Android Delete Rows Data in SQLite Database (Android SQLite) |
Android Delete Rows Data in SQLite Database (Android SQLite) การเขียน Android เพื่อติดต่อกับฐานข้อมูลของ SQLite Database เพื่อทำการลบ Delete ข้อมูลบน SQLite ด้วย Android โดยใช้ class ของ SQLiteOpenHelper และ SQLiteDatabase ในการออกแบบบทความนี้ จะ Activity ประกอบด้วย 2 Form คือ Main Activity และ Delete List มี Popup เมนูสำหรับ Delete รายการ ดูรูปภาพประกอบ
พื้นฐานของ SQLite Database กับ Android สามารถศึกษาได้จากบทความนี้
Flow การทำงาน
ใน Main Activity จะสร้างปุ่ม Button Delete สำหรับคลิกไปยัง Activity ที่เป็น Activity Delete List โดยใช้การวิธี Intent Activity ใน Activity นี้จะแสดงข้อมูลทั้งหมดโดยใช้ ListView และเมื่อคลิกค้าง (Long Click - onLongPress) ในแต่ล่ะแถวก็จะมี Context Menu ที่เป็น Popup Dialog เพื่อเลือกรายการที่จะลบข้อมูล
Context Dialog Popup Menu สำหรับเลือก Delete ข้อมูล
โครงสร้างของ Table และ Data
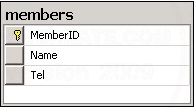
ชื่อว่าตาราง members ประกอบด้วยฟิวด์ MemberID, Name , Tel
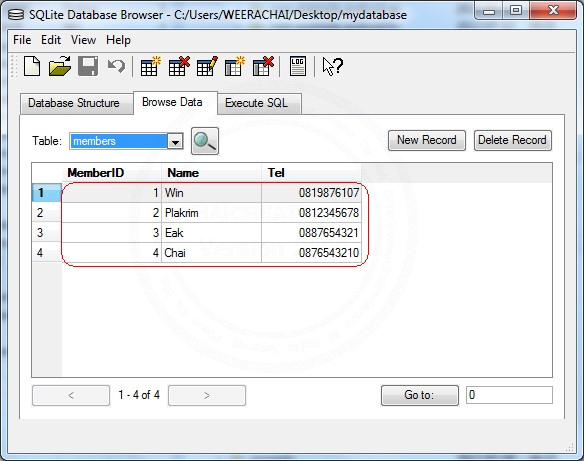
มีข้อมูลอยู่ 4 รายการ
โครงสร้างของ File
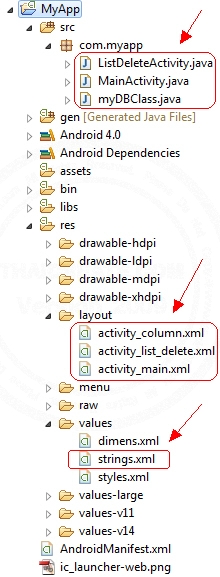
โครงสร้างของไฟล์ประกอบด้วย
- myDBClass.java (เป็น class สำหรับติดต่อกับฐานข้อมูล และบันทึกข้อมูล)
- MainActivity.java และ activity_main.xml (ไฟล์ Activity หลักสำหรับสร้าง Button คลิกไปยัง Activity Delete List)
- ListDeleteActivity.java และ activity_list_delete.xml (ไฟล์ activity ที่เป็น Delete List)
- activity_column.xmll (ไฟล์ XML layout Column ของ Activity Delete List)
- strings.xml (ไฟล์ XML ที่เก็บ Array ของ Menu จัดเก็บไว้ใน /res/values/string.xml)
รายละเอียดของไฟล์
myDBClass.java
package com.myapp;
import java.util.ArrayList;
import java.util.HashMap;
import android.content.Context;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.util.Log;
public class myDBClass extends SQLiteOpenHelper {
// Database Version
private static final int DATABASE_VERSION = 1;
// Database Name
private static final String DATABASE_NAME = "mydatabase";
// Table Name
private static final String TABLE_MEMBER = "members";
public myDBClass(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
// TODO Auto-generated constructor stub
}
@Override
public void onCreate(SQLiteDatabase db) {
// TODO Auto-generated method stub
// Create Table Name
db.execSQL("CREATE TABLE " + TABLE_MEMBER +
"(MemberID INTEGER PRIMARY KEY AUTOINCREMENT," +
" Name TEXT(100)," +
" Tel TEXT(100));");
Log.d("CREATE TABLE","Create Table Successfully.");
}
// Delete Data
public long DeleteData(String strMemberID) {
// TODO Auto-generated method stub
try {
SQLiteDatabase db;
db = this.getWritableDatabase(); // Write Data
/**
* for API 11 and above
SQLiteStatement insertCmd;
String strSQL = "DELETE FROM " + TABLE_MEMBER
+ " WHERE MemberID = ? ";
insertCmd = db.compileStatement(strSQL);
insertCmd.bindString(1, strMemberID);
return insertCmd.executeUpdateDelete();
*
*/
long rows = db.delete(TABLE_MEMBER, "MemberID = ?",
new String[] { String.valueOf(strMemberID) });
db.close();
return rows; // return rows deleted.
