Android ProgressBar/ProgressDialog Search Display result Data from Web Server |
Android Show ProgressBar/ProgressDialog Search Display result Data from Web Server ตัวอย่างการทำระบบ Search ค้นหาข้อมูลจาก Web Server แบบง่าย ๆ ด้วย Android โดยจะดึงข้อมูลจาก Web Server ผ่าน HttpGet และ HttpPost โดย Web Server จะส่งข้อมูลกลับในรูปแบบของ JSON และเมื่อ Android ได้ค่า JSON ที่ถูกส่งมาจาก Server จะใช้ function JSONArray ของ Android ทำการแปลงข้อมูลให้อยู่ในรูปแบบของ Array และแสดงผลข้อมูลที่ได้บน ListView โดยกระบวนการขั้นตอนการทำงานทั้งหมดจะใช้ ProgressBar และ AsyncTask ทำงานควบคู่กัน
รูป Screenshot อธิบายการทำงาน การ ค้นหาข้อมูล (Search) และการแสดง ProgressBar
Android HttpGet and HttpPost
Android AsyncTask and ProgressBar
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Web Server
member
CREATE TABLE `member` (
`MemberID` int(2) NOT NULL auto_increment,
`Username` varchar(50) NOT NULL,
`Password` varchar(50) NOT NULL,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
`Email` varchar(150) NOT NULL,
PRIMARY KEY (`MemberID`),
UNIQUE KEY `Username` (`Username`),
UNIQUE KEY `Email` (`Email`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'weerachai', 'weerachai@1', 'Weerachai Nukitram', '0819876107', '[email protected]');
INSERT INTO `member` VALUES (2, 'adisorn', 'adisorn@2', 'Adisorn Bunsong', '021978032', '[email protected]');
INSERT INTO `member` VALUES (3, 'surachai', 'surachai@3', 'Surachai Sirisart', '0876543210', '[email protected]');
โครงสร้างของข้อมูล MySQL ที่อยู่บน Web Server

searchData.php
<?
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
// $_POST["txtKeyword"] = "a"; // for Sample
$strKeyword = $_POST["txtKeyword"];
$strSQL = "SELECT * FROM member WHERE 1 AND Name LIKE '%".$strKeyword."%' ORDER BY MemberID ASC ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
ไฟล์ php ที่ทำงานอยู่บน Web Server
Android Project
Example 1 การสร้างระบบ Search ค้นหาข้อมูล และการแสดง ProgressDialog ในขณะที่กำลังโหลดข้อมูล
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
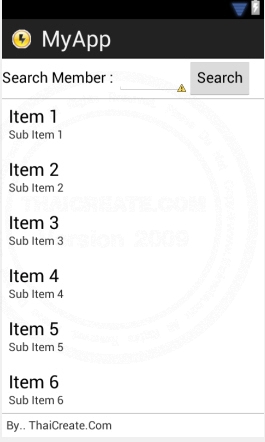
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Search Member : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtKeySearch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="4" >
</EditText>
<Button
android:id="@+id/btnSearch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Search" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>

activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColMemberID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.2"
android:text="CustomerID"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1.0"
android:text="Name"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/ColTel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Tel"
android:textAppearance="?android:attr/textAppearanceSmall" />
</LinearLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.content.Context;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.view.inputmethod.InputMethodManager;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.SimpleAdapter;
public class MainActivity extends Activity {
public static final int DIALOG_DOWNLOAD_JSON_PROGRESS = 0;
private ProgressDialog mProgressDialog;
ArrayList<HashMap<String, String>> MyArrList;
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// btnSearch
final Button btnSearch = (Button) findViewById(R.id.btnSearch);
