Android and Image Gallery Slideshow from Server |
Android and Image Gallery Slideshow from Server บทความการสร้าง Gallery Image และการดึงรุปภาพที่เป็น Thumbnail และ Full Photo มาแสดงบนหน้าจอของ Application หลักการก็คือใช้ PHP และ MySQL ที่ทำงานอยู่ใน Web Server เก็บ Gallery ลงใน Database ซึ่งหลัก ๆ จะเก็บภาพที่เป็น Thumbnail และ Full Photo โดยภาพที่เป็น Thumbnail จะแสดงใน ListView ส่วนภาพ Full Photo จะแสดงผลตอนที่ดูรายละเอียดของแต่ล่ะภาพ ดูภาดประกอบเพื่อความเข้าใจ
ภาพแสดงการดึงข้อมูล Gallery จาก Web Server มาแสดงบน Application ของ Android แสดงข้อมูลในรูปแบบของ Column โดยใช้ GridView ของ Widgets เข้ามาจัดการ
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Web Server (PHP and MySQL)
MySQL Database
CREATE TABLE IF NOT EXISTS `images` (
`ImageID` int(11) NOT NULL auto_increment,
`ImageName` varchar(50) NOT NULL,
`ImagePath_Thumbnail` varchar(150) NOT NULL,
`ImagePath_FullPhoto` varchar(150) NOT NULL,
PRIMARY KEY (`ImageID`)
) ENGINE=MyISAM AUTO_INCREMENT=7 ;
--
-- Dumping data for table `images`
--
INSERT INTO `images` (`ImageID`, `ImageName`, `ImagePath_Thumbnail`, `ImagePath_FullPhoto`) VALUES
(1, 'Image 1', 'https://www.thaicreate.com/android/img1_thum.jpg', 'https://www.thaicreate.com/android/img1_full.jpg'),
(2, 'Image 2', 'https://www.thaicreate.com/android/img2_thum.jpg', 'https://www.thaicreate.com/android/img2_full.jpg'),
(3, 'Image 3', 'https://www.thaicreate.com/android/img3_thum.jpg', 'https://www.thaicreate.com/android/img3_full.jpg'),
(4, 'Image 4', 'https://www.thaicreate.com/android/img4_thum.jpg', 'https://www.thaicreate.com/android/img4_full.jpg'),
(5, 'Image 5', 'https://www.thaicreate.com/android/img5_thum.jpg', 'https://www.thaicreate.com/android/img5_full.jpg'),
(6, 'Image 6', 'https://www.thaicreate.com/android/img6_thum.jpg', 'https://www.thaicreate.com/android/img6_full.jpg');
getByPage.php
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM images WHERE 1 ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
Android Project
โครงสร้างของไฟล์ประกอบด้วย 5 ไฟล์คือ MainActivity.java, PlayActivity.java, activity_main.xml, activity_column.xml และ activity_play.xml
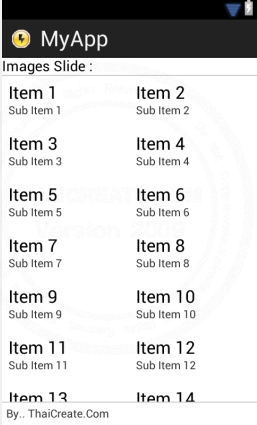
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Images Slide : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceMedium" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<GridView
android:id="@+id/gridView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:numColumns="2" >
</GridView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>

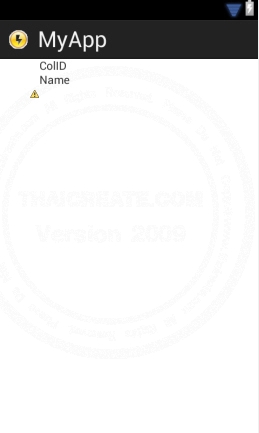
activity_column.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<ImageView
android:id="@+id/ColPhoto"
android:layout_width="50dp"
android:layout_height="50dp"
/>
<LinearLayout
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<LinearLayout
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView android:id="@+id/ColID"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="ColID"
