ImageSwitcher - Android Widgets Example |
ImageSwitcher - Android Widgets สำหรับ ImageSwitcher เป็น Widget ใช้สำหรับแสดงรูปภาพ (ImageView) หรือควบคุมการปรับเปลี่ยนรูปภาพในรูปแบบต่าง ๆ โดยใน ImageSwitcher สามารถใส่ Effect ให้กับรูปภาพได้ เช่นในกรณีที่เปลี่ยนรูปภาพสามารถทำเป็น Fade In หรือ Fade Out ได้ ซึ่ง ImageSwitcher สามารถทำงานร่วมกับ Widgets Gallery เพื่อแสดงรุปภาพก่อนแล้วค่อยนำภาพที่ได้จากการเลือกมาแสดงใน ImageSwitcher

XML Syntax
<ImageSwitcher
android:id="@+id/imageSwitcher1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
Example 1 การใช้ ImageSwitcher ควบคุมการเปลี่ยนแปลงรูปภาพ ImageView แบบง่าย ๆ
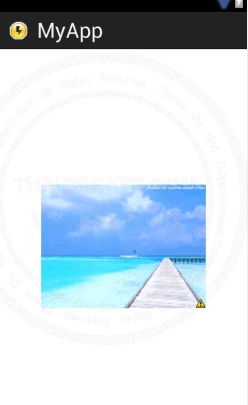
ออกแบบหน้าจอ GraphicLayout ด้วย Widget ตามรูป
activity_main.xml (XML Layout)
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<LinearLayout
android:id="@+id/LinearLayout01"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginTop="75dp" >
<ImageSwitcher
android:id="@+id/imageSwitcher1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="100dp" >
<ImageView
android:id="@+id/imageView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/pic_a" />
<ImageView
android:id="@+id/imageView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/pic_b" />
</ImageSwitcher>
</LinearLayout>
</RelativeLayout>
XML Layout กับ ImageSwitcher
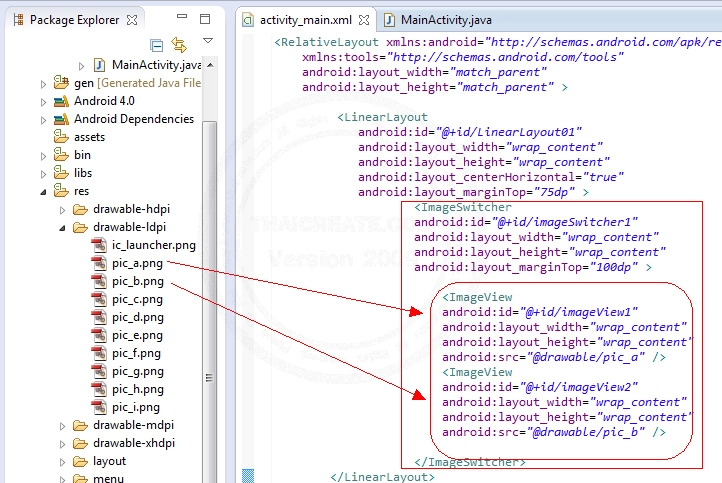
จาก XML Layout จะเห็นว่ามีการใช้ ImageSwitcher เพื่อควบคุม ImageView อยู่ 2 ตัว (กรณีแสดงผลจาก XML Layout จะสามารถกำหนดได้แค่ 2 ตัว)
MainActivity.java (Java Code)
package com.myapp;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.ImageSwitcher;
public class MainActivity extends Activity {
ImageSwitcher ImS;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ImS = (ImageSwitcher) findViewById(R.id.imageSwitcher1);
ImS.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
ImS.showNext();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
จาก Code ของ Java จะเห็นว่า ImageSwitcher มี Property ชื่อว่า showNext(); เพื่อแสดงรูปถัดไป ซึ่งจะสลับไปสลับมา
กรณีที่ต้องการใส่ Effect สามารถเพิ่มได้ตรง
Animation inAnim = new AlphaAnimation(0, 1);
inAnim.setDuration(2000);
Animation outAnim = new AlphaAnimation(1, 0);
outAnim.setDuration(2000);
ImS.setInAnimation(inAnim);
ImS.setOutAnimation(outAnim);
MainActivity.java (Java Code)
package com.myapp;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.view.animation.AlphaAnimation;
import android.view.animation.Animation;
