Android (ListView/GridView) get Result from Web Server and Paging Pagination |
Android (ListView/GridView) get Result from Web Server and Paging Pagination บทความการเขียน Android เพื่ออ่านข้อมูลจาก Web Server ที่อยู่ในรูปแบบของ JSON และเมื่อได้ JSON ที่ส่งมาทั้งหมดในครั้งเดียวมาจาก Web Server ก็จะนำข้อมูลเหล่านั้นมาจัดการแบ่งหน้าข้อมูล โดยแปลงข้อมูลที่ได้ให้อยู่ในรูปแบบ ArrayList และแสดงข้อมูลใน ArrayList ตาม Index ทีก่ำหนดในหน้านั้น ๆ ซึ่งวิธีนี้จะติดต่อกับ Web Server เพียงครั้งเดียว แต่จะใช้การเขียน function เพื่อจัดการกับข้อมูลที่ได้ ให้แสดงผลบน ListView หรือ GridView เป็นหน้า ๆ ตามต้องการ
จากภาพประกอบ แสดงการอ่านข้อมูล Web Server และการแปลงค่า JSON แสดงข้อมูลแบ่งหลาย ๆ หน้าบน ListView และ GridView
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
บทความที่เกี่ยวข้อง
Web Server (PHP and MySQL)
MySQL Database
CREATE TABLE `images` (
`ImageID` int(2) NOT NULL auto_increment,
`ItemID` varchar(50) NOT NULL,
`ImagePath` varchar(50) NOT NULL,
PRIMARY KEY (`ImageID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=31 ;
--
-- Dumping data for table `images`
--
INSERT INTO `images` VALUES (1, 'Item 01', 'https://www.thaicreate.com/android/images/img01.gif');
INSERT INTO `images` VALUES (2, 'Item 02', 'https://www.thaicreate.com/android/images/img02.gif');
INSERT INTO `images` VALUES (3, 'Item 03', 'https://www.thaicreate.com/android/images/img03.gif');
INSERT INTO `images` VALUES (4, 'Item 04', 'https://www.thaicreate.com/android/images/img04.gif');
INSERT INTO `images` VALUES (5, 'Item 05', 'https://www.thaicreate.com/android/images/img05.gif');
INSERT INTO `images` VALUES (6, 'Item 06', 'https://www.thaicreate.com/android/images/img06.gif');
INSERT INTO `images` VALUES (7, 'Item 07', 'https://www.thaicreate.com/android/images/img07.gif');
INSERT INTO `images` VALUES (8, 'Item 08', 'https://www.thaicreate.com/android/images/img08.gif');
INSERT INTO `images` VALUES (9, 'Item 09', 'https://www.thaicreate.com/android/images/img09.gif');
INSERT INTO `images` VALUES (10, 'Item 10', 'https://www.thaicreate.com/android/images/img10.gif');
INSERT INTO `images` VALUES (11, 'Item 11', 'https://www.thaicreate.com/android/images/img11.gif');
INSERT INTO `images` VALUES (12, 'Item 12', 'https://www.thaicreate.com/android/images/img12.gif');
INSERT INTO `images` VALUES (13, 'Item 13', 'https://www.thaicreate.com/android/images/img13.gif');
INSERT INTO `images` VALUES (14, 'Item 14', 'https://www.thaicreate.com/android/images/img14.gif');
INSERT INTO `images` VALUES (15, 'Item 15', 'https://www.thaicreate.com/android/images/img15.gif');
INSERT INTO `images` VALUES (16, 'Item 16', 'https://www.thaicreate.com/android/images/img16.gif');
INSERT INTO `images` VALUES (17, 'Item 17', 'https://www.thaicreate.com/android/images/img17.gif');
INSERT INTO `images` VALUES (18, 'Item 18', 'https://www.thaicreate.com/android/images/img18.gif');
INSERT INTO `images` VALUES (19, 'Item 19', 'https://www.thaicreate.com/android/images/img19.gif');
