Android Spinner and SQLite Database (Android SQLite) |
Android Spinner and SQLite Database (Android SQLite) การเขียน Android กับ Widgets ของ Spinner หรือ DropDownList เพื่อติดต่อกับฐานข้อมูลของ SQLite Database เพื่อนำข้อมูลของ SQLite Databaseที่ได้แสดงข้อมูลใน Spinner (DropDownList )
พื้นฐานของ SQLite Database กับ Android สามารถศึกษาได้จากบทความนี้
Spinner - Android Widgets Example
โครงสร้างตารางและข้อมูล
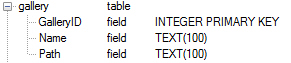
ชื่อตารางว่า gallery ประกอบด้วยฟิวด์ GalleryID, Name, Path
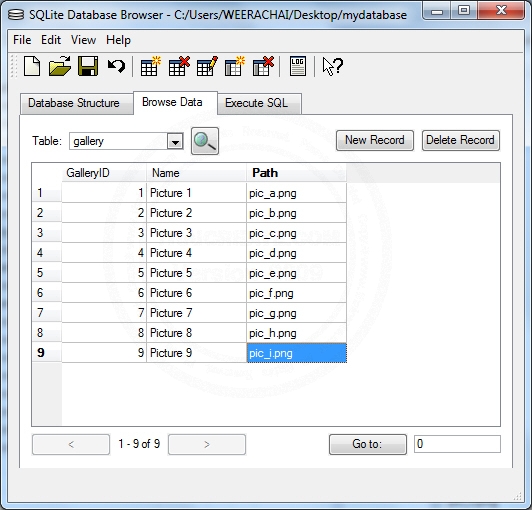
มีข้อมูลทั้งหมดอยู่ 9 รายการ
Example 1 อ่านข้อมูลจาก SQLite Database แบบง่าย ๆ มาแสดงที่ Spinner
ออกแบบหน้าจอ GraphicalLayout ตาม Layout ดังนี้
activity_main.xml (XML Layout)
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<Spinner
android:id="@+id/spinner1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</TableLayout>
XML Layout ของ Activity
myDBClass.java
package com.myapp;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.util.Log;
public class myDBClass extends SQLiteOpenHelper {
// Database Version
private static final int DATABASE_VERSION = 1;
// Database Name
private static final String DATABASE_NAME = "mydatabase";
// Table Name
private static final String TABLE_GALLERY = "gallery";
public myDBClass(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
// TODO Auto-generated constructor stub
}
@Override
public void onCreate(SQLiteDatabase db) {
// TODO Auto-generated method stub
// Create Table Name
db.execSQL("CREATE TABLE " + TABLE_GALLERY +
"(GalleryID INTEGER PRIMARY KEY AUTOINCREMENT," +
" Name TEXT(100)," +
" Path TEXT(100));");
Log.d("CREATE TABLE","Create Table Successfully.");
}
// Insert Data
public long InsertData(String strGalleryID, String strName, String strPath) {
// TODO Auto-generated method stub
try {
SQLiteDatabase db;
db = this.getWritableDatabase(); // Write Data
/**
* for API 11 and above
SQLiteStatement insertCmd;
String strSQL = "INSERT INTO " + TABLE_GALLERY
+ "(GalleryID,Name,Path) VALUES (?,?,?)";
insertCmd = db.compileStatement(strSQL);
insertCmd.bindString(1, strGalleryID);
insertCmd.bindString(2, strName);
insertCmd.bindString(3, strPath);
return insertCmd.executeInsert();
*/
ContentValues Val = new ContentValues();
Val.put("GalleryID", strGalleryID);
Val.put("Name", strName);
Val.put("Path", strPath);
long rows = db.insert(TABLE_GALLERY, null, Val);
db.close();
return rows; // return rows inserted.
