Android Download Save file from Server |
Android Download Save file from Server ตัวอย่างการเขียน Android เพื่อ Download ไฟล์ที่อยู่บน Web Server และทำการ Save ไฟล์ลงใน SD Card บนเครื่อง Emulator หรือบน Smart Phone ที่ทำงานบน Android
รูป Model การทำงานของ Android ในการดาวน์โหลด Save ไฟล์จาก Web Server ที่สามารถเข้าใจได้แบบง่าย ๆ
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Example 1 การสร้าง Android เพื่อดาวน์โหลดไฟล์จาก Server แบบง่าย ๆ
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml
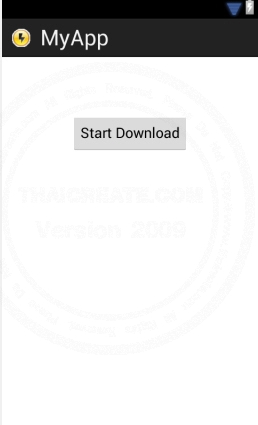
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<Button
android:id="@+id/btnDownload"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="72dp"
android:text="Start Download" />
</RelativeLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLConnection;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends Activity {
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
Button btnDownload = (Button) findViewById(R.id.btnDownload);
btnDownload.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
int count;
String from = "https://www.thaicreate.com/android/myfile.zip";
String path = "/mnt/sdcard/mydata/";
try {
URL url = new URL(from);
URLConnection conexion = url.openConnection();
conexion.connect();
int lenghtOfFile = conexion.getContentLength(); // Size of file
InputStream input = new BufferedInputStream(url.openStream());
String fileName = from.substring(from.lastIndexOf('/')+1, from.length());
OutputStream output = new FileOutputStream(path+fileName); // save to parh sd card
byte data[] = new byte[1024];
while ((count = input.read(data)) != -1) {
output.write(data, 0, count);
}
output.flush();
output.close();
input.close();
Toast.makeText(MainActivity.this,"File has been downloaded. " ,Toast.LENGTH_LONG).show();
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
String from = "https://www.thaicreate.com/android/myfile.zip";
String path = "/mnt/sdcard/mydata/";
Path ของไฟล์ที่อยู่บน Web Server และ Path ของ SD Card ที่จะถูกจัดเก็บ
Screenshot
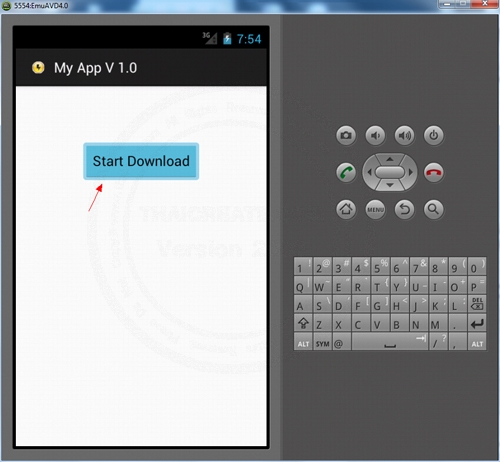
