Android HttpGet and HttpPost |
Android HttpGet and HttpPost ในการเขียนโปรแกรมบน Android เพื่อติดต่ออ่านค่าต่าง ๆ ที่อยู่ใน Server นั้น สามารถทำได้ง่าย ๆ ไม่ต้องอาศัยผ่าน Web Service ด้วยการใช้ HttpGet และ HttpPost ซึ่งทั้ง 2 ตัวนี้เป็นการทำงานแบบ REST จะมีรูปแบบการทำงานต่างกันนิดหน่อย โดยการอ่านวิธีนี้ในฝั่ง Web Server จะต้องสร้างเป็น URL สำหรับทำการแสดงข้อมูล และ Android จะอ่านข้อมูลจาก URL นั้น ๆ โดยส่ง Request ไปยัง Web Server และ Web Server จะ Response ค่าออกมา จะได้รูปแบบที่ต้องการออกมาเป็นข้อความหรือ String วิธีนี้ถ้าเคยเขียน Ajax ที่ทำงานบนเว็บไซต์จะเข้าใจได้เลยในทันที วิธีการใช้งานและส่งค่าไม่ต่างกัน ซึ่งในฝั่งของ Web Server จะทำงานด้วยภาษาอะไรก็ตาม เช่น PHP/ASP/ASP.NET หรือ JSP
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Android and HttpGet
สำหรับ HttpGet เป็นการเขียนโปรแกรม Android เพื่ออ่านและดึงข้อมูลจาก URL ของ Website ที่อยู่ในรูปแบบของ Request-URI โดยจะอ่านค่า Resource ที่ได้จากผลลัพธ์ของ URL นั้น ๆ ที่ได้ประมวลผลเสร้จสิ้นแล้ว
จากภาพประกอบจะเห็นว่า Android จะทำการ Request ไปยัง Web Server ผ่าน HTTP จากนั้น Web Server จะ Response ค่ากลับมา
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
HttpResponse response = client.execute(httpGet);
รูปแบบการติดต่อกับ Web Server ผ่าน HttpGet
Example HttpGet ตัวอย่างการติดต่อข้อมูลกับ Web Server ผ่าน HttpGet
getHttpGet.php
<?php
echo "Server Date/Time : ".date("Y-m-d H:i:s");
?>
สร้างไฟล์ php ที่อยู่บน Server ดังนี้
Server Date/Time : 2012-07-31 21:00:13
ซึ่งผลลัพธ์ที่ได้จาก Web Server คือ
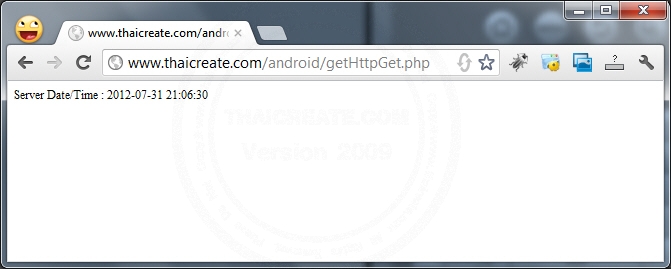
Android Project
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml
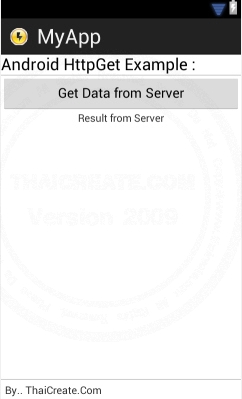
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Android HttpGet Example :"
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<Button
android:id="@+id/btnGetData"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Get Data from Server" />
<TextView
android:id="@+id/txtResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Result from Server"
android:gravity="center_horizontal" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>

MainActivity.java
package com.myapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends Activity {
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// txtResult
final TextView result = (TextView)findViewById(R.id.txtResult);
// btnGetData
final Button getData = (Button) findViewById(R.id.btnGetData);
// Perform action on click
getData.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String url = "https://www.thaicreate.com/android/getHttpGet.php";
String resultServer = getHttpGet(url);
