Android People Contact List, Name, Phone No, Photo Picture, Email and Address |
Android People Contact List, Name, Phone No, Photo Picture, Email and Address ถ้าได้เคยใช้ App พวก LINE หรือ What App ที่มีมากับ iPhone หรือ Android จะเห็นว่า Application เหล่านี้จะสามารถดึงรายชื่อ Contact List ที่อยู่ในหลายเลขโทรศัพท์ของเรามาแสดงใน App ของตัวเอง และเราจะเห็นว่าข้อมูลเหล่านี้ OS เองก็ไม่ได้ห่วงหรือห้าม App อื่น ๆ เรียกใช้ และการเรียกใช้งานก็ไม่ได้ยากซับซ้อนอะไรมากมาย แต่ทั้งนี้ขึ้นอยู่กับว่าจะต้องการดึงข้อมูลอะไรออกมาบ้าง เช่น ต้องการ ชื่อ (DISPLAY_NAME), หมายเลขโทรศัพท์(Phone) , รูปภาพที่อยู่ใน Contact (Photo) หรือข้อมูลอื่น ๆ
Android People Contact List
จากภาพจะเห็นว่าเราสามารถเขียน Android เพื่อดึงข้อมูลจาก People Contact List ได้ทั้งที่เป็น ชื่อ(DISPLAY_NAME), หมายเลขโทรศัพท์(Phone) และ รูปภาพ(Photo)
หน้าจอ Contact People บน Android
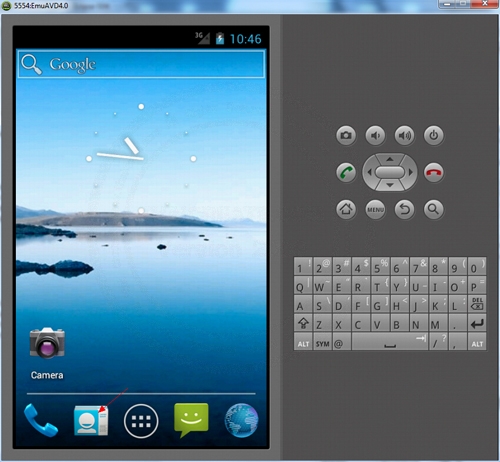
เข้าได้จาก Icons ว่า People
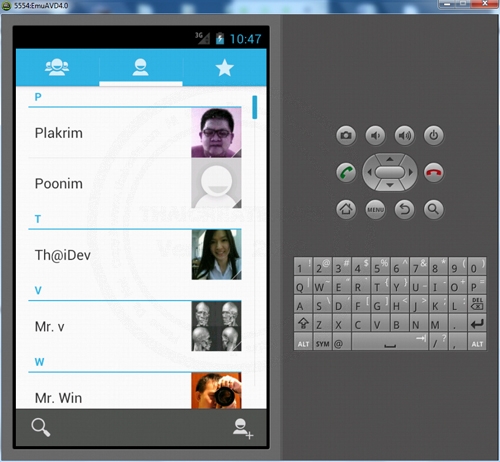
เป็นรายชื่อ Contact ที่อยู่ใน Smartphone
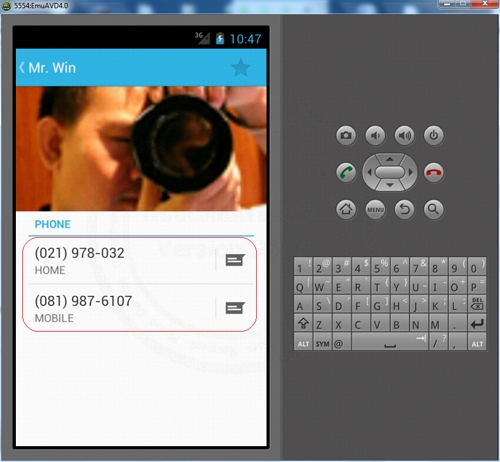
รายละเอียดของ Contact ในตัวอย่างจะเห็นว่าภายใน 1 Contact จะสามารถมีข้อมูลได้หลายรายการ เช่น หมายเลขโทรศัพท์หลายเบอร์ หรือ อีเมล์หลายตัว
ในการเขียนโปรแกรมเพื่อดึง Contact List ที่อยู่ใน Smartphone นั้นจะต้องกำหนด Permission ที่ไฟล์ AndroidManifest.xml ด้วย
สำหรับขั้นตอนในการดึง Contact List ถ้าเป็นแค่ชื่อที่เป็น DISPLAY_NAME จะสามารถทำได้ง่ายเพียงใช้ Cursor ทำการ Query มาจาก ContactsContract.Contacts ก็จะได้ข้อมูลที่อยู่ในรูปแบบของ Cursor และนำไปใช้ได้ในทันที
Cursor cursor = getContentResolver().query(ContactsContract.Contacts.CONTENT_URI, null, null, null, null);
startManagingCursor(cursor);
แต่ถ้าต้องการดึงข้อมูลอื่น ๆ ด้วย เช่น Phone No , Address หรือ Email ซึ่งข้อมูลเหล่านี้ภายใน 1 Contact จะสามารถมีได้หลายรายการ เฉพาะฉะนั้นเมื่อ Loop ข้อมุลในแต่ล่ะ Cursor ก็จะต้องมีการ Loop ข้อมูลของ Phone No ด้วยว่าในแต่ล่ะ Contact มีข้อมูลนั้น ๆ กี่รายการ
และกรณีที่ดึง Photo Contact ก็จะต้องอ่านข้อมูล Photo Contact ที่อยู่ในรุปแบบ Bitmap ก่อนนำมาแสดงผลบน ImageView ด้วย
ในตัวอย่างทั้ง 3 ตัวนี้ ถือได้ว่าเป็นการเลือกข้อมูลที่ค่อนข้างครอบคลุมกับความต้องการใช้งาน ซึ่งยอมรับว่ากว่าจะทำบทความนี้ออกมาได้ก็ใช้เวลาไม่ตำกว่า 5-6 ชม. เพราะข้อมูลบางรายตัวค้นหายาก จะต้องอาสัยการศึกษาจาก Sample Project ที่มากับ Android SDK
<uses-permission android:name="android.permission.READ_CONTACTS" />
การกำหนด Permission สำหรับการอ่าน Contact
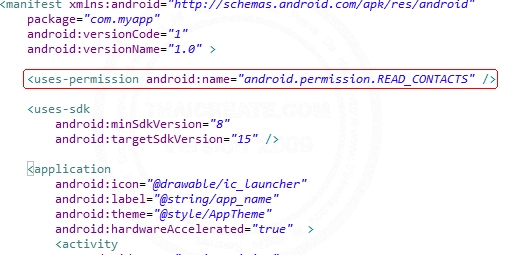
รูปการเพิ่ม Permission บน AndroidManifest.xml
