พื้นฐาน Android Gestures Swipe/flip Sliding ตรวจสอบการ Sliding บนหน้าจอ |
Android Gestures Swipe/flip Sliding ในการเขียน Application บน Smartphone สิ่งที่ขาดไม่ได้คือการตรวจสอบระบบ Touch Screen ซึ่งจะเป็น Event ที่ทำงานควบคู่ไปกับ Application ต่าง ๆ ที่พัฒนาขึ้น เพราะไม่ว่าจะเป็น iOS , Windows Phone หรือ Android ก็มีความสามารถและการทำงานแทบไม่ต่างกัน ขึ้นอยู่กับว่าจะเขียนควบคุม Gestures หรือรุปแบบต่าง ๆ ที่เกิดขึ้นใน Touch Screen นั้นว่าจะให้ทำอะไร
Screenshot Gestures
สำหรับ Android ในการตรวจสอบ Gestures ที่เกิดขึ้นในระบบ Touch Screen จะมี Widgets ที่รองรับการพัฒนาโปรแกรมอยู่ 2 ตัวคือ GestureDetector และ GestureOverlayView โดยที่ GestureDetector จะเป็นการสร้าง Event ที่ใช้ method ต่าง ๆ เข้ามาตรวจสอบ เช่น onFling, onDown, onLongPress, onScroll, onShowPress และ onSingleTapUp โดย method เหล่านี้จะ implement มาอัตโนมัติหลังจากที่ทำการ implements OnGestureListener ตัวอย่าง
public class MainActivity extends Activity implements OnGestureListener {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
gd = new GestureDetector(this, this);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
if (gd.onTouchEvent(event))
return true;
else
return false;
}
public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX,
float velocityY) {
// TODO Auto-generated method stub
return true;
}
public boolean onDown(MotionEvent e) {
// TODO Auto-generated method stub
return false;
}
public void onLongPress(MotionEvent e) {
// TODO Auto-generated method stub
}
public boolean onScroll(MotionEvent e1, MotionEvent e2, float distanceX,
float distanceY) {
// TODO Auto-generated method stub
return false;
}
public void onShowPress(MotionEvent e) {
// TODO Auto-generated method stub
}
public boolean onSingleTapUp(MotionEvent e) {
// TODO Auto-generated method stub
return false;
}
}
รายการ method ที่มาจาก OnGestureListener()
และตัวที่สองคือ GestureOverlayView ตัวนี้จะใช้งานง่ายกกว่า คือ เราสามารถสร้างรูปแบบจาก Gesture Builder จากนั้นจะใช้การตรวจสอบจาก Touch Screen ว่าคล้ายถึงกับรูปแบบไหนที่เราได้สร้างไว้
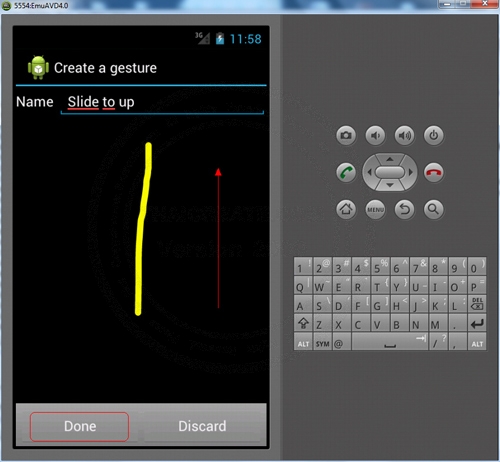
สำหรับ GestureOverlayView อ่านเพิ่มได้ที่บทความนี้
GestureOverlayView - Android Widgets Example

Example ตัวอย่างการใช้ GestureDetector
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml
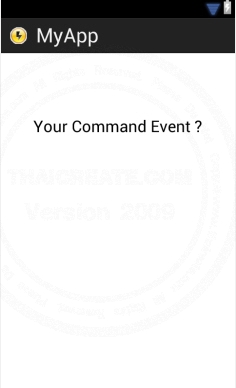
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="87dp"
android:text="Your Command Event ?"