INSERT INTO `images` VALUES (20, 'Item 20', 'https://www.thaicreate.com/android/images/img20.gif');
INSERT INTO `images` VALUES (21, 'Item 21', 'https://www.thaicreate.com/android/images/img21.gif');
INSERT INTO `images` VALUES (22, 'Item 22', 'https://www.thaicreate.com/android/images/img22.gif');
INSERT INTO `images` VALUES (23, 'Item 23', 'https://www.thaicreate.com/android/images/img23.gif');
INSERT INTO `images` VALUES (24, 'Item 24', 'https://www.thaicreate.com/android/images/img24.gif');
INSERT INTO `images` VALUES (25, 'Item 25', 'https://www.thaicreate.com/android/images/img25.gif');
INSERT INTO `images` VALUES (26, 'Item 26', 'https://www.thaicreate.com/android/images/img26.gif');
INSERT INTO `images` VALUES (27, 'Item 27', 'https://www.thaicreate.com/android/images/img27.gif');
INSERT INTO `images` VALUES (28, 'Item 28', 'https://www.thaicreate.com/android/images/img28.gif');
INSERT INTO `images` VALUES (29, 'Item 29', 'https://www.thaicreate.com/android/images/img29.gif');
INSERT INTO `images` VALUES (30, 'Item 30', 'https://www.thaicreate.com/android/images/img30.gif');
getAllData.php
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM images WHERE 1 ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
จาก Code ของ PHP จะทำการ return ค่า JSON ไปยัง Client ได้ค่าดังนี้
JSON Result
[{"ImageID":"1","ItemID":"Item 01","ImagePath":"https://www.thaicreate.com/android/images/img01.gif"}
,{"ImageID":"2","ItemID":"Item 02","ImagePath":"https://www.thaicreate.com/android/images/img02.gif"}
,{"ImageID":"3","ItemID":"Item 03","ImagePath":"https://www.thaicreate.com/android/images/img03.gif"}
,{"ImageID":"4","ItemID":"Item 04","ImagePath":"https://www.thaicreate.com/android/images/img04.gif"}
,{"ImageID":"5","ItemID":"Item 05","ImagePath":"https://www.thaicreate.com/android/images/img05.gif"}
,{"ImageID":"6","ItemID":"Item 06","ImagePath":"https://www.thaicreate.com/android/images/img06.gif"}
,{"ImageID":"7","ItemID":"Item 07","ImagePath":"https://www.thaicreate.com/android/images/img07.gif"}
,{"ImageID":"8","ItemID":"Item 08","ImagePath":"https://www.thaicreate.com/android/images/img08.gif"}
,{"ImageID":"9","ItemID":"Item 09","ImagePath":"https://www.thaicreate.com/android/images/img09.gif"}
,{"ImageID":"10","ItemID":"Item 10","ImagePath":"https://www.thaicreate.com/android/images/img10.gif"}
,{"ImageID":"11","ItemID":"Item 11","ImagePath":"https://www.thaicreate.com/android/images/img11.gif"}
,{"ImageID":"12","ItemID":"Item 12","ImagePath":"https://www.thaicreate.com/android/images/img12.gif"}
,{"ImageID":"13","ItemID":"Item 13","ImagePath":"https://www.thaicreate.com/android/images/img13.gif"}
,{"ImageID":"14","ItemID":"Item 14","ImagePath":"https://www.thaicreate.com/android/images/img14.gif"}
,{"ImageID":"15","ItemID":"Item 15","ImagePath":"https://www.thaicreate.com/android/images/img15.gif"}
,{"ImageID":"16","ItemID":"Item 16","ImagePath":"https://www.thaicreate.com/android/images/img16.gif"}