} catch (Exception e) {
return -1;
}
}
// Show All Data
public ArrayList<HashMap<String, String>> SelectAllData() {
// TODO Auto-generated method stub
try {
ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
SQLiteDatabase db;
db = this.getReadableDatabase(); // Read Data
String strSQL = "SELECT * FROM " + TABLE_MEMBER;
Cursor cursor = db.rawQuery(strSQL, null);
if(cursor != null)
{
if (cursor.moveToFirst()) {
do {
map = new HashMap<String, String>();
map.put("MemberID", cursor.getString(0));
map.put("Name", cursor.getString(1));
map.put("Tel", cursor.getString(2));
MyArrList.add(map);
} while (cursor.moveToNext());
}
}
cursor.close();
db.close();
return MyArrList;
} catch (Exception e) {
return null;
}
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
// TODO Auto-generated method stub
db.execSQL("DROP TABLE IF EXISTS " + TABLE_MEMBER);
// Re Create on method onCreate
onCreate(db);
}
}
ในไฟล์ของ class myDBClass จะมี method หลัก ๆ อยู่ 2 ตัวคือ SelectAllData() และ DeleteData() โดย
- SelectAllData() ใช้สำหรับการ แสดงข้อมูลรายการทั้งหมด
- DeleteData() ใช้สำหรับการลบข้อมูล
MainActivity.java และ activity_main.xml
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="35dp"
android:text="Main Menu"
android:textAppearance="?android:attr/textAppearanceLarge" />
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/textView1"
android:layout_centerHorizontal="true"
android:layout_marginTop="35dp"
android:text="Add" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/button1"
android:layout_centerInParent="true"
android:layout_marginTop="35dp"
android:text="Show" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/button2"
android:layout_centerInParent="true"
android:layout_marginTop="35dp"
android:text="Update" />
<Button
android:id="@+id/button4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/button3"
android:layout_centerInParent="true"
android:layout_marginTop="35dp"
android:text="Delete" />
</RelativeLayout>
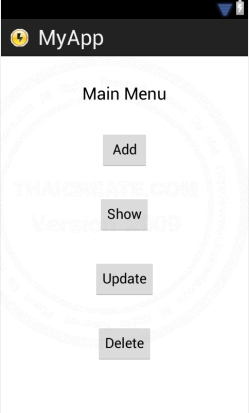
XML Layout ของ Activity หลัก
MainActivity.java
package com.myapp;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.app.Activity;
import android.content.Intent;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Button4 (Delete)
final Button btn4 = (Button) findViewById(R.id.button4);
// Perform action on click
btn4.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Open Form ListDelete
Intent newActivity = new Intent(MainActivity.this,ListDeleteActivity.class);
startActivity(newActivity);
}
});
}
}
ListDeleteActivity.java, activity_list_delete.xml และ activity_column.xml
activity_list_delete.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:gravity="center"
android:text="Delete Member : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:focusable="false"