คลิกที่ Start Download
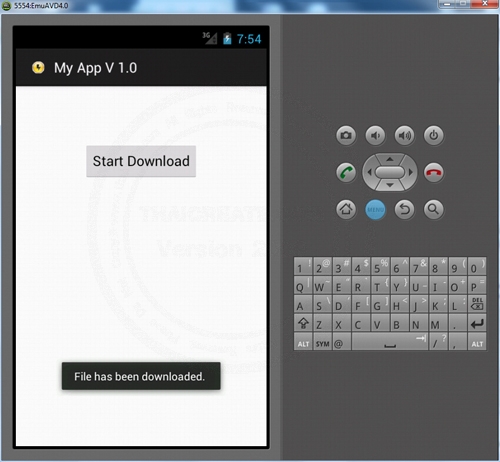
จากนั้นรอซะพัก ในขณะที่โปรแกรมกำลังดาวน์โหลดและ Save ไฟล์ลงใน SD Card
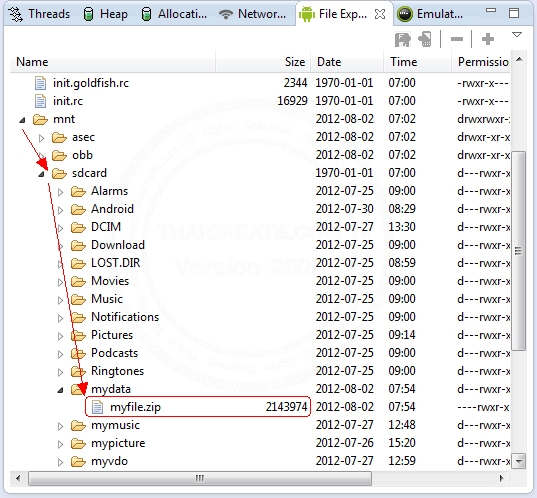
เมื่อดาวน์โหลดเรียบร้อยแล้ว เมื่อตรวจสอบไฟล์บน SD Card ที่กำหนด ก็จะพบกับไฟล์ที่ได้ดาวน์โหลด
สำหรับ Code สามารถศึกษาได้จาก Code Java ที่ได้เขียนไว้ในข้างต้น ซึ่งสามารถเข้าใจได้โดยไม่ยาก
Example 2 แสดง ProgressDialog และเปอร์เซ็นในขณะที่กำลัง Download
ในตัวอย่างที่ 2 จะใช้ AsyncTask เข้ามาจัดการ ProgressDialog ในขณะที่โปรแกรมกำลังทำงาน และการใช้ AsyncTask หน้าจอของ Application จะไม่ค้าง
Android AsyncTask and ProgressBar
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml
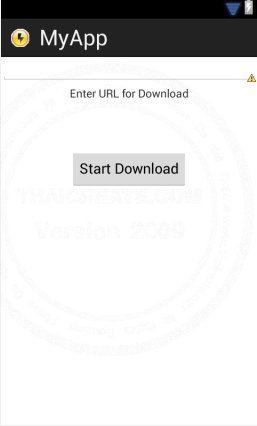
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<Button
android:id="@+id/btnDownload"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="192dp"
android:text="Start Download" />
<EditText
android:id="@+id/editText1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginTop="90dp"
android:ems="10"
android:gravity="center"
android:textSize="12dp" />
<TextView
android:id="@+id/textView1"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginTop="50dp"
android:gravity="center"
android:text="Enter URL for Download" />
</RelativeLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedInputStream;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URL;
import java.net.URLConnection;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends Activity {
public static final int DIALOG_DOWNLOAD_PROGRESS = 0;
private Button startBtn;
private ProgressDialog mProgressDialog;
private String URLDownload;
private EditText txtURL;
/** Called when the activity is first created. */
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// btnDownload
startBtn = (Button)findViewById(R.id.btnDownload);
startBtn.setOnClickListener(new OnClickListener(){
public void onClick(View v) {
startDownload();
}
});
}
private void startDownload() {
// editText1
txtURL = (EditText)findViewById(R.id.editText1);
URLDownload = txtURL.getText().toString();
new DownloadFileAsync().execute(URLDownload);
