและสถานะว่าโปรแกรมกำลังทำงาน และให้ผู้ใช้รอ หรือจะใช้ AsyncTask แสดงผลข้อมูลบางรายการที่ได้โหลดเรียบร้อยแล้วก็สามารถทำได้เช่นเดียวกัน และในบทความของไทยครีเอทก็ได้ยกตัวอย่างเกี่ยวกับการใช้ AsyncTask กับ ProgressBar ไว้หลายตัว สามารถหาอ่านได้จากบทความที่เกี่ยวข้อง
เรามาลองดูการใช้งาน AsyncTask กับ ProgressBar เพื่อแสดงสถานะ Loading กำลังโหลดรายการข้อมูลจาก Server
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Example 1 การใช้ AsyncTask กับ ProgressBar ในขณะที่กำลังโหลด Image Resource จาก URL
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml
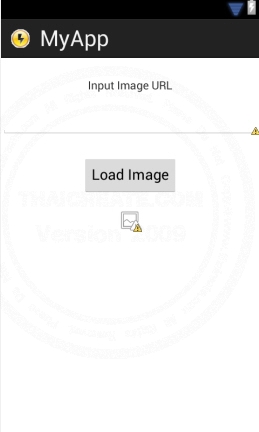
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="25dp"
android:text="Input Image URL" />
<EditText
android:id="@+id/editText1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/textView1"
android:layout_centerHorizontal="true"
android:gravity="center"
android:layout_marginTop="22dp"
android:ems="20"
android:textAppearance="?android:attr/textAppearanceSmall" />
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/editText1"
android:layout_centerHorizontal="true"
android:layout_marginTop="24dp"
android:text="Load Image" />
<ImageView
android:id="@+id/imageView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/button1"
android:layout_centerHorizontal="true"
android:layout_marginTop="16dp"
android:src="@android:drawable/ic_menu_report_image" />
</RelativeLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.ByteArrayOutputStream;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URL;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.StrictMode;
import android.util.Log;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.Toast;
public class MainActivity extends Activity {
private static final String TAG = "ERROR";
private static final int IO_BUFFER_SIZE = 4 * 1024;
public static final int DIALOG_DOWNLOAD_IMAGE_PROGRESS = 0;
private ProgressDialog mProgressDialog;
@SuppressLint("NewApi")
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
final EditText txt1 = (EditText) findViewById(R.id.editText1);
final Button btn1 = (Button) findViewById(R.id.button1);
// Perform action on click
btn1.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String url = txt1.getText().toString();
new DownloadImageFileAsync().execute(url);
}
});
}
public void ShowResult(final Bitmap imgBitmap)
{
final ImageView img1 = (ImageView) findViewById(R.id.imageView1);
try
{
img1.setImageBitmap(imgBitmap);
} catch (Exception e) {
// When Error
img1.setImageResource(android.R.drawable.ic_menu_report_image);
Toast.makeText(MainActivity.this,"Load Image Failed.",
Toast.LENGTH_LONG).show();
}
}
@Override
protected Dialog onCreateDialog(int id) {
switch (id) {
case DIALOG_DOWNLOAD_IMAGE_PROGRESS:
mProgressDialog = new ProgressDialog(this);