result.setText(resultServer);
}
});
}
public String getHttpGet(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Status OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
คำอธิบาย
ใน Web Server จะมีไฟล์ php ซึ่งจัดเก็บไว้ที่
https://www.thaicreate.com/android/getHttpGet.php
และจะใช้ Android อ่านผลลัพธ์ที่ได้จาก URL นี้
Screenshot
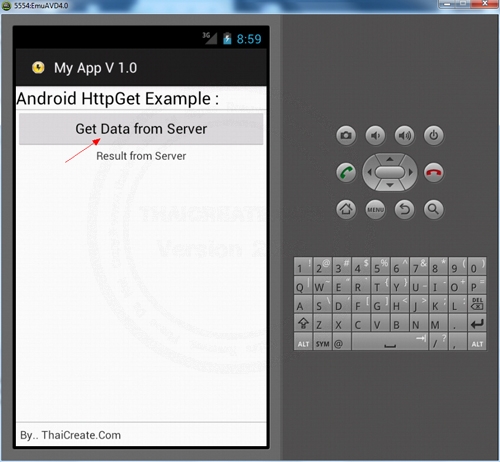
ทดสอบการ Get Data from Server
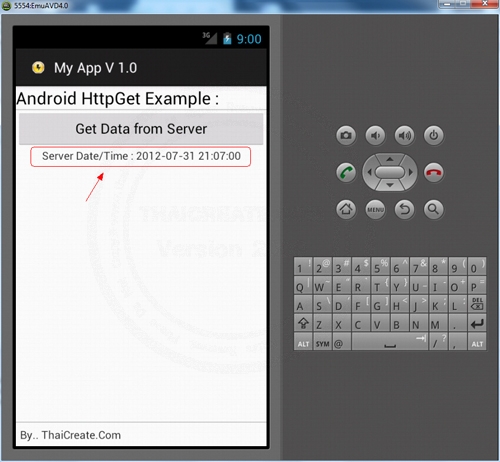
สิ่งที่ได้จาก Web Server
Android and HttpPost
สำหรับ HttpPost จะมีรูปแบบการติดต่อกับ Web Server เหมือนกับ HttpGet แต่จะต่างกันตรงที่ HttpPost สามารถส่งค่า Param หรือ Parameters ผ่าน Method POST ซึ่งฝั่ง Server สามารถใช้การอ่านเหมือนกับรูปแบบของการอ่านค่าจาก Hidden field เช่น
PHP
$_POST["name"];
ASP/ASP.NET
Request.Form("name")
โดยหลังจากที่ Android ได้ส่งค่าไปพร้อมกับ Request เมื่อฝั่ง Server สามรถรับค่าได้แล้วก็จะทำค่าที่ได้ไปใช้ พร้อม ๆ กับการ Response ส่งค่ากลับมาให้กับ Request ของ Android
จากตัวอย่างจะเห็นว่า Android ทำการ Request ผ่าน HTTP ในรูปแบบของ Method POST และฝั่ง Server รับค่า POST และส่งค่ากลับมายัง Android
HttpClient client = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("strA", txtA.getText().toString()));
params.add(new BasicNameValuePair("strB", txtB.getText().toString()));
httpPost.setEntity(new UrlEncodedFormEntity(params));
HttpResponse response = client.execute(httpPost);
รูปแบบการติดต่อกับ Web Server ผ่าน HttpPost
Example HttpPost ตัวอย่างการส่งค่าผ่าน HttpPost และการรับค่าจาก Web Server
getHttpPost.php
<?php
echo "A+B =".($_POST["strA"]+$_POST["strB"]);
?>
ออกแบบคำสั่งของ php ในฝั่ง Web Server รับค่า 2 ตัวคือ strA และ strB จากนั้นนำ 2 ค่านี้มาบวกกัน พร้อมกับส่งค่า Result Response กลับไปยัง Android Client
Android Project
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml
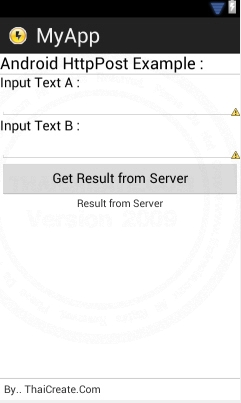
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Android HttpPost Example :"
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Text A :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtA"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" >
</EditText>
<TextView
android:id="@+id/textView4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Text B :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtB"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" >
</EditText>
<Button
android:id="@+id/btnGetData"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Get Result from Server" />
<TextView
android:id="@+id/txtResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Result from Server"