import android.widget.ImageSwitcher;
public class MainActivity extends Activity {
ImageSwitcher ImS;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ImS = (ImageSwitcher) findViewById(R.id.imageSwitcher1);
Animation inAnim = new AlphaAnimation(0, 1);
inAnim.setDuration(2000);
Animation outAnim = new AlphaAnimation(1, 0);
outAnim.setDuration(2000);
ImS.setInAnimation(inAnim);
ImS.setOutAnimation(outAnim);
ImS.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
ImS.showNext();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
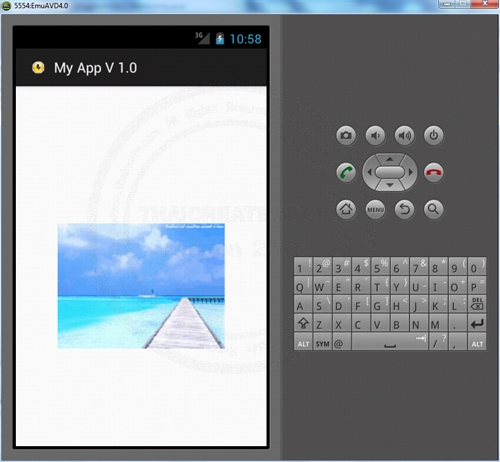
แสดงภาพแรก
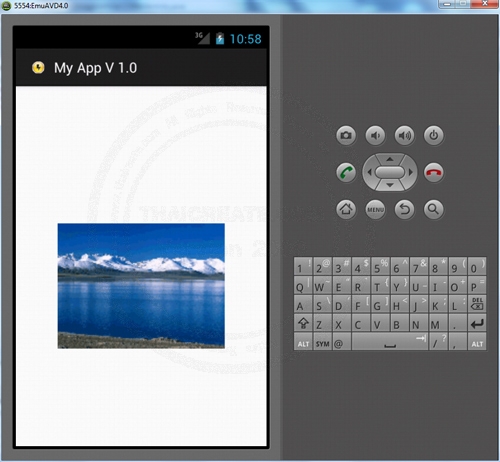
เมื่อคลิกก็จะแสดงอีกภาพ
Example 2 การใช้ ImageSwitcher และรูปภาพแบบ ImageView แบบ Dynamic พร้อม ๆ กับมีปุ่ม Button กำหนดทิศทาง Next หรือ Previous

ออกแบบหน้าจอ GraphicLayout ด้วย Widget ตามรูป
activity_main.xml (XML Layout)
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<LinearLayout
android:id="@+id/LinearLayout01"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginTop="75dp" >
<Button
android:id="@+id/Button02"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="<<" />
<Button
android:id="@+id/Button01"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text=">>" />
</LinearLayout>
<LinearLayout
android:id="@+id/LinearLayout02"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginTop="75dp" >
<ImageSwitcher
android:id="@+id/imageSwitcher1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="100dp" />
</LinearLayout>
</RelativeLayout>
การสร้างเรียกรูปภาพแบบ Dynamic โดยอ้างอิงชื่อรูปภาพจากโฟเดอร์ดังรูป
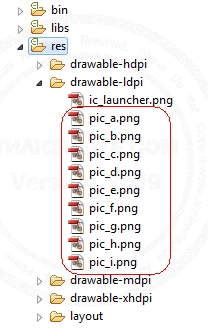
โดยใช้การเก็บข้อมูลในรูปแบบของ Array
private Integer[] mImageIds = {
R.drawable.pic_a,
R.drawable.pic_b,
R.drawable.pic_c,
R.drawable.pic_d,
R.drawable.pic_e,
R.drawable.pic_f,
R.drawable.pic_g,
R.drawable.pic_h,
R.drawable.pic_i
};
Code ทั้งหมด
MainActivity.java (Java Code)
package com.myapp;
import android.os.Bundle;
import android.app.ActionBar.LayoutParams;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.view.animation.AnimationUtils;
import android.widget.Button;
import android.widget.ImageSwitcher;
import android.widget.ImageView;
import android.widget.Toast;
import android.widget.ViewSwitcher.ViewFactory;
public class MainActivity extends Activity implements ViewFactory {
private Integer[] mImageIds = {
R.drawable.pic_a,
R.drawable.pic_b,
R.drawable.pic_c,
R.drawable.pic_d,