mProgressDialog.setMessage("Downloading.....");
mProgressDialog.setProgressStyle(ProgressDialog.STYLE_SPINNER);
mProgressDialog.setCancelable(true);
mProgressDialog.show();
return mProgressDialog;
default:
return null;
}
}
// Download Image in Background
public class DownloadImageFileAsync extends AsyncTask<String, Void, Void> {
Bitmap imgBitmap;
protected void onPreExecute() {
super.onPreExecute();
showDialog(DIALOG_DOWNLOAD_IMAGE_PROGRESS);
}
@Override
protected Void doInBackground(String... params) {
// TODO Auto-generated method stub
String strImagePath = params[0]; // Get Path from params
Bitmap bitmap = null;
InputStream in = null;
BufferedOutputStream out = null;
try {
in = new BufferedInputStream(new URL(strImagePath).openStream(), IO_BUFFER_SIZE);
final ByteArrayOutputStream dataStream = new ByteArrayOutputStream();
out = new BufferedOutputStream(dataStream, IO_BUFFER_SIZE);
copy(in, out);
out.flush();
final byte[] data = dataStream.toByteArray();
BitmapFactory.Options options = new BitmapFactory.Options();
//options.inSampleSize = 1;
bitmap = BitmapFactory.decodeByteArray(data, 0, data.length,options);
} catch (IOException e) {
Log.e(TAG, "Could not load Bitmap from: " + strImagePath);
} finally {
closeStream(in);
closeStream(out);
}
imgBitmap = bitmap;
return null;
}
protected void onPostExecute(Void unused) {
ShowResult(imgBitmap); // When Finish Show Content
dismissDialog(DIALOG_DOWNLOAD_IMAGE_PROGRESS);
removeDialog(DIALOG_DOWNLOAD_IMAGE_PROGRESS);
}
private void closeStream(Closeable stream) {
if (stream != null) {
try {
stream.close();
} catch (IOException e) {
android.util.Log.e(TAG, "Could not close stream", e);
}
}
}
private void copy(InputStream in, OutputStream out) throws IOException {
byte[] b = new byte[IO_BUFFER_SIZE];
int read;
while ((read = in.read(b)) != -1) {
out.write(b, 0, read);
}
}
}
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
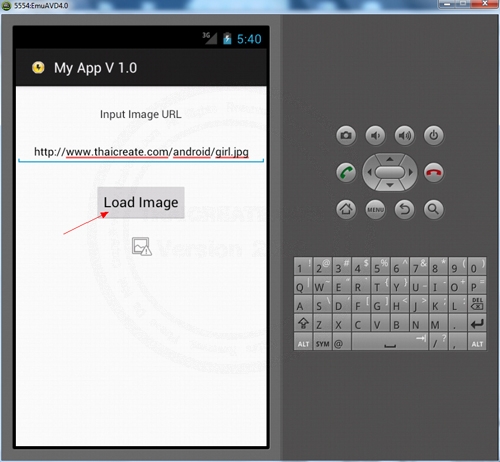
กรอก URL ของรูปภาพแล้วคลิกที่ Load Image
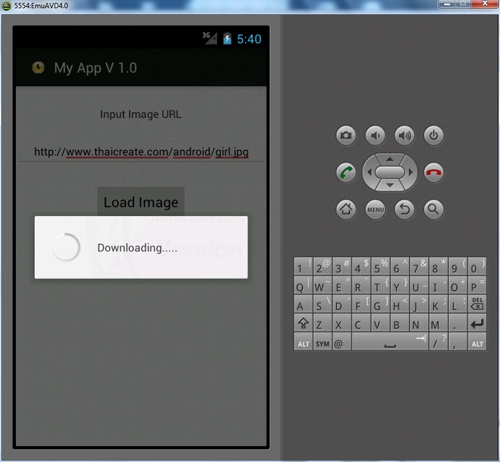
ในขณะที่โหลดข้อมูลจะเห็นว่ามี ProgessBar ที่เป็น Dialog แสดงผลว่ากำลังทำงานอยู่
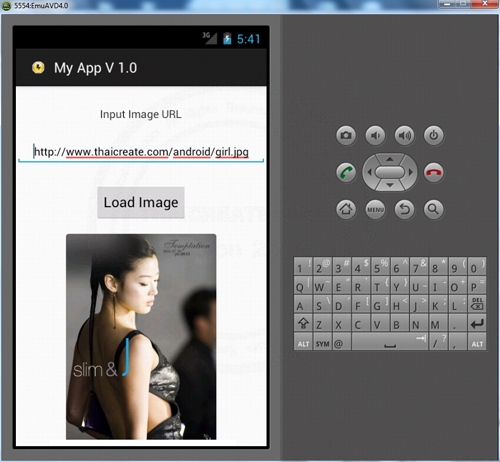
หลังจากโหลดเรียบร้อยแล้ว ProgressDialog ก็จะหายไป และแสดงผลลัพธ์ออกทางหน้าจอ
Example 2 การใช้ AsyncTask กับ ProgressBar ในขณะที่กำลังโหลดข้อมูลจาก Web Server และเมื่อโหลดข้อมูลเรียบร้อยแล้วจะแสดงผลลัพธ์ที่ได้บน ListView
Web Server
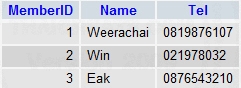
โครงสร้างของข้อมูล
CREATE TABLE `member` (
`MemberID` int(2) NOT NULL,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
PRIMARY KEY (`MemberID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'Weerachai', '0819876107');
INSERT INTO `member` VALUES (2, 'Win', '021978032');
INSERT INTO `member` VALUES (3, 'Eak', '0876543210');
getJSON.php
<?