/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView android:id="@+id/ColName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name"
/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
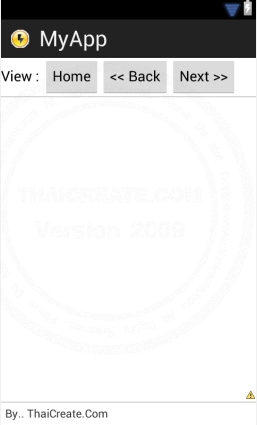
activity_play.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="View : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceMedium" />
<Button
android:id="@+id/btnHome"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Home" />
<Button
android:id="@+id/btnBack"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="<< Back" />
<Button
android:id="@+id/btnNext"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Next >>" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ImageView
android:id="@+id/fullimage"
android:layout_width="match_parent"
android:layout_height="380dp" />
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.ByteArrayOutputStream;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.content.Context;
import android.content.Intent;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.Menu;
import android.view.ViewGroup;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.BaseAdapter;
import android.widget.GridView;
import android.widget.ImageView;
import android.widget.TextView;
public class MainActivity extends Activity {
public static final int DIALOG_DOWNLOAD_JSON_PROGRESS = 0;
private ProgressDialog mProgressDialog;
public String resultServer = "";
ArrayList<HashMap<String, Object>> MyArrList;
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// Download JSON File
new DownloadJSONFileAsync().execute();
}
@Override
protected Dialog onCreateDialog(int id) {
switch (id) {
case DIALOG_DOWNLOAD_JSON_PROGRESS:
mProgressDialog = new ProgressDialog(this);
mProgressDialog.setMessage("Downloading.....");
mProgressDialog.setProgressStyle(ProgressDialog.STYLE_SPINNER);
mProgressDialog.setCancelable(true);
mProgressDialog.show();
return mProgressDialog;
default:
return null;
}
}
// Show All Content
public void ShowAllContent()
{
// gridView1
final GridView gridV = (GridView)findViewById(R.id.gridView1);
gridV.setAdapter(new ImageAdapter(MainActivity.this,MyArrList));
// OnClick
gridV.setOnItemClickListener(new OnItemClickListener() {
public void onItemClick(AdapterView<?> parent, View v,
int position, long id) {
String Position = String.valueOf(position);
Intent newActivity = new Intent(MainActivity.this,PlayActivity.class);
newActivity.putExtra("Position", Position);
newActivity.putExtra("resultServer", resultServer);
startActivity(newActivity);
}
});
}
public class ImageAdapter extends BaseAdapter
{
private Context context;
private ArrayList<HashMap<String, Object>> MyArr = new ArrayList<HashMap<String, Object>>();
public ImageAdapter(Context c, ArrayList<HashMap<String, Object>> myArrList)
{
// TODO Auto-generated method stub
context = c;
MyArr = myArrList;
}
public int getCount() {