} catch (Exception e) {
return -1;
}
}
// Select All Data
public Cursor SelectAllData() {
// TODO Auto-generated method stub
try {
SQLiteDatabase db;
db = this.getReadableDatabase(); // Read Data
String strSQL = "SELECT GalleryID As _id , * FROM " + TABLE_GALLERY;
Cursor cursor = db.rawQuery(strSQL, null);
return cursor;
} catch (Exception e) {
return null;
}
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
// TODO Auto-generated method stub
db.execSQL("DROP TABLE IF EXISTS " + TABLE_GALLERY);
// Re Create on method onCreate
onCreate(db);
}
}
class ของ myDBClass ใช้สำหรับการติดต่อกับฐานข้อมูล
MainActivity.java
package com.myapp;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemSelectedListener;
import android.widget.SimpleCursorAdapter;
import android.widget.Spinner;
import android.app.Activity;
import android.database.Cursor;
import android.widget.Toast;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// get Data from SQLite
final myDBClass myDb = new myDBClass(this);
/*
* for insert statement
myDb.InsertData("1","Picture 1", "pic_a.png");
myDb.InsertData("2","Picture 2", "pic_b.png");
myDb.InsertData("3","Picture 3", "pic_c.png");
myDb.InsertData("4","Picture 4", "pic_d.png");
myDb.InsertData("5","Picture 5", "pic_e.png");
myDb.InsertData("6","Picture 6", "pic_f.png");
myDb.InsertData("7","Picture 7", "pic_g.png");
myDb.InsertData("8","Picture 8", "pic_h.png");
myDb.InsertData("9","Picture 9", "pic_i.png");
*/
final Cursor myData = myDb.SelectAllData();
startManagingCursor(myData);
// spinner1
final Spinner spin = (Spinner)findViewById(R.id.spinner1);
String[] cols = new String[]{"Name"};
int[] names = new int[]{android.R.id.text1};
SimpleCursorAdapter adapter = new SimpleCursorAdapter(this, android.R.layout.simple_list_item_1 , myData ,cols,names);
spin.setAdapter(adapter);
spin.setOnItemSelectedListener(new OnItemSelectedListener() {
public void onItemSelected(AdapterView<?> arg0, View selectedItemView,
int position, long id) {
// TODO Auto-generated method stub
String ItemID = myData.getString(myData.getColumnIndex("Name"));
Toast.makeText(MainActivity.this,
"Your Selected : " + ItemID,
Toast.LENGTH_SHORT).show();
}
public void onNothingSelected(AdapterView<?> arg0) {
// TODO Auto-generated method stub
Toast.makeText(MainActivity.this,
"Your Selected : -",
Toast.LENGTH_SHORT).show();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
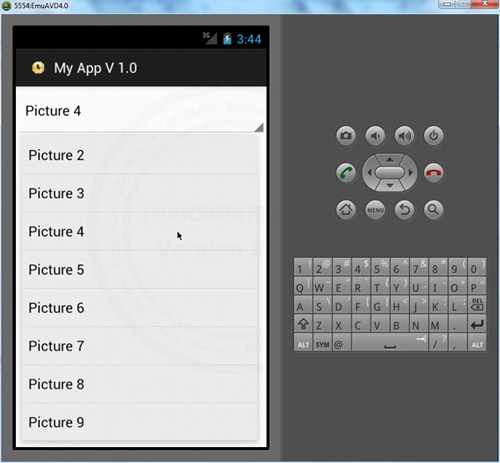
แสดง Spinner กับ SQLite Database