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM member WHERE 1 ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
ไฟล์ php ในฝั่ง Server ที่จะส่งข้อมูลให้กับ Android
Android HttpGet and HttpPost
พื้นฐานให้อ่านบทความ HttpGet กับ HttpPost จะได้เข้าใจง่ายขึ้น
Android Project
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
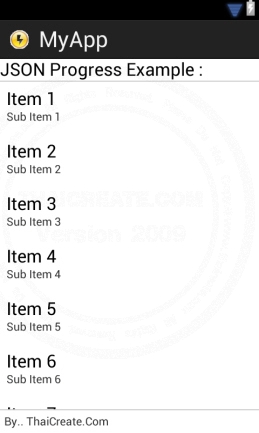
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="JSON Progress Example : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
ไฟล์ XML Layout ของ Activity หลัก
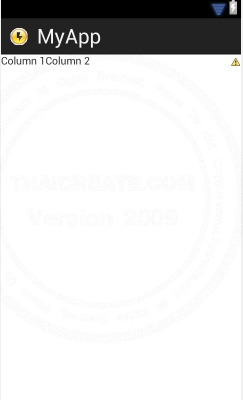
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColMemberID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="MemberID"/>
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="Name"/>
<TextView
android:id="@+id/ColTel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Tel" />
</LinearLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.content.DialogInterface;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.ListView;
import android.widget.SimpleAdapter;
public class MainActivity extends Activity {
public static final int DIALOG_DOWNLOAD_JSON_PROGRESS = 0;
private ProgressDialog mProgressDialog;
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
new DownloadJSONAsync().execute();
}
@Override
protected Dialog onCreateDialog(int id) {
switch (id) {
case DIALOG_DOWNLOAD_JSON_PROGRESS:
mProgressDialog = new ProgressDialog(this);
mProgressDialog.setMessage("Downloading.....");
mProgressDialog.setProgressStyle(ProgressDialog.STYLE_SPINNER);
mProgressDialog.setCancelable(true);
mProgressDialog.show();
return mProgressDialog;
default:
return null;
}
}
public void ShowResult(final ArrayList<HashMap<String, String>> MyArrList)
{
// listView1
final ListView lisView1 = (ListView)findViewById(R.id.listView1);
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(MainActivity.this, MyArrList, R.layout.activity_column,
new String[] {"MemberID", "Name", "Tel"}, new int[] {R.id.ColMemberID, R.id.ColName, R.id.ColTel});
lisView1.setAdapter(sAdap);
final AlertDialog.Builder viewDetail = new AlertDialog.Builder(this);
// OnClick Item
lisView1.setOnItemClickListener(new OnItemClickListener() {
public void onItemClick(AdapterView<?> myAdapter, View myView,
int position, long mylng) {
String sMemberID = MyArrList.get(position).get("MemberID")
.toString();
String sName = MyArrList.get(position).get("Name")
.toString();
String sTel = MyArrList.get(position).get("Tel")
.toString();
//String sMemberID = ((TextView) myView.findViewById(R.id.ColMemberID)).getText().toString();
// String sName = ((TextView) myView.findViewById(R.id.ColName)).getText().toString();
// String sTel = ((TextView) myView.findViewById(R.id.ColTel)).getText().toString();
