Android Delete Data in Server via Web Service |
Android Delete Data in Server via Web Service ตัวอย่างการเขียน Android เพื่อลบ Delete ข้อมูลบน Database ที่อยู่บน Server ผ่านช่องทางของ Web Service และใช้ PHP กับ MySQL ทำงานร่วมกับ Web Service หลักการก็คือ ออกแบบรายการ Web Service ไว้ 2 ตัวคือ
- getMember.php แสดงรายการข้อมูลทั้งหมดไปยัง Client ของ Android
- deleteMemberData.php ลบรายการข้อมูลที่ได้ส่งมาจาก Android
Android and Web Service
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Web Service
member
CREATE TABLE `member` (
`MemberID` int(2) NOT NULL auto_increment,
`Username` varchar(50) NOT NULL,
`Password` varchar(50) NOT NULL,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
`Email` varchar(150) NOT NULL,
PRIMARY KEY (`MemberID`),
UNIQUE KEY `Username` (`Username`),
UNIQUE KEY `Email` (`Email`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'weerachai', 'weerachai@1', 'Weerachai Nukitram', '0819876107', '[email protected]');
INSERT INTO `member` VALUES (2, 'adisorn', 'adisorn@2', 'Adisorn Bunsong', '021978032', '[email protected]');
INSERT INTO `member` VALUES (3, 'surachai', 'surachai@3', 'Surachai Sirisart', '0876543210', '[email protected]');
INSERT INTO `member` VALUES (4, 'surapong', 'surapong@4', 'Surapong Siriphun', '0812345678', '[email protected]');

getMember.php
<?
ob_start();
require_once("lib/nusoap.php");
//Create a new soap server
$server = new soap_server();
//Define our namespace
$namespace = "https://www.thaicreate.com/android/getMember.php";
$server->wsdl->schemaTargetNamespace = $namespace;
//Configure our WSDL
$server->configureWSDL("resultMember");
// Register our method and argument parameters
$varname = array(
'strName' => "xsd:string"
);
$server->register('resultMember',$varname, array('return' => 'xsd:string'));
function resultMember($strName)
{
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM member WHERE 1 AND Name LIKE '%".$strName."%' ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
header('Content-type: application/json');
return json_encode($resultArray);
}
// Get our posted data if the service is being consumed
// otherwise leave this data blank.
$POST_DATA = isset($GLOBALS['HTTP_RAW_POST_DATA']) ? $GLOBALS['HTTP_RAW_POST_DATA'] : '';
// pass our posted data (or nothing) to the soap service
$server->service($POST_DATA);
exit();
?>
deleteMemberData.php
<?
ob_start();
require_once("lib/nusoap.php");
//Create a new soap server
$server = new soap_server();
//Define our namespace
$namespace = "https://www.thaicreate.com/android/deleteMemberData.php";
$server->wsdl->schemaTargetNamespace = $namespace;
//Configure our WSDL
$server->configureWSDL("deleteMember");
// Register our method and argument parameters
$varname = array(
'strMemberID' => "xsd:string"
);
$server->register('deleteMember',$varname, array('return' => 'xsd:string'));
function deleteMember($strMemberID)
{
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "DELETE FROM member WHERE 1 AND MemberID = '".$strMemberID."' ";
$objQuery = mysql_query($strSQL);
if(!$objQuery)
{
$arr["StatusID"] = "0";
$arr["Error"] = "Cannot delete data!";
}
else
{
$arr["StatusID"] = "1";
$arr["Error"] = "";
}
/**
$arr['StatusID'] // (0=Failed , 1=Complete)
$arr['Error' // Error Message
*/
mysql_close($objConnect);
header('Content-type: application/json');
return json_encode($arr);
}
// Get our posted data if the service is being consumed
// otherwise leave this data blank.