,{"ImageID":"17","ItemID":"Item 17","ImagePath":"https://www.thaicreate.com/android/images/img17.gif"}
,{"ImageID":"18","ItemID":"Item 18","ImagePath":"https://www.thaicreate.com/android/images/img18.gif"}
,{"ImageID":"19","ItemID":"Item 19","ImagePath":"https://www.thaicreate.com/android/images/img19.gif"}
,{"ImageID":"20","ItemID":"Item 20","ImagePath":"https://www.thaicreate.com/android/images/img20.gif"}
,{"ImageID":"21","ItemID":"Item 21","ImagePath":"https://www.thaicreate.com/android/images/img21.gif"}
,{"ImageID":"22","ItemID":"Item 22","ImagePath":"https://www.thaicreate.com/android/images/img22.gif"}
,{"ImageID":"23","ItemID":"Item 23","ImagePath":"https://www.thaicreate.com/android/images/img23.gif"}
,{"ImageID":"24","ItemID":"Item 24","ImagePath":"https://www.thaicreate.com/android/images/img24.gif"}
,{"ImageID":"25","ItemID":"Item 25","ImagePath":"https://www.thaicreate.com/android/images/img25.gif"}
,{"ImageID":"26","ItemID":"Item 26","ImagePath":"https://www.thaicreate.com/android/images/img26.gif"}
,{"ImageID":"27","ItemID":"Item 27","ImagePath":"https://www.thaicreate.com/android/images/img27.gif"}
,{"ImageID":"28","ItemID":"Item 28","ImagePath":"https://www.thaicreate.com/android/images/img28.gif"}
,{"ImageID":"29","ItemID":"Item 29","ImagePath":"https://www.thaicreate.com/android/images/img29.gif"}
,{"ImageID":"30","ItemID":"Item 30","ImagePath":"https://www.thaicreate.com/android/images/img30.gif"}]
JSON Code ที่ถูกส่งไปยัง Android
จาก Code ของ JSON จะเห็นว่า JSON จะถุกส่งมาครั้งเดียว โดยถ้าข้อมูลมาหลายร้อยรายการ ก็จะถูกส่งกลับมายัง Web Server ทั้งหมด ทำให้ลดอัตรา Transfer การติดต่อกับ Web Server
แต่ถ้าต้อมูลมีหลายหมื่นหรือแสน Record การใช้วิธีนี้จะเป็นปัญหาอย่างยิ่ง เพราะการที่จะส่งข้อมูลขนาดนั้นมายัง Android Client ย่อมเป็นเรื่องที่ยาก เพราะฉะนั้น ถ้าข้อมูลมามากหลายหมื่นหรือแสนรายการควรจะให้เป็นหน้าที่ของ PHP กับ MySQL ที่จะจัดการกับข้อมูล และทำการอ่านข้อมูลมาแสดงผลเฉพาะในหน้านั้น ๆ ที่ต้องการ สามารถอ่านได้ที่บทความนี้
Android Split Page Data (Next,Previous) result from PHP and MySQL
Android Project
Example 1 การแสดงผลและแบ่งหน้าข้อมูลจาก Web Server บน ListView Widgets
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml

activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="ListView Pagination : "
android:layout_span="1" />
<Button
android:id="@+id/btnPre"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="<< Prev" />
<Button
android:id="@+id/btnNext"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Next >>" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
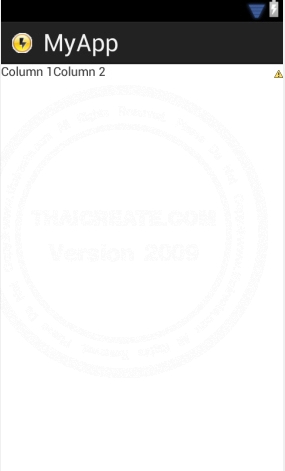
activity_column.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TableRow
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<ImageView
android:id="@+id/ColImagePath"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/ColImageID"
android:text="Column 1" />
<TextView
android:id="@+id/ColItemID"
android:text="Column 2" />
</TableRow>
</TableLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.ByteArrayOutputStream;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.app.Activity;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.AsyncTask;
import android.os.Bundle;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.view.Window;
import android.widget.BaseAdapter;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.ListView;
import android.widget.TextView;
public class MainActivity extends Activity {
private ListView lstView;
private ImageAdapter imageAdapter;
public int currentPage = 1;
public int TotalPage = 0;
public Button btnNext;
public Button btnPre;
ArrayList<HashMap<String, Object>> MyArrList = new ArrayList<HashMap<String, Object>>();
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// ProgressBar
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_main);
// ListView and imageAdapter
lstView = (ListView) findViewById(R.id.listView1);
lstView.setClipToPadding(false);
imageAdapter = new ImageAdapter(getApplicationContext());
lstView.setAdapter(imageAdapter);
// Next
btnNext = (Button) findViewById(R.id.btnNext);
// Perform action on click
btnNext.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
currentPage = currentPage + 1;
ShowData();
}
});
// Previous
btnPre = (Button) findViewById(R.id.btnPre);
// Perform action on click
btnPre.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
currentPage = currentPage - 1;
ShowData();
}
});
// Show first load
ShowData();
}
public void ShowData()
{
btnNext.setEnabled(false);
btnPre.setEnabled(false);
setProgressBarIndeterminateVisibility(true);
new LoadContentFromServer().execute();
}
class LoadContentFromServer extends AsyncTask<Object, Integer, Object> {
@Override
protected Object doInBackground(Object... params) {
String url = "https://www.thaicreate.com/android/getAllData.php";
JSONArray data;
try {
data = new JSONArray(getJSONUrl(url));
MyArrList = new ArrayList<HashMap<String, Object>>();
HashMap<String, Object> map;
/*
* TotalRows = Show for total rows
* TotalPage = Show for total page
*/
int displayPerPage = 7; // Per Page
int TotalRows = data.length();
int indexRowStart = ((displayPerPage*currentPage)-displayPerPage);
if(TotalRows<=displayPerPage)
{
TotalPage =1;
}
else if((TotalRows % displayPerPage)==0)
{
TotalPage =(TotalRows/displayPerPage) ;
}
else
{
TotalPage =(TotalRows/displayPerPage)+1;
TotalPage = (int)TotalPage;
}
int indexRowEnd = displayPerPage * currentPage;
if(indexRowEnd > TotalRows)
{
indexRowEnd = TotalRows;
}
for(int i = indexRowStart; i < indexRowEnd; i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, Object>();
map.put("ImageID", (String)c.getString("ImageID"));
map.put("ItemID", (String)c.getString("ItemID"));
// Thumbnail Get ImageBitmap To Object
map.put("ImagePath", (String)c.getString("ImagePath"));
Bitmap newBitmap = loadBitmap(c.getString("ImagePath"));
map.put("ImagePathBitmap", newBitmap);
MyArrList.add(map);
publishProgress(i);
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
@Override
