Android Shared Preferences (Session) : Create key/value in Application |
Android Shared Preferences (Session) : Create key/value in Application ในการใช้งาน Application บน Android ในกรณีที่ต้องการเก็บค่าตัวแปรเพื่อไปใช้งานใน Activity ต่าง ๆ นั้นเราจะใช้ Shared Preferences เป็น Class ที่ทำหน้าที่เหมือนการใช้งาน Session บน Web Application โดยความสามารถของมันคือ เมื่อเรามีการสร้างค่าตัวแปรใน App ที่ Activity ใด Activity หนึ่ง ตัวแปรนั้นจะสามารถเรียกใช้งานได้ในทุก ๆ ส่วนของ Application คล้าย ๆ กับ Session ของ PHP หรือ ASP.Net และเมื่อทำการปิด App นั้นไปแล้ว ค่าตัวแปรที่ถูกสร้างก็จะหายไปด้วย
ในการใช้งาน Shared Preferences ตัวแปรที่ถูกสร้างนั้นจะอยู่ในรูปแบบของ Key และ Value โดยสามารถสร้างได้หลาย ๆ Key ใน Shared Preferences ที่ถูกสร้างแต่ล่ะครั้ง ซึ่งนิยมนำมาเก็บค่าที่จำเป็นต้องใช้ใน App เช่น UserID หรือ Username
Android Shared Preferences (Session)
การสร้างตัวแปร Shared Preferences
public void createSession(String sUserName,String sPassword) {
editor.putBoolean("LoginStatus", true);
editor.putString("Username", sUserName);
editor.putString("Password", sPassword);
editor.commit();
}
การอ่านค่าตัวแปร Shared Preferences
public boolean getLoginStatus() {
return sharedPerfs.getBoolean("LoginStatus", false);
}
public String getUserName() {
return sharedPerfs.getString("Username", null);
}
public String getPassword() {
return sharedPerfs.getString("Password", null);
}
การลบค่าตัวแปร Shared Preferences
public void deleteSession() {
editor.clear();
editor.commit();
}
Example การสร้างตัวแปรแบบ Shared Preferences เพื่อเก็บค่า Username และ Password และนำไปใช้งานใน Activity อื่น ๆ
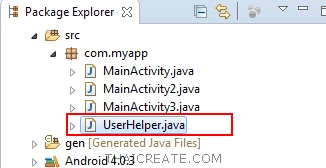
เราจะใช้การสร้าง Class ชื่อว่า UserHelper.java ซึ่งจะเป็น Class ที่ใช้สำหรับการจัดการกับตัวแปร SharedPreferences
UserHelper.java
package com.myapp;
import android.content.Context;
import android.content.SharedPreferences;
import android.content.SharedPreferences.Editor;
public class UserHelper {
Context context;
SharedPreferences sharedPerfs;
Editor editor;
// Prefs Keys
static String perfsName = "UserHelper";
static int perfsMode = 0;
public UserHelper(Context context) {
this.context = context;
this.sharedPerfs = this.context.getSharedPreferences(perfsName, perfsMode);
this.editor = sharedPerfs.edit();
}
public void createSession(String sUserName,String sPassword) {
editor.putBoolean("LoginStatus", true);
editor.putString("Username", sUserName);
editor.putString("Password", sPassword);
editor.commit();
}
public void deleteSession() {
editor.clear();
editor.commit();
}
public boolean getLoginStatus() {
return sharedPerfs.getBoolean("LoginStatus", false);
}
public String getUserName() {
return sharedPerfs.getString("Username", null);
}
public String getPassword() {
return sharedPerfs.getString("Password", null);
}
}
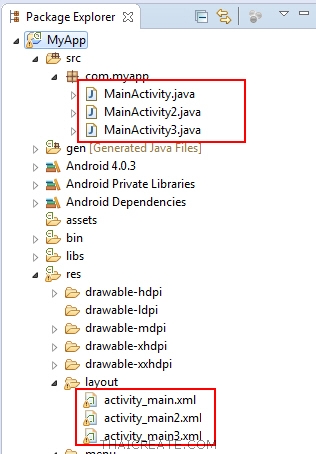
โครงสร้างของไฟล์ในโปรเจคสามารถใช้ได้ทั้งบน Eclipse และ Android Studio
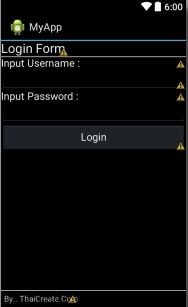
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Login Form "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Username :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" >
</EditText>
<TextView
android:id="@+id/textView4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Password :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10"
android:inputType="textPassword" >
</EditText>
<Button
android:id="@+id/btnLogin"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Login" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
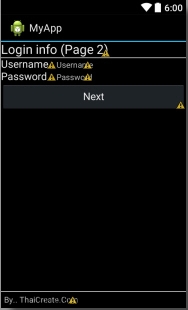
activity_main2.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Login info (Page 2) "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
</TableRow>
<Button
