Android Insert Data to Server via Web Service |
Android Insert Data to Server via Web Service ตัวอย่างการเขียน Android เพื่อเพิ่มข้อมูล หรือ Insert ข้อมูลที่อยู่ใน Server ผ่านช่องทางของ Web Service หลักการง่าย ๆ ก็คือออกแบบ Form บน Android รอรับการ Input ข้อมูล และหลังจากที่ Input ข้อมูลผ่าน Form ก็จะนำข้อมูลเหล่านั้นส่งไปยัง Server ด้วย Web Service และบน Web Service จะมี Application ที่ถูกเขียนด้วย PHP กับ MySQL ทำหน้าที่นำข้อมูลเหล่านั้นบันทึกลงใน Database และหลังจากที่บันทึกเรียบร้อยแล้วก็จะส่งสถานะกลับมายัง Android Client ว่า Insert ผ่านหรือสมบูรณ์หรือไม่
Android and Web Service
รูปอธิบายการทำงานระหว่าง Android กับ Web Service และการ Insert ข้อมูลบน Web Service ด้วย PHP กับ MySQL
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Web Service
member
CREATE TABLE `member` (
`MemberID` int(2) NOT NULL auto_increment,
`Username` varchar(50) NOT NULL,
`Password` varchar(50) NOT NULL,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
`Email` varchar(150) NOT NULL,
PRIMARY KEY (`MemberID`),
UNIQUE KEY `Username` (`Username`),
UNIQUE KEY `Email` (`Email`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'weerachai', 'weerachai@1', 'Weerachai Nukitram', '0819876107', '[email protected]');
INSERT INTO `member` VALUES (2, 'adisorn', 'adisorn@2', 'Adisorn Bunsong', '021978032', '[email protected]');
INSERT INTO `member` VALUES (3, 'surachai', 'surachai@3', 'Surachai Sirisart', '0876543210', '[email protected]');
โครงสร้างของ MySQL บน Web Service

insertMemberData.php ไฟล์ Web Service ที่จะทำหน้าที่ในการ Insert ข้อมูล
<?
ob_start();
require_once("lib/nusoap.php");
//Create a new soap server
$server = new soap_server();
//Define our namespace
$namespace = "https://www.thaicreate.com/android/insertMemberData.php";
$server->wsdl->schemaTargetNamespace = $namespace;
//Configure our WSDL
$server->configureWSDL("insertMember");
// Register our method and argument parameters
$varname = array(
'strUsername' => "xsd:string",
'strPassword' => "xsd:string",
'strName' => "xsd:string",
'strEmail' => "xsd:string",
'strTel' => "xsd:string"
);
$server->register('insertMember',$varname, array('return' => 'xsd:string'));
function insertMember($strUsername,$strPassword,$strName,$strEmail,$strTel)
{
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
/*** Check Username Exists ***/
$strSQL = "SELECT * FROM member WHERE Username = '".$strUsername."' ";
$objQuery = mysql_query($strSQL);
$objResult = mysql_fetch_array($objQuery);
if($objResult)
{
$arr['StatusID'] = "0";
$arr['Error'] = "Username Exists!";
header('Content-type: application/json');
mysql_close($objConnect);
return json_encode($arr);
}
/*** Check Email Exists ***/
$strSQL = "SELECT * FROM member WHERE Email = '".$strEmail."' ";
$objQuery = mysql_query($strSQL);
$objResult = mysql_fetch_array($objQuery);
if($objResult)
{
$arr['StatusID'] = "0";
$arr['Error'] = "Email Exists!";
header('Content-type: application/json');
mysql_close($objConnect);
return json_encode($arr);
}
/*** Insert ***/
$strSQL = "INSERT INTO member (Username,Password,Name,Email,Tel)
VALUES (
'".$strUsername."',
'".$strPassword."',
'".$strName."',
'".$strEmail."',
'".$strTel."'
)
";
$objQuery = mysql_query($strSQL);
if(!$objQuery)
{
$arr['StatusID'] = "0";
$arr['Error'] = "Cannot save data!";
}
else
{
$arr['StatusID'] = "1";
$arr['Error'] = "";
}
/**
$arr['StatusID'] // (0=Failed , 1=Complete)
$arr['Error'] // Error Message
*/
mysql_close($objConnect);
header('Content-type: application/json');
return json_encode($arr);
}
// Get our posted data if the service is being consumed
// otherwise leave this data blank.
