Android Web Service and JSON Parser |
Android Web Service and JSON Parser บทความและตัวอย่าง Basic Web Service และทำความเข้าใจพื้นฐานการนำ JSON มาใช้งานรวมกับ Web Service ในการรับส่งค่า Resource จาก Web Service ที่มาพร้อมกับ JSON Code เหตุผลที่ใช้ JSON ก็คือเราสามารถนำ Result ที่ได้จาก Web Service ที่อยู่ในรูปแบบของ JSON ไปใช้ได้ง่ายและสะดวก โดยจะสะดวกในกรณีที่ข้อมูลนั้นเป็นชุดของ Array ซึ่งถ้าปกติเราจะต้องเขียน function สำหรับแปลงข้อความเหล่านั้นหรือตัดเอาในตำแหน่งต่าง ๆ ที่ต้องการ แต่ถ้าใช้ JSON เราเพียงส่งข้อความให้อยู่ในรุปแบบ Syntax ของ JSON จากนั้นใช้ function เพื่อ Decode ข้อความ JSON ได้ในทันที และอ่านค่าของชุด Array จาก Index ได้ทันที
Android and Web Service
Android and JSON
พื้นฐาน JSON และ Web Service แนะนำให้อ่าน 2 บทความนี้
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
ทดสอบการสร้าง Web Service ด้วย PHP และ Return ค่าเป็น JSON
WebServiceServer.php
<?php
require_once("lib/nusoap.php");
//Create a new soap server
$server = new soap_server();
//Define our namespace
$namespace = "https://www.thaicreate.com/android/WebServiceServer.php";
$server->wsdl->schemaTargetNamespace = $namespace;
//Configure our WSDL
$server->configureWSDL("HelloWorld");
// Register our method and argument parameters
$varname = array(
'strName' => "xsd:string",
'strEmail' => "xsd:string"
);
$server->register('HelloWorld',$varname, array('return' => 'xsd:string'));
function HelloWorld($strName,$strEmail)
{
$arr["sName"] = "Sawatdee : ".$strName;
$arr["sEmail"] = "Sawatdee : ".$strEmail;
header('Content-type: application/json');
return json_encode($arr);
}
// Get our posted data if the service is being consumed
// otherwise leave this data blank.
$POST_DATA = isset($GLOBALS['HTTP_RAW_POST_DATA']) ? $GLOBALS['HTTP_RAW_POST_DATA'] : '';
// pass our posted data (or nothing) to the soap service
$server->service($POST_DATA);
exit();
?>
จาก Web Service ข้างต้นจะ Return ค่าเป็น JSON ดังนี้
Android Project สร้าง Application เพื่ออ่านค่า JSON
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml
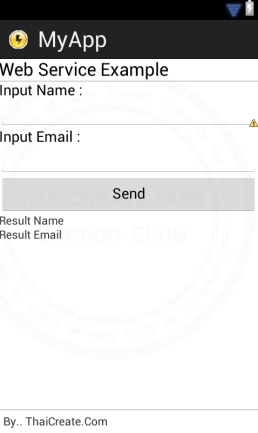
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Web Service Example "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TableRow>
</TableRow>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Name :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" >
</EditText>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Email :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:inputType="textEmailAddress"
android:ems="10" >
</EditText>
<Button
android:id="@+id/btnSend"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Send" />
<TextView
android:id="@+id/txtResultName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textAppearance="?android:attr/textAppearanceSmall"
android:text="Result Name" />
<TextView
android:id="@+id/txtResultEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Result Email" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
MainActivity.java
package com.myapp;
import java.io.IOException;