R.drawable.pic_e,
R.drawable.pic_f,
R.drawable.pic_g,
R.drawable.pic_h,
R.drawable.pic_i
};
ImageSwitcher iSwitcher;
Button btnNext;
Button btnPrev;
int position = 0;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// imageSwitcher1
iSwitcher = (ImageSwitcher) findViewById(R.id.imageSwitcher1);
iSwitcher.setFactory(this);
iSwitcher.setInAnimation(AnimationUtils.loadAnimation(this,
android.R.anim.fade_in));
iSwitcher.setOutAnimation(AnimationUtils.loadAnimation(this,
android.R.anim.fade_out));
iSwitcher.setImageResource(mImageIds[0]);
btnNext = (Button) findViewById(R.id.Button01);
btnNext.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
setPositionNext();
iSwitcher.setImageResource(mImageIds[position]);
Toast.makeText(MainActivity.this, "Your selected position = " + getResources().getResourceName(mImageIds[position]), Toast.LENGTH_SHORT).show();
}
});
btnPrev = (Button) findViewById(R.id.Button02);
btnPrev.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
setPositionPrev();
iSwitcher.setImageResource(mImageIds[position]);
Toast.makeText(MainActivity.this, "Your selected position = " + getResources().getResourceName(mImageIds[position]), Toast.LENGTH_SHORT).show();
}
});
}
public void setPositionNext()
{
position++;
if(position > mImageIds.length -1)
{
position = 0;
}
}
public void setPositionPrev()
{
position--;
if(position < 0)
{
position = mImageIds.length - 1;
}
}
public View makeView() {
// TODO Auto-generated method stub
ImageView iView = new ImageView(this);
iView.setScaleType(ImageView.ScaleType.FIT_CENTER);
iView.setLayoutParams(new
ImageSwitcher.LayoutParams(
LayoutParams.FILL_PARENT,LayoutParams.FILL_PARENT));
iView.setBackgroundColor(0xFF000000);
return iView;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
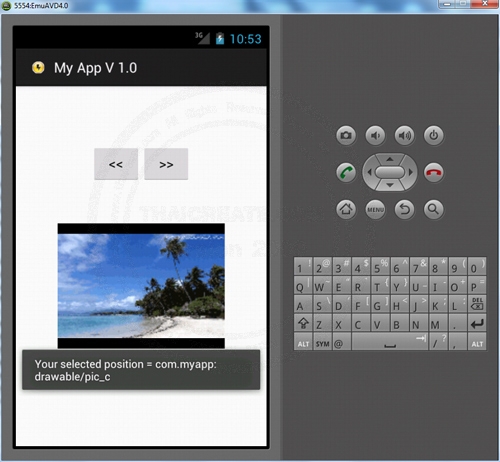
สดงรูปภาพ ซึ่งสามารถกด ถัดไป (Next) หรือ ย้อนกลับได้ (Previous)
Example 3 การใช้ ImageSwitcher และ GestureDetector เพื่อควบคุมการ Slide รุปภาพในทิศทาง ซ้าย-ขวา
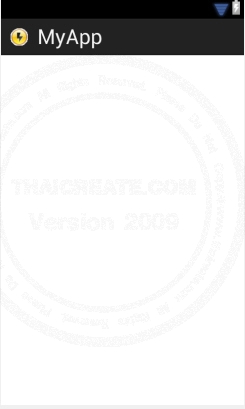
ออกแบบหน้าจอ GraphicLayout ด้วย Widget ตามรูป
activity_main.xml (XML Layout)
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<LinearLayout
android:id="@+id/LinearLayout02"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginTop="50dp" >
<ImageSwitcher
android:id="@+id/imageSwitcher1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="50dp" />
</LinearLayout>
</RelativeLayout>
MainActivity.java (Java Code)