$POST_DATA = isset($GLOBALS['HTTP_RAW_POST_DATA']) ? $GLOBALS['HTTP_RAW_POST_DATA'] : '';
// pass our posted data (or nothing) to the soap service
$server->service($POST_DATA);
exit();
?>
ในไฟล์ของ PHP ที่ทำหน้าที่เป็น Web Service จะมี Method ชื่อว่า insertMember ซึ่งรับค่าตัวแปร $strUsername, $strPassword, $strName, $strEmail, $strTel โดยค่าเหล่าที่จะถูกส่งมาจาก Android เพื่อนำไป Insert ลงในฐานข้อมูล MySQL แต่ก่อนการ Insert จะมีการตรวจสอบความถูกต้องของข้อมูล เช่น Username ซ้ำ หรือ Email ซ้ำ โดยสถานะต่าง ๆ ที่ส่งกลับไปยัง Android จะใช้ JSON เข้ามาช่วย
Android Project
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml
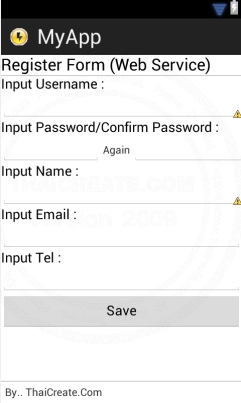
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Register Form (Web Service)"
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Username :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" >
</EditText>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Password/Confirm Password :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<TableRow>
<EditText
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="6"
android:inputType="textPassword" >
</EditText>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text=" Again " />
<EditText
android:id="@+id/txtConPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="7"
android:inputType="textPassword" >
</EditText>
</TableRow>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Name :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" >
</EditText>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Email :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:inputType="textEmailAddress"
android:ems="10" >
</EditText>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Tel :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtTel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:inputType="phone"
android:ems="10" >
</EditText>
<Button
android:id="@+id/btnSave"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Save" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
MainActivity.java
package com.myapp;
import java.io.IOException;
import org.json.JSONException;
import org.json.JSONObject;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
import org.xmlpull.v1.XmlPullParserException;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.view.View;
import android.view.Menu;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
private final String NAMESPACE = "https://www.thaicreate.com/android/insertMemberData.php";
private final String URL = "https://www.thaicreate.com/android/insertMemberData.php?wsdl"; // WSDL URL
private final String SOAP_ACTION = "https://www.thaicreate.com/android/insertMemberData.php/insertMember";
private final String METHOD_NAME = "insertMember"; // Method on web service
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// btnSave
final Button btnSave = (Button) findViewById(R.id.btnSave);
// Perform action on click
btnSave.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
if(SaveData())
{
// When Save Complete
}
}
});
}
public boolean SaveData()
{
// txtUsername,txtPassword,txtName,txtEmail,txtTel
final EditText txtUsername = (EditText)findViewById(R.id.txtUsername);
final EditText txtPassword = (EditText)findViewById(R.id.txtPassword);
final EditText txtConPassword = (EditText)findViewById(R.id.txtConPassword);
final EditText txtName = (EditText)findViewById(R.id.txtName);
final EditText txtEmail = (EditText)findViewById(R.id.txtEmail);
final EditText txtTel = (EditText)findViewById(R.id.txtTel);
// Dialog
