Android Rename Move Copy file in SD Card (Java Android) |
Android Rename Move Copy file in SD Card (Java Android) ตัวอย่างการเขียน Android เปลี่ยนชื่อไฟล์ (Rename), ย้ายไฟล์ (Move), ก๊อปปี้ไฟล์ (Copy) ที่อยู่ใน SD Card
AndroidManifest.xml
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
กำหนด Permission ลงในไฟล์ AndroidManifest.xml
ออกแบบ XML Layout ดังรูป
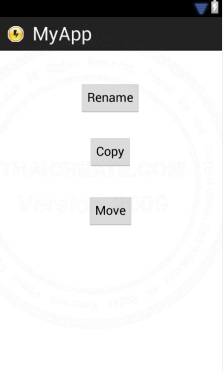
Code ทั้งหมด
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginTop="44dp"
android:text="Rename" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/button1"
android:layout_centerHorizontal="true"
android:layout_marginTop="29dp"
android:text="Copy" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:text="Move" />
</RelativeLayout>
MainActivity.java
package com.myapp;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.channels.FileChannel;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// button1 (Rename)
final Button btn1 = (Button) findViewById(R.id.button1);
// button2 (Copy)
final Button btn2 = (Button) findViewById(R.id.button2);
// button2 (Move)
final Button btn3 = (Button) findViewById(R.id.button3);
// Perform action on click (Rename)
btn1.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
/*** Rename file in SD Card ***/
try {
String OldName = "/mnt/sdcard/mydata/myfile.txt";
String NewName = "/mnt/sdcard/mydata/myNewfile.txt";
