Android and ArrayList (Java) |
Android and ArrayList (Java) สำหรับ ArrayList ในภาษา Java ที่จะพัฒนาบน Android นิยมใช้จัดเก็บกับชุดของข้อมูลที่ไม่ทราบขนาดหรือ Size ที่แท้จริง โดยที่เราสามารถเพิ่ม สมาชิกให้กับ ArrayList ผ่าน method ที่มีชื่อว่า add โดนสามารถเพิ่มสมาชิกหรือข้อมูลใน ArrayList ได้เรื่อย ๆ มากพอกับความต้องการของข้อมูลชุดเหล่านั้น และสามารถเพิ่มปรับลดขนาดของ ArrayList ในตำแหน่ง index ต่าง ๆ ได้ ใน ArrayList สามารถรับข้อมูลได้หลากหลายประเภท ที่อยู่ในชุดประเภทเดียวกัน รองรับข้อมูลที่ซ้ำกันได้ รวมทั้งที่เป็นค่าว่าง (null) โดยสมาชิกของ ArrayList จะถูกจัดเก็บตามลำดับที่เพิ่มเข้ามา และ ArrayList ยังเป็นชนิดที่เป็น unsynchronized สามารถเข้ามาใช้ข้อมูลใน ArrayList ได้ในเวลาเดียวกันหลาย ๆ ครั้ง โดยไม่มีปัญหาจะต้องรอคิวว่าให้ Thread อื่นที่เรียกใช้ ArrayList ตัวนั้น ๆ ทำงานจนเสร็จสิ้นเสียก่อน
รูปแบบการประกาศ ArrayList
ArrayList<String> myArrList = new ArrayList<String>();
myArrList.add("Belgium");
myArrList.add("France");
myArrList.add("Italy");
myArrList.add("Germany");
ประกาศตัวแปร myArrList เป็น ArrayList แบบ String ซึ่งมีสมาชิกอยู่ 4 รายการ คือ Belgium, France, Italy, Germany
การนับขนาด Size ใน ArrayList
myArrList.size();
การ Loop ค่าใน ArrayList มาใช้งาน
for (int i = 0; i < myArrList.size(); i++) {
// myArrList.get(i))
}
อีกวิธี
for (String temp : myArrList) {
//temp;
}
การตรวจสอบว่า ArrayList มีสมาชิกอยู่หรือไม่
if (myArrList.contains("Italy"))
{
// Already member
}
สำหรับ ArrayList การทำงานคล้าย ๆ กับ HashSet เพียงแต่ HashSet จะมไยอมให้มีสมาชิกซ้ำกันได้
Android and HashSet (Java)
Example 1 การใช้ ArrayList บน Android ด้วยภาษา Java แบบง่าย ๆ
ออกแบบ XML Layout
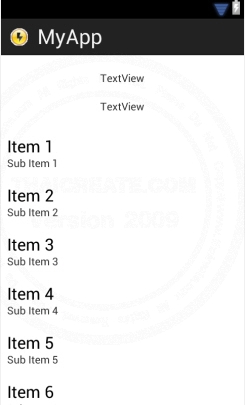
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="22dp"
android:text="TextView" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/textView1"
android:layout_below="@+id/textView1"
android:layout_marginTop="20dp"
android:text="TextView" />
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_marginTop="99dp" >
</ListView>
</RelativeLayout>
MainActivity.java
package com.myapp;
import java.util.ArrayList;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.TextView;
public class MainActivity extends Activity {
ArrayAdapter<String> adapter;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
/**** Sample 1 ****/
ArrayList<String> myArrList = new ArrayList<String>();
myArrList.add("Belgium");
myArrList.add("France");
myArrList.add("Italy");
myArrList.add("Germany");
// Get Size ArrayList
final TextView txtView1 = (TextView)findViewById(R.id.textView1);
txtView1.setText("ArrayList Size = " + myArrList.size());
// Loop Data ArrayList
final TextView txtView2 = (TextView)findViewById(R.id.textView2);
txtView2.setText("");
for (int i = 0; i < myArrList.size(); i++) {
// myArrList.get(i))
txtView2.setText(txtView2.getText() + myArrList.get(i) + ",");
}
// Loop Sample
//for (String temp : myArrList) {
// txtView2.setText(temp);
//}
// Convert ArrayList to Array
String[] myArr = {};
myArr = myArrList.toArray(new String[myArrList.size()]);
// Show Array to ListView
final ListView lView = (ListView)findViewById(R.id.listView1);
