Android and JSON การนำ JSON เข้ามาช่วยในการเขียนโปรแกรมบน Android นั้นจะมีประโยชน์ในด้านการรับส่งข้อมูลระหว่าง Application ที่เป็นแบบ Server -> Client หรือ Client -> Server โดย JSON จะแปลงข้อมูลที่อยู่ในรูปแบบของ Array ให้เป็นข้อความ JSON จากนั้นข้อความเหล่านั้นจะถุกส่งไปยังปลายทาง โดยใน Application ปลายทางก็จะมี function สำหรับการ Decode ข้อความ JSON เช่นเดียวกัน นิยมการกับการรับส่งผ่าน REST หรือ Web Service และเช่นเดียวกันใน Android ที่เขียนด้วย Java ก็มีทั้ง function ที่ใช้สำหรับ EnCode JSON และ DeCode JSON เช่นเดียวกัน
- การอ่าน JSON แบบง่าย ๆ
JSON Code
Java Code
String json = "{\"sName\":\"Sawatdee : Weerachai Nukitram\",\"sEmail\":\"Sawatdee : [email protected]\"}";
JSONObject c = new JSONObject(json);
String strResultName = c.getString("sName");
String strResultEmail = c.getString("sEmail");
การอ่านค่า JSON ที่มาจากข้อมูล Array ชุดเดียว
- การอ่าน JSON ที่ที่ Array แบบ 2 มิติ
JSON Code
[{
"MemberID":"1",
"Name":"Weerachai",
"Tel":"0819876107"
},
{
"MemberID":"2",
"Name":"Win",
"Tel":"021978032"
},
{
"MemberID":"3",
"Name":"Eak",
"Tel":"087654321"
}]
Java Code
String strJSON = "[{\"MemberID\":\"1\",\"Name\":\"Weerachai\",\"Tel\":\"0819876107\"}" +
",{\"MemberID\":\"2\",\"Name\":\"Win\",\"Tel\":\"021978032\"}" +
",{\"MemberID\":\"3\",\"Name\":\"Eak\",\"Tel\":\"0876543210\"}]";
JSONArray data = new JSONArray(strJSON);
ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("MemberID", c.getString("MemberID"));
map.put("Name", c.getString("Name"));
map.put("Tel", c.getString("Tel"));
MyArrList.add(map);
}
การอ่าน JSON ที่มีค่าแบบ Array 2 มิติจะใช้ ArrayList และ HashMap เข้ามาเก็บค่า Index และ Key
- การสร้าง JSON Code ผ่าน Java Android แบบง่าย ๆ
Java Code
JSONObject object = new JSONObject();
object.put("MemberID","1");
object.put("Name", "Weerachai");
object.put("Tel", "0819876107");
JSONArray json = new JSONArray(object);
return json
JSON Code
{"MemberID":"1","Name":"Weerachai","Tel":"0819876107"}
- การสร้าง JSON Code แบบ Array 2 มิติ
Java Code
ArrayList<JSONObject> MyArrJson = new ArrayList<JSONObject >();
JSONObject object;
/*** Rows 1 ***/
object = new JSONObject();
object.put("MemberID","1");
object.put("Name", "Weerachai");
object.put("Tel", "0819876107");
MyArrJson.add(object);
/*** Rows 2 ***/
object = new JSONObject();
object.put("MemberID","2");
object.put("Name", "Win");
object.put("Tel", "021978032");
MyArrJson.add(object);
/*** Rows 3 ***/
object = new JSONObject();
object.put("MemberID","3");
object.put("Name", "Eak");
object.put("Tel", "0876543210");
MyArrJson.add(object);
JSONArray json = new JSONArray(MyArrJson);
return json;
JSON Code
[{
"MemberID":"1",
"Name":"Weerachai",
"Tel":"0819876107"
},
{
"MemberID":"2",
"Name":"Win",
"Tel":"021978032"
},
{
"MemberID":"3",
"Name":"Eak",
"Tel":"087654321"
}]

Example 1 การอ่าน JSON Code แบบง่าย ๆ โดยแสดงผล JSON บน ListView
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
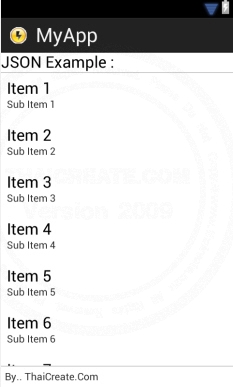
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="JSON Example : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
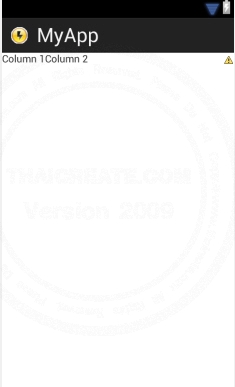
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColMemberID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="MemberID"/>
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="Name"/>
<TextView
android:id="@+id/ColTel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Tel" />
</LinearLayout>
MainActivity.java
package com.myapp;
import java.util.ArrayList;
import java.util.HashMap;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.widget.ListView;