File file = new File(OldName);
file.renameTo(new File(NewName));
file = null;
Toast.makeText(MainActivity.this, "File Rename!",
Toast.LENGTH_LONG).show();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
Toast.makeText(MainActivity.this, "Failed! = " + e.getMessage() ,
Toast.LENGTH_LONG).show();
}
}
});
// Perform action on click (Copy)
btn2.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
/*** Copy file in SD Card ***/
try {
File SourceName = new File("/mnt/sdcard/mydata/myfile.txt");
File DestName = new File("/mnt/sdcard/mypicture/myfile.txt");
copyFile(SourceName,DestName);
Toast.makeText(MainActivity.this, "File Copy!",
Toast.LENGTH_LONG).show();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
Toast.makeText(MainActivity.this, "Failed! = " + e.getMessage() ,
Toast.LENGTH_LONG).show();
}
}
});
// Perform action on click (Move)
btn3.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
/*** Move file in SD Card ***/
try {
File SourceName = new File("/mnt/sdcard/mydata/myfile.txt");
File DestName = new File("/mnt/sdcard/mypicture/myfile.txt");
copyFile(SourceName,DestName); // Copy
SourceName.delete(); // Delete
Toast.makeText(MainActivity.this, "File Move!",
Toast.LENGTH_LONG).show();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
Toast.makeText(MainActivity.this, "Failed! = " + e.getMessage() ,
Toast.LENGTH_LONG).show();
}
}
});
}