package com.myapp;
import android.app.ActionBar.LayoutParams;
import android.app.Activity;
import android.os.Bundle;
import android.view.GestureDetector;
import android.view.GestureDetector.OnGestureListener;
import android.view.MotionEvent;
import android.view.View;
import android.view.animation.AlphaAnimation;
import android.view.animation.Animation;
import android.widget.ImageSwitcher;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.ViewSwitcher.ViewFactory;
import android.widget.Toast;
public class MainActivity extends Activity implements ViewFactory, OnGestureListener {
GestureDetector gd;
TextView tv1;
private Integer[] mImageIds = {
R.drawable.pic_a,
R.drawable.pic_b,
R.drawable.pic_c,
R.drawable.pic_d,
R.drawable.pic_e,
R.drawable.pic_f,
R.drawable.pic_g,
R.drawable.pic_h,
R.drawable.pic_i
};
private static final int SWIPE_MIN_DISTANCE = 120;
private static final int SWIPE_MAX_OFF_PATH = 250;
private static final int SWIPE_THRESHOLD_VELOCITY = 100;
int position = 0;
int i = 0;
ImageSwitcher iSwitcher;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Animation inAnim = new AlphaAnimation(0, 1);
inAnim.setDuration(1000);
Animation outAnim = new AlphaAnimation(1, 0);
outAnim.setDuration(1000);
// imageSwitcher1
iSwitcher = (ImageSwitcher) findViewById(R.id.imageSwitcher1);
iSwitcher.setFactory(this);
iSwitcher.setInAnimation(inAnim);
iSwitcher.setOutAnimation(outAnim);
iSwitcher.setImageResource(mImageIds[0]);
gd = new GestureDetector(this, this);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
if (gd.onTouchEvent(event))
return true;
else
return false;
}
public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX,
float velocityY) {
try {
if (Math.abs(e1.getY() - e2.getY()) > SWIPE_MAX_OFF_PATH)
return false;
// right to left swipe
if (e1.getX() - e2.getX() > SWIPE_MIN_DISTANCE
&& Math.abs(velocityX) > SWIPE_THRESHOLD_VELOCITY) {
setPositionNext();
iSwitcher.setImageResource(mImageIds[position]);
Toast.makeText(MainActivity.this, getResources().getResourceName(mImageIds[position]), Toast.LENGTH_SHORT).show();
} else if (e2.getX() - e1.getX() > SWIPE_MIN_DISTANCE
&& Math.abs(velocityX) > SWIPE_THRESHOLD_VELOCITY) {
//left to right flip
setPositionPrev();
iSwitcher.setImageResource(mImageIds[position]);
Toast.makeText(MainActivity.this, getResources().getResourceName(mImageIds[position]), Toast.LENGTH_SHORT).show();
}
} catch (Exception e) {
// nothing
