Android and Web Server ของ PHP กับ MySQL แสดงบน ListView ในรูปแบบของ JSON |
Android and Web Server ของ PHP กับ MySQL แสดงบน ListView ในรูปแบบของ JSON เหตุผลที่ให้ความสำคัญกับ ListView และได้ยกตัวอย่างประกอบหลายบทความ เพราะพื้นฐานในการเขียนโปรแกรมบน Android เราจะใช้ ListView ทำการจัดการกับข้อมูลเกือบทั้งหมด ไม่ว่าจะเป็นการแสดงข้อมูลจาก Web Server หรือรายไฟล์การต่าง ๆ ก็ล้วนแต่ใช้ ListView จัดการทั้งสิ้น และ ListView เองก็สามารถรองรับกับข้อมูลและรูปแบบได้หลากหลายผ่าน Custom Layout ที่ได้สร้างขึ้น
แสดง ListView กับข้อมูลที่มาจาก Web Server ผ่าน JSON
ในตัวอย่างนี้จะเป็นการใช้ HttpPost อ่านข้อมูลจาก Web Server ของ PHP และ MySQL ที่ได้ออกแบบไว้ โดยในฝั่งของ Web Server จะทำการ return ค่า JSON กลับมาให้กับ Android ทุกครั้งที่ Request เข้าไป
สำหรับบทความต่าง ๆ ที่เกี่ยวกับ JSON / Web Server ได้ Review ไว้ในบทความต่าง ๆ ดังนี้
Android HttpGet and HttpPost
Basic Android and JSON
Basic Android and Web Service
สามารถคลิกเข้าไปอ่านเพื่อทำความเข้าใจได้ในแต่ล่ะหัวข้อ ซึ่งได้ยกตัวอย่างประกอบหลายตัวมาก
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Web Server
customer
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `customer`
--
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'EN', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
โครงสร้างของ MySQL Database

getJSON.php
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
// $_POST["txtKeyword"] = "a"; // for Sample
$strKeyword = $_POST["txtKeyword"];
$strSQL = "SELECT * FROM customer WHERE 1 AND Name LIKE '%".$strKeyword."%' ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
ไฟล์ของ PHP ที่อยู่บน Web Server ที่ทำหน้าที่แปลงข้อมูลจาก MySQL แล้วส่งกลับมายัง Android ด้วย JSON Code
Android Project
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
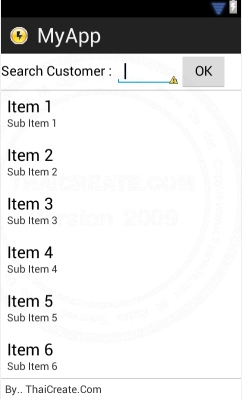
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Search Customer : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/editText1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="4" >
</EditText>
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="OK" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
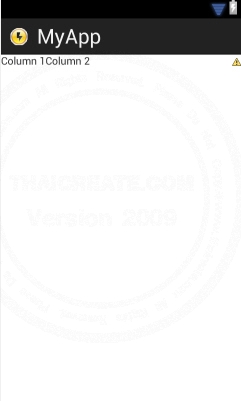
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColCustomerID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.2"
android:text="CustomerID"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1.0"
android:text="Name"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/ColEmail"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Email"
android:textAppearance="?android:attr/textAppearanceSmall" />
</LinearLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.SimpleAdapter;
public class MainActivity extends Activity {
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
final Button btn1 = (Button) findViewById(R.id.button1);
// Perform action on click
btn1.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
SearchData();
}
});
}
public void SearchData()
{
// listView1
final ListView lisView1 = (ListView)findViewById(R.id.listView1);
// editText1
final EditText inputText = (EditText)findViewById(R.id.editText1);
/**
