Android Rating (Vote) and ListView Part 1 |
Android Rating (Vote) and ListView Part 1 บทความนี้จะเป็นเทคนิคการแสดงผล และ Update ข้อมูลบน ListView ของ Android ในระบบของการ Vote Rating โดยสร้างระบบ Vote ขึ้นมาผ่าน Dialog Popup ซึ่งจะแสดงผลหลังจากที่มีการคลิกที่ Item ของ ListView หลังจากที่แสดง Dialog Popup ที่ประกอบด้วย รูปภาพ และปุ่ม Vote Rating สำหรับให้คะแนน และเมื่อผู้ใช้คลิกที่ Vote เรียบร้อยแล้วข้อมูลจะถูกส่งไป Update ที่ Web Server (PHP กับ mySQL) และหลังจากที่ Update เรียบร้อยแล้ว ในส่วนของ ListView จะมีการ Update RatingBar ที่อยู่ใน ListView ทันที และจะทำการ Update เฉพาะข้อมูลใน Item นั้น ๆ ของ ListView โดยไม่ต้องไป Refresh ข้อมูลทั้ง ListView หรือ เรียกข้อมูลมาจาก Server อีกครั้ง
และในตัวอย่าง Example 1.1 จะเป้นการเพิ่มคุณสมบัติของ RatingBar ที่อยู่ใน ListView คือสามารถทำการคลิกหรือลาก RatingBar ที่อยู่ในแต่ล่ะ Item ของ ListView และข้อมูลจะถูกส่งไป Update ที่ Web Server ทันที (สุดยอดจริง ๆ )
จากภาพประกอบ เป็นตัวอย่างการแสดงข้อมูลบน ListView และ Dialog Popup สำหรับการ Vote รวมทั้งเขียน Event เพื่อควบคุม RatingBar ในแต่ล่ะ Item ของ ListView
บทความที่น่าสนใจที่เกี่ยวกับ Rating และการ Vote - Android Vote and Rating (PHP and MySQL)
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Web Server (PHP and MySQL)
MySQL Database
CREATE TABLE `images` (
`ImageID` int(11) NOT NULL auto_increment,
`ImageName` varchar(50) NOT NULL,
`ImagePath_Thumbnail` varchar(150) NOT NULL,
`ImagePath_FullPhoto` varchar(150) NOT NULL,
`Rating` float NOT NULL,
PRIMARY KEY (`ImageID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=7 ;
--
-- Dumping data for table `images`
--
INSERT INTO `images` VALUES (1, 'Image 1', 'https://www.thaicreate.com/android/img1_thum.jpg', 'https://www.thaicreate.com/android/img1_full.jpg', 0);
INSERT INTO `images` VALUES (2, 'Image 2', 'https://www.thaicreate.com/android/img2_thum.jpg', 'https://www.thaicreate.com/android/img2_full.jpg', 0);
INSERT INTO `images` VALUES (3, 'Image 3', 'https://www.thaicreate.com/android/img3_thum.jpg', 'https://www.thaicreate.com/android/img3_full.jpg', 0);
INSERT INTO `images` VALUES (4, 'Image 4', 'https://www.thaicreate.com/android/img4_thum.jpg', 'https://www.thaicreate.com/android/img4_full.jpg', 0);
INSERT INTO `images` VALUES (5, 'Image 5', 'https://www.thaicreate.com/android/img5_thum.jpg', 'https://www.thaicreate.com/android/img5_full.jpg', 0);
INSERT INTO `images` VALUES (6, 'Image 6', 'https://www.thaicreate.com/android/img6_thum.jpg', 'https://www.thaicreate.com/android/img6_full.jpg', 0);
โครงสร้างของตารางและข้อมูล
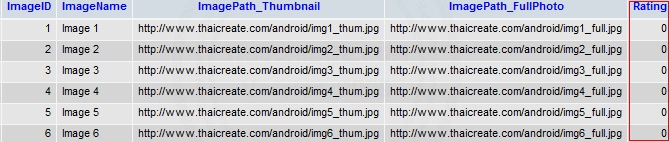
getGallery.php (ไฟล์สำหรับแสดงข้อมูลบน ListView)
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM images WHERE 1 ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
updateRating.php (ไฟล์สำหรับ Update Rating ที่ถูกส่งมาจาก Android Client)
<?php
//$_POST["ImageID"] = "1"; // ImageID
//$_POST["ratingPoint"] = "3.5"; // ratingPoint
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
/*** Update ***/
$strSQL = " UPDATE images SET
Rating = '".$_POST["ratingPoint"]."'