android:id="@+id/btnNext"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Next" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
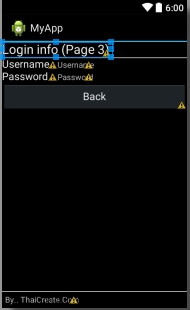
activity_main3.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Login info (Page 3) "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
</TableRow>
<Button
android:id="@+id/btnBack"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Back" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
ไฟล์ Java
MainActivity.java
package com.myapp;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//*** Session Login
final UserHelper usrHelper = new UserHelper(this);
//*** txtUsername & txtPassword
final EditText txtUser = (EditText)findViewById(R.id.txtUsername);
final EditText txtPass = (EditText)findViewById(R.id.txtPassword);
//*** Login Button
final Button btnLogin = (Button) findViewById(R.id.btnLogin);
btnLogin.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Create Session
usrHelper.createSession(txtUser.getText().toString(),txtPass.getText().toString());
// Goto Activity2
Intent newActivity = new Intent(MainActivity.this,MainActivity2.class);
startActivity(newActivity);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
MainActivity2.java
package com.myapp;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity2 extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main2);
//*** Get Session Login
final UserHelper usrHelper = new UserHelper(this);
//*** txtUsername & txtPassword
final TextView txtUser = (TextView)findViewById(R.id.txtUsername);
final TextView txtPass = (TextView)findViewById(R.id.txtPassword);
//*** Get Login Status
if(!usrHelper.getLoginStatus())
{
// Statement when not login
}
//*** Get Username & Password
txtUser.setText(usrHelper.getUserName());
txtPass.setText(usrHelper.getPassword());
//*** Button Next
final Button btnNext = (Button) findViewById(R.id.btnNext);
btnNext.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Goto Acitity2
Intent newActivity = new Intent(MainActivity2.this,MainActivity3.class);
startActivity(newActivity);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
MainActivity3.java
package com.myapp;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity3 extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main3);
//*** Get Session Login
final UserHelper usrHelper = new UserHelper(this);
//*** txtUsername & txtPassword
final TextView txtUser = (TextView)findViewById(R.id.txtUsername);
final TextView txtPass = (TextView)findViewById(R.id.txtPassword);
//*** Get Login Status
if(!usrHelper.getLoginStatus())
{
// Statement when not login
}
//*** Get Username & Password
txtUser.setText(usrHelper.getUserName());
txtPass.setText(usrHelper.getPassword());
//*** Button Back
final Button btnBack = (Button) findViewById(R.id.btnBack);
btnBack.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Goto Activity2
Intent newActivity = new Intent(MainActivity3.this,MainActivity2.class);
startActivity(newActivity);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
AndroidManifest.xml
<activity
android:name=".MainActivity2"
android:label="@string/app_name" />
<activity
android:name=".MainActivity3"
android:label="@string/app_name" />
Screenshot
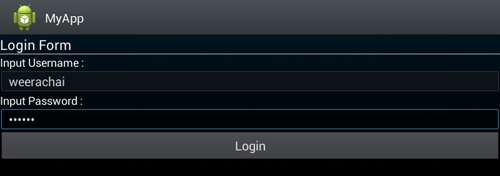
หน้า Login กรอก Username และ Password
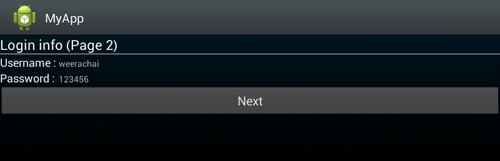
ในหน้าอื่น ๆ จะสามารถเรียกค่าตัวแปร Username และ Password ได้
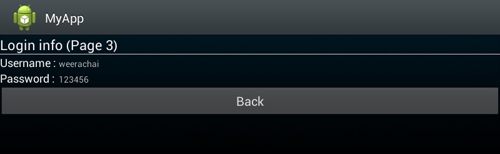
ในหน้าอื่น ๆ จะสามารถเรียกค่าตัวแปร Username และ Password ได้
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2015-11-16 15:53:40 /
2017-03-26 21:05:21 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|