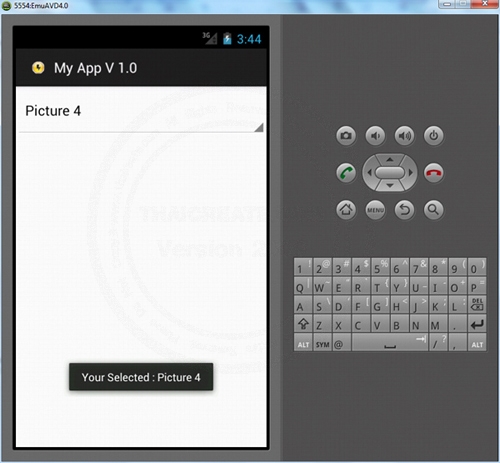
เมื่อเลือกรายการ
Example 2 การกำหนด Custom Layout โดยจะแบ่งหลาย Column อ่านข้อมูลมาจาก SQLite Database
ออกแบบหน้าจอ GraphicalLayout ตาม Layout ดังนี้
activity_main.xml (XML Layout)
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<Spinner
android:id="@+id/spinner1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</TableLayout>
XML Layout ของ Activity
custom_column.xml (XML Layout)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColGalleryID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="GalleryID"/>
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="Name"/>
<TextView
android:id="@+id/ColPatn"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Path" />
</LinearLayout>
Custom Layout ของ Spinner
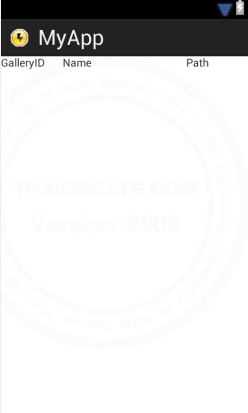
myDBClass.java
package com.myapp;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.util.Log;
public class myDBClass extends SQLiteOpenHelper {
// Database Version
private static final int DATABASE_VERSION = 1;
// Database Name
private static final String DATABASE_NAME = "mydatabase";
// Table Name
private static final String TABLE_GALLERY = "gallery";
public myDBClass(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
// TODO Auto-generated constructor stub
}
@Override
public void onCreate(SQLiteDatabase db) {
// TODO Auto-generated method stub
// Create Table Name
db.execSQL("CREATE TABLE " + TABLE_GALLERY +
"(GalleryID INTEGER PRIMARY KEY AUTOINCREMENT," +
" Name TEXT(100)," +
" Path TEXT(100));");
Log.d("CREATE TABLE","Create Table Successfully.");
}
// Insert Data
public long InsertData(String strGalleryID, String strName, String strPath) {
// TODO Auto-generated method stub
try {
SQLiteDatabase db;
db = this.getWritableDatabase(); // Write Data
/**
* for API 11 and above
SQLiteStatement insertCmd;
String strSQL = "INSERT INTO " + TABLE_GALLERY
+ "(GalleryID,Name,Path) VALUES (?,?,?)";
insertCmd = db.compileStatement(strSQL);
insertCmd.bindString(1, strGalleryID);
insertCmd.bindString(2, strName);
insertCmd.bindString(3, strPath);
return insertCmd.executeInsert();
*/
ContentValues Val = new ContentValues();
Val.put("GalleryID", strGalleryID);
Val.put("Name", strName);
Val.put("Path", strPath);
long rows = db.insert(TABLE_GALLERY, null, Val);
db.close();
return rows; // return rows inserted.
} catch (Exception e) {
return -1;
}
}
// Select All Data
public Cursor SelectAllData() {
// TODO Auto-generated method stub
try {
SQLiteDatabase db;
