Android Google Map : Get Address Name from Latitude,Longitude (Geocoder) |
Android Google Map : Get Address Name from Latitude,Longitude (Geocoder) ในหัวข้อนี้จะเป็นการเขียน Android กับ Google Map API ด้วยการใช้ Geocoder เพื่อค้นหา Address (ที่อยู่) จะค้นหาจากตำแหน่งพิกัดของ Latitude และ Longitude ที่ปักหมุด (Marker) อยู่บน Map ซึ่งค่า Result ที่ได้จะเป็นที่อยู่ของ Address นั้น ๆ ประกอบด้วย CountryName, CountryCode, AdminArea, PostalCode, SubAdminArea, Locality, SubThoroughfare รวมทั้งค่าอื่น ๆ ที่เกี่ยวข้อง
Android Google Map : Get Address Name from Latitude and Longitude
สำหรับตัวอย่างและ Code นี้รองรับการเขียนทั้งบนโปรแกรม Eclipse และ Android Studio
ใน AndroidManifest.xml เพิ่ม Permission ดังนี้
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="com.google.android.providers.gsf.permission.READ_GSERVICES" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
รูปแบบการใช้ Geocoder เพื่อค้นหาและแปลงค่าพิกัด Latitude, Longitude ให้เป็น Address
Geocoder and Address
Geocoder geocoder = new Geocoder(this, Locale.getDefault());
List<Address> addresses = geocoder.getFromLocation(lat, lng, 1);
Address obj = addresses.get(0);
String add = obj.getAddressLine(0);
// obj.getCountryName();
// obj.getCountryCode();
// obj.getAdminArea();
// obj.getPostalCode();
// obj.getSubAdminArea();
// obj.getLocality();
// obj.getSubThoroughfare();
Example 1 : ตัวอย่างการเขียน Android App แสดง Address จากตำแหน่งพิกัดของ Latitude และ Longitude
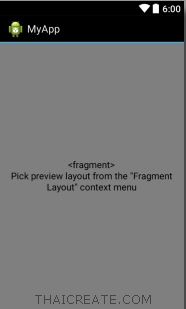
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<fragment xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/googleMap"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:name="com.google.android.gms.maps.SupportMapFragment"/>
MainActivity.java
package com.myapp;
import android.os.Bundle;
import android.support.v4.app.FragmentActivity;
import java.io.IOException;
import java.util.List;
import java.util.Locale;
import com.google.android.gms.maps.CameraUpdateFactory;
import com.google.android.gms.maps.GoogleMap;
import com.google.android.gms.maps.SupportMapFragment;
import com.google.android.gms.maps.model.BitmapDescriptorFactory;
import com.google.android.gms.maps.model.LatLng;
import com.google.android.gms.maps.model.MarkerOptions;
import android.location.Address;
import android.location.Geocoder;
import android.location.LocationListener;
import android.location.LocationManager;
public class MainActivity extends FragmentActivity {
protected LocationManager locationManager;
protected LocationListener locationListener;
// Google Map
private GoogleMap googleMap;
// Latitude & Longitude
private Double Latitude = 0.00;
private Double Longitude = 0.00;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// *** Display Google Map
googleMap = ((SupportMapFragment) getSupportFragmentManager()
.findFragmentById(R.id.googleMap)).getMap();
// ** Latitude & Longitude
Latitude = 13.827496;
Longitude = 100.566949;
googleMap.clear();
// *** Focus & Zoom
LatLng coordinate = new LatLng(Latitude, Longitude);
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_HYBRID);
googleMap.animateCamera(CameraUpdateFactory.newLatLngZoom(coordinate, 17));
// *** Marker
MarkerOptions marker = new MarkerOptions().position(new LatLng(Latitude, Longitude))
.title(getAddress(Latitude, Longitude));
marker.icon(BitmapDescriptorFactory.fromResource(R.drawable.marker));
googleMap.addMarker(marker);
}
public String getAddress(double lat, double lng) {
Geocoder geocoder = new Geocoder(this, Locale.getDefault());
try {
List<Address> addresses = geocoder.getFromLocation(lat, lng, 1);
Address obj = addresses.get(0);
String add = obj.getAddressLine(0);
add = add + "\n" + obj.getCountryName();
add = add + "\n" + obj.getCountryCode();