return true;
}
return true;
}
public void setPositionNext()
{
position++;
if(position > mImageIds.length -1)
{
position = 0;
}
}
public void setPositionPrev()
{
position--;
if(position < 0)
{
position = mImageIds.length - 1;
}
}
public View makeView() {
// TODO Auto-generated method stub
ImageView iView = new ImageView(this);
iView.setScaleType(ImageView.ScaleType.FIT_CENTER);
iView.setLayoutParams(new
ImageSwitcher.LayoutParams(
LayoutParams.FILL_PARENT,LayoutParams.FILL_PARENT));
iView.setBackgroundColor(0xFF000000);
return iView;
}
public boolean onDown(MotionEvent arg0) {
// TODO Auto-generated method stub
return false;
}
public void onLongPress(MotionEvent arg0) {
// TODO Auto-generated method stub
}
public boolean onScroll(MotionEvent arg0, MotionEvent arg1, float arg2,
float arg3) {
// TODO Auto-generated method stub
return false;
}
public void onShowPress(MotionEvent arg0) {
// TODO Auto-generated method stub
}
public boolean onSingleTapUp(MotionEvent arg0) {
// TODO Auto-generated method stub
return false;
}
}
Screenshot
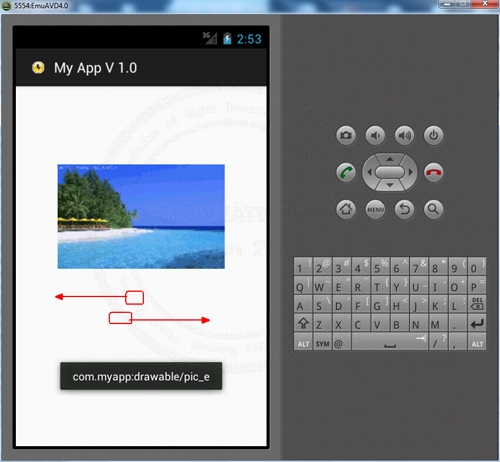
สามารถ Slide ซ้่าย-ขวา เพื่อเปลี่ยนหรือเลื่อนดูรูปภาพ
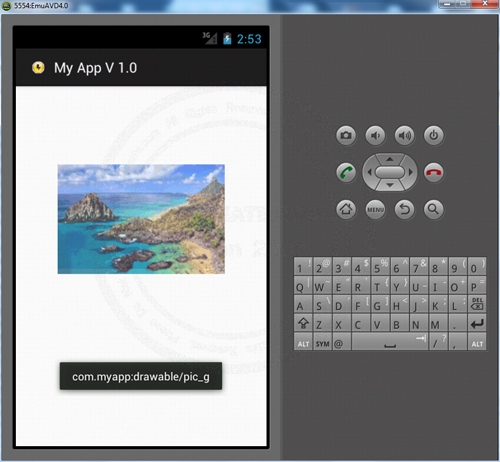
แสดงรูปภาพจากผลลัพธ์ที่ได้
Example 4 การใช้ ImageSwitcher กับ Widgets Gallery โดยจะแสดงรูปภาพในกรอบเล็ก ๆ และสามารถเลือกรูปภาพเพื่อดูขนาดใหญ่ได้
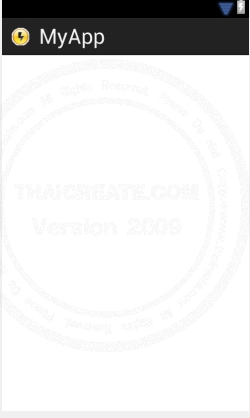
ออกแบบหน้าจอ GraphicLayout ด้วย Widget ตามรูป
activity_main.xml (XML Layout)
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<Gallery
android:id="@+id/gallery1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<ImageSwitcher
android:id="@+id/imageSwitcher1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="100dp" >
</ImageSwitcher>
</TableLayout>
MainActivity.java (Java Code)
package com.myapp;
import android.os.Bundle;
import android.app.ActionBar.LayoutParams;
import android.app.Activity;
import android.content.Context;
import android.view.Menu;
import android.view.View;
import android.view.ViewGroup;
import android.view.animation.AnimationUtils;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.BaseAdapter;
import android.widget.Gallery;
import android.widget.ImageSwitcher;
import android.widget.ImageView;
import android.widget.Toast;
import android.widget.ViewSwitcher.ViewFactory;
public class MainActivity extends Activity implements ViewFactory {
private Integer[] mImageIds = {
R.drawable.pic_a,
R.drawable.pic_b,