Example 1 การดึงข้อมูลจาก Contact List มาแสดงแบบง่าย ๆ โดยแสดง ID , Name และ Tel
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
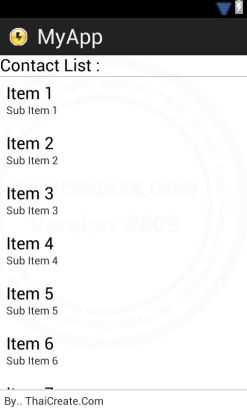
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Contact List : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
ไฟล์ XML Layout ของ Activity หลัก
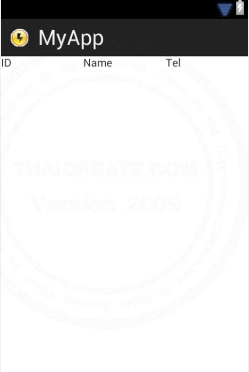
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="ID"/>
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Name"/>
<TextView
android:id="@+id/ColPhoneNo"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Tel" />
</LinearLayout>
XML Layout ของ Custom Layout ของ ListView
MainActivity.java
package com.myapp;
import android.os.Bundle;
import android.provider.ContactsContract;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.View;
import android.view.ViewGroup;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.BaseAdapter;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
import android.app.Activity;
import android.content.Context;
import android.database.Cursor;
public class MainActivity extends Activity {
String arrData[][];
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Cursor cursor = getContentResolver().query(ContactsContract.Contacts.CONTENT_URI, null, null, null, null);
startManagingCursor(cursor);
if(cursor != null)
{
if (cursor.moveToFirst()) {
arrData = new String[cursor.getCount()][cursor.getColumnCount()];
/***
* [x][0] = ID
* [x][1] = Name
* [x][2] = PhoneNo
*/
int i= 0;
do { // Start Loop all contact
String id = cursor.getString(cursor.getColumnIndex(ContactsContract.Contacts._ID));
// ID
arrData[i][0] = cursor.getString(cursor.getColumnIndex(ContactsContract.Contacts._ID));
// Display Name
arrData[i][1] = cursor.getString(cursor.getColumnIndex(ContactsContract.Contacts.DISPLAY_NAME));
// Phone No
if (Integer.parseInt(cursor.getString( cursor.getColumnIndex(ContactsContract.Contacts.HAS_PHONE_NUMBER))) > 0) { // Count of Number
Cursor pCur = getContentResolver().query(
ContactsContract.CommonDataKinds.Phone.CONTENT_URI,
null,
ContactsContract.CommonDataKinds.Phone.CONTACT_ID +" = ?",
new String[]{id}, null);
// Loop for Phone No
while (pCur.moveToNext()) {
String PhoneNo = pCur.getString( pCur.getColumnIndex(ContactsContract.CommonDataKinds.Phone.DATA));
if(arrData[i][2] != null)
{
arrData[i][2] = arrData[i][2] + ", " + PhoneNo;
}
else
{
arrData[i][2] = PhoneNo;
}
}
pCur.close();
}
i++;
} while (cursor.moveToNext());
}
}
