Android ListView Padding and Pagination |
Android ListView Padding and Pagination ตัวอย่างการเขียน Android กับ ListView เพื่อจัดการ Padding และ Pagination โดยเมื่อข้อมูลใน ListView มีจำนวนมาก จะใช้การแบ่งข้อมูลเหล่านั้นแสดงผลออกเป็นหลาย ๆ หน้า แต่ล่ะหน้าจะแสดงข้อมูลขึ้นอยู่กับเรากำหนดขึ้น และมีปุ่ม Back(Previous) และ Next สำหรับการคลิกดูข้อมูลในหน้าถ้ดไป หรือจะย้อนหลังดูข้อมูล พร้อมทั้งการแสดงเลขหน้า ลำดับของข้อมูล
Exalple 1 การอ่านข้อมูลจาก JSON และมาแสดงผลแบ่งหน้าข้อมูลบน ListView
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
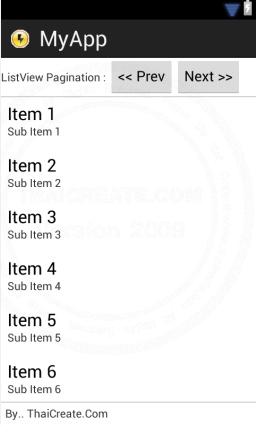
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="ListView Pagination : "
android:layout_span="1" />
<Button
android:id="@+id/btnPre"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="<< Prev" />
<Button
android:id="@+id/btnNext"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Next >>" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
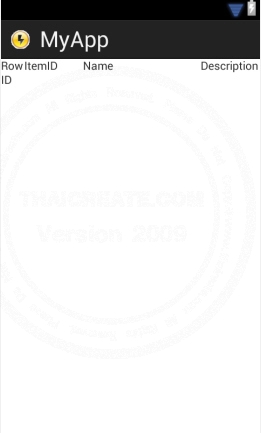
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColRowID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.4"
android:text="RowID"/>
<TextView
android:id="@+id/ColItemID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="ItemID"/>
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="Name"/>
<TextView
android:id="@+id/ColDescription"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Description" />
</LinearLayout>
MainActivity.java
package com.myapp;
import java.util.ArrayList;
import java.util.HashMap;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.ListView;
import android.widget.SimpleAdapter;
public class MainActivity extends Activity {
public String strJSON;
public int currentPage = 1;
public ListView lisView1;
public Button btnNext;
public Button btnPre;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// listView1
lisView1 = (ListView)findViewById(R.id.listView1);
// Next
btnNext = (Button) findViewById(R.id.btnNext);
// Perform action on click
btnNext.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
currentPage = currentPage + 1;
ShowData();
}
});
// Previous
btnPre = (Button) findViewById(R.id.btnPre);
// Perform action on click
btnPre.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
currentPage = currentPage - 1;
ShowData();
}
});
strJSON = "[{\"ItemID\":\"Items 01\",\"Name\":\"Data Row 01\",\"Description\":\"Desc 01\"}" +
",{\"ItemID\":\"Items 02\",\"Name\":\"Data Row 02\",\"Description\":\"Desc 02\"}" +