WHERE ImageID = '".$_POST["ImageID"]."'
";
$objQuery = mysql_query($strSQL);
if(!$objQuery) // When Error
{
$arr['StatusID'] = "0";
$arr['Error'] = "Cannot save data!";
// Return Current Rating
$strSQL = "SELECT * FROM images WHERE 1 AND ImageID = '".$_POST["ImageID"]."' ";
$objQuery = mysql_query($strSQL);
$obResult = mysql_fetch_array($objQuery);
if($obResult)
{
$arr['Rating'] = $obResult["Rating"];
}
else
{
$arr['Rating'] = "0";
}
}
else
{
// Return New Rating Point
$strSQL = "SELECT * FROM images WHERE 1 AND ImageID = '".$_POST["ImageID"]."' ";
$objQuery = mysql_query($strSQL);
$obResult = mysql_fetch_array($objQuery);
if($obResult)
{
$arr['StatusID'] = "1";
$arr['Error'] = "";
$arr['Rating'] = $obResult["Rating"];
}
}
/**
$arr['StatusID'] // (0=Failed , 1=Complete)
$arr['Error'] // Error Message
$arr['Rating'] // New Rating Point
*/
mysql_close($objConnect);
echo json_encode($arr);
?>

Android Project
Example 1 แสดงข้อมูลบน ListView และคลิกที่รูปภาพบน ListView จะแสดง Popup AlertDialog ในการโหวต
โครงสร้างของไฟล์ทั้งหมด
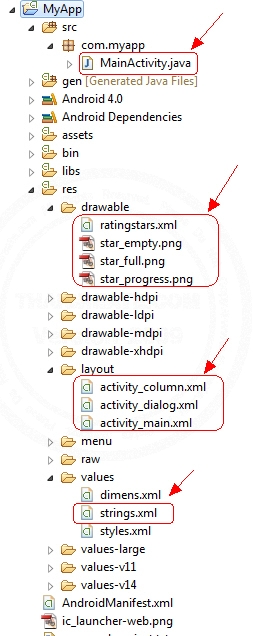
ในตัวอย่างนี้มีการใช้ Custom RatingBar ซึ่งจะสร้าง Style ขึ้นมามีรายละเอียดดังนี้
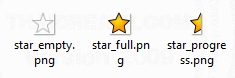
Icons รูปภาพ ที่จะใช้แสดงบน Custom RatingBar
/res/drawable/ratingstars.xml
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+android:id/background"
android:drawable="@drawable/star_empty" />
<item android:id="@+android:id/secondaryProgress"
android:drawable="@drawable/star_empty" />
<item android:id="@+android:id/progress"
android:drawable="@drawable/star_full" />
</layer-list>
สร้าง Style อยู่บนไฟล์ ratingstars.xml
/values/string.xml
<style name="styleRatingBar" parent="@android:style/Widget.RatingBar">
<item name="android:progressDrawable">@drawable/ratingstars</item>
<item name="android:minHeight">22dip</item>
<item name="android:maxHeight">22dip</item>
</style>
เพิ่มคำสั่งชุดนี้ลงใน string.xml
รายละเอียดเพิ่มเติมของ Custom RatingBar สามารถอ่านได้ที่นี่
ออกแบบ XML layout และ Java ดังต่อไปนี้
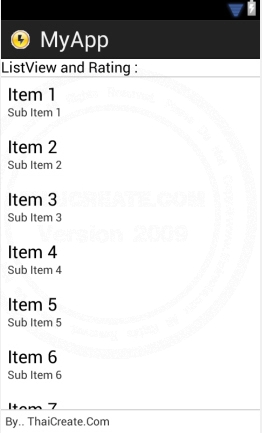
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="ListView and Rating : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceMedium" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
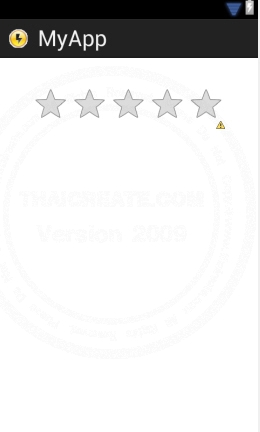
activity_dialog.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/layout_dialog"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="150dp"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="10dp" />
<RatingBar
android:id="@+id/ratingBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/imageView"
android:layout_centerHorizontal="true"
android:layout_marginTop="22dp" />
</RelativeLayout>
activity_column.xml
<TableLayout android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android">
<TableRow
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<ImageView
android:id="@+id/ColImgPath"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/ColImgID"
android:text="Column 1" />
<TextView
android:id="@+id/ColImgName"
android:text="Column 2" />
<RatingBar
android:id="@+id/ColratingBar"
style="@style/styleRatingBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:max="5"
android:numStars="5" />
</TableRow>
</TableLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.ByteArrayOutputStream;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.content.Context;
import android.content.DialogInterface;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.Menu;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.ListView;
import android.widget.RatingBar;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends Activity {
public static final int DIALOG_DOWNLOAD_JSON_PROGRESS = 0;
private ProgressDialog mProgressDialog;
ArrayList<HashMap<String, Object>> MyArrList;
ListView lstView1;
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// Download JSON File
new DownloadJSONFileAsync().execute();
}
@Override
protected Dialog onCreateDialog(int id) {
switch (id) {
case DIALOG_DOWNLOAD_JSON_PROGRESS:
mProgressDialog = new ProgressDialog(this);
mProgressDialog.setMessage("Downloading.....");
mProgressDialog.setProgressStyle(ProgressDialog.STYLE_SPINNER);
mProgressDialog.setCancelable(true);
mProgressDialog.show();
return mProgressDialog;
default:
return null;
}
}
// Show All Content
public void ShowAllContent()
{
// listView1
lstView1 = (ListView)findViewById(R.id.listView1);
lstView1.setAdapter(new ImageAdapter(MainActivity.this,MyArrList));
}
public class ImageAdapter extends BaseAdapter
{
private Context context;
private ArrayList<HashMap<String, Object>> MyArr = new ArrayList<HashMap<String, Object>>();
public ImageAdapter(Context c, ArrayList<HashMap<String, Object>> myArrList)
{
// TODO Auto-generated method stub
context = c;
MyArr = myArrList;
}
public int getCount() {
// TODO Auto-generated method stub
return MyArr.size();
}
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
public View getView(final int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// ColImage
ImageView imageView = (ImageView) convertView.findViewById(R.id.ColImgPath);
imageView.getLayoutParams().height = 80;
imageView.getLayoutParams().width = 80;
imageView.setPadding(10, 10, 10, 10);
imageView.setScaleType(ImageView.ScaleType.CENTER_CROP);
try
{
imageView.setImageBitmap((Bitmap)MyArr.get(position).get("ImageThumBitmap"));
} catch (Exception e) {
// When Error
imageView.setImageResource(android.R.drawable.ic_menu_report_image);
}
// Click on Image
imageView.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String strImageID = MyArr.get(position).get("ImageID").toString();
String strImageName = MyArr.get(position).get("ImageName").toString();
String strCurrentRating = MyArr.get(position).get("Rating").toString();
ShowDialogVote(position, strImageID,
strImageName, strCurrentRating,
MyArr.get(position).get("ImagePathFull").toString()); // Click Show Dialog Vote
}
});
// ColImgID
TextView txtImgID = (TextView) convertView.findViewById(R.id.ColImgID);
txtImgID.setPadding(5, 0, 0, 0);
txtImgID.setText("ID : " + MyArr.get(position).get("ImageID").toString());
// ColImgName
TextView txtPicName = (TextView) convertView.findViewById(R.id.ColImgName);
txtPicName.setPadding(5, 0, 0, 0);
txtPicName.setText("Name : " + MyArr.get(position).get("ImageName").toString());
// ColratingBar
RatingBar Rating = (RatingBar) convertView.findViewById(R.id.ColratingBar);
Rating.setPadding(10, 0, 0, 0);
Rating.setEnabled(false);
Rating.setMax(5);
Rating.setRating(Float.valueOf(MyArr.get(position).get("Rating").toString()));