cursor.close();
final ListView lView1 = (ListView)findViewById(R.id.listView1);
lView1.setAdapter(new ImageAdapter(this,arrData));
// OnClick
lView1.setOnItemClickListener(new OnItemClickListener() {
public void onItemClick(AdapterView<?> parent, View v,
int position, long id) {
Toast.makeText(getApplicationContext(),
"Your selected : " + arrData[position][1].toString(), Toast.LENGTH_SHORT).show();
}
});
}
public class ImageAdapter extends BaseAdapter
{
private Context context;
private String[][] lis;
public ImageAdapter(Context c, String[][] li)
{
// TODO Auto-generated method stub
context = c;
lis = li;
}
public int getCount() {
// TODO Auto-generated method stub
return lis.length;
}
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
public View getView(int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// ColID
TextView txtID = (TextView) convertView.findViewById(R.id.ColID);
txtID.setPadding(0, 0, 0, 0);
txtID.setText(lis[position][0].toString());
// ColName
TextView txtName = (TextView) convertView.findViewById(R.id.ColName);
txtName.setPadding(0, 0, 0, 0);
txtName.setText(lis[position][1].toString());
// ColPhoneNo
TextView txtPhoneNo = (TextView) convertView.findViewById(R.id.ColPhoneNo);
txtPhoneNo.setText(lis[position][2].toString());
return convertView;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
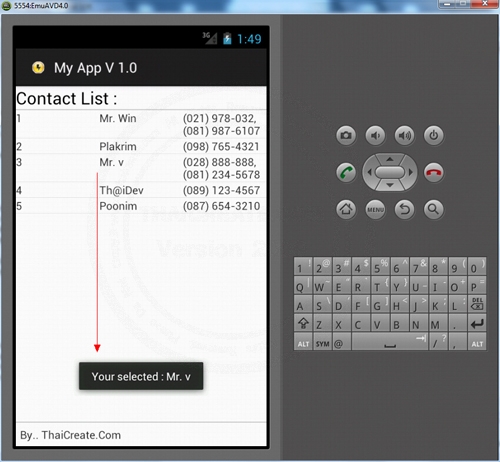
แสดงข้อมูล Contact List โดยมี Display Name และหมายหลขโทรศัพท์
Example 2 การดึง Photo Contact จาก Contact List มาแสดงใน ListView และใช้ ArrayList และ HashMap เข้ามาจัดเก็บข้อมูลเพราะสามารถจัดเก็บได้ทั้งข้อความและ Bitmap ของ Photo Contact
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
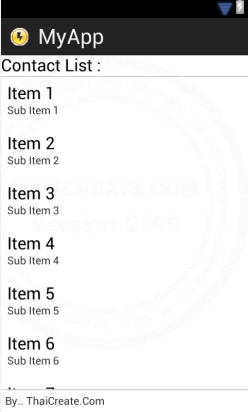
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Contact List : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
ไฟล์ XML Layout ของ Activity หลัก
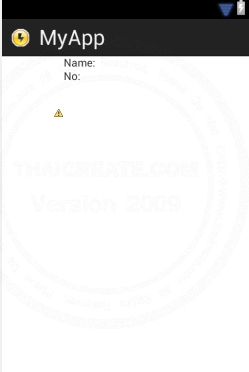
activity_column.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<ImageView
android:id="@+id/ColPhoto"
android:layout_width="60dp"
android:layout_height="60dp"
/>
<LinearLayout
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<LinearLayout
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name: "
/>
<TextView android:id="@+id/ColName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="No: "
/>
<TextView android:id="@+id/ColPhoneNo"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
ไฟล์ Custom Layout ของ ListView
MainActivity.java