// TODO Auto-generated method stub
return MyArr.size();
}
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
public View getView(int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// ColPhoto
ImageView imageView = (ImageView) convertView.findViewById(R.id.ColPhoto);
imageView.getLayoutParams().height = 80;
imageView.getLayoutParams().width = 80;
imageView.setPadding(10, 10, 10, 10);
imageView.setScaleType(ImageView.ScaleType.CENTER_CROP);
try
{
imageView.setImageBitmap((Bitmap)MyArr.get(position).get("ImageThumBitmap"));
} catch (Exception e) {
// When Error
imageView.setImageResource(android.R.drawable.ic_menu_report_image);
}
// ColID
TextView txtID = (TextView) convertView.findViewById(R.id.ColID);
txtID.setPadding(5, 0, 0, 0);
txtID.setText("ID : " + MyArr.get(position).get("ImageID").toString());
// ColName
TextView txtName = (TextView) convertView.findViewById(R.id.ColName);
txtName.setPadding(5, 0, 0, 0);
txtName.setText("Name : " + MyArr.get(position).get("ImageName").toString());
return convertView;
}
}
// Download JSON in Background
public class DownloadJSONFileAsync extends AsyncTask<String, Void, Void> {
protected void onPreExecute() {
super.onPreExecute();
showDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
}
@Override
protected Void doInBackground(String... params) {
// TODO Auto-generated method stub
/** JSON from URL
* [
* {"ImageID":"1","ImageName":"Image 1","ImagePath_Thumbnail":"https://www.thaicreate.com/android/img1_thum.jpg" ,"ImagePath_FullPhoto":"https://www.thaicreate.com/android/img1_full.jpg"},
* {"ImageID":"2","ImageName":"Image 2","ImagePath_Thumbnail":"https://www.thaicreate.com/android/img2_thum.jpg" ,"ImagePath_FullPhoto":"https://www.thaicreate.com/android/img2_full.jpg"},
* {"ImageID":"3","ImageName":"Image 3","ImagePath_Thumbnail":"https://www.thaicreate.com/android/img3_thum.jpg" ,"ImagePath_FullPhoto":"https://www.thaicreate.com/android/img3_full.jpg"},
* {"ImageID":"4","ImageName":"Image 4","ImagePath_Thumbnail":"https://www.thaicreate.com/android/img4_thum.jpg" ,"ImagePath_FullPhoto":"https://www.thaicreate.com/android/img4_full.jpg"},
* {"ImageID":"5","ImageName":"Image 5","ImagePath_Thumbnail":"https://www.thaicreate.com/android/img5_thum.jpg" ,"ImagePath_FullPhoto":"https://www.thaicreate.com/android/img5_full.jpg"},
* {"ImageID":"6","ImageName":"Image 6","ImagePath_Thumbnail":"https://www.thaicreate.com/android/img6_thum.jpg" ,"ImagePath_FullPhoto":"https://www.thaicreate.com/android/img6_full.jpg"}
* ]
*/
String url = "https://www.thaicreate.com/android/getGallery.php";
JSONArray data;
try {
resultServer = getJSONUrl(url);
data = new JSONArray(resultServer);
MyArrList = new ArrayList<HashMap<String, Object>>();
HashMap<String, Object> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, Object>();
map.put("ImageID", (String)c.getString("ImageID"));
map.put("ImageName", (String)c.getString("ImageName"));