add = add + "\n" + obj.getAdminArea();
add = add + "\n" + obj.getPostalCode();
add = add + "\n" + obj.getSubAdminArea();
add = add + "\n" + obj.getLocality();
add = add + "\n" + obj.getSubThoroughfare();
return add;
// TennisAppActivity.showDialog(add);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
return "";
}
}
}
จาก Code นี้จะเป็นการสร้าง Location หลาย ๆ ที่แล้วจัดเก็บลงใน ArrayList จากนั้นค่อยมา Loop เพื่อปักหมุดลงใน Google Map
Screenshot
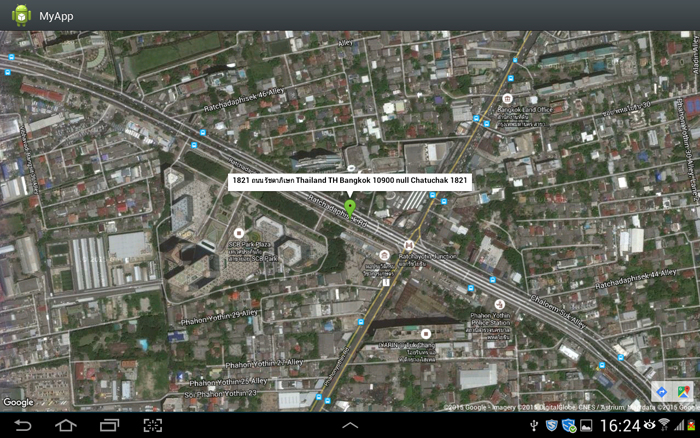
แสดงตำแหน่ง Address จากค่า Latitude และ Longitude
Example 2 : การแสดงที่อยู่ Address ปัจจุบัน (Current Location)
MainActivity.java
package com.myapp;
import android.os.Bundle;
import android.support.v4.app.FragmentActivity;
import java.io.IOException;
import java.util.List;
import java.util.Locale;
import com.google.android.gms.maps.CameraUpdateFactory;
import com.google.android.gms.maps.GoogleMap;
import com.google.android.gms.maps.SupportMapFragment;
import com.google.android.gms.maps.model.BitmapDescriptorFactory;
import com.google.android.gms.maps.model.LatLng;
import com.google.android.gms.maps.model.MarkerOptions;
import android.content.Context;
import android.location.Address;
import android.location.Geocoder;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
public class MainActivity extends FragmentActivity implements LocationListener {
protected LocationManager locationManager;
protected LocationListener locationListener;
// Google Map
private GoogleMap googleMap;
// Latitude & Longitude
private Double Latitude = 0.00;
private Double Longitude = 0.00;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// *** Location
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER, 0, 0, this);
// *** Display Google Map
googleMap = ((SupportMapFragment) getSupportFragmentManager().findFragmentById(R.id.googleMap)).getMap();
}
@Override
public void onLocationChanged(Location location) {
if (location == null) {
return;
}
//** Get Latitude & Longitude
Latitude = location.getLatitude();
Longitude= location.getLongitude();
googleMap.clear();
// *** Focus & Zoom
LatLng coordinate = new LatLng(Latitude, Longitude);
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_HYBRID);
googleMap.animateCamera(CameraUpdateFactory.newLatLngZoom(coordinate, 17));
// *** Marker
MarkerOptions marker = new MarkerOptions().position(new LatLng(Latitude, Longitude))
.title(getAddress(Latitude, Longitude));
marker.icon(BitmapDescriptorFactory.fromResource(R.drawable.marker));
googleMap.addMarker(marker);
}
@Override
public void onProviderDisabled(String provider) {
// Log.d("Latitude","disable");
}
@Override
public void onProviderEnabled(String provider) {
// Log.d("Latitude","enable");
}
@Override
public void onStatusChanged(String provider, int status, Bundle extras) {
// Log.d("Latitude","status");
}
public String getAddress(double lat, double lng) {
Geocoder geocoder = new Geocoder(this, Locale.getDefault());
try {
List<Address> addresses = geocoder.getFromLocation(lat, lng, 1);
Address obj = addresses.get(0);
String add = obj.getAddressLine(0);
add = add + "\n" + obj.getCountryName();
add = add + "\n" + obj.getCountryCode();
add = add + "\n" + obj.getAdminArea();
add = add + "\n" + obj.getPostalCode();
add = add + "\n" + obj.getSubAdminArea();
add = add + "\n" + obj.getLocality();
add = add + "\n" + obj.getSubThoroughfare();
return add;
// TennisAppActivity.showDialog(add);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
return "";
}
}
}
Screenshot
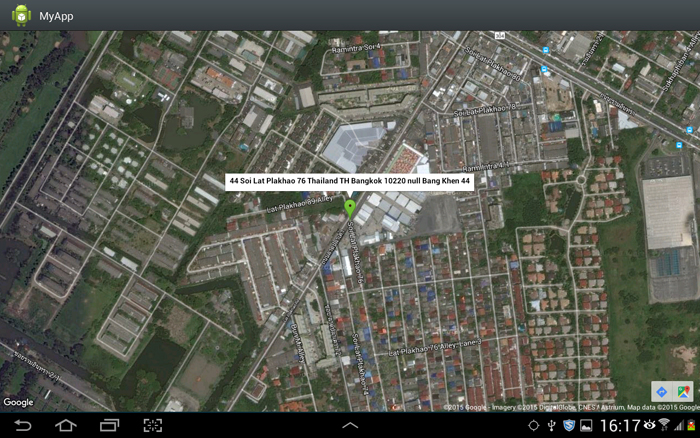
แสดงตำแหน่ง Current Address จากค่า Latitude และ Longitude
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2015-11-21 22:51:41 /
2017-03-26 21:16:36 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|