return convertView;
}
}
// Show Dialog Vote
public void ShowDialogVote(final int position, final String strImageID,
String strImageName,final String strCurrentRating,String FullImagePath)
{
final AlertDialog.Builder popDialog = new AlertDialog.Builder(this);
final AlertDialog.Builder adb = new AlertDialog.Builder(this);
final LayoutInflater inflater = (LayoutInflater) this.getSystemService(LAYOUT_INFLATER_SERVICE);
final View Viewlayout = inflater.inflate(R.layout.activity_dialog,
(ViewGroup) findViewById(R.id.layout_dialog));
final ImageView image = (ImageView)Viewlayout.findViewById(R.id.imageView); // imageView
image.setImageBitmap((Bitmap)loadBitmap(FullImagePath));
final RatingBar rating = (RatingBar)Viewlayout.findViewById(R.id.ratingBar); // ratingBar
rating.setMax(5);
rating.setNumStars(5);
popDialog.setIcon(android.R.drawable.btn_star_big_on);
popDialog.setTitle("Vote!! (" + strImageName + ") ");
popDialog.setView(Viewlayout);
// Button OK
popDialog.setPositiveButton("OK",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
// Save Vote
String url = "https://www.thaicreate.com/android/updateRating.php";
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("ImageID", strImageID));
params.add(new BasicNameValuePair("ratingPoint", String.valueOf(rating.getRating())));
String resultServer = getHttpPost(url,params);
/** Get result from Server (Return the JSON Code)
* StatusID = ? [0=Failed,1=Complete]
* Error = ? [On case error return custom error message]
* Rating = ? [New Reting Point from Server]
*
* Eg Save Failed = {"StatusID":"0","Error":"Not Update Data!","Rating":"0"}
* Eg Save Complete = {"StatusID":"1","Error":"","Rating":"3.5"}
*/
/*** Default Value ***/
String strStatusID = "0";
String strError = "Unknow Status!";
String strRatingPoint = strCurrentRating;
JSONObject c;
try {
c = new JSONObject(resultServer);
strStatusID = c.getString("StatusID");
strError = c.getString("Error");
strRatingPoint = c.getString("Rating"); // New Rating from Server
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Prepare Save Data
if(strStatusID.equals("0"))
{
adb.setMessage(strError);
adb.show();
}
else
{
Log.d("strRatingPoint",strRatingPoint);
UpdateNewRatingPoint(position,strRatingPoint); // Update New Rating Point to ListView (By Row Item)
// Show Toast
Toast.makeText(MainActivity.this, "Vote Finished (Point : " + rating.getRating() + ")", Toast.LENGTH_LONG).show();
}
dialog.dismiss();
}
})
// Button Cancel
.setNegativeButton("Cancel",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
dialog.cancel();
}
});
popDialog.create();
popDialog.show();
}
// Update New Rating to ListView
private void UpdateNewRatingPoint(int position,String newRatingPoint){
View v = lstView1.getChildAt(position - lstView1.getFirstVisiblePosition());
// Update RatingBar
RatingBar rating = (RatingBar)v.findViewById(R.id.ColratingBar);
rating.setEnabled(true); // Enabled
rating.setRating(Float.valueOf(newRatingPoint));
rating.setEnabled(false); // False
}
// Download JSON in Background
public class DownloadJSONFileAsync extends AsyncTask<String, Void, Void> {
protected void onPreExecute() {
super.onPreExecute();
showDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
}
@Override