$POST_DATA = isset($GLOBALS['HTTP_RAW_POST_DATA']) ? $GLOBALS['HTTP_RAW_POST_DATA'] : '';
// pass our posted data (or nothing) to the soap service
$server->service($POST_DATA);
exit();
?>
Android Project
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
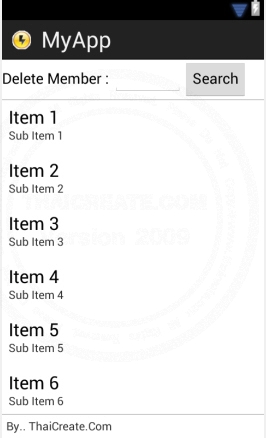
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Delete Member : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtKeySearch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="4" >
</EditText>
<Button
android:id="@+id/btnSearch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Search" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<ImageButton
android:id="@+id/btnDelete"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@android:drawable/ic_notification_clear_all" />
<TextView
android:id="@+id/ColMemberID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.2"
android:text="CustomerID"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1.0"
android:text="Name"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/ColTel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Tel"
android:textAppearance="?android:attr/textAppearanceSmall" />
</LinearLayout>
MainActivity.java
package com.myapp;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
import org.xmlpull.v1.XmlPullParserException;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.Context;
import android.content.DialogInterface;
import android.graphics.Color;
import android.view.LayoutInflater;
import android.view.View;
import android.view.Menu;
import android.view.ViewGroup;
import android.view.inputmethod.InputMethodManager;
import android.widget.BaseAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageButton;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends Activity {
// for List Data
private final String NAMESPACE1 = "https://www.thaicreate.com/android/getMember.php";
private final String URL1 = "https://www.thaicreate.com/android/getMember.php?wsdl"; // WSDL URL
private final String SOAP_ACTION1 = "https://www.thaicreate.com/android/getCustomer.php/resultMember";
private final String METHOD_NAME1 = "resultMember"; // Method on web service
// for Delete Data
private final String NAMESPACE2 = "https://www.thaicreate.com/android/deleteMemberData.php";
private final String URL2 = "https://www.thaicreate.com/android/deleteMemberData.php?wsdl"; // WSDL URL
private final String SOAP_ACTION2 = "https://www.thaicreate.com/android/deleteMemberData.php/deleteMember";
private final String METHOD_NAME2 = "deleteMember"; // Method on web service
ArrayList<HashMap<String, String>> MyArrList;
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
ShowData();
// btnSearch
final Button btnSearch = (Button) findViewById(R.id.btnSearch);
//btnSearch.setBackgroundColor(Color.TRANSPARENT);
// Perform action on click
btnSearch.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
ShowData();
}
});
}
public void ShowData()
{
// listView1
final ListView lisView1 = (ListView)findViewById(R.id.listView1);
// keySearch
EditText strKeySearch = (EditText)findViewById(R.id.txtKeySearch);
// Disbled Keyboard auto focus
InputMethodManager imm = (InputMethodManager)getSystemService(
Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(strKeySearch.getWindowToken(), 0);
SoapObject request = new SoapObject(NAMESPACE1, METHOD_NAME1);
request.addProperty("strName", strKeySearch.getText().toString());
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(
SoapEnvelope.VER11);
envelope.setOutputSoapObject(request);
HttpTransportSE androidHttpTransport = new HttpTransportSE(URL1);
try {
androidHttpTransport.call(SOAP_ACTION1, envelope);
SoapObject result = (SoapObject) envelope.bodyIn;
if (result != null) {
/**
* [{"MemberID":"1","Username":"weerachai","Password":"weerachai@1","Name":"Weerachai Nukitram","Tel":"0819876107","Email":"[email protected]"},
* {"MemberID":"2","Username":"adisorn","Password":"adisorn@2","Name":"Adisorn Bunsong","Tel":"021978032","Email":"[email protected]"},
* {"MemberID":"3","Username":"surachai","Password":"surachai@3","Name":"Surachai Sirisart","Tel":"0876543210","Email":"[email protected]"}]
*/
MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
JSONArray data = new JSONArray(result.getProperty(0).toString());
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("MemberID", c.getString("MemberID"));
map.put("Username", c.getString("Username"));
map.put("Password", c.getString("Password"));
map.put("Name", c.getString("Name"));
map.put("Email", c.getString("Email"));
map.put("Tel", c.getString("Tel"));
MyArrList.add(map);
}
lisView1.setAdapter(new ImageAdapter(this));
} else {
Toast.makeText(getApplicationContext(),
"Web Service not Response!", Toast.LENGTH_LONG)
.show();
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (XmlPullParserException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public class ImageAdapter extends BaseAdapter
{
private Context context;
public ImageAdapter(Context c)
{
// TODO Auto-generated method stub
context = c;
}
public int getCount() {
// TODO Auto-generated method stub
return MyArrList.size();
}
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
public View getView(final int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// imgCmdDelete
ImageButton cmdDelete = (ImageButton) convertView.findViewById(R.id.btnDelete);
cmdDelete.setBackgroundColor(Color.TRANSPARENT);
final AlertDialog.Builder adb1 = new AlertDialog.Builder(MainActivity.this);
final AlertDialog.Builder adb2 = new AlertDialog.Builder(MainActivity.this);
cmdDelete.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
adb1.setTitle("Delete?");
adb1.setMessage("Are you sure delete [" + MyArrList.get(position).get("Name") +"]");
adb1.setNegativeButton("Cancel", null);
adb1.setPositiveButton("Ok", new AlertDialog.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
// Request to Delete data.
