Android Connect to Web Server(Website) on Host (PHP and MySQL) |
Android Connect to Web Server(Website) on Host (PHP and MySQL) ตัวอย่างการเขียน Android เพื่อติดต่อกับข้อมูลบน Web Server ทีทำงานด้วย PHP กับ MySQL ผ่าน HttpGet และ HttpPost แบบง่าย ๆ โดย PHP กับ MySQL จะทำงานอยู่ในฝั่งของ Web Server รอรับการ Request ข้อมูลจาก Client และเมื่อฝั่งของ Client ทำการ Request มาแล้วก็จะ Response กลับไปยัง Client ด้วยการส่งข้อมูลในรูปแบบของ JSON กลับไป ฝั่งของ Client ก็จะทำการแปลง JSON และแสดงผลข้อมูลในรูปแบบต่าง ๆ ที่ต้องการ
จากรูปอธิบายการทำงานจะเห็นว่าฝั่ง Android ซึ่งจะเป็น Client จะทำการ Request ข้อมูลไปยัง Web Server และฝั่ง Web Server ซึ่งทำงานด้วย PHP กับ MySQL เมื่อประมวลผลเรียบร้อยแล้วจะ Response ค่า JSON กลับมา
เพิ่อความเข้าใจแนะนำให้อ่าน 2 บทความนี้
Android HttpGet and HttpPost
Android and JSON
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
มาลองเขียนโปรแกรม Android เพื่ออ่านข้อมูลจาก PHP กับ MySQL โดยออกแบบฟังก์ชั่นอยู่ 2 รายการคือ 1.ทำการค้นหาข้อมูล 2. แสดงข้อมูลเมื่อคลิกแต่ล่ะรายการ
ฝั่ง Web Server
ออกแบบ PHP และ MySQL ดังนี้สร้างตารางชื่อ member และไฟล์ showAllData.php และ getByMemberID.php
member
CREATE TABLE `member` (
`MemberID` int(2) NOT NULL auto_increment,
`Username` varchar(50) NOT NULL,
`Password` varchar(50) NOT NULL,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
`Email` varchar(150) NOT NULL,
PRIMARY KEY (`MemberID`),
UNIQUE KEY `Username` (`Username`),
UNIQUE KEY `Email` (`Email`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'weerachai', 'weerachai@1', 'Weerachai Nukitram', '0819876107', '[email protected]');
INSERT INTO `member` VALUES (2, 'adisorn', 'adisorn@2', 'Adisorn Bunsong', '021978032', '[email protected]');
INSERT INTO `member` VALUES (3, 'surachai', 'surachai@3', 'Surachai Sirisart', '0876543210', '[email protected]');
โครงสร้างของตารางบน MySQL

showAllData.php ใช้สำหรับแสดงข้อมูลทั้งหมด
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
// $_POST["txtKeyword"] = "a"; // for Sample
$strKeyword = $_POST["txtKeyword"];
$strSQL = "SELECT * FROM member WHERE 1 AND Name LIKE '%".$strKeyword."%' ORDER BY MemberID ASC ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
Response Result
[{"MemberID":"1","Username":"weerachai","Password":"weerachai@1","Name":"Weerachai Nukitram","Tel":"0819876107","Email":"[email protected]"},
{"MemberID":"2","Username":"adisorn","Password":"adisorn@2","Name":"Adisorn Bunsong","Tel":"021978032","Email":"[email protected]"},
{"MemberID":"3","Username":"surachai","Password":"surachai@3","Name":"Surachai Sirisart","Tel":"0876543210","Email":"[email protected]"}]
getByMemberID.php ใช้สำหรับแสดงข้อมูลแต่ล่ะรายการของ Record
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
//$_POST["sMemberID"] = "1"; // for Sample
$strMemberID = $_POST["sMemberID"];
$strSQL = "SELECT * FROM member WHERE 1 AND MemberID = '".$strMemberID."' ";
$objQuery = mysql_query($strSQL);
$obResult = mysql_fetch_array($objQuery);
if($obResult)
{
$arr["MemberID"] = $obResult["MemberID"];
$arr["Username"] = $obResult["Username"];
$arr["Password"] = $obResult["Password"];
$arr["Name"] = $obResult["Name"];
