Android SeekBar Control Audio Sound Volume (AudioManager) |
Android SeekBar Control Audio Sound Volume (AudioManager) ตัวอย่างการใช้ SeekBar ในการแสดงสถานะของ Audio Sound Volume หรือความดังของลำโพงบนเครื่อง Smartphone หรือ Tablets โดยจะใช้ SeekBar แสดงตำแหน่ง และสามารถใช้ SeekBar ทำการเพิ่มหรือลดเสียงของลำโพง
ในการเขียน Android เพื่อควบคุม Audio Sound จะใช้ class ของ AudioManager และ AUDIO_SERVICE
AudioManager audioManager = (AudioManager) getSystemService(Context.AUDIO_SERVICE);
// audioManager.getStreamVolume(AudioManager.STREAM_MUSIC)) // Sound Volume
โดยใช้คำสั่งง่าย ๆ ก็สามารถเรียกค่าของ Sound Volume มาแสดงได้
Example 1 แสดง Audio Sound Volume บน SeekBar แบบง่าย ๆ
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml
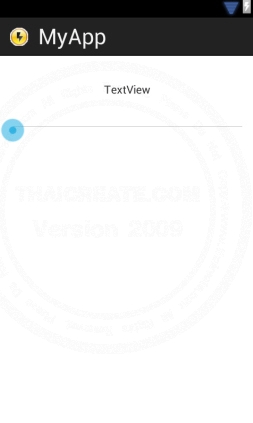
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="34dp"
android:text="TextView" />
<SeekBar
android:id="@+id/seekBar2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_below="@+id/textView1"
android:layout_marginTop="26dp" />
</RelativeLayout>
MainActivity.java
package com.myapp;
import android.media.AudioManager;
import android.os.Bundle;
import android.app.Activity;
import android.content.Context;
import android.view.Menu;
import android.widget.SeekBar;
import android.widget.SeekBar.OnSeekBarChangeListener;
import android.widget.TextView;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// call method ValumnControls()
ValumnControls();
}
private void ValumnControls()
{
try
{
final TextView txtView = (TextView)findViewById(R.id.textView1); // TextView
SeekBar seekBar2 = (SeekBar)findViewById(R.id.seekBar2); // SeekBar
final AudioManager audioManager = (AudioManager) getSystemService(Context.AUDIO_SERVICE);
seekBar2.setMax(audioManager
.getStreamMaxVolume(AudioManager.STREAM_MUSIC));
seekBar2.setProgress(audioManager
.getStreamVolume(AudioManager.STREAM_MUSIC));
// TextView
txtView.setText(String.valueOf(audioManager
.getStreamVolume(AudioManager.STREAM_MUSIC)));
seekBar2.setOnSeekBarChangeListener(new OnSeekBarChangeListener()
{
public void onStopTrackingTouch(SeekBar arg0)
{
}
public void onStartTrackingTouch(SeekBar arg0)
{
}
public void onProgressChanged(SeekBar arg0, int progress, boolean arg2)
{
audioManager.setStreamVolume(AudioManager.STREAM_MUSIC,
progress, 0);
txtView.setText(String.valueOf(progress));
}
});
}
catch (Exception e)
{
e.printStackTrace();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
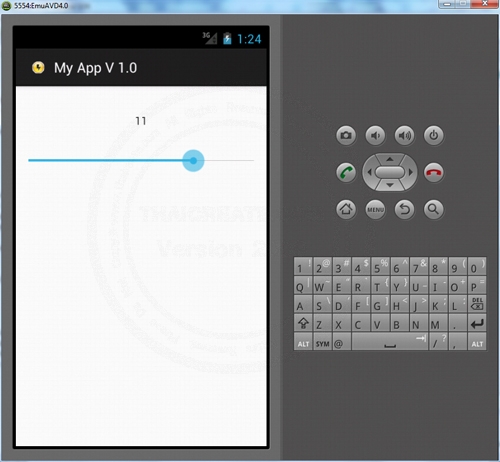
แสดง SeekBar และตำแหน่งของ Audio Sound Volume ซึ่งสามารถเลื่อน SeekBar เพื่อเพิ่มหรือลดเสียงได้
Example 2 ทดสอบใช้งาน Audio Sound Volume ร่วมกับการเล่นเพลง mp3
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml
สำหรับตัวอย่างการเช่นไฟล์ mp3 สามารถอ่านได้จาก 2 ตัวอย่างนี้
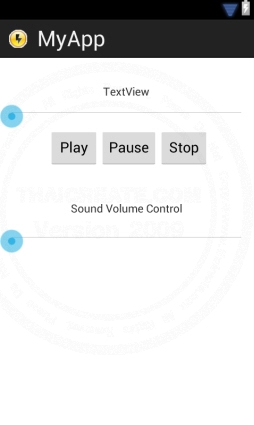
ออกแบบ XML Layout ดังภาพ
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginLeft="62dp"
android:layout_marginTop="89dp"
android:text="Play" />
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="34dp"
android:text="TextView" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/button1"
android:layout_alignBottom="@+id/button1"
android:layout_toRightOf="@+id/button1"
android:text="Pause" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/button2"
android:layout_alignBottom="@+id/button2"
android:layout_toRightOf="@+id/button2"
android:text="Stop" />
<SeekBar
android:id="@+id/seekBar1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_above="@+id/button2"
android:layout_alignParentLeft="true" />
<SeekBar
android:id="@+id/seekBar2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_centerVertical="true" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@+id/seekBar2"
android:layout_centerHorizontal="true"
android:layout_marginBottom="16dp"
android:text="Sound Volume Control" />
</RelativeLayout>
MainActivity.java
package com.myapp;
import java.io.IOException;
import android.media.AudioManager;
import android.media.MediaPlayer;
import android.os.Bundle;
import android.os.Handler;
import android.app.Activity;
import android.content.Context;
import android.view.Menu;
import android.view.View;
import android.view.View.OnTouchListener;
import android.widget.Button;
import android.view.MotionEvent;
import android.widget.SeekBar;
import android.widget.SeekBar.OnSeekBarChangeListener;
import android.widget.TextView;
public class MainActivity extends Activity {
private MediaPlayer mMedia;
private Handler handler = new Handler();
private SeekBar seekBar1;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// call method ValumnControls()
ValumnControls();
if(mMedia != null){
mMedia.release();
}
final TextView txtView = (TextView)findViewById(R.id.textView1);
txtView.setText("Source : music.mp3");
/* Resource in R.