// Thumbnail Get ImageBitmap To Bitmap
map.put("ImagePathThum", (String)c.getString("ImagePath_Thumbnail"));
map.put("ImageThumBitmap", (Bitmap)loadBitmap(c.getString("ImagePath_Thumbnail")));
// Full (for View Full)
map.put("ImagePathFull", (String)c.getString("ImagePath_FullPhoto"));
MyArrList.add(map);
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
protected void onPostExecute(Void unused) {
ShowAllContent(); // When Finish Show Content
dismissDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
removeDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
}
}
/*** Get JSON Code from URL ***/
public String getJSONUrl(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download file..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
/***** Get Image Resource from URL (Start) *****/
private static final String TAG = "Image";
private static final int IO_BUFFER_SIZE = 4 * 1024;
public static Bitmap loadBitmap(String url) {
Bitmap bitmap = null;
InputStream in = null;
BufferedOutputStream out = null;
try {
in = new BufferedInputStream(new URL(url).openStream(), IO_BUFFER_SIZE);
final ByteArrayOutputStream dataStream = new ByteArrayOutputStream();
out = new BufferedOutputStream(dataStream, IO_BUFFER_SIZE);
copy(in, out);
out.flush();
final byte[] data = dataStream.toByteArray();
BitmapFactory.Options options = new BitmapFactory.Options();
//options.inSampleSize = 1;
bitmap = BitmapFactory.decodeByteArray(data, 0, data.length,options);
} catch (IOException e) {
Log.e(TAG, "Could not load Bitmap from: " + url);
} finally {
closeStream(in);
closeStream(out);
}
return bitmap;
}
private static void closeStream(Closeable stream) {
if (stream != null) {
try {
stream.close();
} catch (IOException e) {
android.util.Log.e(TAG, "Could not close stream", e);
}
}
}
private static void copy(InputStream in, OutputStream out) throws IOException {
byte[] b = new byte[IO_BUFFER_SIZE];
int read;
while ((read = in.read(b)) != -1) {
out.write(b, 0, read);
}
}
/***** Get Image Resource from URL (End) *****/
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}

PlayActivity.java
package com.myapp;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.ByteArrayOutputStream;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.content.Intent;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.Toast;
public class PlayActivity extends Activity {
public static final int DIALOG_DOWNLOAD_JSON_PROGRESS = 0;
private ProgressDialog mProgressDialog;
public int curPosition = 0;
public String resultServer;
public ArrayList<HashMap<String, Object>> MyArrList;
public Button back;
public Button next;
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_play);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
Intent intent= getIntent();
curPosition = Integer.parseInt(intent.getStringExtra("Position"));
resultServer = String.valueOf(intent.getStringExtra("resultServer"));
try {