package com.myapp;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.HashMap;
import android.net.Uri;
import android.os.Bundle;
import android.provider.ContactsContract;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.View;
import android.view.ViewGroup;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
import android.app.Activity;
import android.content.ContentResolver;
import android.content.ContentUris;
import android.content.Context;
import android.database.Cursor;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
public class MainActivity extends Activity {
ArrayList<HashMap<String, Object>> MyArrList;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Cursor cursor = getContentResolver().query(ContactsContract.Contacts.CONTENT_URI, null, null, null, null);
startManagingCursor(cursor);
MyArrList = new ArrayList<HashMap<String, Object>>();
HashMap<String, Object> map;
if(cursor != null)
{
if (cursor.moveToFirst()) {
map = new HashMap<String, Object>();
/***
* ID
* NAME
* PHONENO
* PHOTO
*/
do { // Start Loop all contact
String id = cursor.getString(cursor.getColumnIndex(ContactsContract.Contacts._ID));
map = new HashMap<String, Object>();
// ID
map.put("ID", (String)cursor.getString( cursor.getColumnIndex(ContactsContract.Contacts._ID)));
// Display Name
map.put("NAME", (String)cursor.getString( cursor.getColumnIndex(ContactsContract.Contacts.DISPLAY_NAME)));
// Phone No
String tmpPhoneNo = "";
if (Integer.parseInt(cursor.getString(cursor.getColumnIndex( ContactsContract.Contacts.HAS_PHONE_NUMBER))) > 0) { // Count of Number
Cursor pCur = getContentResolver().query(
ContactsContract.CommonDataKinds.Phone.CONTENT_URI,
null,
ContactsContract.CommonDataKinds.Phone.CONTACT_ID +" = ?",
new String[]{id}, null);
// Loop for Phone No
while (pCur.moveToNext()) {
String PhoneNo = pCur.getString(pCur.getColumnIndex( ContactsContract.CommonDataKinds.Phone.DATA));
if(tmpPhoneNo != null)
{
tmpPhoneNo = tmpPhoneNo + ", " + PhoneNo;
}
else
{
tmpPhoneNo = PhoneNo;
}
}
pCur.close();
}
// PhoneNo
map.put("PHONENO", (String)tmpPhoneNo);
// Photo Bitmap
Bitmap tmpPhoto = null;
final ContentResolver resolver = getContentResolver();
Cursor sCur = resolver.query(
ContactsContract.Data.CONTENT_URI,
null,
ContactsContract.Data.CONTACT_ID + "=" + id + " AND "
+ ContactsContract.Data.MIMETYPE + "='"
+ ContactsContract.CommonDataKinds.Photo.CONTENT_ITEM_TYPE + "'", null,
null);
if (sCur == null) {
tmpPhoto = null;
}
else
{