protected Void doInBackground(String... params) {
// TODO Auto-generated method stub
String url = "https://www.thaicreate.com/android/getGallery.php";
JSONArray data;
try {
data = new JSONArray(getJSONUrl(url));
MyArrList = new ArrayList<HashMap<String, Object>>();
HashMap<String, Object> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, Object>();
map.put("ImageID", (String)c.getString("ImageID"));
map.put("ImageName", (String)c.getString("ImageName"));
// Thumbnail Get ImageBitmap To Object
map.put("ImagePathThum", (String)c.getString("ImagePath_Thumbnail"));
map.put("ImageThumBitmap", (Bitmap)loadBitmap(c.getString("ImagePath_Thumbnail")));
// Full (for View Popup)
map.put("ImagePathFull", (String)c.getString("ImagePath_FullPhoto"));
map.put("Rating", (String)c.getString("Rating"));
MyArrList.add(map);
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
protected void onPostExecute(Void unused) {
ShowAllContent(); // When Finish Show Content
dismissDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
removeDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
}
}
// get Http Post
public String getHttpPost(String url,List<NameValuePair> params) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
try {
httpPost.setEntity(new UrlEncodedFormEntity(params));
HttpResponse response = client.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Status OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
/*** Get JSON Code from URL ***/
public String getJSONUrl(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download file..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
/***** Get Image Resource from URL (Start) *****/
private static final String TAG = "Image";
private static final int IO_BUFFER_SIZE = 4 * 1024;
public static Bitmap loadBitmap(String url) {
Bitmap bitmap = null;
InputStream in = null;
BufferedOutputStream out = null;
try {
in = new BufferedInputStream(new URL(url).openStream(), IO_BUFFER_SIZE);
final ByteArrayOutputStream dataStream = new ByteArrayOutputStream();
out = new BufferedOutputStream(dataStream, IO_BUFFER_SIZE);
copy(in, out);
out.flush();
final byte[] data = dataStream.toByteArray();
BitmapFactory.Options options = new BitmapFactory.Options();
//options.inSampleSize = 1;
bitmap = BitmapFactory.decodeByteArray(data, 0, data.length,options);
} catch (IOException e) {
Log.e(TAG, "Could not load Bitmap from: " + url);
} finally {
closeStream(in);
closeStream(out);
}
return bitmap;
}
private static void closeStream(Closeable stream) {
if (stream != null) {
try {
stream.close();
} catch (IOException e) {
android.util.Log.e(TAG, "Could not close stream", e);
}
}
}
private static void copy(InputStream in, OutputStream out) throws IOException {
byte[] b = new byte[IO_BUFFER_SIZE];
int read;
while ((read = in.read(b)) != -1) {
out.write(b, 0, read);
}
}
/***** Get Image Resource from URL (End) *****/
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
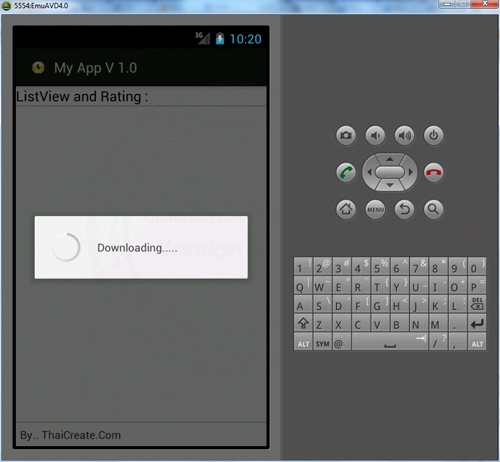
กำลังแสดงข้อมูลบน ListView โดยจะแสดงสถานะ ProgressBar ในขณะที่กำลังโหลดข้อมูล
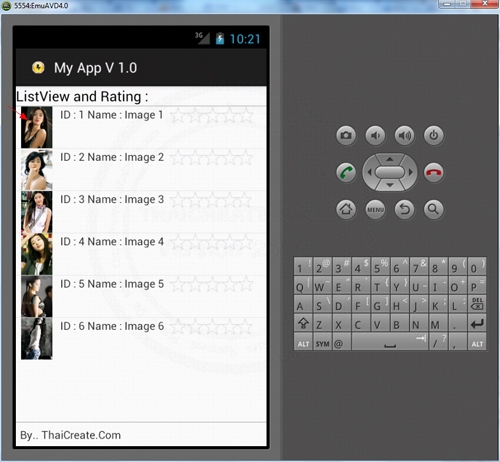
แสดงข้อมูลบน ListView หลังจากทที่โหลดข้อมูลเรียบร้อยแล้ว สามารถคลิกที่รูปภาพของแต่ล่ะ Item เพื่อเปิด Dialog Popup
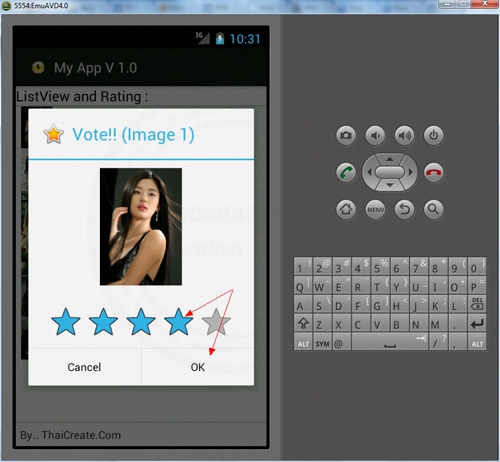