viewDetail.setIcon(android.R.drawable.btn_star_big_on);
viewDetail.setTitle("Member Detail");
viewDetail.setMessage("MemberID : " + sMemberID + "\n"
+ "Name : " + sName + "\n" + "Tel : " + sTel);
viewDetail.setPositiveButton("OK",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
// TODO Auto-generated method stub
dialog.dismiss();
}
});
viewDetail.show();
}
});
}
// Download JSON in Background
public class DownloadJSONAsync extends AsyncTask<String, Void, Void> {
ArrayList<HashMap<String, String>> mArrList;
protected void onPreExecute() {
super.onPreExecute();
showDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
}
@Override
protected Void doInBackground(String... params) {
// TODO Auto-generated method stub
/** JSON return
* [{"MemberID":"1","Name":"Weerachai","Tel":"0819876107"},
* {"MemberID":"2","Name":"Win","Tel":"021978032"},
* {"MemberID":"3","Name":"Eak","Tel":"0876543210"}]
*/
String url = "https://www.thaicreate.com/android/getJSON.php";
try {
JSONArray data = new JSONArray(getJSONUrl(url));
mArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("MemberID", c.getString("MemberID"));
map.put("Name", c.getString("Name"));
map.put("Tel", c.getString("Tel"));
mArrList.add(map);
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
protected void onPostExecute(Void unused) {
ShowResult(mArrList); // When Finish Show Content
dismissDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
removeDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
}
}
public String getJSONUrl(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download file..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
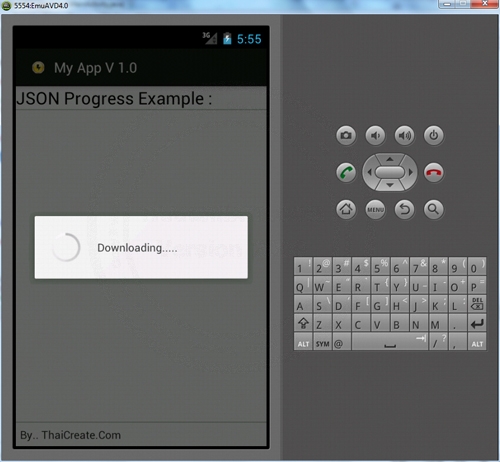
ในขณะที่กำลังโหลดข้อมูลจาก Server จะแสดง Progress Dialog เพื่อให้ผู้ใช้ทราบว่ากำลังทำงานอยู่
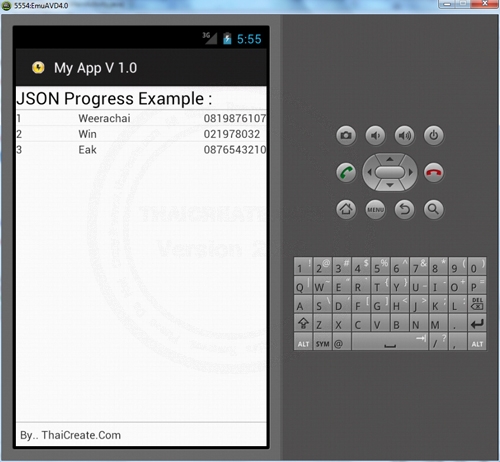
เมื่อโหลดข้อมูลเรียบร้อยแล้วก็จะซ่อน Progress Dialog และแสดงข้อมูลจาก Server ที่ได้บน ListView
Example 2.1 การแสดง Progress Bar แบบ Indeterminate (Icons หมุ่น ๆ บน Title Bar)
แก้ไขไฟล์ MainActivity.java ดังนี้
MainActivity.java
package com.myapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.view.Window;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.ListView;
import android.widget.SimpleAdapter;
public class MainActivity extends Activity {
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_main);
setProgressBarIndeterminateVisibility(false);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
new DownloadJSONAsync().execute();
}
public void ShowResult(final ArrayList<HashMap<String, String>> MyArrList)
{
// listView1
final ListView lisView1 = (ListView)findViewById(R.id.listView1);
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(MainActivity.this, MyArrList, R.layout.activity_column,
new String[] {"MemberID", "Name", "Tel"}, new int[] {R.id.ColMemberID, R.id.ColName, R.id.ColTel});