Uri uri = ContentUris.withAppendedId(ContactsContract.Contacts.CONTENT_URI, Long
.parseLong(id));
InputStream input = ContactsContract.Contacts.openContactPhotoInputStream(resolver, uri);
if (input == null) {
tmpPhoto = null;
}
else
{
tmpPhoto= BitmapFactory.decodeStream(input);
}
}
sCur.close();
map.put("PHOTO", (Bitmap)tmpPhoto);
// Add to ArrayList
MyArrList.add(map);
} while (cursor.moveToNext());
}
}
cursor.close();
final ListView lView1 = (ListView)findViewById(R.id.listView1);
lView1.setAdapter(new ImageAdapter(this));
// OnClick
lView1.setOnItemClickListener(new OnItemClickListener() {
public void onItemClick(AdapterView<?> parent, View v,
int position, long id) {
Toast.makeText(getApplicationContext(),
"Your selected : " + MyArrList.get(position).get("ID"), Toast.LENGTH_SHORT).show();
//*** for ACTION_CALL
/*
*
Intent intent = new Intent(Intent.ACTION_CALL);
long phoneId = Integer.parseInt(MyArrList.get(position).get("ID"));
intent.setData(ContentUris.withAppendedId(ContactsContract.Contacts.CONTENT_URI, phoneId));
startActivity(intent);
*/
}
});
}
public class ImageAdapter extends BaseAdapter
{
private Context context;
public ImageAdapter(Context c)
{
// TODO Auto-generated method stub
context = c;
}
public int getCount() {
// TODO Auto-generated method stub
return MyArrList.size();
}
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
public View getView(int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// ColPhoto
ImageView imgPhoto = (ImageView) convertView.findViewById(R.id.ColPhoto);
imgPhoto.setPadding(5, 5, 5, 5);
Bitmap bm = (Bitmap)MyArrList.get(position).get("PHOTO");
if (bm != null) {
imgPhoto.setImageBitmap(bm);
} else {
imgPhoto.setImageResource(android.R.drawable.sym_def_app_icon); // No Photo (Default)
}
// ColName
TextView txtName = (TextView) convertView.findViewById(R.id.ColName);
txtName.setPadding(10, 0, 0, 0);
txtName.setText(MyArrList.get(position).get("NAME").toString());
// ColPhoneNo
TextView txtPhoneNo = (TextView) convertView.findViewById(R.id.ColPhoneNo);
txtPhoneNo.setText(MyArrList.get(position).get("PHONENO").toString());
return convertView;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
แสดง Contact List ซึ่งจะดึง Photo Contact มาแสดงใน ListView ด้วย
Screenshot
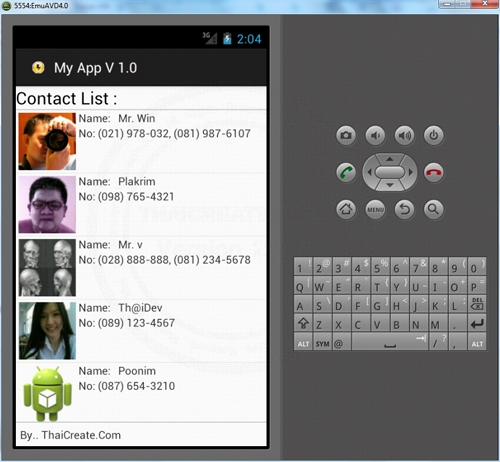
Example 2.1 แสดงรายละเอียดอื่น ๆ เมื่อคลิกที่ Contact แต่ล่ะคนใน ListView
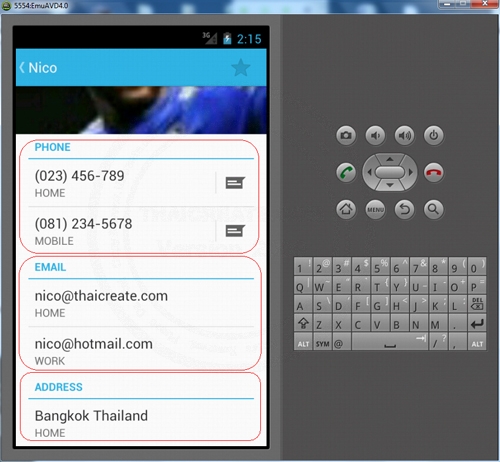