public static void copyFile(File sourceFile, File destFile) throws IOException {
if(!destFile.exists()) {
destFile.createNewFile();
}
FileChannel source = null;
FileChannel destination = null;
try {
source = new FileInputStream(sourceFile).getChannel();
destination = new FileOutputStream(destFile).getChannel();
long count = 0;
long size = source.size();
while((count += destination.transferFrom(source, count, size-count))<size);
}
finally {
if(source != null) {
source.close();
}
if(destination != null) {
destination.close();
}
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
การ Rename ชื่อไฟล์
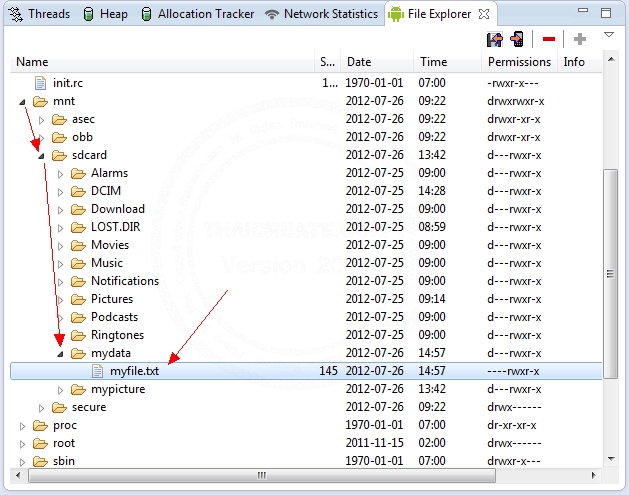
ไฟล์ก่อนการ Rename
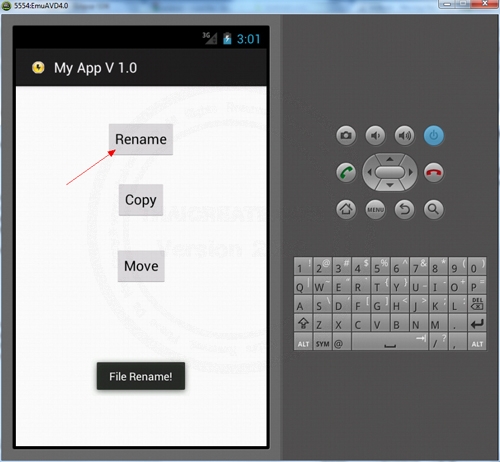
คลิกที่ Rename ไฟล์
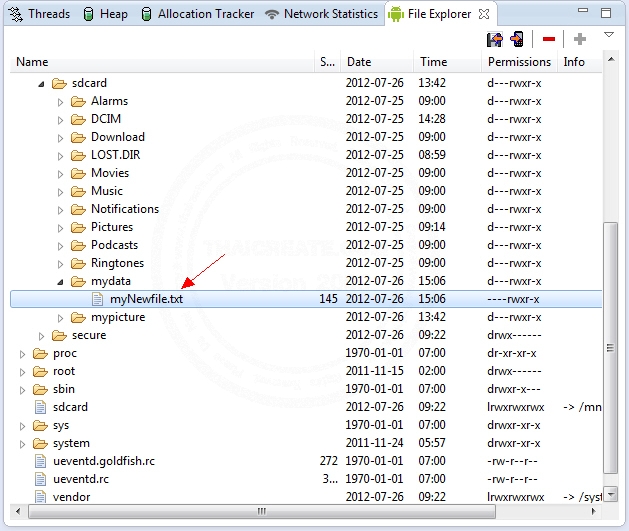
ไฟล์ที่ถูก Rename
การ Copy ชื่อไฟล์
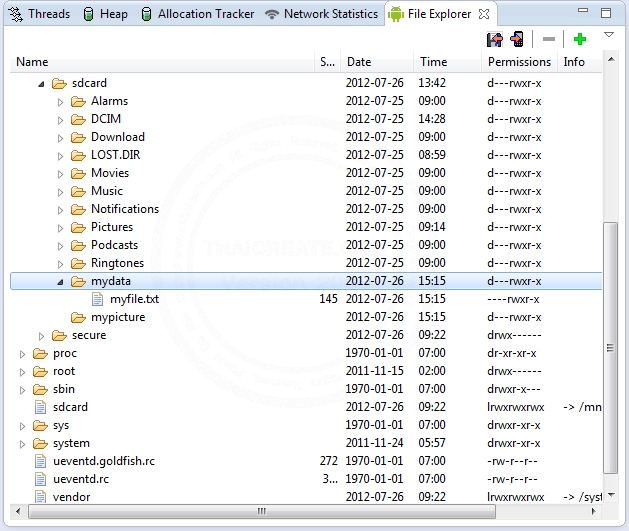
ไฟล์ก่อนทำการ Copy
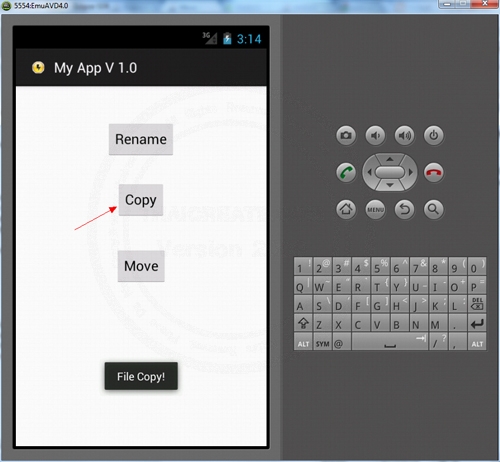
คลิกที่ Copy
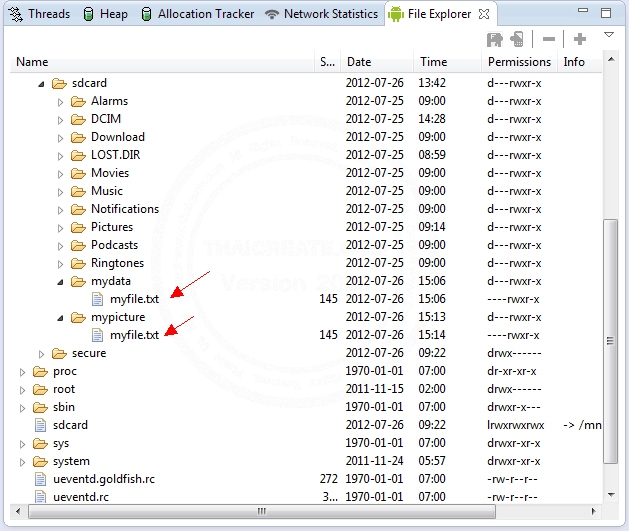
ไฟ์ที่ถูก Copy
การ Move ไฟล์
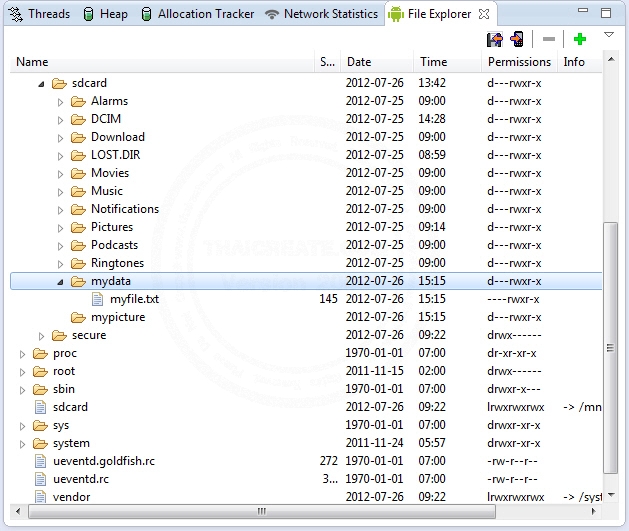
ไฟล์ก่อนการ Move
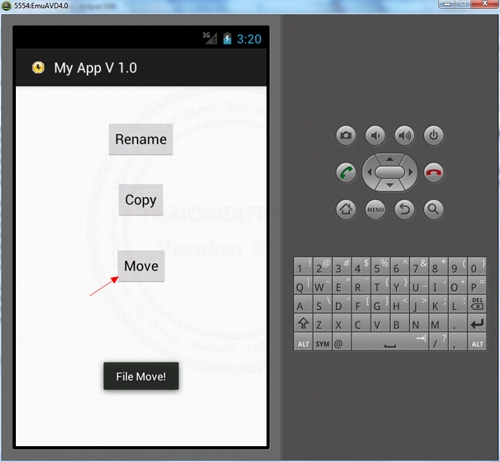
คลิกที่ Move
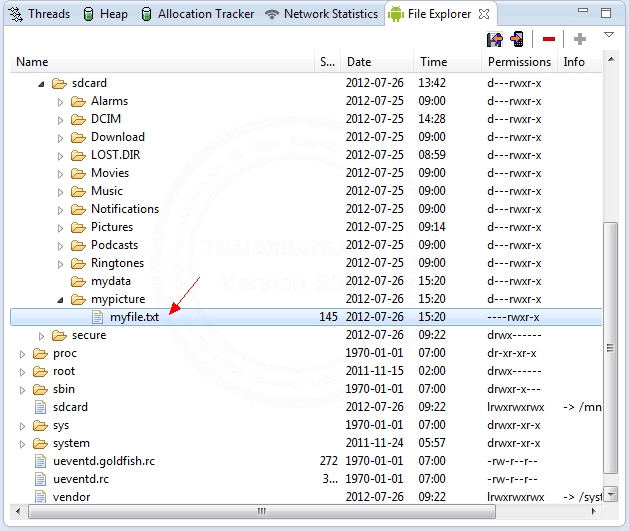
ไฟล์ที่ได้ทำการ Move เรียบร้อยแล้ว
พื้นฐาน SD Card บน Emulator สามารถอ่านได้ที่บทความนี้

Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2012-07-29 10:58:18 /
2012-07-29 13:10:22 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|