lisView1.setAdapter(sAdap);
final AlertDialog.Builder viewDetail = new AlertDialog.Builder(this);
// OnClick Item
lisView1.setOnItemClickListener(new OnItemClickListener() {
public void onItemClick(AdapterView<?> myAdapter, View myView,
int position, long mylng) {
String sMemberID = MyArrList.get(position).get("MemberID")
.toString();
String sName = MyArrList.get(position).get("Name")
.toString();
String sTel = MyArrList.get(position).get("Tel")
.toString();
//String sMemberID = ((TextView) myView.findViewById(R.id.ColMemberID)).getText().toString();
// String sName = ((TextView) myView.findViewById(R.id.ColName)).getText().toString();
// String sTel = ((TextView) myView.findViewById(R.id.ColTel)).getText().toString();
viewDetail.setIcon(android.R.drawable.btn_star_big_on);
viewDetail.setTitle("Member Detail");
viewDetail.setMessage("MemberID : " + sMemberID + "\n"
+ "Name : " + sName + "\n" + "Tel : " + sTel);
viewDetail.setPositiveButton("OK",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
// TODO Auto-generated method stub
dialog.dismiss();
}
});
viewDetail.show();
}
});
}
// Download JSON in Background
public class DownloadJSONAsync extends AsyncTask<String, Void, Void> {
ArrayList<HashMap<String, String>> mArrList;
protected void onPreExecute() {
super.onPreExecute();
setProgressBarIndeterminateVisibility(true);
}
@Override
protected Void doInBackground(String... params) {
// TODO Auto-generated method stub
/** JSON return
* [{"MemberID":"1","Name":"Weerachai","Tel":"0819876107"},
* {"MemberID":"2","Name":"Win","Tel":"021978032"},
* {"MemberID":"3","Name":"Eak","Tel":"0876543210"}]
*/
String url = "https://www.thaicreate.com/android/getJSON.php";
try {
JSONArray data = new JSONArray(getJSONUrl(url));
mArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("MemberID", c.getString("MemberID"));
map.put("Name", c.getString("Name"));
map.put("Tel", c.getString("Tel"));
mArrList.add(map);
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
protected void onPostExecute(Void unused) {
ShowResult(mArrList); // When Finish Show Content
setProgressBarIndeterminateVisibility(false);
}
}
public String getJSONUrl(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download file..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
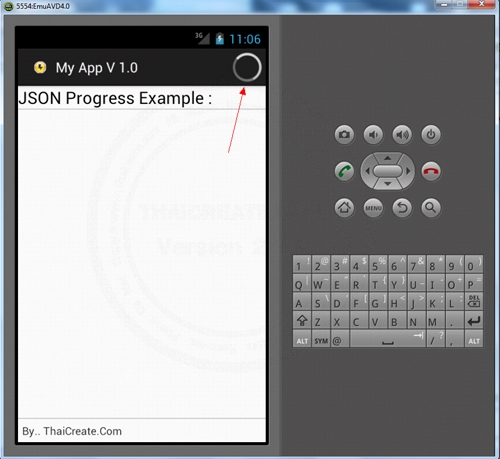
แสดง Icons หมุ่น ๆ ด้านบนของ Title Bar ในขณะที่กำลังโหลดข้อมูล
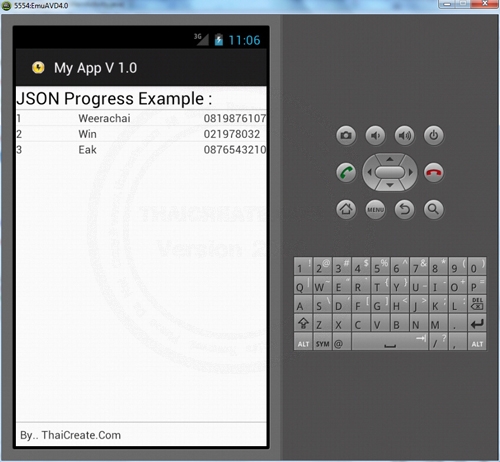
หลังจากโหลดข้อมูลเรียบร้อยแล้วก็จะแสดงข้อมูลที่ได้ และ Progress Bar ก็จะหายไป
AsyncTask and ProgressDialog (showDialog, dismissDialog, removeDialog : Deprecated)
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-08-11 08:02:50 /
2017-03-26 22:46:56 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|