ใน Contact ของ Android จะสังเกตุว่าจะมีข้อมูลอื่น ๆ ที่สามารถจัดเก็บได้ด้วย เช่น Email , Address ซึ่งข้อมูลเหล่านี้สามารถมีได้หลายรายการ
MainActivity.java
Intent newActivity = new Intent(MainActivity.this,DetailActivity.class);
newActivity.putExtra("PhoneID", MyArrList.get(position).get("ID"));
startActivity(newActivity);
ใน Event ของ lView1.setOnItemClickListener() ให้แก้ไขเป็นการ Intent มายัง DetailActivity.class
เพิ่มไฟล์ activity_detail.xml และ DetailActivity.java
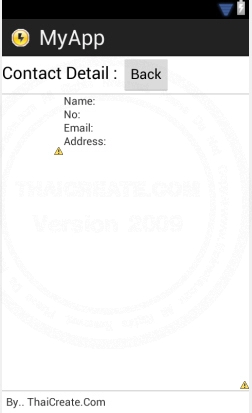
activity_detail.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Contact Detail : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
<Button
android:id="@+id/btnBack"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Back" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<ImageView
android:id="@+id/ColPhoto"
android:layout_width="80dp"
android:layout_height="80dp"
/>
<LinearLayout
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<LinearLayout
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name: "
/>
<TextView android:id="@+id/ColName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="No: "
/>
<TextView android:id="@+id/ColPhoneNo"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Email: "
/>
<TextView android:id="@+id/ColEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Address: "
/>
<TextView android:id="@+id/ColAddress"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
DetailActivity.java
package com.myapp;
import java.io.InputStream;
import android.app.Activity;
import android.content.ContentResolver;
import android.content.ContentUris;
import android.content.Intent;
import android.database.Cursor;
import android.graphics.BitmapFactory;
import android.net.Uri;
import android.os.Bundle;
import android.provider.ContactsContract;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
public class DetailActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_detail);
//******** Show Detail **********//
Intent intent= getIntent();
final long PhoneID = Integer.parseInt(intent.getStringExtra("PhoneID"));
// Photo Bitmap
ImageView imgPhoto = (ImageView) findViewById(R.id.ColPhoto);
imgPhoto.setPadding(10, 10, 10, 10);
final ContentResolver resolver = getContentResolver();
Cursor sCur = resolver.query(
ContactsContract.Data.CONTENT_URI,
null,
ContactsContract.Data.CONTACT_ID + "=" + PhoneID + " AND "
+ ContactsContract.Data.MIMETYPE + "='"
+ ContactsContract.CommonDataKinds.Photo.CONTENT_ITEM_TYPE + "'", null,
null);
if (sCur == null) {