import android.widget.SimpleAdapter;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// listView1
final ListView lisView1 = (ListView)findViewById(R.id.listView1);
/*** Sample JSON Code ***'
[{
"MemberID":"1",
"Name":"Weerachai",
"Tel":"0819876107"
},
{
"MemberID":"2",
"Name":"Win",
"Tel":"021978032"
},
{
"MemberID":"3",
"Name":"Eak",
"Tel":"0876543210"
}]
*/
String strJSON = "[{\"MemberID\":\"1\",\"Name\":\"Weerachai\",\"Tel\":\"0819876107\"}" +
",{\"MemberID\":\"2\",\"Name\":\"Win\",\"Tel\":\"021978032\"}" +
",{\"MemberID\":\"3\",\"Name\":\"Eak\",\"Tel\":\"0876543210\"}]";
try {
JSONArray data = new JSONArray(strJSON);
ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("MemberID", c.getString("MemberID"));
map.put("Name", c.getString("Name"));
map.put("Tel", c.getString("Tel"));
MyArrList.add(map);
}
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(MainActivity.this, MyArrList, R.layout.activity_column,
new String[] {"MemberID", "Name", "Tel"}, new int[] {R.id.ColMemberID, R.id.ColName, R.id.ColTel});
lisView1.setAdapter(sAdap);
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
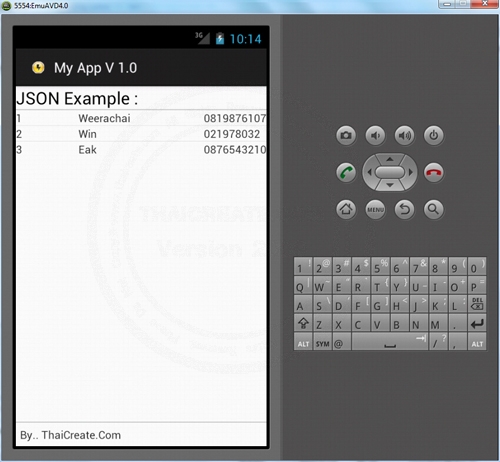
แสดง JSON Code บน ListView
Example 2 การสร้าง JSON Code และการอ่าน JSON Code แบบง่าย ๆ
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
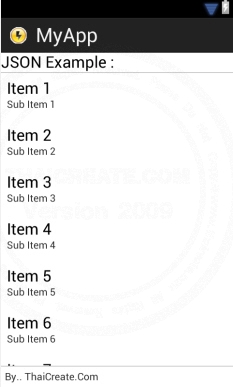
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="JSON Example : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColMemberID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="MemberID"/>
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="Name"/>
<TextView
android:id="@+id/ColTel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Tel" />
</LinearLayout>
MainActivity.java
package com.myapp;
import java.util.ArrayList;
import java.util.HashMap;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.widget.ListView;
import android.widget.SimpleAdapter;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// listView1
final ListView lisView1 = (ListView)findViewById(R.id.listView1);
try {
JSONArray data = createJSON();
ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("MemberID", c.getString("MemberID"));
map.put("Name", c.getString("Name"));
map.put("Tel", c.getString("Tel"));
MyArrList.add(map);
}
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(MainActivity.this, MyArrList, R.layout.activity_column,
new String[] {"MemberID", "Name", "Tel"}, new int[] {R.id.ColMemberID, R.id.ColName, R.id.ColTel});
lisView1.setAdapter(sAdap);
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/*** Create JSON ****/
private JSONArray createJSON() throws JSONException
{
ArrayList<JSONObject> MyArrJson = new ArrayList<JSONObject >();
JSONObject object;
/*** Rows 1 ***/
object = new JSONObject();
object.put("MemberID","1");
object.put("Name", "Weerachai");
object.put("Tel", "0819876107");
MyArrJson.add(object);
/*** Rows 2 ***/
object = new JSONObject();
object.put("MemberID","2");
object.put("Name", "Win");
object.put("Tel", "021978032");
MyArrJson.add(object);
/*** Rows 3 ***/
object = new JSONObject();
object.put("MemberID","3");
object.put("Name", "Eak");
object.put("Tel", "0876543210");
MyArrJson.add(object);
JSONArray json = new JSONArray(MyArrJson);
return json;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
คำอธิบาย
สังเกตุว่าใน Code ของ Java จะมี function สำหรับการสร้าง JSON Code คือ method createJSON() และการนำ JSON ที่ได้ไปแปลงค่าให้อยู่ในรูปแบบของ Array ก่อนที่จะแสดงผลบน ListView
Screenshot
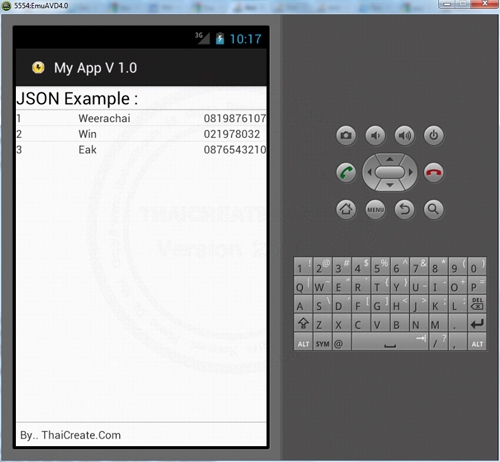
แสดงค่า JSON บน ListView
|