แสดง Dialog Popup พร้อมกับ Vote ในส่วนของ RatingBar
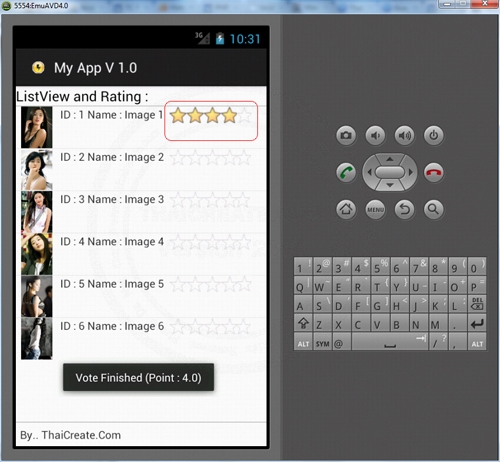
เมื่อโหวด (Vote) เรียบร้อยแล้ว ListView จะถูก Update ทันที โดยการทำงานจะมีการ Update เฉพาะ Item นั้น ๆ ซึ่งจะไม่ต้อง Refresh ListView ใหม่
private void UpdateNewRatingPoint(int position,String newRatingPoint){
View v = lstView1.getChildAt(position - lstView1.getFirstVisiblePosition());
// Update RatingBar
RatingBar rating = (RatingBar)v.findViewById(R.id.ColratingBar);
rating.setEnabled(true); // Enabled
rating.setRating(Float.valueOf(newRatingPoint));
rating.setEnabled(false); // False
}
จาก Code จะเห็นว่ามีการอ้างอึง Widget ของ RatingBar ที่อยู่ใน ListView เฉพาะ Item ที่อ้างถึง เพาะฉะนั้นวิธีนี้จะเป็นผลดีที่ไม่ต้องมีการโหลดข้อมูล หรอเรียกข้อมูลจาก Server ซ้ำ
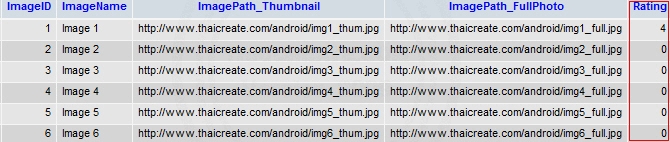
เมื่อกลับไปดู phpMyAdmin เปิดดู MySQL Database บนฝั่ง Web Server จะเห็นว่ามีการ Update Rating ในส่วนของ รายการนั้น ๆ
Example 1.1 เพิ่มคุณสมบัติให้สามารถคลิกบน RatingBar บน ListView เพื่อโหวตได้ทันที
จากภาพเมื่อคลิกที่ RatingBar ที่อยู่บน ListView จะมีการโหวตข้อมูลทันที และสามารถเลือกรายการหลาย ๆ รายการพร้อมกัน
MainActivity.java
package com.myapp;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.ByteArrayOutputStream;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.Menu;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.ListView;
import android.widget.RatingBar;
import android.widget.RatingBar.OnRatingBarChangeListener;
import android.widget.TextView;
public class MainActivity extends Activity {
public static final int DIALOG_DOWNLOAD_JSON_PROGRESS = 0;
private ProgressDialog mProgressDialog;
ArrayList<HashMap<String, Object>> MyArrList;
ListView lstView1;
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// Download JSON File
new DownloadJSONFileAsync().execute();
}
@Override
protected Dialog onCreateDialog(int id) {
switch (id) {
case DIALOG_DOWNLOAD_JSON_PROGRESS:
mProgressDialog = new ProgressDialog(this);
mProgressDialog.setMessage("Downloading.....");
mProgressDialog.setProgressStyle(ProgressDialog.STYLE_SPINNER);
mProgressDialog.setCancelable(true);
mProgressDialog.show();
return mProgressDialog;
default:
return null;
}
}
// Show All Content
public void ShowAllContent()
{
// listView1
lstView1 = (ListView)findViewById(R.id.listView1);
lstView1.setAdapter(new ImageAdapter(MainActivity.this,MyArrList));
}
public class ImageAdapter extends BaseAdapter
{
private Context context;
private ArrayList<HashMap<String, Object>> MyArr = new ArrayList<HashMap<String, Object>>();
public ImageAdapter(Context c, ArrayList<HashMap<String, Object>> myArrList)
{
// TODO Auto-generated method stub
context = c;
MyArr = myArrList;
}
public int getCount() {
// TODO Auto-generated method stub
return MyArr.size();
}
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
public View getView(final int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// ColImage
ImageView imageView = (ImageView) convertView.findViewById(R.id.ColImgPath);
imageView.getLayoutParams().height = 80;
imageView.getLayoutParams().width = 80;
imageView.setPadding(10, 10, 10, 10);
imageView.setScaleType(ImageView.ScaleType.CENTER_CROP);
try
{
imageView.setImageBitmap((Bitmap)MyArr.get(position).get("ImageThumBitmap"));
} catch (Exception e) {