}
@Override
protected Dialog onCreateDialog(int id) {
switch (id) {
case DIALOG_DOWNLOAD_PROGRESS:
mProgressDialog = new ProgressDialog(this);
mProgressDialog.setMessage("Downloading file..");
mProgressDialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
mProgressDialog.setCancelable(false);
mProgressDialog.show();
return mProgressDialog;
default:
return null;
}
}
class DownloadFileAsync extends AsyncTask<String, String, String> {
@Override
protected void onPreExecute() {
super.onPreExecute();
showDialog(DIALOG_DOWNLOAD_PROGRESS);
}
@Override
protected String doInBackground(String... aurl) {
int count;
try {
URL url = new URL(aurl[0]);
URLConnection conexion = url.openConnection();
conexion.connect();
int lenghtOfFile = conexion.getContentLength();
Log.d("ANDRO_ASYNC", "Lenght of file: " + lenghtOfFile);
InputStream input = new BufferedInputStream(url.openStream());
// Get File Name from URL
String fileName = URLDownload.substring( URLDownload.lastIndexOf('/')+1, URLDownload.length() );
OutputStream output = new FileOutputStream("/sdcard/MyData/"+fileName);
byte data[] = new byte[1024];
long total = 0;
while ((count = input.read(data)) != -1) {
total += count;
publishProgress(""+(int)((total*100)/lenghtOfFile));
output.write(data, 0, count);
}
output.flush();
output.close();
input.close();
} catch (Exception e) {}
return null;
}
protected void onProgressUpdate(String... progress) {
Log.d("ANDRO_ASYNC",progress[0]);
mProgressDialog.setProgress(Integer.parseInt(progress[0]));
}
@Override
protected void onPostExecute(String unused) {
dismissDialog(DIALOG_DOWNLOAD_PROGRESS);
}
}
}
Screenshot
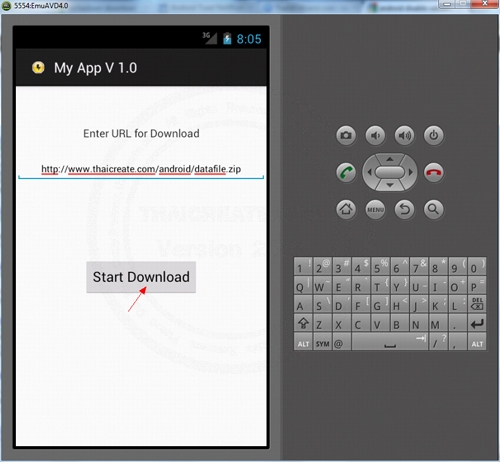
กรอก URL ของไฟล์ที่ต้องการดาวน์โหลด
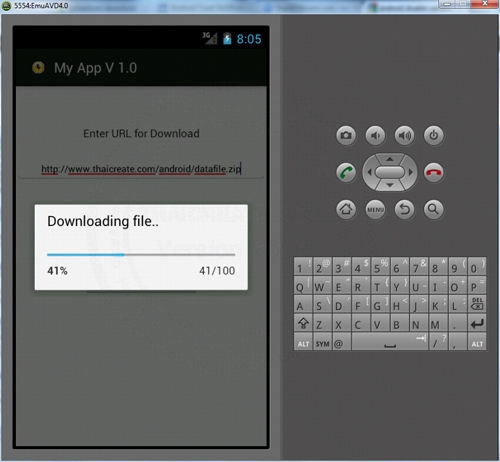
แสดงสถานะและเปอร์เซ็นต์ของการทำงานในขณะที่ดาวน์โหลดไฟล์อยู่
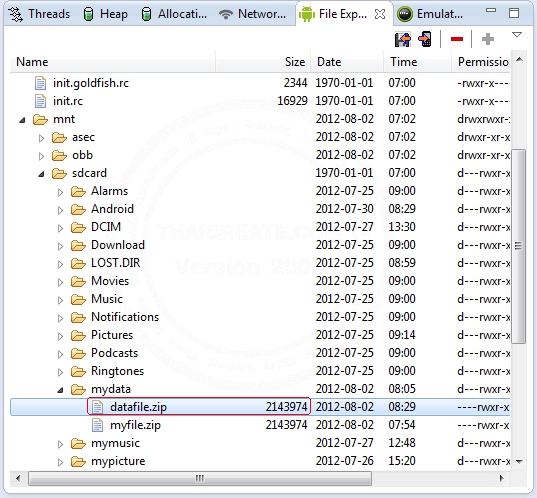
เมื่อโหลดเสร็จสิ้นไฟล์ก็จะอยู่ไฟล์ SD Card ตาม Path ที่กำหนด
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-07-03 17:19:23 /
2017-03-26 21:37:04 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|