android:focusableInTouchMode="false"
android:clickable="false">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<Button
android:id="@+id/btnCancel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Cancel" />
</LinearLayout>
</TableLayout>
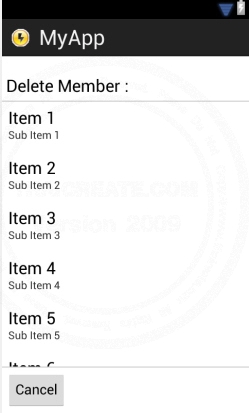
เป็น Layout ของ Activity ที่เป็น Delete List
string.xml (/res/values/)
<resources>
<string name="app_name">MyApp</string>
<string name="hello_world">Hello world!</string>
<string name="menu_settings">Settings</string>
<string name="title_activity_main">My App V 1.0</string>
<string-array name="CmdMenu">
<item>Edit</item>
<item>Delete</item>
</string-array>
</resources>
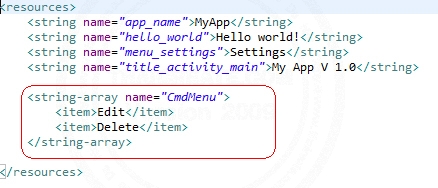
เป็นตัวแปร Array ที่ถุกจัดเก็บไว้ใน string.xml เพื่อจะนำไปสร้างเป็นเมนู
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColMemberID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="MemberID"/>
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="Name"/>
<TextView
android:id="@+id/ColTel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Tel" />
</LinearLayout>
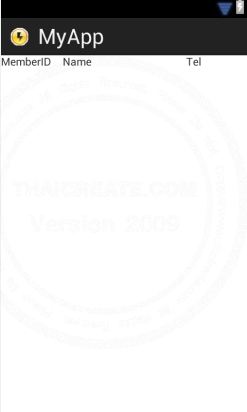
XML Layout ที่เป็น Custom Column ของ ListView
ListDeleteActivity.java
package com.myapp;
import java.util.ArrayList;
import java.util.HashMap;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.ContextMenu;
import android.view.ContextMenu.ContextMenuInfo;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.AdapterView;
import android.widget.Button;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import android.widget.Toast;
public class ListDeleteActivity extends Activity {
ArrayList<HashMap<String, String>> MebmerList;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_list_delete);
// Call Show List All Data
ShowListData();
// btnCancel (Cancel)
final Button cancel = (Button) findViewById(R.id.btnCancel);
cancel.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Open Form Main
Intent newActivity = new Intent(ListDeleteActivity.this,MainActivity.class);
startActivity(newActivity);
}
});
}
// Show List data
public void ShowListData()
{
myDBClass myDb = new myDBClass(this);
MebmerList = myDb.SelectAllData();
// listView1
ListView lisView1 = (ListView)findViewById(R.id.listView1);
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(ListDeleteActivity.this, MebmerList, R.layout.activity_column,
new String[] {"MemberID", "Name", "Tel"}, new int[] {R.id.ColMemberID, R.id.ColName, R.id.ColTel});
lisView1.setAdapter(sAdap);
registerForContextMenu(lisView1);
}
@Override
public void onCreateContextMenu(ContextMenu menu, View v,
ContextMenuInfo menuInfo) {
//if (v.getId()==R.id.list) {
AdapterView.AdapterContextMenuInfo info = (AdapterView.AdapterContextMenuInfo)menuInfo;