* [{"CustomerID":"C001","Name":"Win Weerachai","Email":"[email protected]" ,"CountryCode":"TH","Budget":"1000000","Used":"600000"},
* {"CustomerID":"C002","Name":"John Smith","Email":"[email protected]" ,"CountryCode":"EN","Budget":"2000000","Used":"800000"},
* {"CustomerID":"C003","Name":"Jame Born","Email":"[email protected]" ,"CountryCode":"US","Budget":"3000000","Used":"600000"},
* {"CustomerID":"C004","Name":"Chalee Angel","Email":"[email protected]" ,"CountryCode":"US","Budget":"4000000","Used":"100000"}]
*/
String url = "https://www.thaicreate.com/android/getJSON.php";
// Paste Parameters
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("txtKeyword", inputText.getText().toString()));
try {
JSONArray data = new JSONArray(getJSONUrl(url,params));
final ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("CustomerID", c.getString("CustomerID"));
map.put("Name", c.getString("Name"));
map.put("Email", c.getString("Email"));
map.put("CountryCode", c.getString("CountryCode"));
map.put("Budget", c.getString("Budget"));
map.put("Used", c.getString("Used"));
MyArrList.add(map);
}
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(MainActivity.this, MyArrList, R.layout.activity_column,
new String[] {"CustomerID", "Name", "Email"}, new int[] {R.id.ColCustomerID, R.id.ColName, R.id.ColEmail});
lisView1.setAdapter(sAdap);
final AlertDialog.Builder viewDetail = new AlertDialog.Builder(this);
// OnClick Item
lisView1.setOnItemClickListener(new OnItemClickListener() {
public void onItemClick(AdapterView<?> myAdapter, View myView,
int position, long mylng) {
String strCustomerID = MyArrList.get(position).get("CustomerID")
.toString();
String sName = MyArrList.get(position).get("Name")
.toString();
String strEmail = MyArrList.get(position).get("Email")
.toString();
String strCountryCode = MyArrList.get(position).get("CountryCode")
.toString();
String strBudget = MyArrList.get(position).get("Budget")
.toString();
String strUsed = MyArrList.get(position).get("Used")
.toString();
viewDetail.setIcon(android.R.drawable.btn_star_big_on);
viewDetail.setTitle("Member Detail");
viewDetail.setMessage("MemberID : " + strCustomerID + "\n"
+ "Name : " + sName + "\n"
+ "strEmail : " + strEmail + "\n"
+ "strCountryCode : " + strCountryCode + "\n"
+ "strBudget : " + strBudget + "\n"
+ "Used : " + strUsed);
viewDetail.setPositiveButton("OK",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
// TODO Auto-generated method stub
dialog.dismiss();
}
});
viewDetail.show();
}
});
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public String getJSONUrl(String url,List<NameValuePair> params) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
try {
httpPost.setEntity(new UrlEncodedFormEntity(params));
HttpResponse response = client.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download file..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
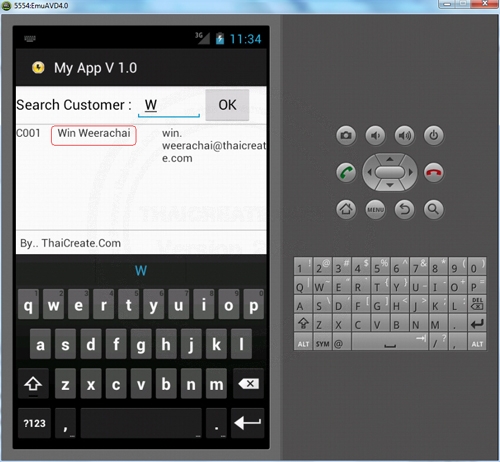
แสดงการค้นหาและแสดงข้อมูลจาก Web Server
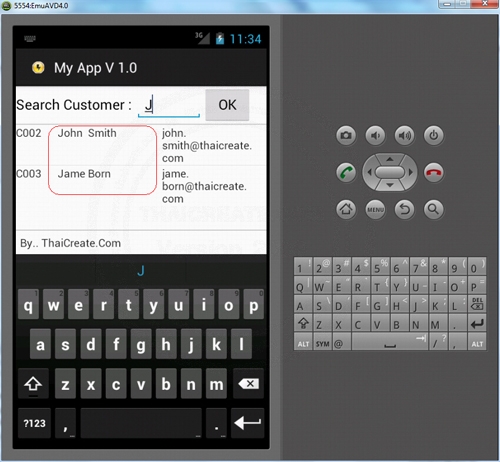
แสดงการค้นหาและแสดงข้อมูลจาก Web Server
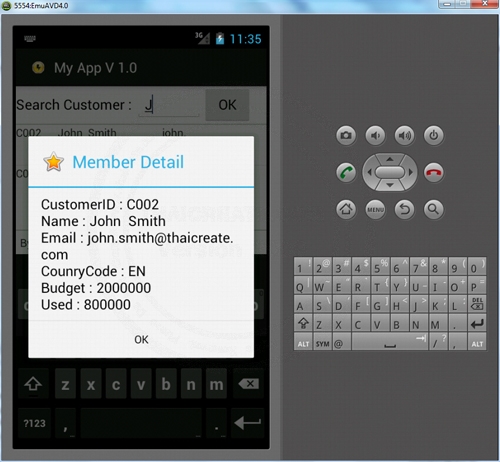
แสดงรายละเอียดของแต่ล่ะ Item บน Dialog Popup
|