db = this.getReadableDatabase(); // Read Data
String strSQL = "SELECT GalleryID As _id , * FROM " + TABLE_GALLERY;
Cursor cursor = db.rawQuery(strSQL, null);
return cursor;
} catch (Exception e) {
return null;
}
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
// TODO Auto-generated method stub
db.execSQL("DROP TABLE IF EXISTS " + TABLE_GALLERY);
// Re Create on method onCreate
onCreate(db);
}
}
class ของ myDBClass ใช้สำหรับการติดต่อกับฐานข้อมูล
MainActivity.java
package com.myapp;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemSelectedListener;
import android.widget.SimpleCursorAdapter;
import android.widget.Spinner;
import android.app.Activity;
import android.database.Cursor;
import android.widget.Toast;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// get Data from SQLite
final myDBClass myDb = new myDBClass(this);
/*
* for insert statement
myDb.InsertData("1","Picture 1", "pic_a.png");
myDb.InsertData("2","Picture 2", "pic_b.png");
myDb.InsertData("3","Picture 3", "pic_c.png");
myDb.InsertData("4","Picture 4", "pic_d.png");
myDb.InsertData("5","Picture 5", "pic_e.png");
myDb.InsertData("6","Picture 6", "pic_f.png");
myDb.InsertData("7","Picture 7", "pic_g.png");
myDb.InsertData("8","Picture 8", "pic_h.png");
myDb.InsertData("9","Picture 9", "pic_i.png");
*/
final Cursor myData = myDb.SelectAllData();
startManagingCursor(myData);
// spinner1
final Spinner spin = (Spinner)findViewById(R.id.spinner1);
SimpleCursorAdapter adapter;
adapter = new SimpleCursorAdapter(MainActivity.this, R.layout.custom_column, myData
,new String[] {"GalleryID","Name","Path"}
,new int[] {R.id.ColGalleryID, R.id.ColName, R.id.ColPatn});
spin.setAdapter(adapter);
spin.setOnItemSelectedListener(new OnItemSelectedListener() {
public void onItemSelected(AdapterView<?> arg0, View selectedItemView,
int position, long id) {
// TODO Auto-generated method stub
String ItemID = myData.getString(myData.getColumnIndex("Name"));
Toast.makeText(MainActivity.this,
"Your Selected : " + ItemID,
Toast.LENGTH_SHORT).show();
}
public void onNothingSelected(AdapterView<?> arg0) {
// TODO Auto-generated method stub
Toast.makeText(MainActivity.this,
"Your Selected : -",
Toast.LENGTH_SHORT).show();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
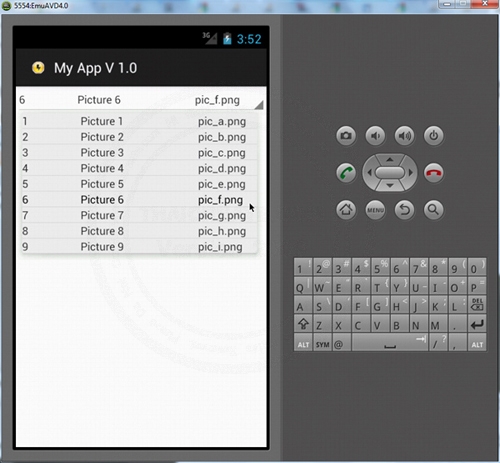
แสดง Spinner กับ SQLite Database โดยจะแสดง Column ตาม Layout ที่เป็น Custom layout
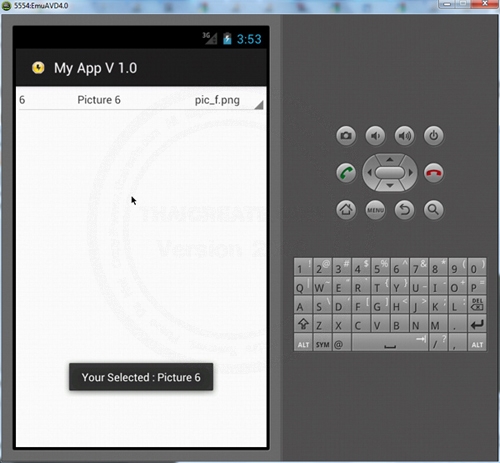
เมื่อเลือกรายการข้อมูล
Example 3 การกำหนด Custom Layout และการแสดงรูปภาพ (Image) ที่อยู่ใน SD Card