",{\"ItemID\":\"Items 03\",\"Name\":\"Data Row 03\",\"Description\":\"Desc 03\"}" +
",{\"ItemID\":\"Items 04\",\"Name\":\"Data Row 04\",\"Description\":\"Desc 04\"}" +
",{\"ItemID\":\"Items 05\",\"Name\":\"Data Row 05\",\"Description\":\"Desc 05\"}" +
",{\"ItemID\":\"Items 06\",\"Name\":\"Data Row 06\",\"Description\":\"Desc 06\"}" +
",{\"ItemID\":\"Items 07\",\"Name\":\"Data Row 07\",\"Description\":\"Desc 07\"}" +
",{\"ItemID\":\"Items 08\",\"Name\":\"Data Row 08\",\"Description\":\"Desc 08\"}" +
",{\"ItemID\":\"Items 09\",\"Name\":\"Data Row 09\",\"Description\":\"Desc 09\"}" +
",{\"ItemID\":\"Items 10\",\"Name\":\"Data Row 10\",\"Description\":\"Desc 10\"}" +
",{\"ItemID\":\"Items 11\",\"Name\":\"Data Row 11\",\"Description\":\"Desc 11\"}" +
",{\"ItemID\":\"Items 12\",\"Name\":\"Data Row 12\",\"Description\":\"Desc 12\"}" +
",{\"ItemID\":\"Items 13\",\"Name\":\"Data Row 13\",\"Description\":\"Desc 13\"}" +
",{\"ItemID\":\"Items 14\",\"Name\":\"Data Row 14\",\"Description\":\"Desc 14\"}" +
",{\"ItemID\":\"Items 15\",\"Name\":\"Data Row 15\",\"Description\":\"Desc 15\"}" +
",{\"ItemID\":\"Items 16\",\"Name\":\"Data Row 16\",\"Description\":\"Desc 16\"}" +
",{\"ItemID\":\"Items 17\",\"Name\":\"Data Row 17\",\"Description\":\"Desc 17\"}" +
",{\"ItemID\":\"Items 18\",\"Name\":\"Data Row 18\",\"Description\":\"Desc 18\"}" +
",{\"ItemID\":\"Items 19\",\"Name\":\"Data Row 19\",\"Description\":\"Desc 19\"}" +
",{\"ItemID\":\"Items 20\",\"Name\":\"Data Row 20\",\"Description\":\"Desc 20\"}" +
",{\"ItemID\":\"Items 21\",\"Name\":\"Data Row 21\",\"Description\":\"Desc 21\"}" +
",{\"ItemID\":\"Items 22\",\"Name\":\"Data Row 22\",\"Description\":\"Desc 22\"}" +
",{\"ItemID\":\"Items 23\",\"Name\":\"Data Row 23\",\"Description\":\"Desc 23\"}" +
",{\"ItemID\":\"Items 24\",\"Name\":\"Data Row 24\",\"Description\":\"Desc 24\"}" +
",{\"ItemID\":\"Items 25\",\"Name\":\"Data Row 25\",\"Description\":\"Desc 25\"}" +
",{\"ItemID\":\"Items 26\",\"Name\":\"Data Row 26\",\"Description\":\"Desc 26\"}" +
",{\"ItemID\":\"Items 27\",\"Name\":\"Data Row 27\",\"Description\":\"Desc 27\"}" +
",{\"ItemID\":\"Items 28\",\"Name\":\"Data Row 28\",\"Description\":\"Desc 28\"}" +
",{\"ItemID\":\"Items 29\",\"Name\":\"Data Row 29\",\"Description\":\"Desc 29\"}" +
",{\"ItemID\":\"Items 30\",\"Name\":\"Data Row 30\",\"Description\":\"Desc 29\"}" +