public void onProgressUpdate(Integer... progress) {
imageAdapter.notifyDataSetChanged();
}
@Override
protected void onPostExecute(Object result) {
// Disabled Button Next
if(currentPage >= TotalPage)
{
btnNext.setEnabled(false);
}
else
{
btnNext.setEnabled(true);
}
// Disabled Button Previos
if(currentPage <= 1)
{
btnPre.setEnabled(false);
}
else
{
btnPre.setEnabled(true);
}
setProgressBarIndeterminateVisibility(false); // When Finish
}
}
class ImageAdapter extends BaseAdapter {
private Context mContext;
public ImageAdapter(Context context) {
mContext = context;
}
public int getCount() {
return MyArrList.size();
}
public Object getItem(int position) {
return MyArrList.get(position);
}
public long getItemId(int position) {
return position;
}
public View getView(int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) mContext
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// ColImagePath
ImageView imageView = (ImageView) convertView.findViewById(R.id.ColImagePath);
imageView.getLayoutParams().height = 60;
imageView.getLayoutParams().width = 60;
imageView.setPadding(5, 5, 5, 5);
imageView.setScaleType(ImageView.ScaleType.CENTER_CROP);
try
{
imageView.setImageBitmap((Bitmap)MyArrList.get(position).get("ImagePathBitmap"));
} catch (Exception e) {
// When Error
imageView.setImageResource(android.R.drawable.ic_menu_report_image);
}
// ColImageID
TextView txtImgID = (TextView) convertView.findViewById(R.id.ColImageID);
txtImgID.setPadding(10, 0, 0, 0);
txtImgID.setText("ID : " + MyArrList.get(position).get("ImageID").toString());
// ColItemID
TextView txtItemID = (TextView) convertView.findViewById(R.id.ColItemID);
txtItemID.setPadding(50, 0, 0, 0);
txtItemID.setText("Item : " + MyArrList.get(position).get("ItemID").toString());
return convertView;
}
}
/*** Get JSON Code from URL ***/
public String getJSONUrl(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download file..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
/***** Get Image Resource from URL (Start) *****/
private static final String TAG = "Image";
private static final int IO_BUFFER_SIZE = 4 * 1024;
public static Bitmap loadBitmap(String url) {
Bitmap bitmap = null;
InputStream in = null;
BufferedOutputStream out = null;
try {
in = new BufferedInputStream(new URL(url).openStream(), IO_BUFFER_SIZE);
final ByteArrayOutputStream dataStream = new ByteArrayOutputStream();
out = new BufferedOutputStream(dataStream, IO_BUFFER_SIZE);
copy(in, out);
out.flush();
final byte[] data = dataStream.toByteArray();
BitmapFactory.Options options = new BitmapFactory.Options();
//options.inSampleSize = 1;
bitmap = BitmapFactory.decodeByteArray(data, 0, data.length,options);
} catch (IOException e) {
Log.e(TAG, "Could not load Bitmap from: " + url);
} finally {
closeStream(in);
closeStream(out);
}
return bitmap;
}
private static void closeStream(Closeable stream) {
if (stream != null) {
try {
stream.close();
} catch (IOException e) {
android.util.Log.e(TAG, "Could not close stream", e);