$arr["Email"] = $obResult["Email"];
$arr["Tel"] = $obResult["Tel"];
}
mysql_close($objConnect);
/*** return JSON by MemberID ***/
/* Eg :
{"MemberID":"2",
"Username":"adisorn",
"Password":"adisorn@2",
"Name":"Adisorn Bunsong",
"Tel":"021978032",
"Email":"[email protected]"}
*/
echo json_encode($arr);
?>
Response Result
{"MemberID":"2",
"Username":"adisorn",
"Password":"adisorn@2",
"Name":"Adisorn Bunsong",
"Tel":"021978032",
"Email":"[email protected]"}
Android Project
โครงสร้างของไฟล์ประกอบด้วย 5 ไฟล์คือ MainActivity.java, activity_main.xml , DetailActivity.java, activity_detail.xml และ activity_column.xml
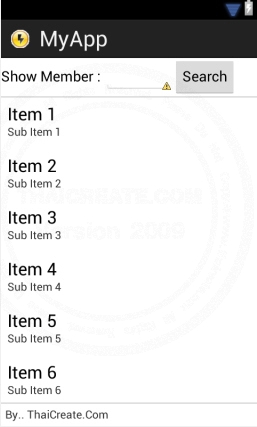
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Show Member : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtKeySearch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="4" >
</EditText>
<Button
android:id="@+id/btnSearch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Search" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColMemberID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.2"
android:text="CustomerID"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1.0"
android:text="Name"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/ColTel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Tel"
android:textAppearance="?android:attr/textAppearanceSmall" />
</LinearLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.Menu;
import android.view.ViewGroup;
import android.view.inputmethod.InputMethodManager;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.BaseAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.TextView;
public class MainActivity extends Activity {
ArrayList<HashMap<String, String>> MyArrList;
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
ShowData();
// btnSearch
final Button btnSearch = (Button) findViewById(R.id.btnSearch);
//btnSearch.setBackgroundColor(Color.TRANSPARENT);
// Perform action on click
btnSearch.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
ShowData();
}
});
}
public void ShowData()
{
// listView1
final ListView lisView1 = (ListView)findViewById(R.id.listView1);
// keySearch
EditText strKeySearch = (EditText)findViewById(R.id.txtKeySearch);
// Disbled Keyboard auto focus
InputMethodManager imm = (InputMethodManager)getSystemService(
Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(strKeySearch.getWindowToken(), 0);
/**
* [{"MemberID":"1","Username":"weerachai","Password":"weerachai@1","Name":"Weerachai Nukitram","Tel":"0819876107","Email":"[email protected]"},
* {"MemberID":"2","Username":"adisorn","Password":"adisorn@2","Name":"Adisorn Bunsong","Tel":"021978032","Email":"[email protected]"},
* {"MemberID":"3","Username":"surachai","Password":"surachai@3","Name":"Surachai Sirisart","Tel":"0876543210","Email":"[email protected]"}]
*/
String url = "https://www.thaicreate.com/android/showAllData.php";
// Paste Parameters
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("txtKeyword", strKeySearch.getText().toString()));
try {
JSONArray data = new JSONArray(getJSONUrl(url,params));
MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("MemberID", c.getString("MemberID"));
map.put("Username", c.getString("Username"));
map.put("Password", c.getString("Password"));
map.put("Name", c.getString("Name"));
map.put("Email", c.getString("Email"));
map.put("Tel", c.getString("Tel"));
MyArrList.add(map);
}
lisView1.setAdapter(new ImageAdapter(this));
// OnClick Item
lisView1.setOnItemClickListener(new OnItemClickListener() {
public void onItemClick(AdapterView<?> myAdapter, View myView,
int position, long mylng) {
String sMemberID = MyArrList.get(position).get("MemberID")
.toString();
String sName = MyArrList.get(position).get("Name")
.toString();
String sTel = MyArrList.get(position).get("Tel")
.toString();
Intent newActivity = new Intent(MainActivity.this,DetailActivity.class);
newActivity.putExtra("MemberID", sMemberID);
startActivity(newActivity);
}
});
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public class ImageAdapter extends BaseAdapter
{
private Context context;
public ImageAdapter(Context c)
{
// TODO Auto-generated method stub
context = c;
}
public int getCount() {
// TODO Auto-generated method stub
return MyArrList.size();
}
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
public View getView(final int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// ColMemberID
TextView txtMemberID = (TextView) convertView.findViewById(R.id.ColMemberID);
txtMemberID.setPadding(10, 0, 0, 0);
txtMemberID.setText(MyArrList.get(position).get("MemberID") +".");
// R.id.ColName
TextView txtName = (TextView) convertView.findViewById(R.id.ColName);
txtName.setPadding(5, 0, 0, 0);
txtName.setText(MyArrList.get(position).get("Name"));
// R.id.ColTel
TextView txtTel = (TextView) convertView.findViewById(R.id.ColTel);
txtTel.setPadding(5, 0, 0, 0);
txtTel.setText(MyArrList.get(position).get("Tel"));
return convertView;
}
}
public String getJSONUrl(String url,List<NameValuePair> params) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
try {
httpPost.setEntity(new UrlEncodedFormEntity(params));
HttpResponse response = client.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
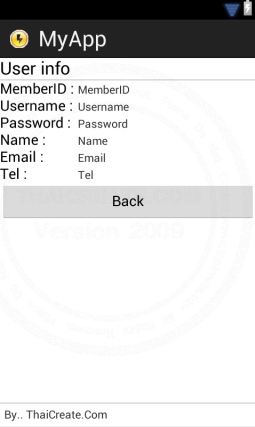
activity_detail.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="User info "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="MemberID : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtMemberID"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="MemberID" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Email : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Email" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tel : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtTel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tel" />
</TableRow>
<Button
android:id="@+id/btnBack"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Back" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
DetailActivity.java
package com.myapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.content.Intent;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.widget.Button;
import android.widget.TextView;