android:textAppearance="?android:attr/textAppearanceLarge" />
</RelativeLayout>
MainActivity.java
package com.myapp;
import android.os.Bundle;
import android.view.GestureDetector;
import android.view.GestureDetector.OnGestureListener;
import android.view.Menu;
import android.view.MotionEvent;
import android.widget.TextView;
import android.widget.Toast;
import android.app.Activity;
public class MainActivity extends Activity implements OnGestureListener {
GestureDetector gd;
private static final int SWIPE_MIN_DISTANCE = 120;
private static final int SWIPE_MAX_OFF_PATH = 250;
private static final int SWIPE_THRESHOLD_VELOCITY = 100;
TextView txtView2;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
gd = new GestureDetector(this, this);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
if (gd.onTouchEvent(event))
return true;
else
return false;
}
public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX,
float velocityY) {
// TODO Auto-generated method stub
try {
if (Math.abs(e1.getY() - e2.getY()) > SWIPE_MAX_OFF_PATH)
return false;
// right to left swipe
if(e1.getX() - e2.getX() > SWIPE_MIN_DISTANCE && Math.abs(velocityX) > SWIPE_THRESHOLD_VELOCITY) {
Toast.makeText(MainActivity.this, "<---- Left Swipe", Toast.LENGTH_SHORT).show();
} else if (e2.getX() - e1.getX() > SWIPE_MIN_DISTANCE && Math.abs(velocityX) > SWIPE_THRESHOLD_VELOCITY) {
Toast.makeText(MainActivity.this, "----> Right Swipe", Toast.LENGTH_SHORT).show();
}
else if(e1.getY() - e2.getY() > SWIPE_MIN_DISTANCE && Math.abs(velocityX) > SWIPE_THRESHOLD_VELOCITY) {
Toast.makeText(MainActivity.this, "Swipe up", Toast.LENGTH_SHORT).show();
} else if (e2.getY() - e1.getY() > SWIPE_MIN_DISTANCE && Math.abs(velocityX) > SWIPE_THRESHOLD_VELOCITY) {
Toast.makeText(MainActivity.this, "Swipe down", Toast.LENGTH_SHORT).show();
}
} catch (Exception e) {
// nothing
}
return true;
}
public boolean onDown(MotionEvent e) {
// TODO Auto-generated method stub
//Toast.makeText(MainActivity.this, "onDown", Toast.LENGTH_SHORT).show();
return false;
}
public void onLongPress(MotionEvent e) {
// TODO Auto-generated method stub
Toast.makeText(MainActivity.this, "onLongPress", Toast.LENGTH_SHORT).show();
}
public boolean onScroll(MotionEvent e1, MotionEvent e2, float distanceX,
float distanceY) {