}
}
}
private static void copy(InputStream in, OutputStream out) throws IOException {
byte[] b = new byte[IO_BUFFER_SIZE];
int read;
while ((read = in.read(b)) != -1) {
out.write(b, 0, read);
}
}
/***** Get Image Resource from URL (End) *****/
}
Screenshot
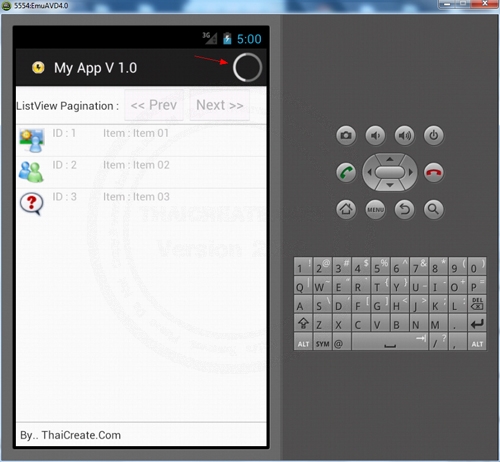
กำลังโหลดและแสดงข้อมูลจาก Web Server และจัดการกับข้อมูลโดยการแบ่งการแสดงผลออกเป็นหลายหน้า
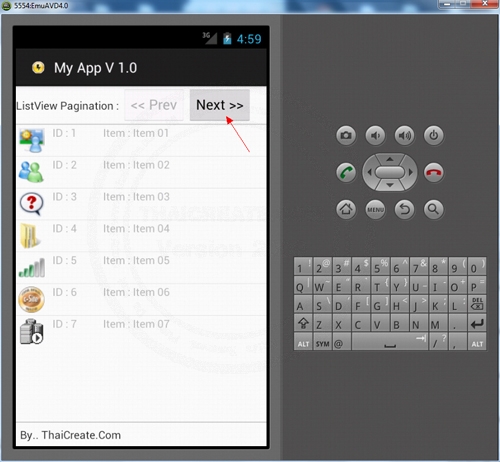
ทดสอบคลิกไปที่ Next >> เพื่อไปยังหน้าถัดไป
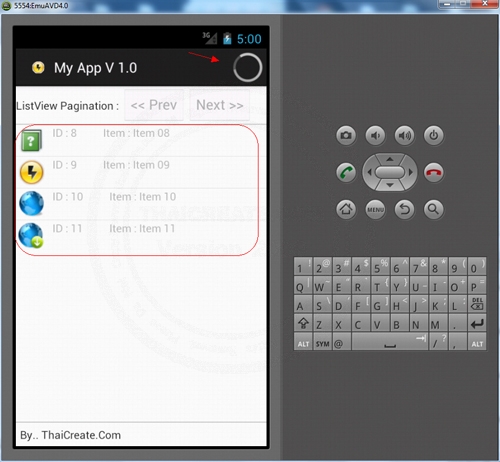
ในการเปลี่ยนแปลงหน้าทุกครั้งจะมีการใช้ ProgressBar ควบคุมการทำงาน เพื่อป้องกันโปรแกรมค้าง
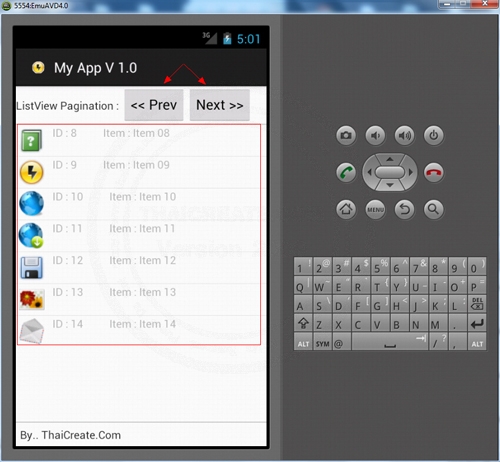
สามารถคลิก << Prev หรือ Next >> เพื่อดูรายการข้อมูลในหน้าต่าง ๆ
Example 2 การแสดงผลและแบ่งหน้าข้อมูลจาก Web Server บน GridView Widgets
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
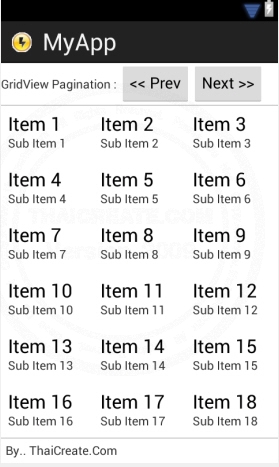
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="GridView Pagination : "
android:layout_span="1" />
<Button
android:id="@+id/btnPre"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="<< Prev" />
<Button
android:id="@+id/btnNext"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Next >>" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<GridView
android:id="@+id/gridView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:numColumns="3" >
</GridView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
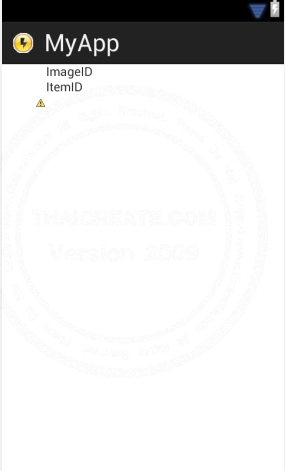
activity_column.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<ImageView
android:id="@+id/ColPhoto"
android:layout_width="50dp"
android:layout_height="50dp"
/>
<LinearLayout
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<LinearLayout
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView android:id="@+id/ColImageID"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="ImageID"
/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView android:id="@+id/ColItemID"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="ItemID"
/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.ByteArrayOutputStream;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.app.Activity;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.AsyncTask;
import android.os.Bundle;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.view.Window;
import android.widget.BaseAdapter;
import android.widget.Button;
import android.widget.GridView;
import android.widget.ImageView;
import android.widget.TextView;
public class MainActivity extends Activity {
private GridView gridV;
private ImageAdapter imageAdapter;
public int currentPage = 1;
public int TotalPage = 0;
public Button btnNext;
public Button btnPre;
ArrayList<HashMap<String, Object>> MyArrList = new ArrayList<HashMap<String, Object>>();
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// ProgressBar
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_main);
// GridView and imageAdapter
gridV = (GridView) findViewById(R.id.gridView1);