// When Error
imageView.setImageResource(android.R.drawable.ic_menu_report_image);
}
// ColImgID
TextView txtImgID = (TextView) convertView.findViewById(R.id.ColImgID);
txtImgID.setPadding(5, 0, 0, 0);
txtImgID.setText("ID : " + MyArr.get(position).get("ImageID").toString());
// ColImgName
TextView txtPicName = (TextView) convertView.findViewById(R.id.ColImgName);
txtPicName.setPadding(5, 0, 0, 0);
txtPicName.setText("Name : " + MyArr.get(position).get("ImageName").toString());
// ColratingBar
RatingBar Rating = (RatingBar) convertView.findViewById(R.id.ColratingBar);
Rating.setPadding(10, 0, 0, 0);
Rating.setMax(5);
Rating.setRating(Float.valueOf(MyArr.get(position).get("Rating").toString()));
// When Change Rating Bar
Rating.setOnRatingBarChangeListener(new OnRatingBarChangeListener() {
public void onRatingChanged(RatingBar ratingBar, float rating,
boolean fromUser) {
if(fromUser)
{
String strImageID = MyArr.get(position).get("ImageID").toString();
String strImageName = MyArr.get(position).get("ImageName").toString();
// Save Vote
String url = "https://www.thaicreate.com/android/updateRating.php";
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("ImageID", strImageID));
params.add(new BasicNameValuePair("ratingPoint", String.valueOf(rating)));
String resultServer = getHttpPost(url,params);
/** Get result from Server (Return the JSON Code)
* StatusID = ? [0=Failed,1=Complete]
* Error = ? [On case error return custom error message]
* Rating = ? [New Reting Point from Server]
*
* Eg Save Failed = {"StatusID":"0","Error":"Not Update Data!","Rating":"0"}
* Eg Save Complete = {"StatusID":"1","Error":"","Rating":"3.5"}
*/
/*** Default Value ***/
String strStatusID = "0";
String strError = "Unknow Status!";
String strRatingPoint = "0";
JSONObject c;
try {
c = new JSONObject(resultServer);
strStatusID = c.getString("StatusID");
strError = c.getString("Error");
strRatingPoint = c.getString("Rating"); // New Rating from Server
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Prepare Save Data
if(strStatusID.equals("0"))
{
UpdateNewRatingPoint(position,strRatingPoint); // When Error Update Old Rating
}
else
{
UpdateNewRatingPoint(position,strRatingPoint); // Update New Rating Point to ListView (By Row Item)
}
}
}
});
return convertView;
}
}
// Update New Rating to ListView
private void UpdateNewRatingPoint(int position,String newRatingPoint){
View v = lstView1.getChildAt(position - lstView1.getFirstVisiblePosition());
// Update RatingBar
RatingBar rating = (RatingBar)v.findViewById(R.id.ColratingBar);
rating.setRating(Float.valueOf(newRatingPoint));
}
// Download JSON in Background
public class DownloadJSONFileAsync extends AsyncTask<String, Void, Void> {
protected void onPreExecute() {
super.onPreExecute();
showDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
}
@Override
protected Void doInBackground(String... params) {
// TODO Auto-generated method stub
String url = "https://www.thaicreate.com/android/getGallery.php";
JSONArray data;
try {
data = new JSONArray(getJSONUrl(url));
MyArrList = new ArrayList<HashMap<String, Object>>();
HashMap<String, Object> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, Object>();
map.put("ImageID", (String)c.getString("ImageID"));
