Android Upload Send file to Web Server (Website) |
Android Upload Send file to Web Server (Website) การเขียน Android เพื่อทำการ Upload ไฟล์ไปยัง Server ใน Android นั้นสามารถทำได้ง่าย ๆ ด้วยการเชื่อมต่อกับ Web Server ผ่าน HttpURLConnection โดยกำหนด Content-Type เป็นแบบ Binary จากนั้นในฝั่งของ Web Server ก็สร้าง PHP ไว้สำหรับการรับค่าจาก Binary จาก Android แล้วบันทึกจัดเก็บไว้ใน Web Server ได้ง่าย ๆ
รูปอธิบกายขั้นตอนการทำงานและการอัพโหลดไฟล์ไปยัง Server ด้วย Android จะเห็นว่าในฝั่งของ Web Server จะใช้ PHP เข้ามาทำการ Save ไฟล์ที่ Upload จาก Android
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Web Server
uploadFile.php
<?php
if(@move_uploaded_file($_FILES["filUpload"]["tmp_name"],"myfile/".$_FILES["filUpload"]["name"]))
{
$arr["StatusID"] = "1";
$arr["Error"] = "";
}
else
{
$arr["StatusID"] = "0";
$arr["Error"] = "Error cannot upload file.";
}
echo json_encode($arr);
?>
ในฝั่งของ Web Server ออกแบบไฟล์ PHP ให้ Save ไฟล์ง่าย ๆ ด้วยคำสั่ง Copy โดย Save ลงในโฟเดอร์ myfile ของ Web Server เฉพาะฉะนั้นจะต้อง CHMOD 777 โฟเดอร์นี้ด้วย
Android Project
Example 1 การ Upload ไฟล์จาก Android ไปยัง Web Server ของ PHP แบบง่าย ๆ
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml
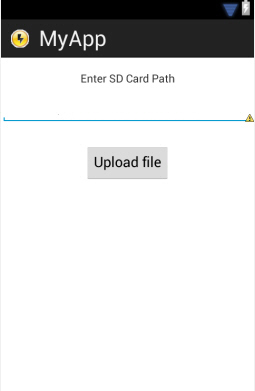
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/textView1"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginTop="18dp"
android:gravity="center"
android:text="Enter SD Card Path" />
<EditText
android:id="@+id/txtSDCard"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_below="@+id/textView1"
android:layout_marginTop="16dp"
android:ems="10"
android:gravity="center"
android:textSize="12dp" >
<requestFocus />
</EditText>
<Button
android:id="@+id/btnUpload"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/txtSDCard"
android:layout_centerHorizontal="true"
android:layout_marginTop="25dp"
android:text="Upload file" />
</RelativeLayout>
MainActivity.java
package com.myapp;
import java.io.ByteArrayOutputStream;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
final AlertDialog.Builder ad = new AlertDialog.Builder(this);
// txtSDCard
final EditText txtSDCard = (EditText)findViewById(R.id.txtSDCard);
// btnUpload
Button btnUpload = (Button)findViewById(R.id.btnUpload);
btnUpload.setOnClickListener(new OnClickListener(){
public void onClick(View v) {
// TODO Auto-generated stub
String strSDPath = txtSDCard.getText().toString();
String strUrlServer = "https://www.thaicreate.com/android/uploadFile.php";
String resServer = uploadFiletoServer(strSDPath,strUrlServer);
/** Get result from Server (Return the JSON Code)
* StatusID = ? [0=Failed,1=Complete]
* Error = ? [On case error return custom error message]
*
* Eg Upload Failed = {"StatusID":"0","Error":"Cannot Upload file!"}
* Eg Upload Complete = {"StatusID":"1","Error":""}
*/
/*** Default Value ***/
String strStatusID = "0";
String strError = "Unknow Status!";
try {
JSONObject c = new JSONObject(resServer);
strStatusID = c.getString("StatusID");
strError = c.getString("Error");
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