gridV.setClipToPadding(false);
imageAdapter = new ImageAdapter(getApplicationContext());
gridV.setAdapter(imageAdapter);
// Next
btnNext = (Button) findViewById(R.id.btnNext);
// Perform action on click
btnNext.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
currentPage = currentPage + 1;
ShowData();
}
});
// Previous
btnPre = (Button) findViewById(R.id.btnPre);
// Perform action on click
btnPre.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
currentPage = currentPage - 1;
ShowData();
}
});
// Show first load
ShowData();
}
public void ShowData()
{
btnNext.setEnabled(false);
btnPre.setEnabled(false);
setProgressBarIndeterminateVisibility(true);
new LoadContentFromServer().execute();
}
class LoadContentFromServer extends AsyncTask<Object, Integer, Object> {
@Override
protected Object doInBackground(Object... params) {
String url = "https://www.thaicreate.com/android/getAllData.php";
JSONArray data;
try {
data = new JSONArray(getJSONUrl(url));
MyArrList = new ArrayList<HashMap<String, Object>>();
HashMap<String, Object> map;
/*
* TotalRows = Show for total rows
* TotalPage = Show for total page
*/
int displayPerPage = 9; // Per Page
int TotalRows = data.length();
int indexRowStart = ((displayPerPage*currentPage)-displayPerPage);
if(TotalRows<=displayPerPage)
{
TotalPage =1;
}
else if((TotalRows % displayPerPage)==0)
{
TotalPage =(TotalRows/displayPerPage) ;
}
else
{
TotalPage =(TotalRows/displayPerPage)+1;
TotalPage = (int)TotalPage;
}
int indexRowEnd = displayPerPage * currentPage;
if(indexRowEnd > TotalRows)
{
indexRowEnd = TotalRows;
}
for(int i = indexRowStart; i < indexRowEnd; i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, Object>();
map.put("ImageID", (String)c.getString("ImageID"));
map.put("ItemID", (String)c.getString("ItemID"));
// Thumbnail Get ImageBitmap To Object
map.put("ImagePath", (String)c.getString("ImagePath"));
Bitmap newBitmap = loadBitmap(c.getString("ImagePath"));
map.put("ImagePathBitmap", newBitmap);
MyArrList.add(map);
publishProgress(i);
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
@Override
public void onProgressUpdate(Integer... progress) {
imageAdapter.notifyDataSetChanged();
}
@Override
protected void onPostExecute(Object result) {
// Disabled Button Next
if(currentPage >= TotalPage)
{
btnNext.setEnabled(false);
}
else
{
btnNext.setEnabled(true);
}
// Disabled Button Previos
if(currentPage <= 1)
{
btnPre.setEnabled(false);
}
else
{
btnPre.setEnabled(true);
}
setProgressBarIndeterminateVisibility(false); // When Finish
}
}
class ImageAdapter extends BaseAdapter {
private Context mContext;
public ImageAdapter(Context context) {
mContext = context;
}
public int getCount() {
return MyArrList.size();
}
public Object getItem(int position) {
return MyArrList.get(position);
}
public long getItemId(int position) {
return position;
}
public View getView(int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) mContext
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// ColPhoto