final AlertDialog.Builder ad = new AlertDialog.Builder(this);
ad.setTitle("Error! ");
ad.setIcon(android.R.drawable.btn_star_big_on);
ad.setPositiveButton("Close", null);
// Check Username
if(txtUsername.getText().length() == 0)
{
ad.setMessage("Please input [Username] ");
ad.show();
txtUsername.requestFocus();
return false;
}
// Check Password
if(txtPassword.getText().length() == 0 || txtConPassword.getText().length() == 0 )
{
ad.setMessage("Please input [Password/Confirm Password] ");
ad.show();
txtPassword.requestFocus();
return false;
}
// Check Password and Confirm Password (Match)
if(!txtPassword.getText().toString().equals(txtConPassword.getText().toString()))
{
ad.setMessage("Password and Confirm Password Not Match! ");
ad.show();
txtConPassword.requestFocus();
return false;
}
// Check Name
if(txtName.getText().length() == 0)
{
ad.setMessage("Please input [Name] ");
ad.show();
txtName.requestFocus();
return false;
}
// Check Email
if(txtEmail.getText().length() == 0)
{
ad.setMessage("Please input [Email] ");
ad.show();
txtEmail.requestFocus();
return false;
}
// Check Tel
if(txtTel.getText().length() == 0)
{
ad.setMessage("Please input [Tel] ");
ad.show();
txtTel.requestFocus();
return false;
}
SoapObject request = new SoapObject(NAMESPACE, METHOD_NAME);
request.addProperty("strUsername", txtUsername.getText().toString());
request.addProperty("strPassword", txtPassword.getText().toString());
request.addProperty("strName", txtName.getText().toString());
request.addProperty("strEmail", txtEmail.getText().toString());
request.addProperty("strTel", txtTel.getText().toString());
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(
SoapEnvelope.VER11);
envelope.setOutputSoapObject(request);
HttpTransportSE androidHttpTransport = new HttpTransportSE(URL);
String resultServer = null;
try {
androidHttpTransport.call(SOAP_ACTION, envelope);
SoapObject result = (SoapObject) envelope.bodyIn;
resultServer = result.getProperty(0).toString();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (XmlPullParserException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
/** Get result from Server (Return the JSON Code)
* StatusID = ? [0=Failed,1=Complete]
* Error = ? [On case error return custom error message]
*
* Eg Save Failed = {"StatusID":"0","Error":"Email Exists!"}
* Eg Save Complete = {"StatusID":"1","Error":""}
*/
/*** Default Value ***/
String strStatusID = "0";
String strError = "Unknow Status!";
JSONObject c;
try {
c = new JSONObject(resultServer);
strStatusID = c.getString("StatusID");
strError = c.getString("Error");
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Prepare Save Data
if(strStatusID.equals("0"))
{
ad.setMessage(strError);
ad.show();
}
else
{
Toast.makeText(MainActivity.this, "Save Data Successfully", Toast.LENGTH_SHORT).show();
txtUsername.setText("");
txtPassword.setText("");
txtConPassword.setText("");
txtName.setText("");
txtEmail.setText("");
txtTel.setText("");
}
return true;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
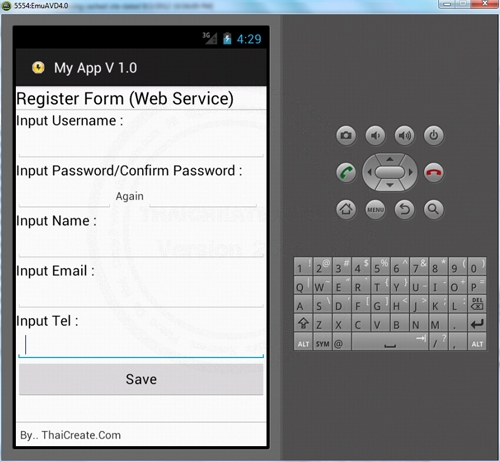
Activity Form สำหรับ Input ข้อมูล
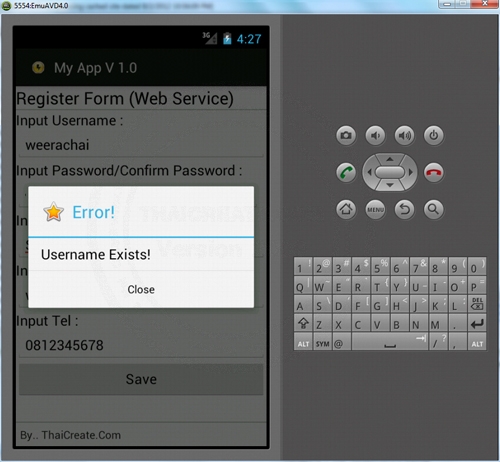
กรณีที่ Username ซ้ำ
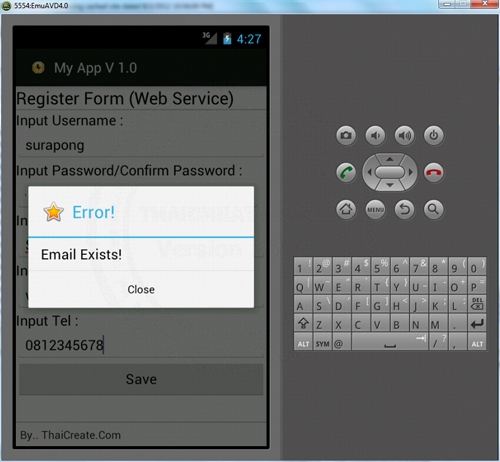
กรณีที่ Email ซ้ำ
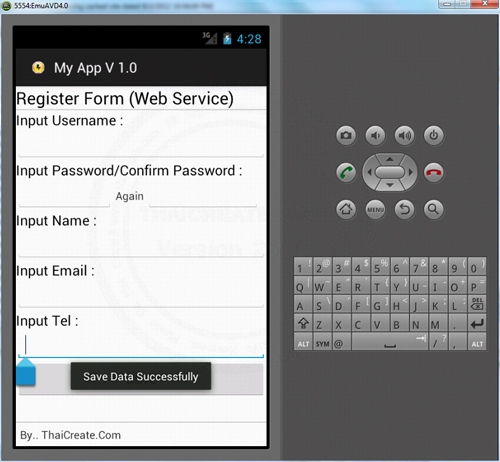
ถ้าข้อมูลถูก Insert สมบูรณ์ ก็จะแสดงข้อความแจ้งให้ทราบ เหมือนในรูปภาพประกอบ

หลังจากที่ Insert ข้อมูลแล้ว เมื่อกลับไปดู Database ที่อยู่บน Web Service ก็จะมีรายการข้อมูลขึ้นมา 1 Record

Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-08-11 15:54:20 /
2012-08-13 15:38:27 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|