if(strStatusID.equals("0"))
{
ad.setTitle("Error!");
ad.setIcon(android.R.drawable.btn_star_big_on);
ad.setMessage(strError);
ad.setPositiveButton("Close", null);
ad.show();
}
else
{
Toast.makeText(MainActivity.this, "Upload file Successfully", Toast.LENGTH_SHORT).show();
}
}
});
}
public String uploadFiletoServer(String strSDPath, String strUrlServer)
{
int bytesRead, bytesAvailable, bufferSize;
byte[] buffer;
int maxBufferSize = 1 * 1024 * 1024;
int resCode = 0;
String resMessage = "";
String lineEnd = "\r\n";
String twoHyphens = "--";
String boundary = "*****";
try {
/** Check file on SD Card ***/
File file = new File(strSDPath);
if(!file.exists())
{
return "{\"StatusID\":\"0\",\"Error\":\"Please check path on SD Card\"}";
}
FileInputStream fileInputStream = new FileInputStream(new File(strSDPath));
URL url = new URL(strUrlServer);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoInput(true);
conn.setDoOutput(true);
conn.setUseCaches(false);
conn.setRequestMethod("POST");
conn.setRequestProperty("Connection", "Keep-Alive");
conn.setRequestProperty("Content-Type",
"multipart/form-data;boundary=" + boundary);
DataOutputStream outputStream = new DataOutputStream(conn
.getOutputStream());
outputStream.writeBytes(twoHyphens + boundary + lineEnd);
outputStream
.writeBytes("Content-Disposition: form-data; name=\"filUpload\";filename=\""
+ strSDPath + "\"" + lineEnd);
outputStream.writeBytes(lineEnd);
bytesAvailable = fileInputStream.available();
bufferSize = Math.min(bytesAvailable, maxBufferSize);
buffer = new byte[bufferSize];
// Read file
bytesRead = fileInputStream.read(buffer, 0, bufferSize);
while (bytesRead > 0) {
outputStream.write(buffer, 0, bufferSize);
bytesAvailable = fileInputStream.available();
bufferSize = Math.min(bytesAvailable, maxBufferSize);
bytesRead = fileInputStream.read(buffer, 0, bufferSize);
}
outputStream.writeBytes(lineEnd);
outputStream.writeBytes(twoHyphens + boundary + twoHyphens + lineEnd);
// Response Code and Message
resCode = conn.getResponseCode();
if(resCode == HttpURLConnection.HTTP_OK)
{
InputStream is = conn.getInputStream();
ByteArrayOutputStream bos = new ByteArrayOutputStream();
int read = 0;
while ((read = is.read()) != -1) {
bos.write(read);
}
byte[] result = bos.toByteArray();
bos.close();
resMessage = new String(result);
}
Log.d("resCode=",Integer.toString(resCode));
Log.d("resMessage=",resMessage.toString());
fileInputStream.close();
outputStream.flush();
outputStream.close();
return resMessage.toString();
} catch (Exception ex) {
// Exception handling
return null;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
คำอธิบาย
outputStream
.writeBytes("Content-Disposition: form-data; name=\"filUpload\";filename=\""
+ strSDPath + "\"" + lineEnd);
จาก Code ของ Java จะเห็นว่ามีกำการกำหนดชื่อเป็น filUpload เพราะฉะนั้นในฝั่งของ PHP จะรับเป็น
PHP
$_FILES["filUpload"];
(สามารถใช้ภาษาอื่น ๆ ได้เช่น ASP/ASP.NET หรือ JSP ก็ได้เช่นเดียวกัน)
Screenshot
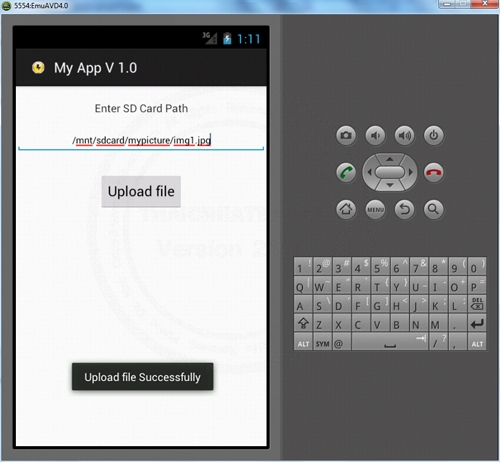
กรอก Path ของไฟล์ที่อยู่ใน SD Card และคลิก Upload file
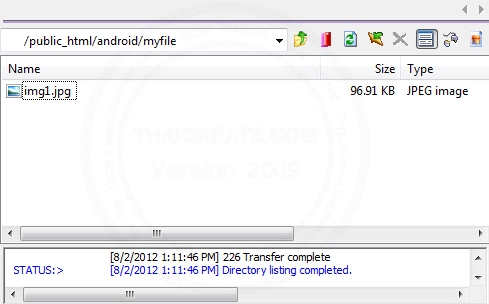