ImageView imageView = (ImageView) convertView.findViewById(R.id.ColPhoto);
imageView.getLayoutParams().height = 60;
imageView.getLayoutParams().width = 60;
imageView.setPadding(5, 5, 5, 5);
imageView.setScaleType(ImageView.ScaleType.CENTER_CROP);
try
{
imageView.setImageBitmap((Bitmap)MyArrList.get(position).get("ImagePathBitmap"));
} catch (Exception e) {
// When Error
imageView.setImageResource(android.R.drawable.ic_menu_report_image);
}
// ColImageID
TextView txtImageID = (TextView) convertView.findViewById(R.id.ColImageID);
txtImageID.setPadding(5, 0, 0, 0);
txtImageID.setText("ID : " + MyArrList.get(position).get("ImageID").toString());
// ColItemID
TextView txtItemID = (TextView) convertView.findViewById(R.id.ColItemID);
txtItemID.setPadding(5, 0, 0, 0);
txtItemID.setText("Item : " + MyArrList.get(position).get("ItemID").toString());
return convertView;
}
}
/*** Get JSON Code from URL ***/
public String getJSONUrl(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download file..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
/***** Get Image Resource from URL (Start) *****/
private static final String TAG = "Image";
private static final int IO_BUFFER_SIZE = 4 * 1024;
public static Bitmap loadBitmap(String url) {
Bitmap bitmap = null;
InputStream in = null;
BufferedOutputStream out = null;
try {
in = new BufferedInputStream(new URL(url).openStream(), IO_BUFFER_SIZE);
final ByteArrayOutputStream dataStream = new ByteArrayOutputStream();
out = new BufferedOutputStream(dataStream, IO_BUFFER_SIZE);
copy(in, out);
out.flush();
final byte[] data = dataStream.toByteArray();
BitmapFactory.Options options = new BitmapFactory.Options();
//options.inSampleSize = 1;
bitmap = BitmapFactory.decodeByteArray(data, 0, data.length,options);
} catch (IOException e) {
Log.e(TAG, "Could not load Bitmap from: " + url);
} finally {
closeStream(in);
closeStream(out);
}
return bitmap;
}
private static void closeStream(Closeable stream) {
if (stream != null) {
try {
stream.close();
} catch (IOException e) {
android.util.Log.e(TAG, "Could not close stream", e);
}
}
}
private static void copy(InputStream in, OutputStream out) throws IOException {
byte[] b = new byte[IO_BUFFER_SIZE];
int read;
while ((read = in.read(b)) != -1) {
out.write(b, 0, read);
}
}
/***** Get Image Resource from URL (End) *****/
}
Screenshot
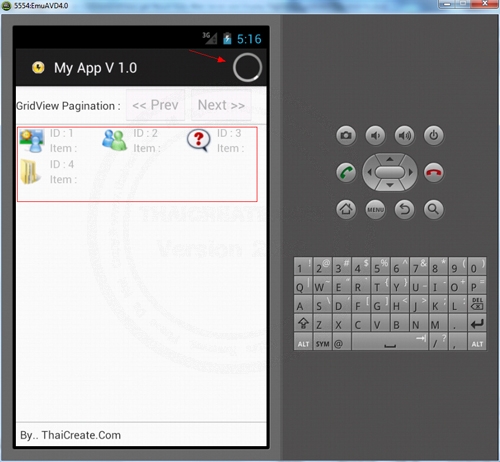
กำลังแสดงผลข้อมูลจาก Web Server บน GridView และมีการแบ่งหน้าการแสดงผลข้อมูล
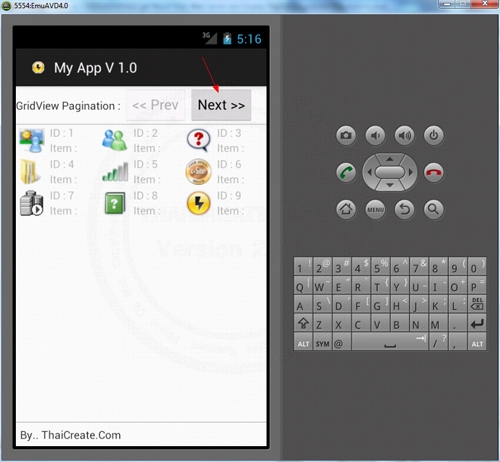
ทดสอบคลิกที่ Next >> เพื่อไปหน้าถัดไป
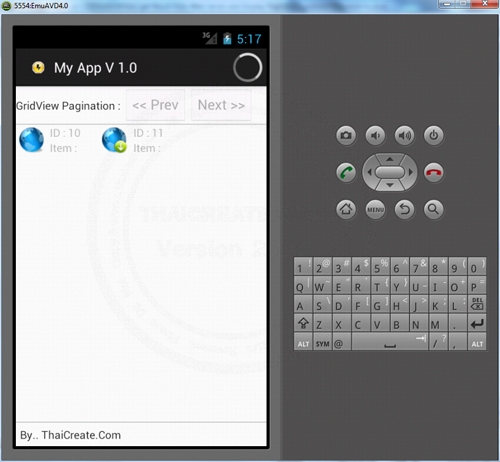
กำลังโหลดหน้าข้อมูลหในหน้าอื่น ๆ
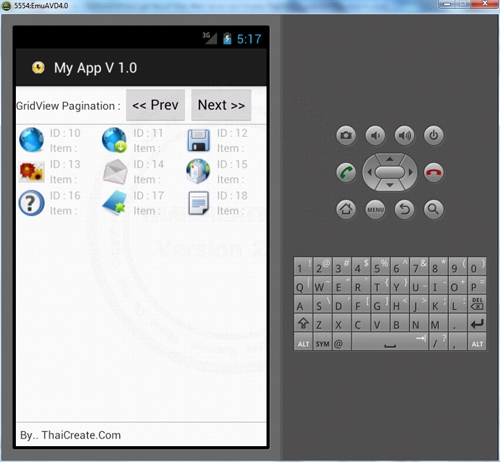
สามารถคลิกเพื่อไปดูข้อมูลในหน้าต่าง ๆ ได้
เพิ่มเติม
วิธีนี้จะเป็นการติดต่อกับ Web Server เพียงครั้งเดียว ซึ่งจะเหมาะกับข้อมูลไม่มาก อาจจะอยู่ในหลัก สิบหรือร้อยรายการ แต่ถ้าข้อมูลมีมากกว่า พัน หมื่น หรือ แสนรายการ แนะนำให้ใช้วิธีนี้
Android Split Page Data (Next,Previous) result from PHP and MySQL
|