MyArrList = ConvertJSONtoArrayList(resultServer);
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
Log.d("ArrayList Size",String.valueOf(MyArrList.size()));
// Show Image Full
new DownloadFullPhotoFileAsync().execute();
// Button Home
final Button home = (Button) findViewById(R.id.btnHome);
// Perform action on click
home.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent newActivity = new Intent(PlayActivity.this,MainActivity.class);
startActivity(newActivity);
}
});
// Button Back
back = (Button) findViewById(R.id.btnBack);
back.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
curPosition = curPosition - 1;
new DownloadFullPhotoFileAsync().execute();
}
});
// Button Next
next = (Button) findViewById(R.id.btnNext);
next.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
curPosition = curPosition + 1;
new DownloadFullPhotoFileAsync().execute();
}
});
}
// Show Image Full
public void ShowImageFull(String imageName, Bitmap imgFull)
{
// Prepare Button (Back)
if(curPosition <= 0)
{
back.setEnabled(false);
}
else
{
back.setEnabled(true);
}
// Prepare Button (Next)
Log.d("curPosition",String.valueOf(curPosition));
Log.d("MyArrList.size",String.valueOf(MyArrList.size()));
if(curPosition >= MyArrList.size() - 1)
{
next.setEnabled(false);
}
else
{
next.setEnabled(true);
}
ImageView image = (ImageView) findViewById(R.id.fullimage);
try
{
image.setImageBitmap(imgFull);
} catch (Exception e) {
// When Error
image.setImageResource(android.R.drawable.ic_menu_report_image);
}
// Show Toast
Toast.makeText(PlayActivity.this,imageName,Toast.LENGTH_LONG).show();
}
public ArrayList<HashMap<String, Object>> ConvertJSONtoArrayList(String json) throws JSONException
{
JSONArray data = new JSONArray(resultServer);
ArrayList<HashMap<String, Object>> arr = new ArrayList<HashMap<String, Object>>();
HashMap<String, Object> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, Object>();
map.put("ImageID", (String)c.getString("ImageID"));
map.put("ImageName", (String)c.getString("ImageName"));
map.put("ImagePathThum", (String)c.getString("ImagePath_Thumbnail"));
map.put("ImagePathFull", (String)c.getString("ImagePath_FullPhoto"));
arr.add(map);
}
return arr;
}
@Override
protected Dialog onCreateDialog(int id) {
switch (id) {
case DIALOG_DOWNLOAD_FULL_PHOTO_PROGRESS:
mProgressDialog = new ProgressDialog(this);
mProgressDialog.setMessage("Downloading.....");
mProgressDialog.setProgressStyle(ProgressDialog.STYLE_SPINNER);
mProgressDialog.setCancelable(true);
mProgressDialog.show();
return mProgressDialog;
default:
return null;
}
}
public static final int DIALOG_DOWNLOAD_FULL_PHOTO_PROGRESS = 1;
// Download Full Photo in Background
public class DownloadFullPhotoFileAsync extends AsyncTask<String, Void, Void> {
String strImageName = "";
String ImageFullPhoto = "";
Bitmap ImageFullBitmap = null;
protected void onPreExecute() {
super.onPreExecute();
showDialog(DIALOG_DOWNLOAD_FULL_PHOTO_PROGRESS);
}
@Override