//btnSearch.setBackgroundColor(Color.TRANSPARENT);
// Perform action on click
btnSearch.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Download JSON File
new DownloadSearchFileAsync().execute();
}
});
}
@Override
protected Dialog onCreateDialog(int id) {
switch (id) {
case DIALOG_DOWNLOAD_JSON_PROGRESS:
mProgressDialog = new ProgressDialog(this);
mProgressDialog.setMessage("Downloading.....");
mProgressDialog.setProgressStyle(ProgressDialog.STYLE_SPINNER);
mProgressDialog.setCancelable(true);
mProgressDialog.show();
return mProgressDialog;
default:
return null;
}
}
public class DownloadSearchFileAsync extends AsyncTask<String, Void, Void> {
protected void onPreExecute() {
super.onPreExecute();
showDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
}
@Override
protected Void doInBackground(String... arg) {
// TODO Auto-generated method stub
// keySearch
EditText strKeySearch = (EditText)findViewById(R.id.txtKeySearch);
// Disbled Keyboard auto focus
InputMethodManager imm = (InputMethodManager)getSystemService(
Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(strKeySearch.getWindowToken(), 0);
/**
* [
* {"MemberID":"1","Username":"weerachai","Password":"weerachai@1","Name":"Weerachai Nukitram","Tel":"0819876107","Email":"[email protected]"},
* {"MemberID":"2","Username":"adisorn","Password":"adisorn@2","Name":"Adisorn Bunsong","Tel":"021978032","Email":"[email protected]"},
* {"MemberID":"3","Username":"surachai","Password":"surachai@3","Name":"Surachai Sirisart","Tel":"0876543210","Email":"[email protected]"}
* ]
*/
String url = "https://www.thaicreate.com/android/searchData.php";
// Paste Parameters
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("txtKeyword", strKeySearch.getText().toString()));
try {
JSONArray data = new JSONArray(getJSONUrl(url,params));
MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("MemberID", c.getString("MemberID"));
map.put("Username", c.getString("Username"));
map.put("Password", c.getString("Password"));
map.put("Name", c.getString("Name"));
map.put("Email", c.getString("Email"));
map.put("Tel", c.getString("Tel"));
MyArrList.add(map);
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
protected void onPostExecute(Void unused) {
ShowSearchData(); // When Finish Show Content
dismissDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
removeDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
}
}
// Show Search Data
public void ShowSearchData()
{
// listView1
final ListView lisView1 = (ListView)findViewById(R.id.listView1);
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(MainActivity.this, MyArrList, R.layout.activity_column,
new String[] {"MemberID", "Name", "Tel"}, new int[] {R.id.ColMemberID, R.id.ColName, R.id.ColTel});
lisView1.setAdapter(sAdap);
}
// Get JSON code from URL
public String getJSONUrl(String url,List<NameValuePair> params) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
try {
httpPost.setEntity(new UrlEncodedFormEntity(params));
HttpResponse response = client.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}

Screenshot
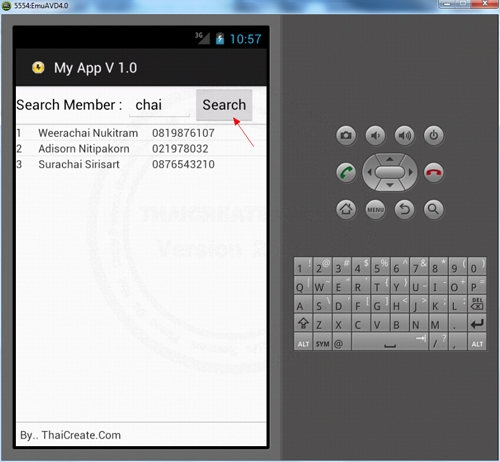
กรอก Keyword ที่ต้องการค้นหา
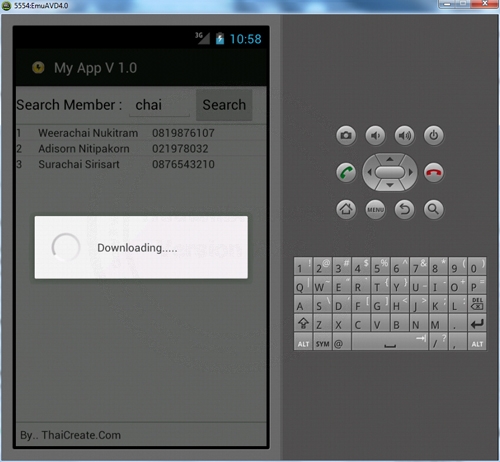
ค้นหาข้อมูล และแสดง ProgressDialog
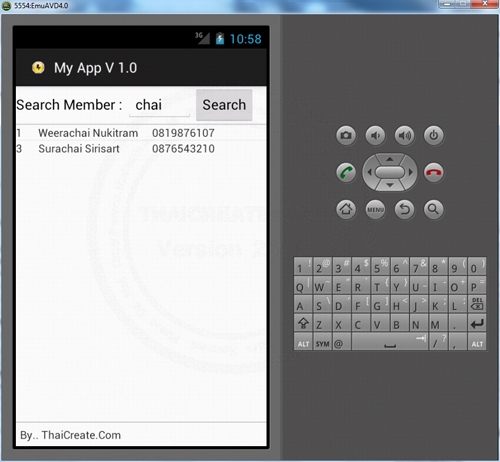
แสดงผลหลังจากที่ได้ทำการค้นหาเรียบร้อยแล้ว
Example 1.1 สร้าง ProgressBar แบบ Indeterminate Progress Bar (Icon หมุ่น อยู่ด้านบนขวาของ Title Bar)
MainActivity.java