ในตัวอย่างนี้จะใช้ Spinner แสดงข้อมูลจาก SQLite ซึ่งจะมีรูปภาพ ที่ถูกจัดเก็บไว้ใน SD Card
Android อ่านข้อมูลรูปภาพแสดงใน ImageView จาก SD Card สดงแบบ Column บน GridView
โครงสร้าง Database และ รูปภาพที่จัดเก็บ
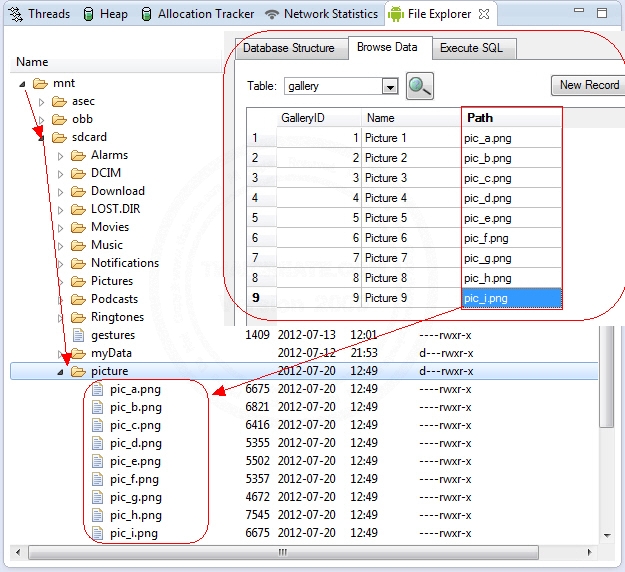
โดยรูปภาพเก็บไว้ที่ /mnt/sdcard/picture
ออกแบบหน้าจอ GraphicalLayout ตาม Layout ดังนี้
activity_main.xml (XML Layout)
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<Spinner
android:id="@+id/spinner1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</TableLayout>
XML Layout ของ Activity
custom_column.xml (XML Layout)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColGalleryID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="GalleryID"/>
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="Name"/>
<ImageView
android:id="@+id/ColPath"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
Custom Layout ของ Spinner
myDBClass.java
package com.myapp;
import java.util.ArrayList;
import java.util.HashMap;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.util.Log;
public class myDBClass extends SQLiteOpenHelper {
// Database Version
private static final int DATABASE_VERSION = 1;
// Database Name
private static final String DATABASE_NAME = "mydatabase";
// Table Name
private static final String TABLE_GALLERY = "gallery";
public myDBClass(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
// TODO Auto-generated constructor stub
}
@Override
public void onCreate(SQLiteDatabase db) {
// TODO Auto-generated method stub
// Create Table Name
db.execSQL("CREATE TABLE " + TABLE_GALLERY +
"(GalleryID INTEGER PRIMARY KEY AUTOINCREMENT," +
" Name TEXT(100)," +
" Path TEXT(100));");
Log.d("CREATE TABLE","Create Table Successfully.");
}
// Insert Data
public long InsertData(String strGalleryID, String strName, String strPath) {
// TODO Auto-generated method stub
try {
SQLiteDatabase db;
db = this.getWritableDatabase(); // Write Data
/**
* for API 11 and above
SQLiteStatement insertCmd;
String strSQL = "INSERT INTO " + TABLE_GALLERY
+ "(GalleryID,Name,Path) VALUES (?,?,?)";
insertCmd = db.compileStatement(strSQL);
insertCmd.bindString(1, strGalleryID);
insertCmd.bindString(2, strName);
insertCmd.bindString(3, strPath);
return insertCmd.executeInsert();
*/
ContentValues Val = new ContentValues();
Val.put("GalleryID", strGalleryID);
Val.put("Name", strName);
Val.put("Path", strPath);
long rows = db.insert(TABLE_GALLERY, null, Val);
db.close();
return rows; // return rows inserted.