",{\"ItemID\":\"Items 31\",\"Name\":\"Data Row 31\",\"Description\":\"Desc 31\"}]";
ShowData();
}
public void ShowData()
{
try {
JSONArray data = new JSONArray(strJSON);
int displayPerPage = 5; // Per Page
int TotalRows = data.length();
int indexRowStart = ((displayPerPage*currentPage)-displayPerPage);
int TotalPage = 0;
if(TotalRows<=displayPerPage)
{
TotalPage =1;
}
else if((TotalRows % displayPerPage)==0)
{
TotalPage =(TotalRows/displayPerPage) ;
}
else
{
TotalPage =(TotalRows/displayPerPage)+1;
TotalPage = (int)TotalPage;
}
int indexRowEnd = displayPerPage * currentPage;
if(indexRowEnd > TotalRows)
{
indexRowEnd = TotalRows;
}
// Disabled Button Next
if(currentPage >= TotalPage)
{
btnNext.setEnabled(false);
}
else
{
btnNext.setEnabled(true);
}
// Disabled Button Previos
if(currentPage <= 1)
{
btnPre.setEnabled(false);
}
else
{
btnPre.setEnabled(true);
}
// Load Data from Index
int RowID = 1;
ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
// RowID
if(currentPage > 1)
{
RowID = (displayPerPage * (currentPage-1)) + 1;
}
for(int i = indexRowStart; i < indexRowEnd; i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("RowID", String.valueOf(RowID));
map.put("ItemID", c.getString("ItemID"));
map.put("Name", c.getString("Name"));
map.put("Description", c.getString("Description"));
MyArrList.add(map);
RowID = RowID + 1;
}
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(MainActivity.this, MyArrList, R.layout.activity_column,
new String[] {"RowID", "ItemID", "Name", "Description"}, new int[] {R.id.ColRowID, R.id.ColItemID, R.id.ColName, R.id.ColDescription});
lisView1.setAdapter(sAdap);
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
จาก Code หลักการง่าย ๆ ก็คือ จำนวนข้อมูลมาคำนวณจำนวนหน้า โดยหาตำแหน่งของ Array ตามนำนวนหน้าและจำนวนข้อมูลที่ต้องแสดงผล
int displayPerPage = 5; // Per Page
int TotalRows = data.length();
int indexRowStart = ((displayPerPage*currentPage)-displayPerPage);
int TotalPage = 0;
if(TotalRows<=displayPerPage)
{
TotalPage =1;
}
else if((TotalRows % displayPerPage)==0)
{
TotalPage =(TotalRows/displayPerPage) ;
}
else
{
TotalPage =(TotalRows/displayPerPage)+1;
TotalPage = (int)TotalPage;
}
int indexRowEnd = displayPerPage * currentPage;
if(indexRowEnd > TotalRows)
{
indexRowEnd = TotalRows;
}
การคำนวณหาตำแหน่งของ Array ที่จะแสดงข้อมูลในแต่ล่ะหน้า โดยจะได้่ indexRowStart และ indexRowEnd เป็น Index ของ Array ที่จะแสดงข้อมูลในหน้านั้น ๆ
Screenshot
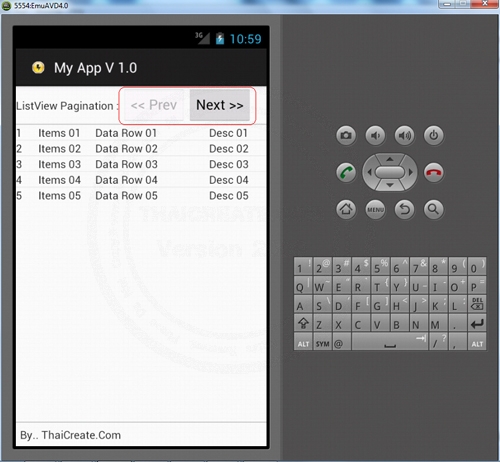