map.put("ImageName", (String)c.getString("ImageName"));
// Thumbnail Get ImageBitmap To Object
map.put("ImagePathThum", (String)c.getString("ImagePath_Thumbnail"));
map.put("ImageThumBitmap", (Bitmap)loadBitmap(c.getString("ImagePath_Thumbnail")));
// Full (for View Popup)
map.put("ImagePathFull", (String)c.getString("ImagePath_FullPhoto"));
map.put("Rating", (String)c.getString("Rating"));
MyArrList.add(map);
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
protected void onPostExecute(Void unused) {
ShowAllContent(); // When Finish Show Content
dismissDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
removeDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
}
}
// get Http Post
public String getHttpPost(String url,List<NameValuePair> params) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
try {
httpPost.setEntity(new UrlEncodedFormEntity(params));
HttpResponse response = client.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Status OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
/*** Get JSON Code from URL ***/
public String getJSONUrl(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download file..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
/***** Get Image Resource from URL (Start) *****/
private static final String TAG = "Image";
private static final int IO_BUFFER_SIZE = 4 * 1024;
public static Bitmap loadBitmap(String url) {
Bitmap bitmap = null;
InputStream in = null;
BufferedOutputStream out = null;
try {
in = new BufferedInputStream(new URL(url).openStream(), IO_BUFFER_SIZE);
final ByteArrayOutputStream dataStream = new ByteArrayOutputStream();
out = new BufferedOutputStream(dataStream, IO_BUFFER_SIZE);
copy(in, out);
out.flush();
final byte[] data = dataStream.toByteArray();
BitmapFactory.Options options = new BitmapFactory.Options();
//options.inSampleSize = 1;
bitmap = BitmapFactory.decodeByteArray(data, 0, data.length,options);
} catch (IOException e) {
Log.e(TAG, "Could not load Bitmap from: " + url);
} finally {
closeStream(in);
closeStream(out);
}
return bitmap;
}
private static void closeStream(Closeable stream) {
if (stream != null) {
try {
stream.close();
} catch (IOException e) {
android.util.Log.e(TAG, "Could not close stream", e);
}
}
}
private static void copy(InputStream in, OutputStream out) throws IOException {
byte[] b = new byte[IO_BUFFER_SIZE];
int read;
while ((read = in.read(b)) != -1) {
out.write(b, 0, read);
}
}
/***** Get Image Resource from URL (End) *****/
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
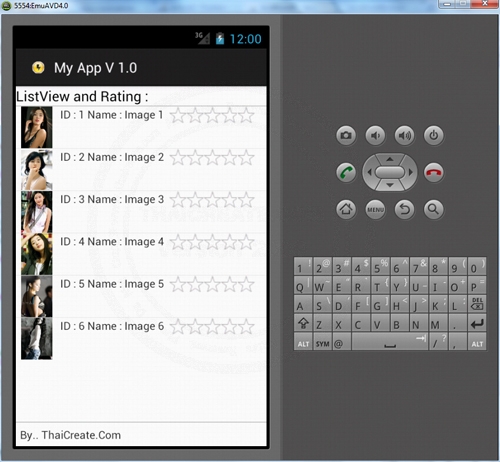
แสดงข้อมูลบน ListView
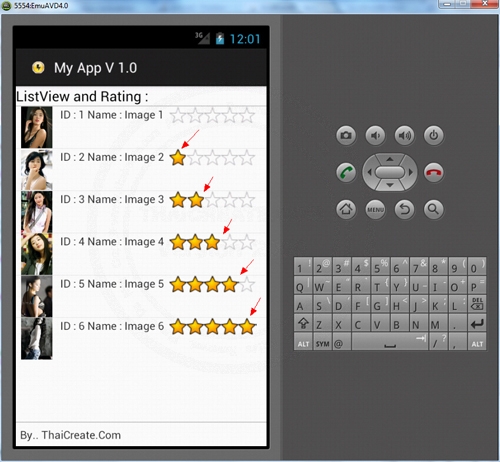
สามารถ Drag หรือ Click ที่ RatingBar เพื่อ Vote ข้อมูลในรายการ Item นั้น ๆ และสามารถ คลิกรายการอื่น ๆ ได้เลยโดยไม่ต้องรอให้ข้อมูล Update เสร็จ
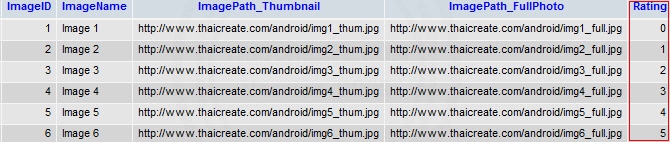
เมื่อตรวจสอบที่ฝั่ง Web Server จะเห็นว่ารายการได้ถูก Update
เพิ่มเติมจาก Example 1.1
ในกรณีที่ทำการโหวตในเวลาติด ๆ กัน ซึ่งจะเกิด Request ซ้ำ ๆ กัน แนะนำให้ใช้พวก Thread หรือ AsyncTask เข้ามาจัดการ Process เหล่านี้ ซึ่งจะทำให้โปรแกรมไม่ค้างในขณะทำงาน
Android Thread and Handler
Android AsyncTask and ProgressBar
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-08-26 12:21:29 /
2017-03-26 22:37:49 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|
|