Android Google Map : Change Map Type (Normal, Satellite, Hybrid and Terrain) |
Android Google Map : Change Map Type (Normal, Satellite, Hybrid and Terrain) ในหัวข้อที่สามนี้จะเป็นการเขียน Android App เพื่อเรียกใช้งาน Google Map จะแสดงวิธีการเรียก Map Type หรือรูปแบบพื้นผิวของ Map ซึ่งในพื้นฐานประเภทของ Map ที่ได้รับความนิยมจะมีอยู่ประมาณ 4-5 ประเภทคือ None, Normal, Hybrid, Satellite และ Terrain โดยในแต่ล่ะรูปแบบก็ใช้ประโยนช์ที่แตกต่างกัน
Android Google Map : Change Map Type (Normal, Satellite, Hybrid and Terrain)
สำหรับตัวอย่างและ Code นี้รองรับการเขียนทั้งบนโปรแกรม Eclipse และ Android Studio
ใน AndroidManifest.xml เพิ่ม Permission ดังนี้
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="com.google.android.providers.gsf.permission.READ_GSERVICES" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
รูปแบบของ Map Type มีอยู่ประมาณ 4-5 รูปแบบคือ None, Normal, Hybrid, Satellite และ Terrain
Normal : NONE (ไม่แสดง Map)
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_NONE);
Normal : NORMAL (ปกติ)
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_NORMAL);
Satellite : SATELLITE (ภาพจากดาวเทียม)
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_SATELLITE);
Terrain : TERRAIN(แบบภาพภูมิศาสตร์)
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_TERRAIN);
Hybrid : HYBRID (แบบปกติผสมกับดาวเทียม)
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_HYBRID);
Example 1 : ตัวอย่างการเขียน Android App เพื่อ เปลี่ยนมุมมองลอง Map Type
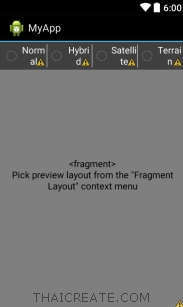
activity_main.xml
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MapActivity" >
<fragment
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/googleMap"
android:name="com.google.android.gms.maps.SupportMapFragment"
android:layout_width="fill_parent"
android:layout_height="fill_parent" />
<RadioGroup
android:id="@+id/radio_group_list_selector"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#80000000"
android:orientation="horizontal"
android:padding="4dp" >
<RadioButton
android:id="@+id/rdoNormal"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center_horizontal|center_vertical"
android:text="Normal" />
<View
android:id="@+id/VerticalLine"
android:layout_width="1dip"
android:layout_height="match_parent"
android:background="#aaa" />
<RadioButton
android:id="@+id/rdoHybrid"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center_horizontal|center_vertical"
android:text="Hybrid" />
<View
android:id="@+id/VerticalLine1"
android:layout_width="1dip"
android:layout_height="match_parent"
android:background="#aaa" />
<RadioButton
android:id="@+id/rdoSatellite"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center_horizontal|center_vertical"
android:text="Satellite" />
<View
android:id="@+id/VerticalLine2"
android:layout_width="1dip"
android:layout_height="match_parent"
