 |
iOS - ตอนนี้ทำหน้า search อยู่ค่ะต้องการทำหน้า detail หนังสือ แต่มันยังerror อยู่ค่ะไม่ทราบว่าโค้ดผิดตรงไหนมั้ยค่ะหาไม่เจอ |
|
 |
|
|
 |
 |
|
พอแตะที่ชื่อหนังสือเพื่อไปหน้ารายละเอียดหนังสือแล้วมันขึ้น error เหมือนไม่ส่งค่าไปหน้า detail ค่ะแต่ไม่รู้ว่าโค้ดผิดตรงไหนค่ะรบกวนผู้รู้ด้วยน่ะค่ะ
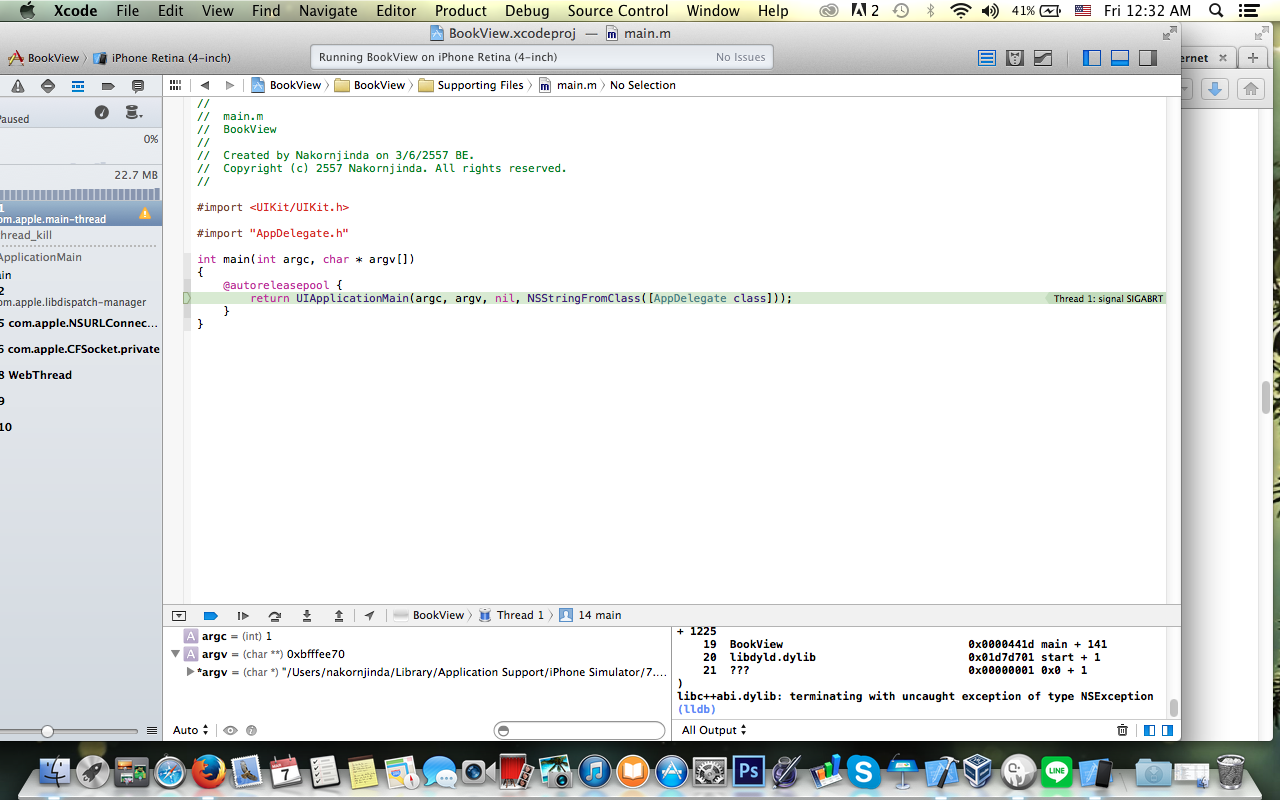
MasterViewController.h
Code (Objective-C)
#import <UIKit/UIKit.h>
@interface MasterViewController : UITableViewController<UITableViewDataSource,UITableViewDelegate,UISearchBarDelegate>
@property (strong, nonatomic) IBOutlet UITableView *tableData;
@property(nonatomic,assign) NSMutableData *receivedData;
@end
MasterViewController.m
Code (Objective-C)
#import "MasterViewController.h"
#import "DetailViewController.h"
@interface MasterViewController ()
{
NSMutableArray *allObject;
NSMutableArray *displayObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *idbook;
NSString *title1;
NSString *access;
NSString *w_name;
NSString *status;
NSString *bcontent;
}
@end
@implementation MasterViewController
- (void)awakeFromNib
{
[super awakeFromNib];
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Define keys
idbook = @"Idbook";
title1 = @"title1";
access = @"access";
w_name = @"w_name";
status= @"status1";
bcontent=@"content";
// Create array to hold dictionaries
allObject = [[NSMutableArray alloc] init];
NSData *jsonData = [NSData dataWithContentsOfURL:
[NSURL URLWithString:@"http://localhost:8888/searchtest.php"]];
id jsonObjects = [NSJSONSerialization JSONObjectWithData:jsonData options:NSJSONReadingMutableContainers error:nil];
// values in foreach loop
for (NSDictionary *dataDict in jsonObjects) {
NSString *strIdbook = [dataDict objectForKey:@"Idbook"];
NSString *strtitle1 = [dataDict objectForKey:@"title1"];
NSString *straccess = [dataDict objectForKey:@"access"];
NSString *strw_name= [dataDict objectForKey:@"w_name"];
NSString *strstatus1= [dataDict objectForKey:@"status1"];
NSString *strcontent= [dataDict objectForKey:@"content"];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
strIdbook, idbook,
strtitle1, title1,
straccess, access,
strw_name, w_name,
strstatus1,status,
strcontent,bcontent,
nil];
[allObject addObject:dict];
}
displayObject =[[NSMutableArray alloc] initWithArray:allObject];
// Do any additional setup after loading the view, typically from a nib.