adapter = new ArrayAdapter<String>(this,
android.R.layout.simple_list_item_1, myArr);
lView.setAdapter(adapter);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
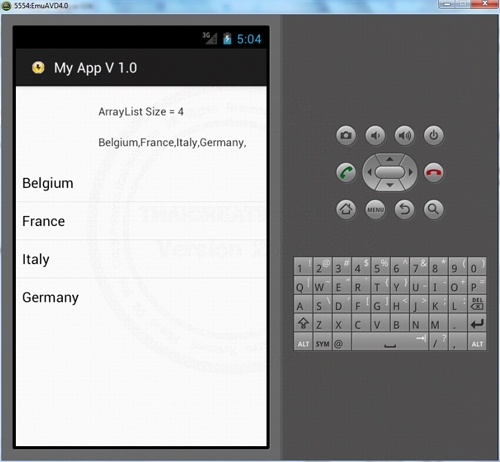
Example 2 การใช้ Array ร่วมกับ HashMap เพื่อเก็บข้อมูลที่มี Key และ Value
ออกแบบ XML Layout
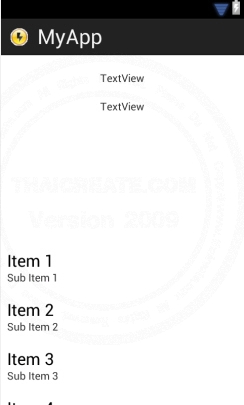
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="22dp"
android:text="TextView" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/textView1"
android:layout_below="@+id/textView1"
android:layout_marginTop="20dp"
android:text="TextView" />
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_marginTop="250dp" >
</ListView>
</RelativeLayout>
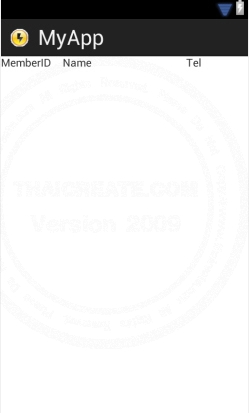
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColMemberID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="MemberID"/>
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="Name"/>
<TextView
android:id="@+id/ColTel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Tel" />
</LinearLayout>
MainActivity.java
package com.myapp;
import java.util.ArrayList;
import java.util.HashMap;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import android.widget.TextView;
public class MainActivity extends Activity {
ArrayAdapter<String> adapter;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ArrayList<HashMap<String, String>> myArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
/*** Rows 1 ***/
map = new HashMap<String, String>();
map.put("MemberID", "1");
map.put("Name", "Weerachai");
map.put("Tel", "0819876107");
myArrList.add(map);
/*** Rows 2 ***/
map = new HashMap<String, String>();
map.put("MemberID", "2");
map.put("Name", "Win");
map.put("Tel", "021978032");
myArrList.add(map);
/*** Rows 3 ***/
map = new HashMap<String, String>();
map.put("MemberID", "3");
map.put("Name", "Eak");
map.put("Tel", "0123456789");
myArrList.add(map);
// Get Size ArrayList
final TextView txtView1 = (TextView)findViewById(R.id.textView1);
txtView1.setText("ArrayList Size = " + myArrList.size());
// Loop Data ArrayList
final TextView txtView2 = (TextView)findViewById(R.id.textView2);
txtView2.setText("");
for (int i = 0; i < myArrList.size(); i++) {
String sMemberID = myArrList.get(i).get("MemberID").toString();
String sName = myArrList.get(i).get("Name").toString();
String sTel = myArrList.get(i).get("Tel").toString();
txtView2.setText(txtView2.getText() + "MemberID = " + sMemberID + ",\r\n");
txtView2.setText(txtView2.getText() + "Name = " + sName + ",\r\n");
txtView2.setText(txtView2.getText() + "Tel = " + sTel + "\r\n\r\n");
}
// Show ArrayList to ListView
final ListView lView = (ListView)findViewById(R.id.listView1);
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(MainActivity.this, myArrList, R.layout.activity_column,
new String[] {"MemberID", "Name", "Tel"}, new int[] {R.id.ColMemberID, R.id.ColName, R.id.ColTel});
lView.setAdapter(sAdap);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot

Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2012-07-29 17:45:42 /
2012-07-30 11:06:10 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|