} catch (Exception e) {
return -1;
}
}
// Show All Data
public ArrayList<HashMap<String, String>> SelectAllData() {
// TODO Auto-generated method stub
try {
ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
SQLiteDatabase db;
db = this.getReadableDatabase(); // Read Data
String strSQL = "SELECT * FROM " + TABLE_GALLERY;
Cursor cursor = db.rawQuery(strSQL, null);
if(cursor != null)
{
if (cursor.moveToFirst()) {
do {
map = new HashMap<String, String>();
map.put("GalleryID", cursor.getString(0));
map.put("Name", cursor.getString(1));
map.put("Path", cursor.getString(2));
MyArrList.add(map);
} while (cursor.moveToNext());
}
}
cursor.close();
db.close();
return MyArrList;
} catch (Exception e) {
return null;
}
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
// TODO Auto-generated method stub
db.execSQL("DROP TABLE IF EXISTS " + TABLE_GALLERY);
// Re Create on method onCreate
onCreate(db);
}
}
class ของ myDBClass ใช้สำหรับการติดต่อกับฐานข้อมูล
MainActivity.java
package com.myapp;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.view.ViewGroup;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemSelectedListener;
import android.widget.ImageView;
import android.widget.SimpleAdapter;
import android.widget.Spinner;
import android.widget.TextView;
import android.app.Activity;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.widget.Toast;
public class MainActivity extends Activity {
ArrayList<HashMap<String, String>> GalleryList;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// get Data from SQLite
final myDBClass myDb = new myDBClass(this);
/*
* for insert statement
myDb.InsertData("1","Picture 1", "pic_a.png");
myDb.InsertData("2","Picture 2", "pic_b.png");
myDb.InsertData("3","Picture 3", "pic_c.png");
myDb.InsertData("4","Picture 4", "pic_d.png");
myDb.InsertData("5","Picture 5", "pic_e.png");
myDb.InsertData("6","Picture 6", "pic_f.png");
myDb.InsertData("7","Picture 7", "pic_g.png");
myDb.InsertData("8","Picture 8", "pic_h.png");
myDb.InsertData("9","Picture 9", "pic_i.png");
*/
GalleryList = myDb.SelectAllData();
// spinner1
final Spinner spin = (Spinner)findViewById(R.id.spinner1);
myAdapter adapter = new myAdapter(getApplicationContext(), GalleryList,
R.layout.custom_column, new String[] { "GalleryID","Name", "Path" },
new int[] { R.id.ColGalleryID, R.id.ColName, R.id.ColPath });
spin.setAdapter(adapter);
spin.setOnItemSelectedListener(new OnItemSelectedListener() {
public void onItemSelected(AdapterView<?> arg0, View selectedItemView,
int position, long id) {
// TODO Auto-generated method stub
String ItemID = GalleryList.get(position).get("Name").toString();
Toast.makeText(getApplicationContext(),
"Your selected : " + ItemID, Toast.LENGTH_SHORT).show();
}
public void onNothingSelected(AdapterView<?> arg0) {
// TODO Auto-generated method stub
Toast.makeText(MainActivity.this,
"Your Selected : -",
Toast.LENGTH_SHORT).show();
}
});
}
private class myAdapter extends SimpleAdapter {
public myAdapter(Context context, List<? extends Map<String, ?>> data,
int resource, String[] from, int[] to) {
super(context, data, resource, from, to);
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = getLayoutInflater().inflate(R.layout.custom_column,
null);
}
@SuppressWarnings("unchecked")
HashMap<String, Object> data = (HashMap<String, Object>) getItem(position);
((TextView) convertView.findViewById(R.id.ColGalleryID))
.setText((String) data.get("GalleryID"));
((TextView) convertView.findViewById(R.id.ColName))
.setText((String) data.get("Name"));
String strPath = "/mnt/sdcard/picture/"+data.get("Path");
Bitmap bm = BitmapFactory.decodeFile(strPath);
((ImageView) convertView.findViewById(R.id.ColPath))
.setImageBitmap(bm);
return convertView;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
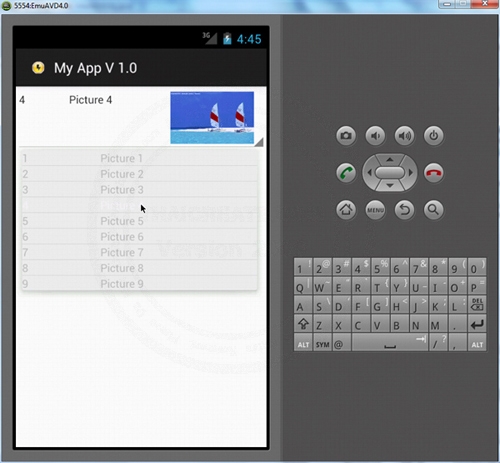
แสดง Spinner กับ SQLite Database แสดงรูปภาพเมื่อมีการเลือกรายการ
Property & Method (Others Related) |
|