เมื่อตรวจสอบไฟล์บน Web Server ไฟล์ก็จะถูกอัพโหลดไปไว้ยังโฟเดอร์ที่ต้องการ
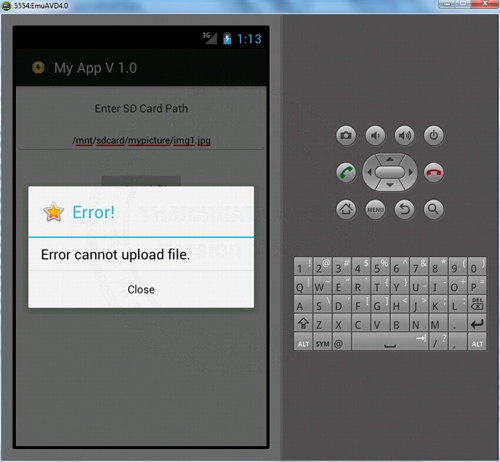
กรณีที่ไม่สามารถอัพโหลดไฟล์ได้
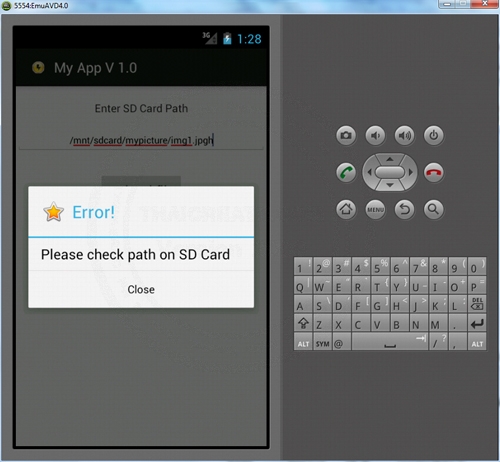
กรณีที่ Path ของ SD Card ไม่ถูกต้อง
Example 2 แสดง Progress Dialog ในขณะที่กำลังอัพไฟล์
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/textView1"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginTop="18dp"
android:gravity="center"
android:text="Enter SD Card Path" />
<EditText
android:id="@+id/txtSDCard"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_below="@+id/textView1"
android:layout_marginTop="16dp"
android:ems="10"
android:text="/mnt/sdcard/mypicture/img1.jpg"
android:gravity="center"
android:textSize="12dp" >
<requestFocus />
</EditText>
<Button
android:id="@+id/btnUpload"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/txtSDCard"
android:layout_centerHorizontal="true"
android:layout_marginTop="25dp"
android:text="Upload file" />
</RelativeLayout>
MainActivity.java
package com.myapp;
import java.io.ByteArrayOutputStream;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
public static final int DIALOG_UPLOAD_PROGRESS = 0;
private ProgressDialog mProgressDialog;
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// txtSDCard
final EditText txtSDCard = (EditText)findViewById(R.id.txtSDCard);
// btnUpload
Button btnUpload = (Button)findViewById(R.id.btnUpload);
btnUpload.setOnClickListener(new OnClickListener(){
public void onClick(View v) {
// TODO Auto-generated stub
final String strSDPath = txtSDCard.getText().toString();
final String strUrlServer = "https://www.thaicreate.com/android/uploadFile.php";
new UploadFileAsync().execute(strSDPath,strUrlServer);
}
});
}
public void showSuscess(String resServer)
{
/** Get result from Server (Return the JSON Code)
* StatusID = ? [0=Failed,1=Complete]
* Error = ? [On case error return custom error message]
*
* Eg Upload Failed = {"StatusID":"0","Error":"Cannot Upload file!"}
* Eg Upload Complete = {"StatusID":"1","Error":""}
*/
/*** Default Value ***/
String strStatusID = "0";
String strError = "Unknow Status!";
try {
JSONObject c = new JSONObject(resServer);
strStatusID = c.getString("StatusID");
strError = c.getString("Error");
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Prepare Status
if(strStatusID.equals("0"))
{
AlertDialog.Builder ad = new AlertDialog.Builder(this);
ad.setTitle("Error!");
ad.setIcon(android.R.drawable.btn_star_big_on);
ad.setMessage(strError);
ad.setPositiveButton("Close", null);
ad.show();
}
else
{
Toast.makeText(MainActivity.this, "Upload file Successfully", Toast.LENGTH_SHORT).show();
}
}
@Override
protected Dialog onCreateDialog(int id) {
switch (id) {
case DIALOG_UPLOAD_PROGRESS:
mProgressDialog = new ProgressDialog(this);
mProgressDialog.setMessage("Uploading file.....");
mProgressDialog.setProgressStyle(ProgressDialog.STYLE_SPINNER);
mProgressDialog.setCancelable(true);
mProgressDialog.show();
return mProgressDialog;
default:
return null;
}
}
public class UploadFileAsync extends AsyncTask<String, Void, Void> {