* mMedia = MediaPlayer.create(this, R.raw.music);
* mMedia.start();
*/
/*
* from DataSource
* mMedia = new MediaPlayer();
* mMedia.setDataSource("https://www.thaicreate.com/music/mymusic.mp3");
* mMedia.start();
*
*/
mMedia = MediaPlayer.create(this, R.raw.music);
// seekBar1 Control media
seekBar1 = (SeekBar)findViewById(R.id.seekBar1);
seekBar1.setMax(mMedia.getDuration());
seekBar1.setOnTouchListener(new OnTouchListener() {
public boolean onTouch(View v, MotionEvent event) {
UpdateseekChange(v);
return false;
}
});
final Button btn1 = (Button) findViewById(R.id.button1); // Start
final Button btn2 = (Button) findViewById(R.id.button2); // Pause
final Button btn3 = (Button) findViewById(R.id.button3); // Stop
// Start
btn1.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
txtView.setText("Playing : music.mp3....");
mMedia.start();
startPlayProgressUpdater();
btn1.setEnabled(false);
btn2.setEnabled(true);
btn3.setEnabled(true);
}
});
// Pause
btn2.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
txtView.setText("Pause : music.mp3");
mMedia.pause();
btn1.setEnabled(true);
btn2.setEnabled(false);
btn3.setEnabled(false);
}
});
// Stop
btn3.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
txtView.setText("Stop Play : music.mp3");
mMedia.stop();
btn1.setEnabled(true);
btn2.setEnabled(false);
btn3.setEnabled(false);
try {
mMedia.prepare();
mMedia.seekTo(0);
} catch (IllegalStateException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
});
}
private void UpdateseekChange(View v){
if(mMedia.isPlaying()){
SeekBar sb = (SeekBar)v;
mMedia.seekTo(sb.getProgress());
}
}
public void startPlayProgressUpdater() {
seekBar1.setProgress(mMedia.getCurrentPosition());
if (mMedia.isPlaying()) {
Runnable notification = new Runnable() {
public void run() {
startPlayProgressUpdater();
}
};
handler.postDelayed(notification,1000);
}
}
@Override
protected void onDestroy() {
// TODO Auto-generated method stub
super.onDestroy();
if(mMedia != null){
mMedia.release();
}
}
private void ValumnControls()
{
try
{
SeekBar seekBar2 = (SeekBar)findViewById(R.id.seekBar2);
final AudioManager audioManager = (AudioManager) getSystemService(Context.AUDIO_SERVICE);
seekBar2.setMax(audioManager
.getStreamMaxVolume(AudioManager.STREAM_MUSIC));
seekBar2.setProgress(audioManager
.getStreamVolume(AudioManager.STREAM_MUSIC));
seekBar2.setOnSeekBarChangeListener(new OnSeekBarChangeListener()
{
public void onStopTrackingTouch(SeekBar arg0)
{
}
public void onStartTrackingTouch(SeekBar arg0)
{
}
public void onProgressChanged(SeekBar arg0, int progress, boolean arg2)
{
audioManager.setStreamVolume(AudioManager.STREAM_MUSIC,
progress, 0);
}
});
}
catch (Exception e)
{
e.printStackTrace();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}

Screenshot
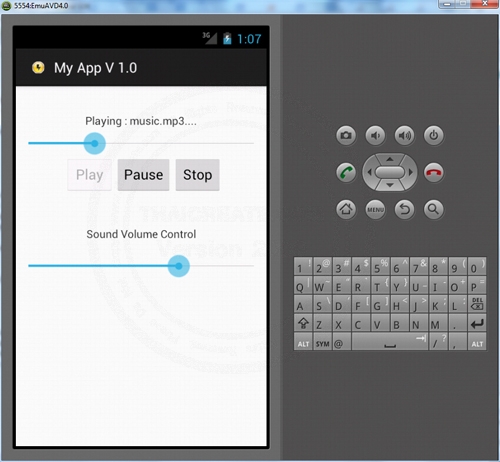
ทดสอบการใช้งานจริงร่วมกับการเล่นเพลง mp3
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2012-08-25 15:47:51 /
2017-03-26 22:41:58 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|