android:gravity="center_horizontal" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends Activity {
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// txtA & txtB
final EditText txtA = (EditText)findViewById(R.id.txtA);
final EditText txtB = (EditText)findViewById(R.id.txtB);
// txtResult
final TextView result = (TextView)findViewById(R.id.txtResult);
// btnGetData
final Button getData = (Button) findViewById(R.id.btnGetData);
// Perform action on click
getData.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String url = "https://www.thaicreate.com/android/getHttpPost.php";
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("strA", txtA.getText().toString()));
params.add(new BasicNameValuePair("strB", txtB.getText().toString()));
String resultServer = getHttpPost(url,params);
result.setText(resultServer);
}
});
}
public String getHttpPost(String url,List<NameValuePair> params) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
try {
httpPost.setEntity(new UrlEncodedFormEntity(params));
HttpResponse response = client.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Status OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
คำอธิบาย
ใน Web Server จะมีไฟล์ php ซึ่งจัดเก็บไว้ที่
https://www.thaicreate.com/android/getHttpPost.php
และจะใช้ Android จะส่งค่า POST และอ่านค่า Result จาก URL นี้
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("strA", txtA.getText().toString()));
params.add(new BasicNameValuePair("strB", txtB.getText().toString()));
ใน Android จะเห็นว่ามีการส่งค่าตัวแปรชื่อ strA และ strB เพราะฉันนั้นในฝั่งของ Web Server ที่เป็น PHP จะสามารถอ่านค่าตัวแปรนี้ได้ในทันที
Screenshot
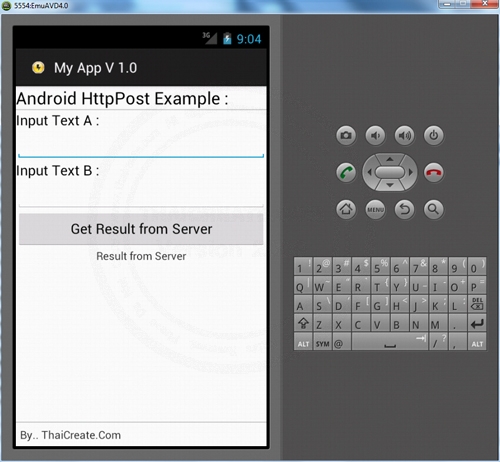
กรอกข้อมูลทั้ง 2 ช่อง
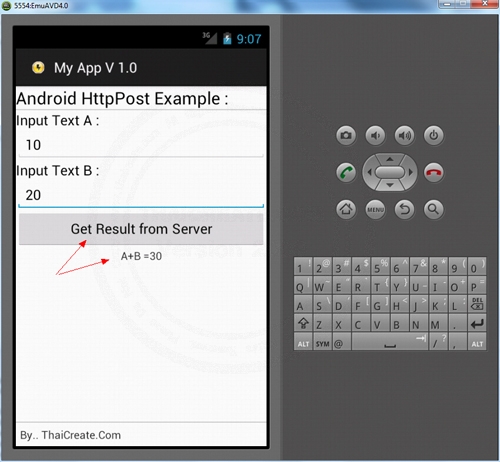
สิ่งที่ Web Server ส่งค่ากลับมา ซึ่งสามารถเขียนเงื่อนไขต่าง ๆ ได้จากไฟล์ของ PHP ที่อยู่ในฝั่ง Web Server
สรุป
จะเห็นว่า Android นั้นสามารถติดต่อกับ Web Server ได้อย่างง่าย ๆ ซึ่งจาก 2 ตัวอย่างที่ได้ยกเป็นตัวอย่างประกอบนั้น สามารถที่จะ Apply กับการโปรแกรมต่าง ๆ ที่มีความซับซ้อนได้อย่างมากมาย แต่การใช้งานผ่าน HttpGet กับ HttpPost นั้น จะมีความเสี่ยงในด้านความปลอดภัย เพาะฉะนั้นในการรับส่งค่าจาก Android กับ Web Server ควรจะส่งค่า Parameters เพื่อจะตรวจสอบระหว่าง Client กับ Web Server นั้นเป็นการเชื่อมต่อที่ถูกต้อง เช่น ถ้ามีการติดต่อข้อมูลของสมาชิกต่าง ๆ ก็ควรจะส่ง Username และ Password ไปตรวจสอบด้วยทุกครั้ง
Android HttpPost / HttpGet (UTF-8 Encoding)
Android PHP/MySQL (UTF-8) : รับ-ส่งค่าภาษาไทย ระหว่าง Android กับ MySQL
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-08-11 13:52:56 /
2017-03-26 22:17:44 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|