// TODO Auto-generated method stub
//Toast.makeText(MainActivity.this, "onScroll", Toast.LENGTH_SHORT).show();
return false;
}
public void onShowPress(MotionEvent e) {
// TODO Auto-generated method stub
//Toast.makeText(MainActivity.this, "onShowPress", Toast.LENGTH_SHORT).show();
}
public boolean onSingleTapUp(MotionEvent e) {
// TODO Auto-generated method stub
//Toast.makeText(MainActivity.this, "onSingleTapUp", Toast.LENGTH_SHORT).show();
return false;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
เพิ่มเติม
public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX,
float velocityY) {
// TODO Auto-generated method stub
try {
if (Math.abs(e1.getY() - e2.getY()) > SWIPE_MAX_OFF_PATH)
return false;
// right to left swipe
if(e1.getX() - e2.getX() > SWIPE_MIN_DISTANCE && Math.abs(velocityX) > SWIPE_THRESHOLD_VELOCITY) {
Toast.makeText(MainActivity.this, "<---- Left Swipe", Toast.LENGTH_SHORT).show();
} else if (e2.getX() - e1.getX() > SWIPE_MIN_DISTANCE && Math.abs(velocityX) > SWIPE_THRESHOLD_VELOCITY) {
Toast.makeText(MainActivity.this, "----> Right Swipe", Toast.LENGTH_SHORT).show();
}
else if(e1.getY() - e2.getY() > SWIPE_MIN_DISTANCE && Math.abs(velocityX) > SWIPE_THRESHOLD_VELOCITY) {
Toast.makeText(MainActivity.this, "Swipe up", Toast.LENGTH_SHORT).show();
} else if (e2.getY() - e1.getY() > SWIPE_MIN_DISTANCE && Math.abs(velocityX) > SWIPE_THRESHOLD_VELOCITY) {
Toast.makeText(MainActivity.this, "Swipe down", Toast.LENGTH_SHORT).show();
}
} catch (Exception e) {
// nothing
}
return true;
}
จาก Event ของ onFling ที่ผ่านการ implement มาจาก OnGestureListener จะไม่มี method สำหรับที่จะตรวจสอบ การ Slide ไปทิศทางต่าง ๆ แต่เราจะใช้ onFling ตรวจสอบ Event ที่เกิดขึ้น แล้วนำมาคำนวณเพื่อตรวจสอบและแสดงผล
Screenshot
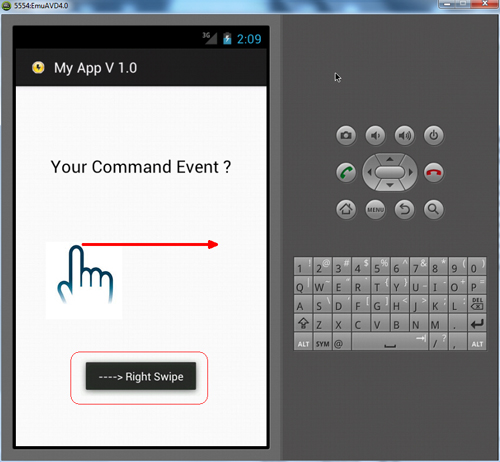
Slide Swipe ไปทางขวา (Right)
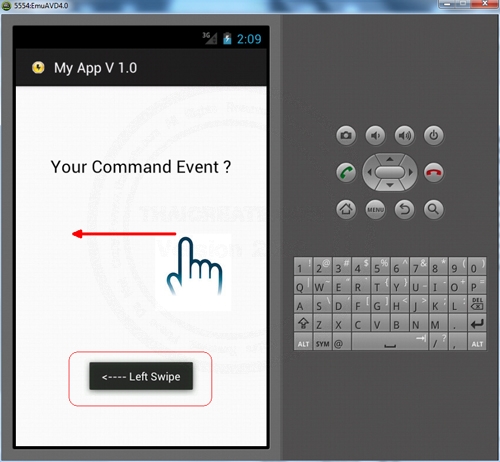
Slide Swipe ไปทางซ้าย (Left)
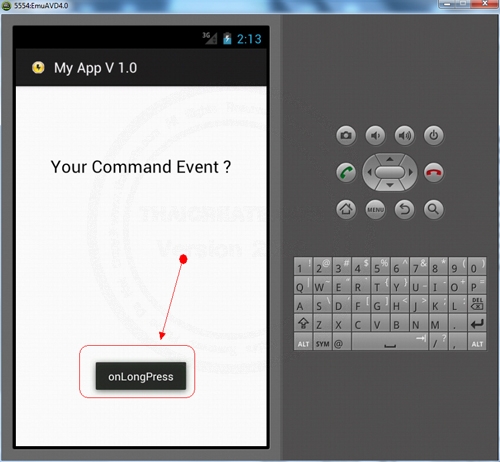
Event อื่น ๆ เช่น OnLongPress
ตัวอย่างการใช้ Android Gestures
Android Gesture on ListView Detecting Sliding Items (Flip and Swipe)
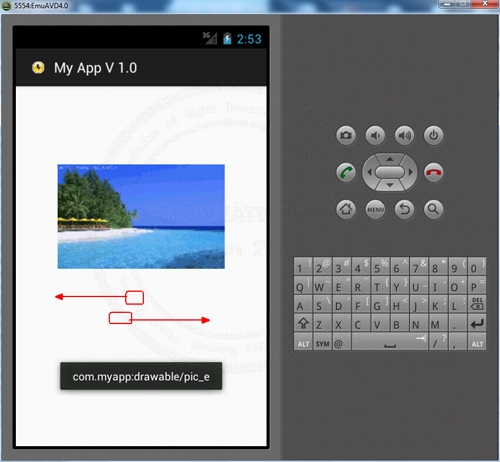
ImageSwitcher - Android Widgets Example
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-07-28 12:12:48 /
2017-03-26 20:24:15 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|