menu.setHeaderTitle("Command for : " + MebmerList.get(info.position).get("Name").toString());
String[] menuItems = getResources().getStringArray(R.array.CmdMenu);
for (int i = 0; i<menuItems.length; i++) {
menu.add(Menu.NONE, i, i, menuItems[i]);
}
//}
}
@Override
public boolean onContextItemSelected(MenuItem item) {
AdapterView.AdapterContextMenuInfo info = (AdapterView.AdapterContextMenuInfo)item.getMenuInfo();
int menuItemIndex = item.getItemId();
String[] menuItems = getResources().getStringArray(R.array.CmdMenu);
String CmdName = menuItems[menuItemIndex];
String MemID = MebmerList.get(info.position).get("MemberID").toString();
//String MemName = MebmerList.get(info.position).get("Name").toString();
// Check Event Command
if ("Edit".equals(CmdName)) {
// Show on new activity
//Intent newActivity = new Intent(ListDeleteActivity.this,UpdateActivity.class);
//newActivity.putExtra("MemID", MebmerList.get(info.position).get("ID").toString());
//startActivity(newActivity);
// for Delete Command
} else if ("Delete".equals(CmdName)) {
myDBClass myDb = new myDBClass(this);
long flg = myDb.DeleteData(MemID);
if(flg > 0)
{
Toast.makeText(ListDeleteActivity.this,"Delete Data Successfully",
Toast.LENGTH_LONG).show();
}
else
{
Toast.makeText(ListDeleteActivity.this,"Delete Data Failed.",
Toast.LENGTH_LONG).show();
}
// Call Show Data again
ShowListData();
}
return true;
}
}
คำสั่ง Java ใช้ในการอ่านข้อมูลจาก SQLite มาแสดงใน ListView และเมื่อคลิกค้าง (Long Click - onLongPress) ในแต่ล่ะรายการประมาณ 1 นาทีก็จะมี Context Menu แสดงเป็น Dialog Popup สำหรับการ Delete ข้อมูล
AndroidManifest.xml
<activity
android:name="ListDeleteActivity"
android:theme="@style/AppTheme"
android:screenOrientation="portrait"
android:label="@string/title_activity_main" />
เพิ่ม ListDeleteActivityลงในไฟล์ AndroidManifest.xml
Screenshot
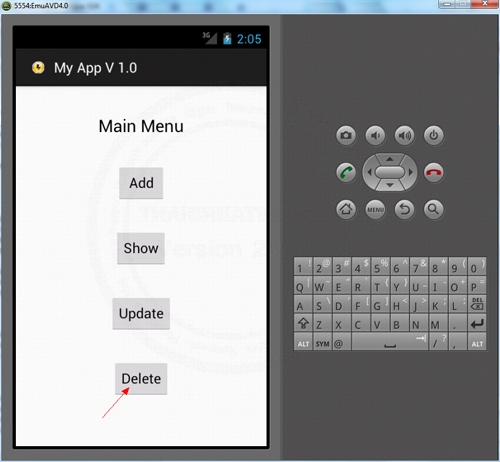
คลิกที่ Delete
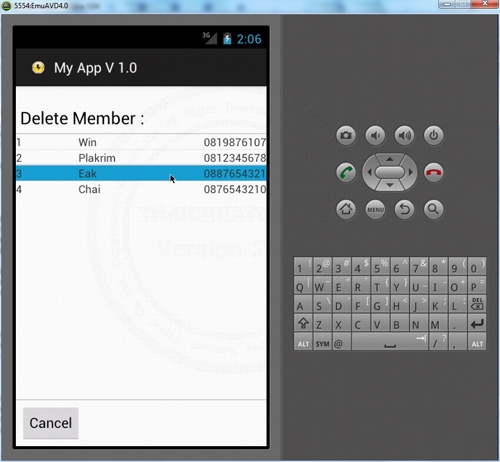
คลิกที่รายการที่ต้องการลบ โดยคลิกค้าง (Long Click - onLongPress) ประมาณ 1 วินาที
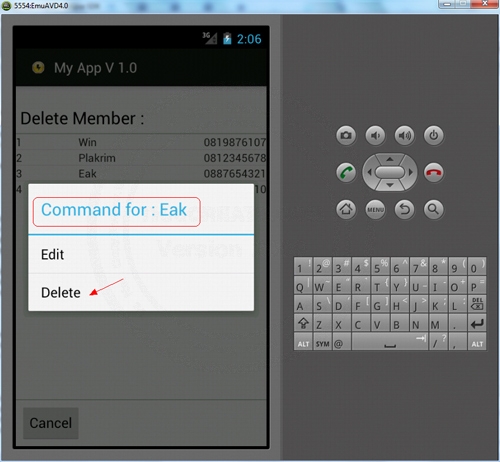
แสดงรายการ Context Dialog Menu สำหรับทำการราย Delete ข้อมูล
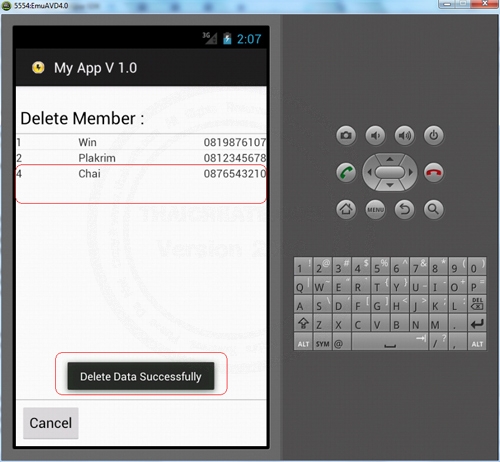
ข้อมูลได้ถูกลบไปแล้ว
จากตัวอย่างจะเห็นว่าจะมี Update Command สามารถเขียนคำสั่ง Intent เพื่อส่งค่าไปยัง Form Intent ได้เช่นเดียวกัน
if ("Edit".equals(CmdName)) {
// Show on new activity
Intent newActivity = new Intent(ListDeleteActivity.this,UpdateActivity.class);
newActivity.putExtra("MemID", MebmerList.get(info.position).get("ID").toString());
startActivity(newActivity);
}
ดูตัวอย่างการ Update ข้อมูลได้จาก
Android Edit Update Data in SQLite Database (Android SQLite)

Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2012-07-17 17:38:44 /
2012-07-22 07:18:59 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|