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
#pragma mark - Table View
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return displayObject.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
// Use the default cell style.
cell = [[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier];
}
NSDictionary *tmpDict = [displayObject objectAtIndex:indexPath.row];
NSString *cellValue;
cellValue = [tmpDict objectForKey:title1];
NSString* detailbook;
detailbook = [NSString stringWithFormat:@"รหัส%@ , ผู้แต่ง %@, สถานะ %@ "
,[tmpDict objectForKey:access]
,[tmpDict objectForKey:w_name]
,[tmpDict objectForKey:status]];
cell.textLabel.text = cellValue;
cell.detailTextLabel.text= detailbook;
cell.accessoryType=UITableViewCellAccessoryDisclosureIndicator;
return cell;
}
- (BOOL)searchDisplayController:(UISearchDisplayController *)controller shouldReloadTableForSearchString:(NSString *)searchString
{
if([searchString length] == 0)
{
[displayObject removeAllObjects];
[displayObject addObjectsFromArray:allObject];
}
else
{
[displayObject removeAllObjects];
for(NSDictionary *tmpDict in allObject)
{
NSString *val = [tmpDict objectForKey:title1];
NSRange r = [val rangeOfString:searchString options:NSCaseInsensitiveSearch];
if(r.location != NSNotFound)
{
[displayObject addObject:tmpDict];
}
}
}
return YES;
}
- (void)searchBarCancelButtonClicked:(UISearchBar *)searchBar {
searchBar.text=@"";
[searchBar setShowsCancelButton:NO animated:YES];
[searchBar resignFirstResponder];
}
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath
{
// Return NO if you do not want the specified item to be editable.
return YES;
}
#pragma mark - TableView Delegate
-(void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath {
// Perform segue to candy detail
[self performSegueWithIdentifier:@"candyDetail" sender:tableView];
}
/*
// Override to support rearranging the table view.
- (void)tableView:(UITableView *)tableView moveRowAtIndexPath:(NSIndexPath *)fromIndexPath toIndexPath:(NSIndexPath *)toIndexPath
{
}
*/
/*
// Override to support conditional rearranging of the table view.
- (BOOL)tableView:(UITableView *)tableView canMoveRowAtIndexPath:(NSIndexPath *)indexPath
{
// Return NO if you do not want the item to be re-orderable.
return YES;
}
*/
#pragma mark - Segue
-(void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender
{
if ([segue.identifier isEqualToString:@"lodgedetail"]) {
NSIndexPath * indexpath = [self.tableView indexPathForSelectedRow];
NSDictionary *tmpDict = [allObject objectAtIndex:indexpath.row];
[[segue destinationViewController] setNameItem:[tmpDict objectForKey:title1]];
[[segue destinationViewController] setAccessItem:[tmpDict objectForKey:access]];
[[segue destinationViewController] setDetailItem:[tmpDict objectForKey:bcontent]];
}
}
@end
DetailViewController.h
Code (Objective-C)
#import <UIKit/UIKit.h>
@interface DetailViewController : UIViewController
{
IBOutlet UILabel *namebook;
IBOutlet UILabel *accessbook;
IBOutlet UILabel *detailbook;
}
@property (strong, nonatomic) id detailItem;
@property(strong,nonatomic) id nameItem;
@property(strong,nonatomic) id accessItem;
-(void)setNameItem:(id)newtitle1;
-(void)setAccessItem:(id)newaccess;
-(void)setDetailItem:(id)newbcontent;
@end
DetailViewController.m
Code (Objective-C)
#import "DetailViewController.h"
@interface DetailViewController ()
@end
@implementation DetailViewController
@synthesize detailItem,accessItem,nameItem;
#pragma mark - Managing the detail item
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view.
namebook.text = [nameItem description];
accessbook.text = [accessItem description];
detailbook.text = [detailItem description];
}
-(void)setNameItem:(id)newtitle1
{
nameItem = newtitle1;
}
-(void)setAccessItem:(id)newaccess
{
accessItem = newaccess;
}
-(void)setDetailItem:(id)newbcontent
{
detailItem = newbcontent;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
-(void)dealloc{
[namebook release];
[accessbook release];
[detailbook release];
[super dealloc];
}
@end
Tag : Mobile, MySQL, iOS, iPhone, Objective-C, Mac
|
|
 |
 |
 |
 |
Date :
2014-03-07 00:46:01 |
By :
fern_105 |
View :
1091 |
Reply :
3 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
คงจะต้องได้นั่ง Debug ดูแล้วครับว่า Error ตรงไหน 
|
 |
 |
 |
 |
Date :
2014-03-07 09:26:34 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ลองดูที่การผูกของในหน้า Story ว่าผูกครบไหม ตัว View หลุดไหม แล้วก็ลองนั่ง Debug ตั้งแต่ตอนที่เริ่มแตะไปอะครับว่ามันถึงตรงไหนแล้วเกิด Error ครับ :)
|
 |
 |
 |
 |
Date :
2014-03-07 13:59:03 |
By :
thannam001 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
น่าจะผิดตอนที่เชื่อมพวก IBOutlet ครับ
|
 |
 |
 |
 |
Date :
2014-03-08 06:59:51 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|