R.drawable.pic_c,
R.drawable.pic_d,
R.drawable.pic_e,
R.drawable.pic_f,
R.drawable.pic_g,
R.drawable.pic_h,
R.drawable.pic_i
};
ImageSwitcher iSwitcher;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// gallery1
Gallery galry = (Gallery) findViewById(R.id.gallery1);
galry.setSpacing(1);
galry.setAdapter(new ImageAdapter(this));
// imageSwitcher1
iSwitcher = (ImageSwitcher) findViewById(R.id.imageSwitcher1);
iSwitcher.setFactory(this);
iSwitcher.setInAnimation(AnimationUtils.loadAnimation(this,
android.R.anim.fade_in));
iSwitcher.setOutAnimation(AnimationUtils.loadAnimation(this,
android.R.anim.fade_out));
galry.setOnItemClickListener(new OnItemClickListener() {
public void onItemClick(AdapterView<?> parent, View v, int position, long id) {
iSwitcher.setImageResource(mImageIds[position]);
Toast.makeText(MainActivity.this, "Your selected position = " + getResources().getResourceName(mImageIds[position]), Toast.LENGTH_SHORT).show();
}
});
}
public class ImageAdapter extends BaseAdapter {
private Context mContext;
public ImageAdapter(Context c) {
mContext = c;
}
public int getCount() {
return mImageIds.length;
}
public Object getItem(int position) {
return position;
}
public long getItemId(int position) {
return position;
}
public View getView(int arg0, View arg1, ViewGroup arg2) {
// TODO Auto-generated method stub
ImageView i = new ImageView(mContext);
i.setImageResource(mImageIds[arg0]);
i.setLayoutParams(new Gallery.LayoutParams(100, 100));
//i.setScaleType(ImageView.ScaleType.CENTER);
//i.setScaleType(ImageView.ScaleType.CENTER_CROP);
//i.setScaleType(ImageView.ScaleType.CENTER_INSIDE);
//i.setScaleType(ImageView.ScaleType.FIT_CENTER);
//i.setScaleType(ImageView.ScaleType.FIT_XY);
//i.setScaleType(ImageView.ScaleType.FIT_END);
i.setScaleType(ImageView.ScaleType.FIT_XY);
return i;
}
}
public View makeView() {
// TODO Auto-generated method stub
ImageView iView = new ImageView(this);
iView.setScaleType(ImageView.ScaleType.FIT_CENTER);
iView.setLayoutParams(new
ImageSwitcher.LayoutParams(
LayoutParams.FILL_PARENT,LayoutParams.FILL_PARENT));
iView.setBackgroundColor(0xFF000000);
return iView;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
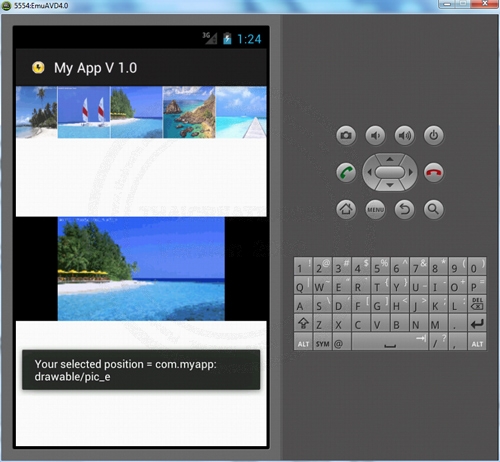
แสดงรูปภาพ Gallery และ ImageSwitcher

Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-07-01 16:22:31 /
2012-07-15 11:19:06 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|