imgPhoto.setImageResource(android.R.drawable.sym_def_app_icon);
}
else
{
Uri uri = ContentUris.withAppendedId(ContactsContract.Contacts.CONTENT_URI, PhoneID);
InputStream input = ContactsContract.Contacts.openContactPhotoInputStream(resolver, uri);
if (input == null) {
imgPhoto.setImageResource(android.R.drawable.sym_def_app_icon);
}
else
{
imgPhoto.setImageBitmap(BitmapFactory.decodeStream(input));
}
}
sCur.close();
/*** Get Contact Name by ID ***/
Cursor cursor = getContentResolver().query(
ContactsContract.Contacts.CONTENT_URI,
null,
ContactsContract.Contacts._ID +" = ?",
new String[]{String.valueOf(PhoneID)}, null);
startManagingCursor(cursor);
if(cursor != null)
{
cursor.moveToFirst();
// Name
TextView txtName = (TextView) findViewById(R.id.ColName);
txtName.setText(cursor.getString( cursor.getColumnIndex(ContactsContract.Contacts.DISPLAY_NAME)));
// Phone No
Cursor pCur = getContentResolver().query(
ContactsContract.CommonDataKinds.Phone.CONTENT_URI,
null,
ContactsContract.CommonDataKinds.Phone.CONTACT_ID +" = ?",
new String[]{String.valueOf(PhoneID)}, null);
// Loop for Phone No
String pNo = null;
while (pCur.moveToNext()) {
String PhoneNo = pCur.getString( pCur.getColumnIndex(ContactsContract.CommonDataKinds.Phone.DATA));
String PhoneType = pCur.getString( pCur.getColumnIndex(ContactsContract.CommonDataKinds.Phone.TYPE));
if(pNo != null)
{
pNo = pNo + ", " + "(" + fPhoneType(PhoneType) + ")"+ PhoneNo;;
}
else
{
pNo = "(" + fPhoneType(PhoneType) + ")"+ PhoneNo;
}
}
pCur.close();
// Phone No
TextView txtPhone = (TextView) findViewById(R.id.ColPhoneNo);
txtPhone.setText(pNo);
// Email
Cursor eCur = getContentResolver().query(
ContactsContract.CommonDataKinds.Email.CONTENT_URI,
null,
ContactsContract.CommonDataKinds.Email.CONTACT_ID +" = ?",
new String[]{String.valueOf(PhoneID)}, null);
// Loop for Email
String eNo = null;
while (eCur.moveToNext()) {
String EmailNo = eCur.getString( eCur.getColumnIndex(ContactsContract.CommonDataKinds.Email.DATA));
String EmailType = eCur.getString( eCur.getColumnIndex(ContactsContract.CommonDataKinds.Email.TYPE));
if(eNo != null)
{
eNo = eNo + ", " + "(" + fEmailType(EmailType) + ")"+ EmailNo;;
}
else
{
eNo = "(" + fEmailType(EmailType) + ")"+ EmailNo;
}
}
eCur.close();
// Email
TextView txtEmail = (TextView) findViewById(R.id.ColEmail);
txtEmail.setText(eNo);
// Address
String addrWhere = ContactsContract.Data.CONTACT_ID + " = ? AND " + ContactsContract.Data.MIMETYPE + " = ?";