package com.myapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.content.Context;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.view.Window;
import android.view.inputmethod.InputMethodManager;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.SimpleAdapter;
public class MainActivity extends Activity {
ArrayList<HashMap<String, String>> MyArrList;
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_main);
setProgressBarIndeterminateVisibility(false);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// btnSearch
final Button btnSearch = (Button) findViewById(R.id.btnSearch);
//btnSearch.setBackgroundColor(Color.TRANSPARENT);
// Perform action on click
btnSearch.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Download JSON File
new DownloadSearchFileAsync().execute();
}
});
}
public class DownloadSearchFileAsync extends AsyncTask<String, Void, Void> {
protected void onPreExecute() {
super.onPreExecute();
setProgressBarIndeterminateVisibility(true);
}
@Override
protected Void doInBackground(String... arg) {
// TODO Auto-generated method stub
// keySearch
EditText strKeySearch = (EditText)findViewById(R.id.txtKeySearch);
// Disbled Keyboard auto focus
InputMethodManager imm = (InputMethodManager)getSystemService(
Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(strKeySearch.getWindowToken(), 0);
/**
* [
* {"MemberID":"1","Username":"weerachai","Password":"weerachai@1","Name":"Weerachai Nukitram","Tel":"0819876107","Email":"[email protected]"},
* {"MemberID":"2","Username":"adisorn","Password":"adisorn@2","Name":"Adisorn Bunsong","Tel":"021978032","Email":"[email protected]"},
* {"MemberID":"3","Username":"surachai","Password":"surachai@3","Name":"Surachai Sirisart","Tel":"0876543210","Email":"[email protected]"}
* ]
*/
String url = "https://www.thaicreate.com/android/searchData.php";
// Paste Parameters
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("txtKeyword", strKeySearch.getText().toString()));
try {
JSONArray data = new JSONArray(getJSONUrl(url,params));
MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("MemberID", c.getString("MemberID"));
map.put("Username", c.getString("Username"));
map.put("Password", c.getString("Password"));
map.put("Name", c.getString("Name"));
map.put("Email", c.getString("Email"));
map.put("Tel", c.getString("Tel"));
MyArrList.add(map);
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
protected void onPostExecute(Void unused) {
ShowSearchData(); // When Finish Show Content
setProgressBarIndeterminateVisibility(false);
}
}
// Show Search Data
public void ShowSearchData()
{
// listView1
final ListView lisView1 = (ListView)findViewById(R.id.listView1);
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(MainActivity.this, MyArrList, R.layout.activity_column,
new String[] {"MemberID", "Name", "Tel"}, new int[] {R.id.ColMemberID, R.id.ColName, R.id.ColTel});
lisView1.setAdapter(sAdap);
}
// Get JSON code from URL
public String getJSONUrl(String url,List<NameValuePair> params) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
try {
httpPost.setEntity(new UrlEncodedFormEntity(params));
HttpResponse response = client.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
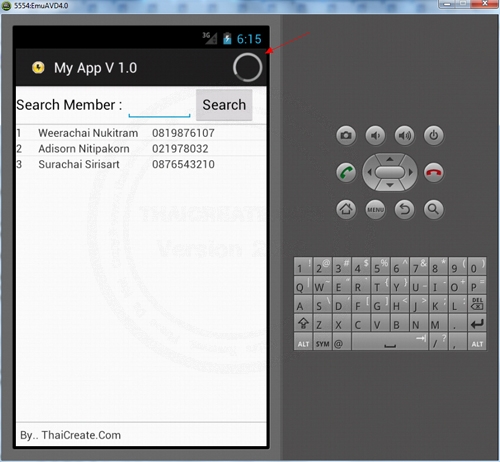
แสดง Indeterminate Progress Bar ในขณะที่กำลังโหลดข้อมูลจาก Web Server
AsyncTask and ProgressDialog (showDialog, dismissDialog, removeDialog : Deprecated)
|