Android Get Result / Search Data from Server via Web Service |
Android Get Result / Search Data from Server via Web Service ตัวอย่างการใช้ Android เพื่ออ่านข้อมูลจาก Web Service โดยใน Web Service จะใช้ PHP กับ MySQL เปิด Service ที่จะรอการเรียกข้อมูลด้วย Android และใช้การส่งข้อมูลจาก Web Service กลับไปยัง Client ด้วย JSON
Android and Web Service
รูปอธิบายขั้นตอนการทำงานระหว่าง Android กับ Web Service และการทำงานบน PHP กับ MySQL
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Web Service
customer
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `customer`
--
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'EN', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
โครงสร้าของ MySQL Database ที่อยู่บน Web Service

getCustomer.php ไฟล์สำหรับ Web Service ที่ทำหน้าที่ส่งค่ากลับไปยัง Client ในรูปแบบของ JSON
<?php
ob_start();
require_once("lib/nusoap.php");
//Create a new soap server
$server = new soap_server();
//Define our namespace
$namespace = "https://www.thaicreate.com/android/getCustomer.php";
$server->wsdl->schemaTargetNamespace = $namespace;
//Configure our WSDL
$server->configureWSDL("resultCustomer");
// Register our method and argument parameters
$varname = array(
'strName' => "xsd:string"
);
$server->register('resultCustomer',$varname, array('return' => 'xsd:string'));
function resultCustomer($strName)
{
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM customer WHERE 1 AND Name LIKE '%".$strName."%' ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
header('Content-type: application/json');
return json_encode($resultArray);
}
// Get our posted data if the service is being consumed
// otherwise leave this data blank.
$POST_DATA = isset($GLOBALS['HTTP_RAW_POST_DATA']) ? $GLOBALS['HTTP_RAW_POST_DATA'] : '';
// pass our posted data (or nothing) to the soap service
$server->service($POST_DATA);
exit();
?>
จากไฟล์ Web Service ของ PHP จะมี Method ชื่อว่า resultCustomer โดยจะรับค่า strName มาจาก Client จากนั้นจะใช้การค้นหาข้อมูลจาก MySQL และ return ค่าทั้งหมดที่ได้กลับไปยัง Client ด้วย JSON
Android Project
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
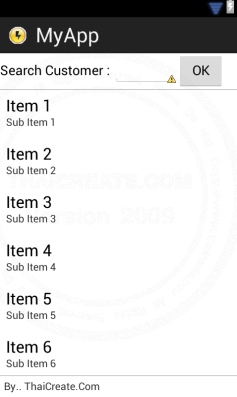
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Search Customer : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtKeySearch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="4" >
</EditText>
<Button
android:id="@+id/btnSearch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="OK" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
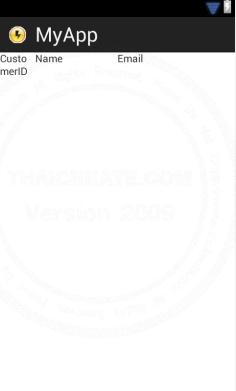
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColCustomerID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.3"
android:text="CustomerID"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.7"
android:text="Name"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/ColEmail"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Email"
android:textAppearance="?android:attr/textAppearanceSmall" />
</LinearLayout>
MainActivity.java
package com.myapp;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
import org.xmlpull.v1.XmlPullParserException;
import android.os.Bundle;
import android.os.StrictMode;
import android.view.Menu;
import android.view.View;
import android.view.inputmethod.InputMethodManager;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import android.widget.Toast;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.content.Context;
public class MainActivity extends Activity {
private final String NAMESPACE = "https://www.thaicreate.com/android/getCustomer.php";
private final String URL = "https://www.thaicreate.com/android/getCustomer.php?wsdl"; // WSDL URL
private final String SOAP_ACTION = "https://www.thaicreate.com/android/getCustomer.php/resultCustomer";
private final String METHOD_NAME = "resultCustomer"; // Method on web service
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder()
.permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// btnSearch
Button btnSearch = (Button) this.findViewById(R.id.btnSearch);
btnSearch.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// txtKeySearch
EditText txtKeySearch = (EditText) findViewById(R.id.txtKeySearch);
// Disbled Keyboard auto focus
InputMethodManager imm = (InputMethodManager)getSystemService(
Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(txtKeySearch.getWindowToken(), 0);
// listView1
final ListView lisView1 = (ListView)findViewById(R.id.listView1);
SoapObject request = new SoapObject(NAMESPACE, METHOD_NAME);
request.addProperty("seySearch", txtKeySearch.getText().toString());
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(
SoapEnvelope.VER11);
envelope.setOutputSoapObject(request);
HttpTransportSE androidHttpTransport = new HttpTransportSE(URL);
try {
androidHttpTransport.call(SOAP_ACTION, envelope);
SoapObject result = (SoapObject) envelope.bodyIn;
if (result != null) {
/** Get result from WebService (Return the JSON Code)
* [{"CustomerID":"C001","Name":"Win Weerachai","Email":"[email protected]" ,"CountryCode":"TH","Budget":"1000000","Used":"600000"},
* {"CustomerID":"C002","Name":"John Smith","Email":"[email protected]" ,"CountryCode":"EN","Budget":"2000000","Used":"800000"},
* {"CustomerID":"C003","Name":"Jame Born","Email":"[email protected]" ,"CountryCode":"US","Budget":"3000000","Used":"600000"},
* {"CustomerID":"C004","Name":"Chalee Angel","Email":"[email protected]" ,"CountryCode":"US","Budget":"4000000","Used":"100000"}]
*/
final ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
JSONArray data = new JSONArray(result.getProperty(0).toString());
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("CustomerID", c.getString("CustomerID"));
map.put("Name", c.getString("Name"));
map.put("Email", c.getString("Email"));
map.put("CountryCode", c.getString("CountryCode"));
map.put("Budget", c.getString("Budget"));
map.put("Used", c.getString("Used"));
MyArrList.add(map);
}
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(MainActivity.this, MyArrList, R.layout.activity_column,
new String[] {"CustomerID", "Name", "Email"}, new int[] {R.id.ColCustomerID, R.id.ColName, R.id.ColEmail});
lisView1.setAdapter(sAdap);
} else {
Toast.makeText(getApplicationContext(),
"Web Service not Response!", Toast.LENGTH_LONG)
.show();
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (XmlPullParserException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
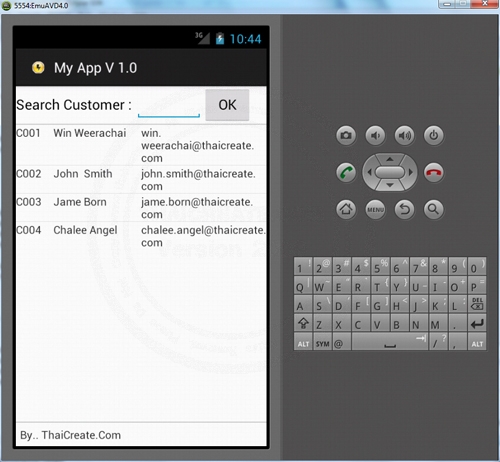
แสดงข้อมูลที่ได้จาก Web Service

เมื่อทำการค้นหาข้อมูล
|