ปุ่ม << Prev และ ปุ่ม Next >> โดยถ้าอยู่หน้าแรก ปุ่ม Previous จะถุก Disabled
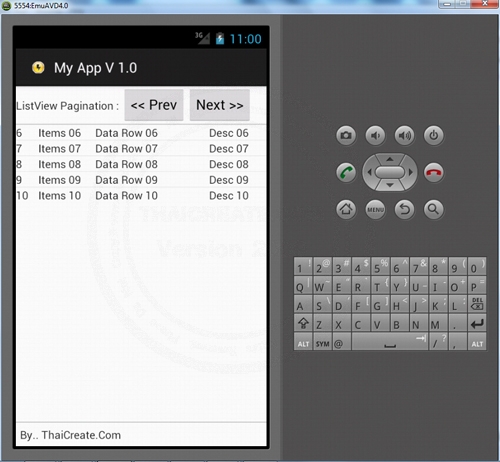
แสดงข้อมูลในหน้าถัดไป
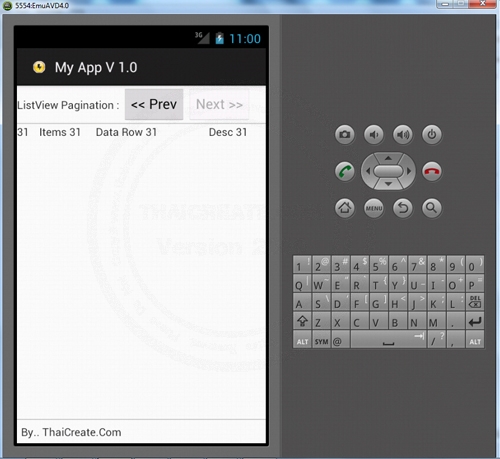
เมื่ออยู่หน้าสุดท้ายปุ่ม Next >> จะถูก Disabled
Example 2 การแบ่งหน้าข้อมูลบน ListView ที่อยู่ในรุปแบบของ ArrayList
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
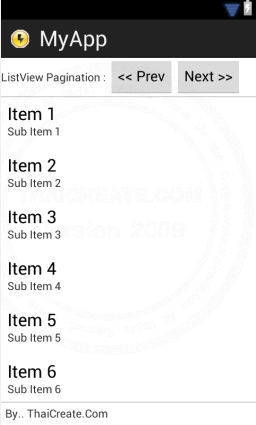
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="ListView Pagination : "
android:layout_span="1" />
<Button
android:id="@+id/btnPre"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="<< Prev" />
<Button
android:id="@+id/btnNext"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Next >>" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
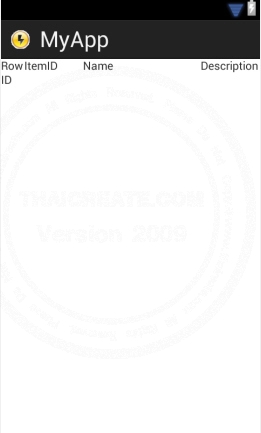
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColRowID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.4"
android:text="RowID"/>
<TextView
android:id="@+id/ColItemID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="ItemID"/>
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="Name"/>
<TextView
android:id="@+id/ColDescription"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Description" />
</LinearLayout>
MainActivity.java
package com.myapp;
import java.util.ArrayList;
import java.util.HashMap;
import android.os.Bundle;
import android.app.Activity;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.Button;
import android.widget.ListView;
import android.widget.TextView;
public class MainActivity extends Activity {
public int currentPage = 1;
public int displayPerPage = 6; // Display Perpage
public int indexRowStart = 0;
public int indexRowEnd = 0;
public int TotalRows = 0;
public ListView lisView1;
public Button btnNext;
public Button btnPre;
ArrayList<HashMap<String, String>> myArrList;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// listView1
lisView1 = (ListView)findViewById(R.id.listView1);
// Next
btnNext = (Button) findViewById(R.id.btnNext);
// Perform action on click
btnNext.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
currentPage = currentPage + 1;
progressShowData();
}
});
// Previous
btnPre = (Button) findViewById(R.id.btnPre);
// Perform action on click
btnPre.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
currentPage = currentPage - 1;
progressShowData();
}
});
/*
* ArrayList Data
* ArrayList Data
* ArrayList Data
*/
myArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 1; i<=53; i++)
{
map = new HashMap<String, String>();
map.put("RowID", String.valueOf(i));
map.put("ItemID", "Items " + (i));
map.put("Name", "Data Row " + (i));
map.put("Description", "Desc " + (i));
myArrList.add(map);
}
progressShowData();
}
public void progressShowData()
{
// Total Record
TotalRows = myArrList.size();
// Start Index
indexRowStart = ((displayPerPage*currentPage)-displayPerPage);
int TotalPage = 0;
if(TotalRows<=displayPerPage)
{
TotalPage =1;
}
else if((TotalRows % displayPerPage)==0)
{
TotalPage =(TotalRows/displayPerPage) ;
}
else
{
TotalPage =(TotalRows/displayPerPage)+1;
TotalPage = (int)TotalPage;
}
// Disabled Button Next
if(currentPage >= TotalPage)
{
btnNext.setEnabled(false);
}
else
{
btnNext.setEnabled(true);
}
// Disabled Button Previos
if(currentPage <= 1)
{
btnPre.setEnabled(false);
}
else
{
btnPre.setEnabled(true);
}
// Show Data in listView
lisView1.setAdapter(new myAdapter(this));
}
public class myAdapter extends BaseAdapter
{
private Context context;
public myAdapter(Context c)
{
// TODO Auto-generated method stub
context = c;
}
public int getCount() {
// TODO Auto-generated method stub
if(displayPerPage > TotalRows - indexRowStart)
{
return TotalRows - indexRowStart;
}
else
{
return displayPerPage;
}
}
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
public View getView(final int position, View convertView,
ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// ColRowID
TextView RowID = (TextView) convertView.findViewById(R.id.ColRowID);
RowID.setText(myArrList.get(position+indexRowStart).get("RowID") +".");
// ColItemID
TextView ItemID = (TextView) convertView.findViewById(R.id.ColItemID);
ItemID.setText(myArrList.get(position+indexRowStart).get("ItemID"));
// ColName
TextView Name = (TextView) convertView.findViewById(R.id.ColName);
Name.setText(myArrList.get(position+indexRowStart).get("Name"));
// ColDescription
TextView Description = (TextView) convertView.findViewById(R.id.ColDescription);
Description.setText(myArrList.get(position+indexRowStart).get("Description"));
return convertView;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
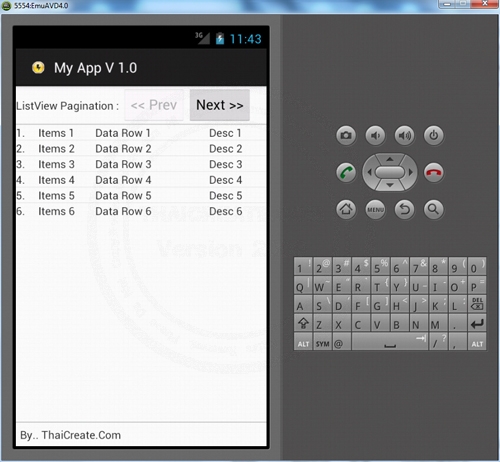
แสดงการแบ่งหน้าของข้อมูลที่อยุ่ใน ArrayList บน ListView
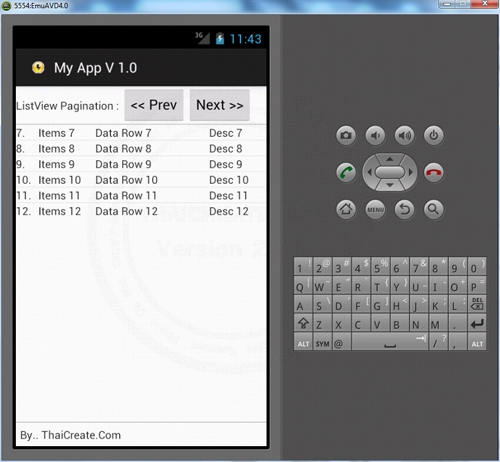
แสดงหน้าอื่น ๆ
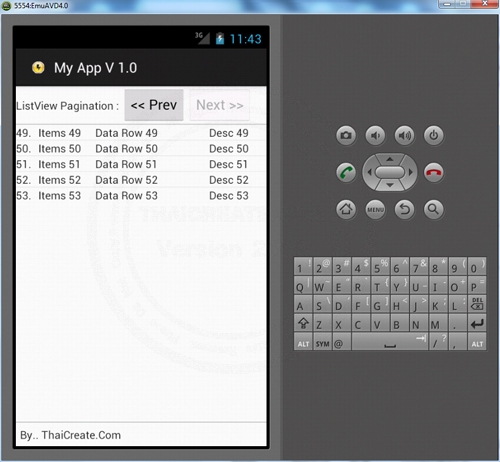
เมื่อถึงหน้าสุดท้ายและจะไม่สามารถคลิกปุ่ม Next เพื่อไปหน้าถัดไปได้
|