|
 |
|
ขอโค๊ด คำสั่ง search โดยแสดงเป็น listview ข้อมูลจาก database โดยใช้ JSON |
|
 |
|
|
 |
 |
|
ขอโค๊ด คำสั่ง search โดยแสดงเป็น listview ข้อมูลจาก database โดยใช้ JSON
code
Code (Android-Java)
private static final String TAG_SUCCESS = "success";
private static final String TAG_ABSENT = "absent";
//output
private static final String TAG_EMPCODE = "empcode";
private static final String TAG_FNAME = "fname";
private static final String TAG_LNAME = "lname";
private static final String TAG_DEP_NAME = "dep_name";
private static final String TAG_BRANCH = "branch";
private static final String TAG_IMG_URL = "img_url";
String img_url,empcode,fname,lname,dep_name,branch;
String action = "employee_list";
EditText inputSearch;
int textlength=0;
Button btn_search;
JSONParser jParser = new JSONParser();
ArrayList<HashMap<String , String >> data;
ArrayList<HashMap<String , String >> searchResults;
ArrayList<HashMap<String , String>> search;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout_aex_employee);
try{
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("action",action));
// getting employee's details by making HTTP request
// Note that employee's details URL will use GET request
Log.i("params",params.toString());
JSONObject json = jParser.makeHttpRequest(url, "GET" ,params);
Log.d("json",json.toString());
int success = json.getInt(TAG_SUCCESS);
if (success == 1) {
JSONArray empObj = json.getJSONArray(TAG_ABSENT); // JSON Array
final ListView list_employee=(ListView)findViewById(R.id.list_employee);
data = new ArrayList<HashMap<String,String>>();
for(int i=0;i<empObj.length();i++){
JSONObject c= empObj.getJSONObject(i);
empcode =c.getString(TAG_EMPCODE);
fname =c.getString(TAG_FNAME);
lname =c.getString(TAG_LNAME);
dep_name =c.getString(TAG_DEP_NAME);
branch =c.getString(TAG_BRANCH);
img_url =c.getString(TAG_IMG_URL);
HashMap<String , String >map=new HashMap<String, String>();
map.put(TAG_EMPCODE, empcode);
map.put(TAG_FNAME, fname);
map.put(TAG_LNAME, lname);
map.put(TAG_DEP_NAME, dep_name);
map.put(TAG_BRANCH, branch);
map.put(TAG_IMG_URL, img_url);
data.add(map);
}
list_employee.setAdapter(new AbsentAdapter(this));
list_employee.setOnItemClickListener(new OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> arg0,
View view, int position, long id) {
// TODO Auto-generated method stub
TextView empcode =(TextView)view.findViewById(R.id.textView1);
empcode.setText(data.get(position).get(TAG_EMPCODE));
String emp=empcode.getText().toString();
Intent intent = new Intent(getApplicationContext(),aex_employee_personal.class);
intent.putExtra("empcode", emp);
startActivity(intent);
}
});
searchResults=new ArrayList<HashMap<String,String>>(data);
inputSearch=(EditText)findViewById(R.id.edit_search);
InputMethodManager imm =(InputMethodManager)getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(inputSearch.getWindowToken(), 0);
inputSearch.addTextChangedListener(new TextWatcher() {
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
// TODO Auto-generated method stub
String searchString=inputSearch.getText().toString();
textlength=searchString.length();
searchResults.clear();
for(int i=0;i<data.size();i++){
String playerName=data.get(i).get(TAG_EMPCODE).toString();
if(textlength<=playerName.length()){
if(searchString.equalsIgnoreCase(playerName.substring(0,textlength))){
Log.i("searchResults",searchResults.toString());
searchResults.add(data.get(i));
String[] from ={"TextView","TextView","TextView","TextView","TextView"};
int[] to={R.id.textView1,R.id.textView2,R.id.textView3,R.id.textView4,R.id.textView5};
SimpleAdapter sAdap = new SimpleAdapter(aex_employee.this,searchResults,
R.layout.layout_item_employee,from,to);
list_employee.setAdapter(sAdap);
}
}
}
}
@Override
public void beforeTextChanged(CharSequence s, int start, int count,
int after) {
// TODO Auto-generated method stub
}
@Override
public void afterTextChanged(Editable s) {
// TODO Auto-generated method stub
}
});
} else {
Toast.makeText(getApplicationContext(), "ไม่พบข้อมูล", Toast.LENGTH_SHORT).show();
}
} catch (JSONException e) {
e.printStackTrace();
Toast.makeText(getApplicationContext(), e.toString(), Toast.LENGTH_SHORT).show();
}
}
class AbsentAdapter extends BaseAdapter{
private Context context;
public AbsentAdapter(Context c){
context=c;
}
public AbsentAdapter(TextWatcher textWatcher){
}
@Override
public int getCount() {
// TODO Auto-generated method stub
return data.size();
}
@Override
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
@Override
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
@Override
public View getView(int position, View view, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater infater =(LayoutInflater) context.getSystemService(context.LAYOUT_INFLATER_SERVICE);
if(view == null){
view = infater.inflate(R.layout.layout_item_employee,null);
}
//empcode
TextView txt_empcode =(TextView)view.findViewById(R.id.textView1);
txt_empcode.setText(data.get(position).get(TAG_EMPCODE));
//fname
TextView txt_fname =(TextView)view.findViewById(R.id.textView2);
txt_fname.setText(data.get(position).get(TAG_FNAME));
//lname
TextView txt_lname =(TextView)view.findViewById(R.id.textView3);
txt_lname.setText(data.get(position).get(TAG_LNAME));
//dep_name
TextView txt_dep_name =(TextView)view.findViewById(R.id.textView4);
txt_dep_name.setText(data.get(position).get(TAG_DEP_NAME));
//branch
TextView txt_branch =(TextView)view.findViewById(R.id.textView5);
txt_branch.setText(data.get(position).get(TAG_BRANCH));
//img_url
ImageView img =(ImageView)view.findViewById(R.id.imageView1);
URL url;
try {
url = new URL(data.get(position).get(TAG_IMG_URL));
img.getLayoutParams().height =90;
img.getLayoutParams().width= 90;
img.setImageBitmap(BitmapFactory.decodeStream(url.openStream()));
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return view;
}
}
}
Tag : Mobile, Android, JAVA, Windows

|
|
 |
 |
 |
 |
Date :
2013-02-08 11:39:01 |
By :
v_rong |
View :
2489 |
Reply :
2 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตัวนี้เลยครับ
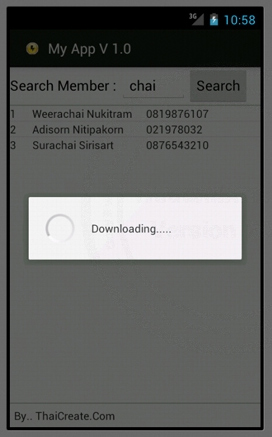
Android ProgressBar/ProgressDialog Search Display result Data from Web Server
|
 |
 |
 |
 |
Date :
2013-02-08 12:51:49 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 03
|