SoapObject request = new SoapObject(NAMESPACE2, METHOD_NAME2);
request.addProperty("strMemberID", MyArrList.get(position).get("MemberID"));
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(
SoapEnvelope.VER11);
envelope.setOutputSoapObject(request);
HttpTransportSE androidHttpTransport = new HttpTransportSE(URL2);
try {
androidHttpTransport.call(SOAP_ACTION2, envelope);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (XmlPullParserException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
SoapObject result = (SoapObject) envelope.bodyIn;
/** Get result delete data from Server (Return the JSON Code)
* StatusID = ? [0=Failed,1=Complete]
* Error = ? [On case error return custom error message]
*
* Eg Login Failed = {"StatusID":"0","Error":"Cannot delete data!"}
* Eg Login Complete = {"StatusID":"1","Error":""}
*/
String strStatusID = "0";
String strError = "Unknow Status";
try {
JSONObject c = new JSONObject(result.getProperty(0).toString());
strStatusID = c.getString("StatusID");
strError = c.getString("Error");
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Prepare Delete
if(strStatusID.equals("0"))
{
// Dialog
adb2.setTitle("Error! ");
adb2.setIcon(android.R.drawable.btn_star_big_on);
adb2.setPositiveButton("Close", null);
adb2.setMessage(strError);
adb2.show();
}
else
{
Toast.makeText(MainActivity.this, "Delete data successfully.", Toast.LENGTH_SHORT).show();
ShowData(); // reload data again
}
}});
adb1.show();
}
});
// ColMemberID
TextView txtMemberID = (TextView) convertView.findViewById(R.id.ColMemberID);
txtMemberID.setPadding(10, 0, 0, 0);
txtMemberID.setText(MyArrList.get(position).get("MemberID") +".");
// R.id.ColName
TextView txtName = (TextView) convertView.findViewById(R.id.ColName);
txtName.setPadding(5, 0, 0, 0);
txtName.setText(MyArrList.get(position).get("Name"));
// R.id.ColTel
TextView txtTel = (TextView) convertView.findViewById(R.id.ColTel);
txtTel.setPadding(5, 0, 0, 0);
txtTel.setText(MyArrList.get(position).get("Tel"));
return convertView;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
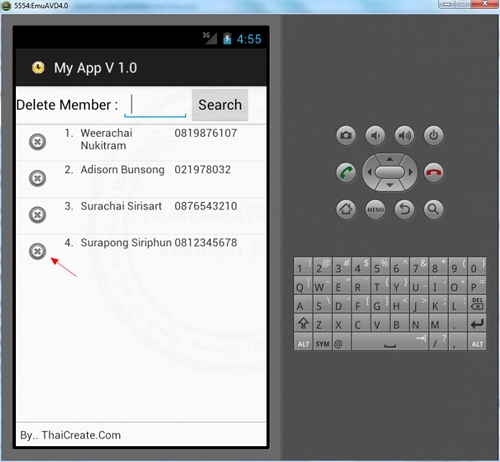
แสดงรายการจาก Web Service โดยแสดงผลบน ListView
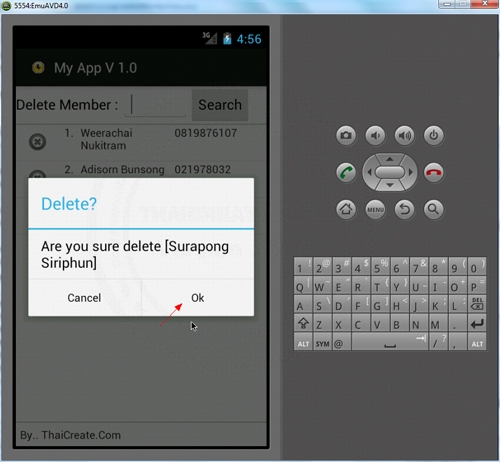
คลิกเลือกลบรายการที่ต้องการ
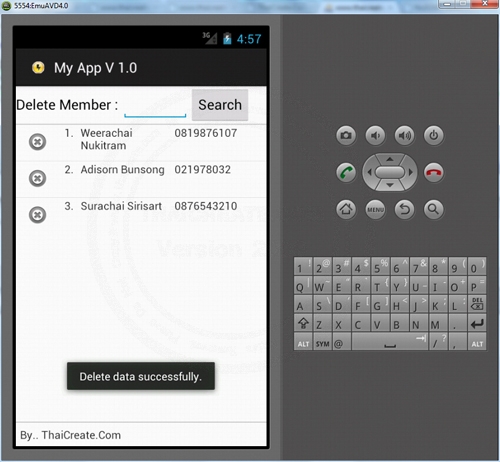
เมื่อรายการถูกลบเรียบร้อยแล้ว ข้อมูลก็จะหายไปดังภาพประกอบ

เมื่อตรวจสอบข้อมูลในฝั่งของ Web Service ข้อมูลที่ลบก็จะหายไปจาก Database

Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-08-11 16:26:38 /
2012-08-13 15:59:24 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|