import org.json.JSONException;
import org.json.JSONObject;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
import org.xmlpull.v1.XmlPullParserException;
import android.os.Bundle;
import android.os.StrictMode;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import android.annotation.SuppressLint;
import android.app.Activity;
public class MainActivity extends Activity {
private final String NAMESPACE = "https://www.thaicreate.com/android/WebServiceServer.php";
private final String URL = "https://www.thaicreate.com/android/WebServiceServer.php?wsdl"; // WSDL URL
private final String SOAP_ACTION = "https://www.thaicreate.com/android/WebServiceServer.php/HelloWorld";
private final String METHOD_NAME = "HelloWorld"; // Method on web service
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder()
.permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// btnSend
Button btnSend = (Button) this.findViewById(R.id.btnSend);
btnSend.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// txtName
EditText txtName = (EditText) findViewById(R.id.txtName);
// txtEmail
EditText txtEmail = (EditText) findViewById(R.id.txtEmail);
// txtResultName
TextView txtResultName = (TextView) findViewById(R.id.txtResultName);
// txtResultEmail
TextView txtResultEmail = (TextView) findViewById(R.id.txtResultEmail);
SoapObject request = new SoapObject(NAMESPACE, METHOD_NAME);
request.addProperty("strName", txtName.getText().toString());
request.addProperty("strEmail", txtEmail.getText().toString());
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(
SoapEnvelope.VER11);
envelope.setOutputSoapObject(request);
HttpTransportSE androidHttpTransport = new HttpTransportSE(URL);
try {
androidHttpTransport.call(SOAP_ACTION, envelope);
SoapObject result = (SoapObject) envelope.bodyIn;
if (result != null) {
/** Get result from WebService (Return the JSON Code)
* Eg Login Failed = {"sName":"Sawatdee : Weerachai Nukitram","sEmail":"Sawatdee : [email protected]"}
*/
JSONObject c = new JSONObject(result.getProperty(0).toString());
String strResultName = c.getString("sName");
String strResultEmail = c.getString("sEmail");
txtResultName.setText(strResultName);
txtResultEmail.setText(strResultEmail);
} else {
Toast.makeText(getApplicationContext(),
"Web Service not Response!", Toast.LENGTH_LONG)
.show();
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (XmlPullParserException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
คำอธิบาย
JSONObject c = new JSONObject(result.getProperty(0).toString());
String strResultName = c.getString("sName");
String strResultEmail = c.getString("sEmail");
เป็นการอ่าน Result ที่อยู่ในรูปแบบ JSON โดยอ้างถึง Index ของ Array
Screenshot
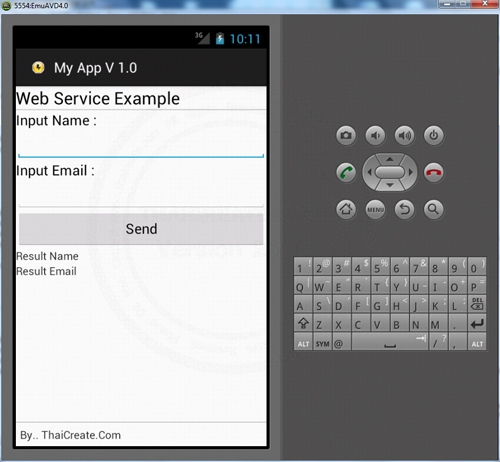
กรอกข้อมูล
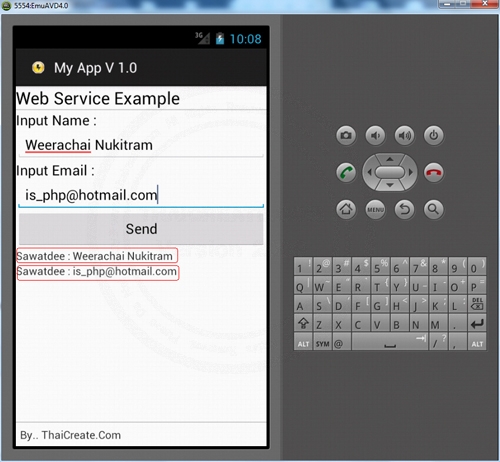
แสดงค่าจกา JSON ที่ถูกกแปลงเรียบร้อยแล้ว
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-08-11 15:03:04 /
2017-03-26 22:55:01 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|