public class DetailActivity extends Activity {
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_detail);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
showInfo();
// btnBack
final Button btnBack = (Button) findViewById(R.id.btnBack);
// Perform action on click
btnBack.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent newActivity = new Intent(DetailActivity.this,MainActivity.class);
startActivity(newActivity);
}
});
}
public void showInfo()
{
// txtMemberID,txtMemberID,txtUsername,txtPassword,txtName,txtEmail,txtTel
final TextView tMemberID = (TextView)findViewById(R.id.txtMemberID);
final TextView tUsername = (TextView)findViewById(R.id.txtUsername);
final TextView tPassword = (TextView)findViewById(R.id.txtPassword);
final TextView tName = (TextView)findViewById(R.id.txtName);
final TextView tEmail = (TextView)findViewById(R.id.txtEmail);
final TextView tTel = (TextView)findViewById(R.id.txtTel);
String url = "https://www.thaicreate.com/android/getByMemberID.php";
Intent intent= getIntent();
final String MemberID = intent.getStringExtra("MemberID");
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("sMemberID", MemberID));
/** Get result from Server (Return the JSON Code)
*
* {"MemberID":"2","Username":"adisorn","Password":"adisorn@2","Name":"Adisorn Bunsong","Tel":"021978032","Email":"[email protected]"}
*/
String resultServer = getHttpPost(url,params);
String strMemberID = "";
String strUsername = "";
String strPassword = "";
String strName = "";
String strEmail = "";
String strTel = "";
JSONObject c;
try {
c = new JSONObject(resultServer);
strMemberID = c.getString("MemberID");
strUsername = c.getString("Username");
strPassword = c.getString("Password");
strName = c.getString("Name");
strEmail = c.getString("Email");
strTel = c.getString("Tel");
if(!strMemberID.equals(""))
{
tMemberID.setText(strMemberID);
tUsername.setText(strUsername);
tPassword.setText(strPassword);
tName.setText(strName);
tEmail.setText(strEmail);
tTel.setText(strTel);
}
else
{
tMemberID.setText("-");
tUsername.setText("-");
tPassword.setText("-");
tName.setText("-");
tEmail.setText("-");
tTel.setText("-");
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public String getHttpPost(String url,List<NameValuePair> params) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
try {
httpPost.setEntity(new UrlEncodedFormEntity(params));
HttpResponse response = client.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Status OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
AndroidManifest.xml
<activity
android:name="DetailActivity"
android:theme="@style/AppTheme"
android:screenOrientation="portrait"
android:label="@string/title_activity_main" />
คำอธิบาย
MainActivity.java จะทำการ Request ไปยัง https://www.thaicreate.com/android/showAllData.php
DetailActivity.java จะทำการ Request ไปยัง https://www.thaicreate.com/android/getByMemberID.php
Screenshot
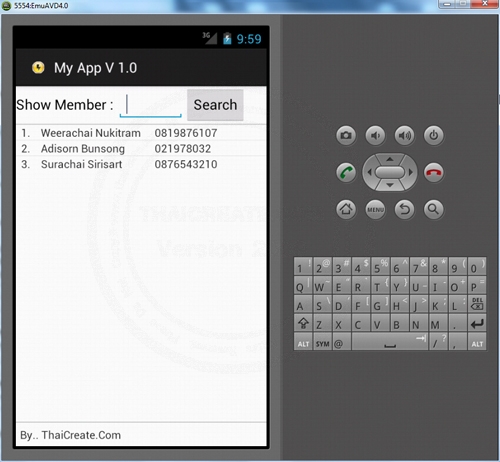
ทดสอบโปรแกรม
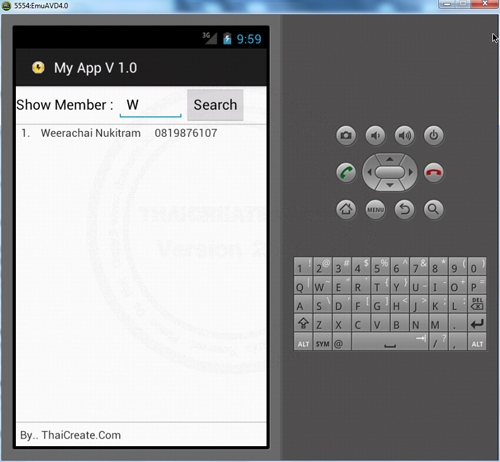
เมื่อทำการค้นหาข้อมูล
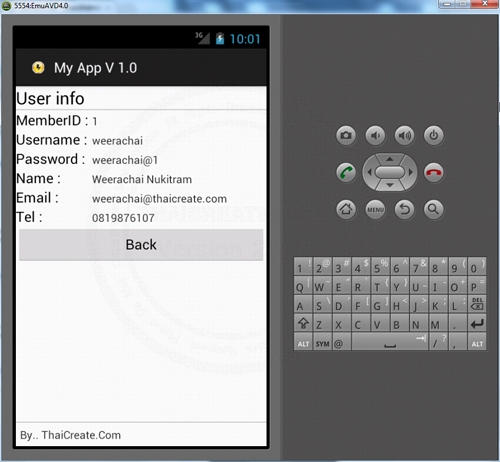
เมื่อคลิกแสดงข้อมูลแต่ล่ะรายการ
.
|