protected Void doInBackground(String... params) {
strImageName = MyArrList.get(curPosition).get("ImageName").toString();
ImageFullPhoto = MyArrList.get(curPosition).get("ImagePathFull").toString();
ImageFullBitmap = (Bitmap)loadBitmap(ImageFullPhoto);
return null;
}
protected void onPostExecute(Void unused) {
ShowImageFull(strImageName,ImageFullBitmap); // When Finish Show Images
dismissDialog(DIALOG_DOWNLOAD_FULL_PHOTO_PROGRESS);
removeDialog(DIALOG_DOWNLOAD_FULL_PHOTO_PROGRESS);
}
}
/***** Get Image Resource from URL (Start) *****/
private static final String TAG = "Image";
private static final int IO_BUFFER_SIZE = 4 * 1024;
public static Bitmap loadBitmap(String url) {
Bitmap bitmap = null;
InputStream in = null;
BufferedOutputStream out = null;
try {
in = new BufferedInputStream(new URL(url).openStream(), IO_BUFFER_SIZE);
final ByteArrayOutputStream dataStream = new ByteArrayOutputStream();
out = new BufferedOutputStream(dataStream, IO_BUFFER_SIZE);
copy(in, out);
out.flush();
final byte[] data = dataStream.toByteArray();
BitmapFactory.Options options = new BitmapFactory.Options();
//options.inSampleSize = 1;
bitmap = BitmapFactory.decodeByteArray(data, 0, data.length,options);
} catch (IOException e) {
Log.e(TAG, "Could not load Bitmap from: " + url);
} finally {
closeStream(in);
closeStream(out);
}
return bitmap;
}
private static void closeStream(Closeable stream) {
if (stream != null) {
try {
stream.close();
} catch (IOException e) {
android.util.Log.e(TAG, "Could not close stream", e);
}
}
}
private static void copy(InputStream in, OutputStream out) throws IOException {
byte[] b = new byte[IO_BUFFER_SIZE];
int read;
while ((read = in.read(b)) != -1) {
out.write(b, 0, read);
}
}
/***** Get Image Resource from URL (End) *****/
}
เพิ่ม PlayActivity ลงในไฟล์ AndroidManifest.xml
AndroidManifest.xml
<activity
android:name="PlayActivity"
android:theme="@style/AppTheme"
android:screenOrientation="portrait"
android:label="@string/title_activity_main" />
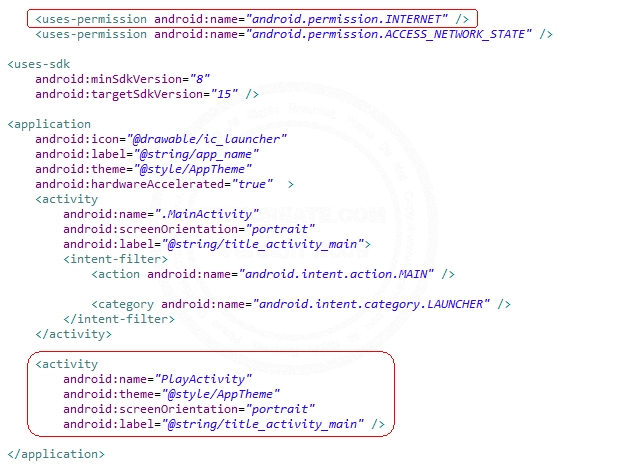
Screenshot
ทดสอบการรันผ่าน Emulator
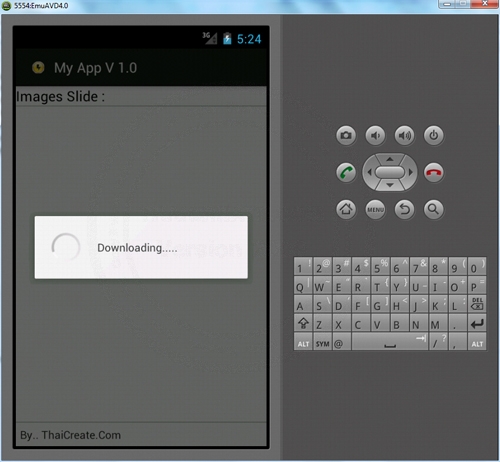
กำลังแสดง ProgressBar เพื่อโหลดข้อมูลจาก Web Server
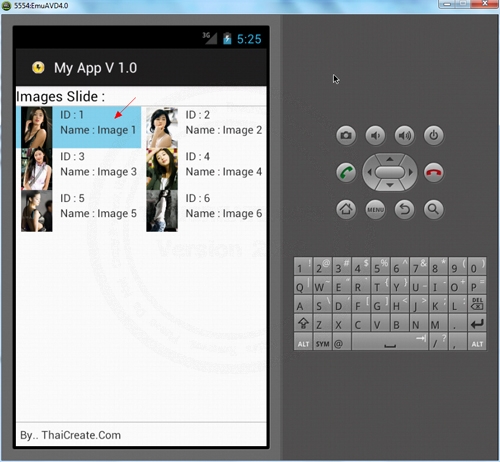
แสดง Gallery บน GridView ทดสอบคลิกรูปที่ 1
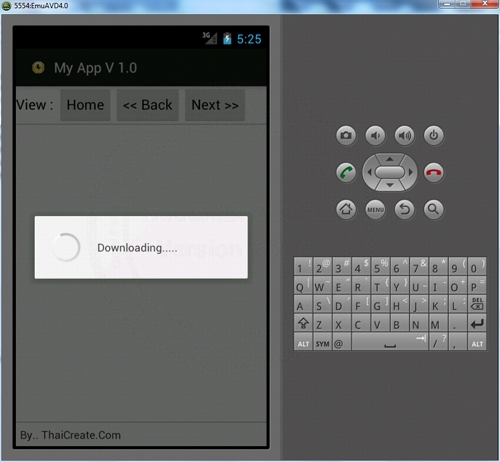
เมื่อคลิกเพื่อดู Full Image ในขณะที่กำลังโหลดข้อมูลก็จะแสดง PregressBar เช่นเดียวกัน
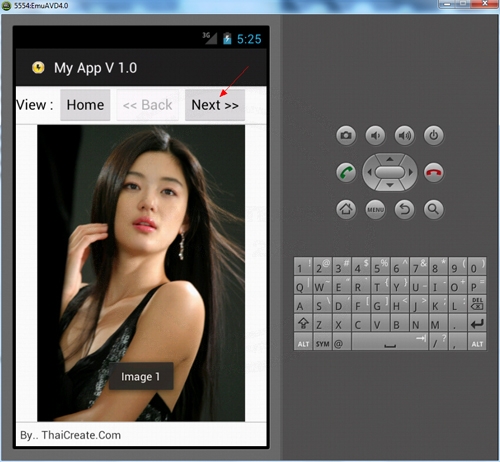
แสดงรูปภาพ Full Photo เมื่อโหลดข้อมูลเรียบร้อย และสามารถกด Next >> เพื่อดูรูปอื่น ๆ
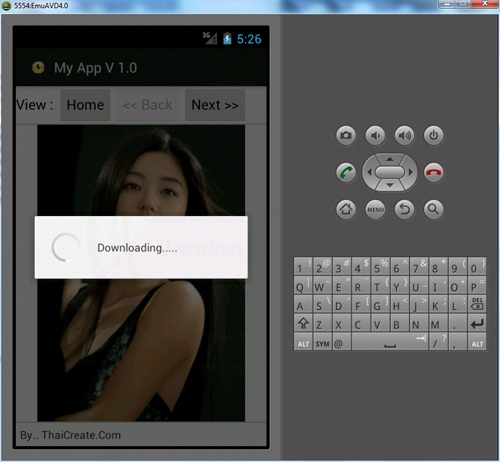
ในทุก ๆ ครั้งที่โหลดข้อมูลจะแสดง ProgressBar ด้วยทุกครั้ง เพื่อป้องกันโปรแกรมค้าง
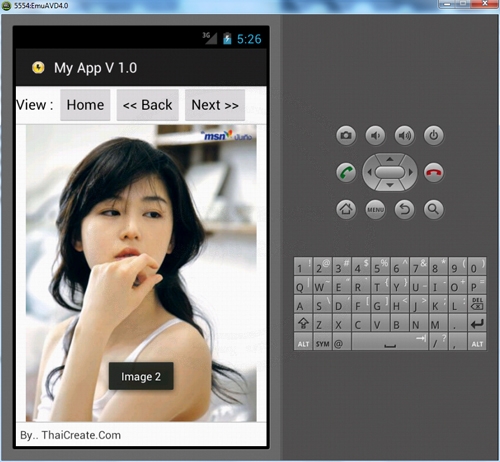
แสดงข้อมูลเมื่อโหลดเรียบร้อย
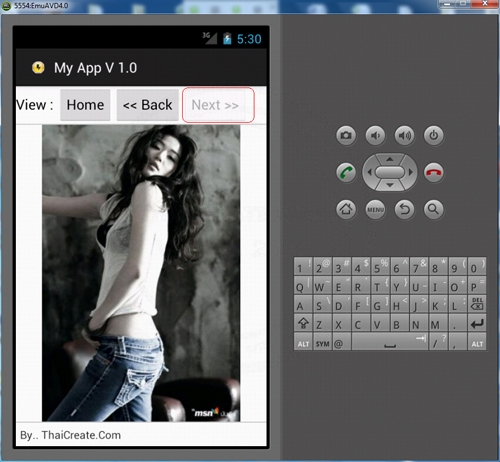
กรณีหน้าสุดท้ายแล้วจะไม่สามารถคลิกเลือก Next >> ได้
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-08-26 11:40:04 /
2017-03-26 22:39:24 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|