String[] addrWhereParams = new String[]{String.valueOf(PhoneID),
ContactsContract.CommonDataKinds.StructuredPostal.CONTENT_ITEM_TYPE};
Cursor addrCur = getContentResolver().query(ContactsContract.Data.CONTENT_URI,
null, addrWhere, addrWhereParams, null);
while(addrCur.moveToNext()) {
String poBox = addrCur.getString(
addrCur.getColumnIndex( ContactsContract.CommonDataKinds.StructuredPostal.POBOX));
String street = addrCur.getString(
addrCur.getColumnIndex( ContactsContract.CommonDataKinds.StructuredPostal.STREET));
String city = addrCur.getString(
addrCur.getColumnIndex( ContactsContract.CommonDataKinds.StructuredPostal.CITY));
String state = addrCur.getString(
addrCur.getColumnIndex( ContactsContract.CommonDataKinds.StructuredPostal.REGION));
String postalCode = addrCur.getString(
addrCur.getColumnIndex( ContactsContract.CommonDataKinds.StructuredPostal.POSTCODE));
String country = addrCur.getString(
addrCur.getColumnIndex( ContactsContract.CommonDataKinds.StructuredPostal.COUNTRY));
String type = addrCur.getString(
addrCur.getColumnIndex( ContactsContract.CommonDataKinds.StructuredPostal.TYPE));
TextView txtAddress = (TextView) findViewById(R.id.ColAddress);
txtAddress.setText(txtAddress.getText() + "- " + street);
}
addrCur.close();
}
cursor.close();
// btnBack
final Button Back = (Button)findViewById(R.id.btnBack);
Back.setOnClickListener(new View.OnClickListener() {
public void onClick(View arg0) {
// TODO Auto-generated method stub
Intent newActivity = new Intent(DetailActivity.this,MainActivity.class);
startActivity(newActivity);
}
});
}
public String fPhoneType(String TypeID)
{
if ("1".equals(TypeID)) {
return "Home";
} else if ("2".equals(TypeID)) {
return "Mobile";
}
return TypeID;
}
public String fEmailType(String TypeID)
{
if ("1".equals(TypeID)) {
return "Home";
} else if ("2".equals(TypeID)) {
return "Work";
}
return TypeID;
}
}
เพิ่ม DetailActivity ลงใน AndroidManifest.xml
<activity
android:name="DetailActivity"
android:theme="@style/AppTheme"
android:screenOrientation="portrait"
android:label="@string/title_activity_main" />
เพิ่มลงในไฟล์ AndroidManifest.xml ด้วย
Screenshot
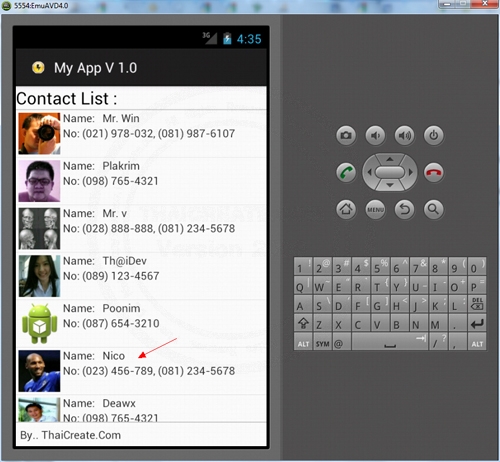
แสดงรายชื่อ Contact List บน ListView
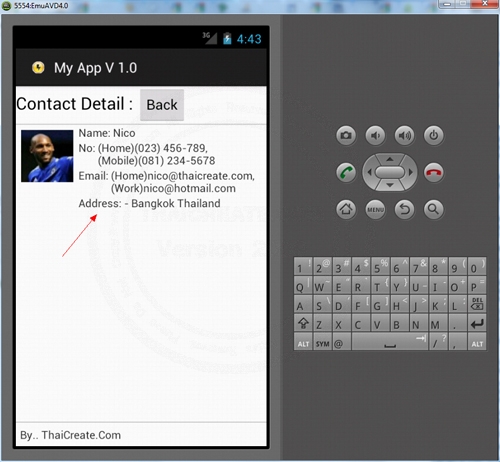
แสดงรายละเอียดของ Contact เมื่อคลิกในแต่ล่ะรายการของ ListView
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-07-28 20:20:12 /
2017-03-26 22:20:47 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|