Android Spinner / DropDownList from PHP and MySQL (Web Server) |
Android Spinner / DropDownList from PHP and MySQL (Web Server) ตัวอย่างการเขียน Android กับ Spinner หรือ DropDownList เพื่ออ่าน JSON Code ที่อยู่ในรูปแบบของ URL Website จากแหล่งต่าง ๆ โดย Android จะใช้ HttpPost และ HttpGet ในการอ่านข้อความ JSON ที่ถูกส่งมาพร้อมกับ URL ที่ได้ทำการ Request ไปที่ Web Server ผ่าน HTTP Method จากนั้นเมื่อได้ค่า JSON แล้วก็จะทำการแปลงข้อความ JSON ที่ได้ให้อยู่ในรูปแบบของ Array และนำ Array ที่ได้แสดงผลข้อมูลบน Spinner หรือ DropDownList
Basic Android and JSON
รูปอธิบายการทำงานระหว่าง Android กับ JSON Code ที่อยู่บน Internet Web Server
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Example การอ่าน JSON จาก URL (PHP กับ MySQL) และแสดงผลบน Spinner แบบง่าย ๆ
Web Server (PHP and MySQL)
member
CREATE TABLE `member` (
`MemberID` int(2) NOT NULL,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
PRIMARY KEY (`MemberID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'Weerachai', '0819876107');
INSERT INTO `member` VALUES (2, 'Win', '021978032');
INSERT INTO `member` VALUES (3, 'Eak', '0876543210');
โครงสร้างของข้อมูล MySQL Database
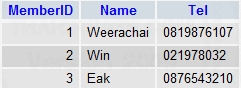
getJSON.php
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM member WHERE 1 ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
JSON Result
[{"MemberID":"1","Name":"Weerachai","Tel":"0819876107"},{"MemberID":"2","Name":"Win","Tel":"021978032"},{"MemberID":"3","Name":"Eak","Tel":"0876543210"}]
Android Project
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
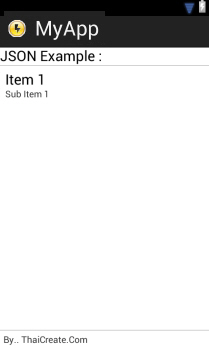
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="JSON Example : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<Spinner
android:id="@+id/spinner1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
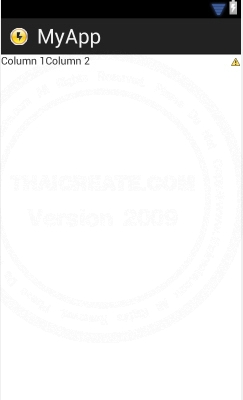
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColMemberID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="MemberID"/>
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="Name"/>
<TextView
android:id="@+id/ColTel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Tel" />
</LinearLayout>

MainActivity.java
package com.myapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.Bundle;
import android.os.StrictMode;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemSelectedListener;
import android.widget.SimpleAdapter;
import android.widget.Spinner;
import android.widget.Toast;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// spinner1
final Spinner spin = (Spinner)findViewById(R.id.spinner1);
/** JSON return
* [{"MemberID":"1","Name":"Weerachai","Tel":"0819876107"},
* {"MemberID":"2","Name":"Win","Tel":"021978032"},
* {"MemberID":"3","Name":"Eak","Tel":"0876543210"}]
*/
String url = "https://www.thaicreate.com/android/getJSON.php";
try {
JSONArray data = new JSONArray(getJSONUrl(url));
final ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("MemberID", c.getString("MemberID"));
map.put("Name", c.getString("Name"));
map.put("Tel", c.getString("Tel"));
MyArrList.add(map);
}
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(MainActivity.this, MyArrList, R.layout.activity_column,
new String[] {"MemberID", "Name", "Tel"}, new int[] {R.id.ColMemberID, R.id.ColName, R.id.ColTel});
spin.setAdapter(sAdap);
final AlertDialog.Builder viewDetail = new AlertDialog.Builder(this);
spin.setOnItemSelectedListener(new OnItemSelectedListener() {
public void onItemSelected(AdapterView<?> arg0, View selectedItemView,
int position, long id) {
String sMemberID = MyArrList.get(position).get("MemberID")
.toString();
String sName = MyArrList.get(position).get("Name")
.toString();
String sTel = MyArrList.get(position).get("Tel")
.toString();
//String sMemberID = ((TextView) myView.findViewById(R.id.ColMemberID)).getText().toString();
// String sName = ((TextView) myView.findViewById(R.id.ColName)).getText().toString();
// String sTel = ((TextView) myView.findViewById(R.id.ColTel)).getText().toString();
viewDetail.setIcon(android.R.drawable.btn_star_big_on);
viewDetail.setTitle("Member Detail");
viewDetail.setMessage("MemberID : " + sMemberID + "\n"
+ "Name : " + sName + "\n" + "Tel : " + sTel);
viewDetail.setPositiveButton("OK",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
// TODO Auto-generated method stub
dialog.dismiss();
}
});
viewDetail.show();
}
public void onNothingSelected(AdapterView<?> arg0) {
// TODO Auto-generated method stub
Toast.makeText(MainActivity.this,
"Your Selected : Nothing",
Toast.LENGTH_SHORT).show();
}
});
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public String getJSONUrl(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
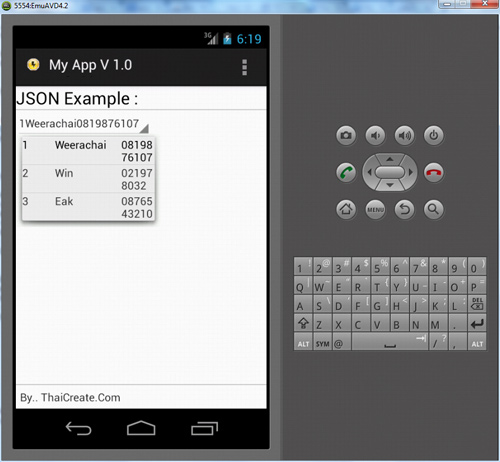
แสดงค่า JSON จาก URL (PHP กับ MySQL) บน Spinner / DropDownList
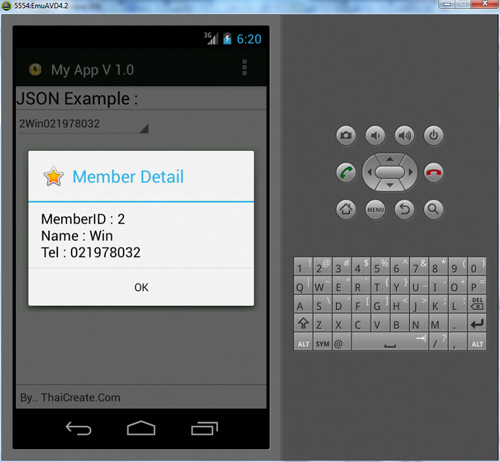
แสดงรายละเอียดของข้อมูลในแต่ล่ะรายการของ JSON Code ที่แสดงผลบน Spinner
Android HttpGet and HttpPost
Basic Android and JSON
Basic Android and Web Service
|