android:background="#aaa" />
<RadioButton
android:id="@+id/rdoTerrain"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center_horizontal|center_vertical"
android:text="Terrain" />
</RadioGroup>
</FrameLayout>

MainActivity.java
package com.myapp;
import com.google.android.gms.maps.GoogleMap;
import com.google.android.gms.maps.SupportMapFragment;
import com.google.android.gms.maps.model.LatLng;
import android.os.Bundle;
import android.support.v4.app.FragmentActivity;
import android.view.View;
import android.widget.RadioButton;
import com.google.android.gms.maps.CameraUpdateFactory;
public class MainActivity extends FragmentActivity {
// Google Map
private GoogleMap googleMap;
// Latitude & Longitude
private Double Latitude = 13.844205;
private Double Longitude = 100.598856;
// RadioButton
RadioButton rdoNormal, rdoHybrid, rdoSatellite, rdoTerrain;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//*** Display Google Map
googleMap = ((SupportMapFragment)getSupportFragmentManager()
.findFragmentById(R.id.googleMap)).getMap();
LatLng coordinate = new LatLng(Latitude, Longitude);
//*** Focus & Zoom
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_HYBRID);
googleMap.animateCamera(CameraUpdateFactory.newLatLngZoom(coordinate, 17));
//*** Zoom Control
googleMap.getUiSettings().setRotateGesturesEnabled(true);
//*** RadioButton
rdoNormal = (RadioButton)findViewById(R.id.rdoNormal);
rdoHybrid = (RadioButton)findViewById(R.id.rdoHybrid);
rdoSatellite = (RadioButton)findViewById(R.id.rdoSatellite);
rdoTerrain = (RadioButton)findViewById(R.id.rdoTerrain);
rdoNormal.setOnClickListener(myOptionOnClickListener);
rdoHybrid.setOnClickListener(myOptionOnClickListener);
rdoSatellite.setOnClickListener(myOptionOnClickListener);
rdoTerrain.setOnClickListener(myOptionOnClickListener);
}
RadioButton.OnClickListener myOptionOnClickListener = new RadioButton.OnClickListener() {
public void onClick(View v) {
// TODO Auto-generated method stub
if(rdoNormal.isChecked())
{
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_NORMAL);
}else if(rdoHybrid.isChecked())
{
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_HYBRID);
}else if(rdoSatellite.isChecked())
{
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_SATELLITE);
}else if(rdoTerrain.isChecked())
{
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_TERRAIN);
}
}
};
}
Screenshot

แสดง Radibo Button สำหรับเปลี่ยน Map Type ต่าง ๆ
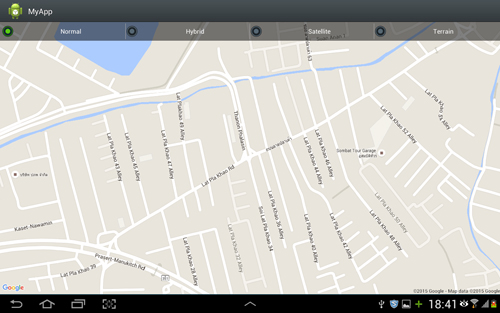
Map Type แบบ Normal
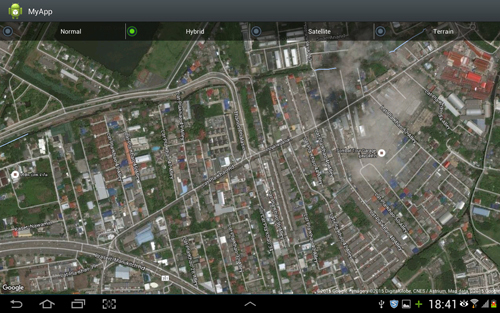
Map Type แบบ Hybrid
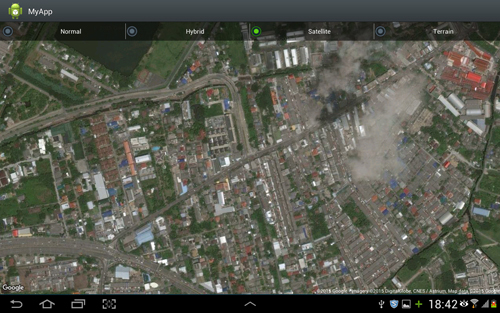
Map Type แบบ Satellite
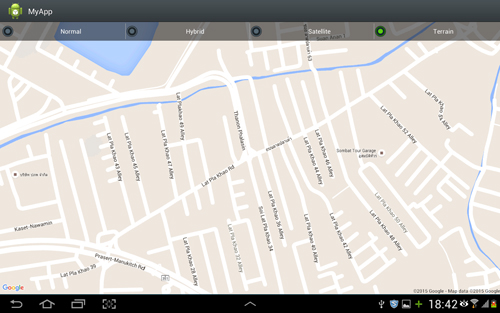
Map Type แบบ Terrain
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2015-11-21 22:49:44 /
2017-03-26 21:14:30 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|