String resServer;
protected void onPreExecute() {
super.onPreExecute();
showDialog(DIALOG_UPLOAD_PROGRESS);
}
@Override
protected Void doInBackground(String... params) {
// TODO Auto-generated method stub
int bytesRead, bytesAvailable, bufferSize;
byte[] buffer;
int maxBufferSize = 1 * 1024 * 1024;
int resCode = 0;
String resMessage = "";
String lineEnd = "\r\n";
String twoHyphens = "--";
String boundary = "*****";
String strSDPath = params[0];
String strUrlServer = params[1];
try {
/** Check file on SD Card ***/
File file = new File(strSDPath);
if(!file.exists())
{
resServer = "{\"StatusID\":\"0\",\"Error\":\"Please check path on SD Card\"}";
return null;
}
FileInputStream fileInputStream = new FileInputStream(new File(strSDPath));
URL url = new URL(strUrlServer);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoInput(true);
conn.setDoOutput(true);
conn.setUseCaches(false);
conn.setRequestMethod("POST");
conn.setRequestProperty("Connection", "Keep-Alive");
conn.setRequestProperty("Content-Type",
"multipart/form-data;boundary=" + boundary);
DataOutputStream outputStream = new DataOutputStream(conn
.getOutputStream());
outputStream.writeBytes(twoHyphens + boundary + lineEnd);
outputStream
.writeBytes("Content-Disposition: form-data; name=\"filUpload\";filename=\""
+ strSDPath + "\"" + lineEnd);
outputStream.writeBytes(lineEnd);
bytesAvailable = fileInputStream.available();
bufferSize = Math.min(bytesAvailable, maxBufferSize);
buffer = new byte[bufferSize];
// Read file
bytesRead = fileInputStream.read(buffer, 0, bufferSize);
while (bytesRead > 0) {
outputStream.write(buffer, 0, bufferSize);
bytesAvailable = fileInputStream.available();
bufferSize = Math.min(bytesAvailable, maxBufferSize);
bytesRead = fileInputStream.read(buffer, 0, bufferSize);
}
outputStream.writeBytes(lineEnd);
outputStream.writeBytes(twoHyphens + boundary + twoHyphens + lineEnd);
// Response Code and Message
resCode = conn.getResponseCode();
if(resCode == HttpURLConnection.HTTP_OK)
{
InputStream is = conn.getInputStream();
ByteArrayOutputStream bos = new ByteArrayOutputStream();
int read = 0;
while ((read = is.read()) != -1) {
bos.write(read);
}
byte[] result = bos.toByteArray();
bos.close();
resMessage = new String(result);
}
Log.d("resCode=",Integer.toString(resCode));
Log.d("resMessage=",resMessage.toString());
fileInputStream.close();
outputStream.flush();
outputStream.close();
resServer = resMessage.toString();
} catch (Exception ex) {
// Exception handling
return null;
}
return null;
}
protected void onPostExecute(Void unused) {
showSuscess(resServer);
dismissDialog(DIALOG_UPLOAD_PROGRESS);
removeDialog(DIALOG_UPLOAD_PROGRESS);
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
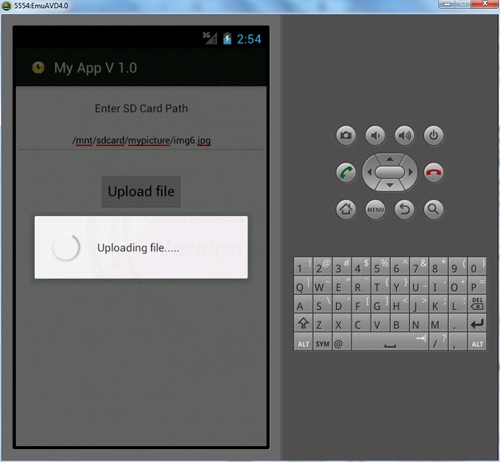
แสดง ProgressDialog ในขณะที่กำลัง Upload ไฟล์
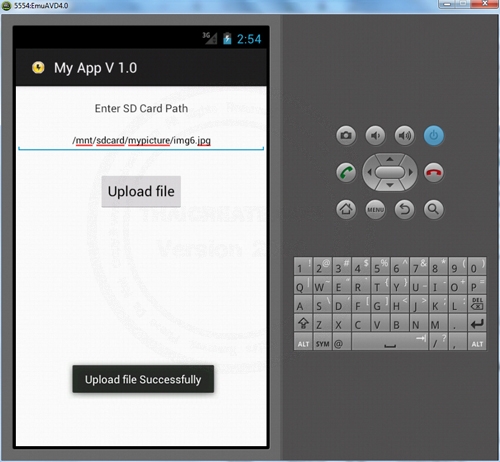
หลังจากอัพโหลดเรียบร้อยแล้ว Progress Dialog ก็จะหายไป
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-08-11 11:27:53 /
2017-03-26 22:45:43 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|