 |
|
สอบถามคือผมอยากคลิกตรงเบอร์โทรแล้ว เบอร์จะไปโผล่หน้ากรอกเบอร์ ตามรูปอ่ะครับไม่รู้ทำยังไง
พอดีไปเจอแอพหนึ่งทำได้ แต่ผมไม่รู้ว่าใช่คำสั่งอะไร ช่วยดูโค้ดให้ทีครับผมจะลองไปทำดู ขอบคุณครับ
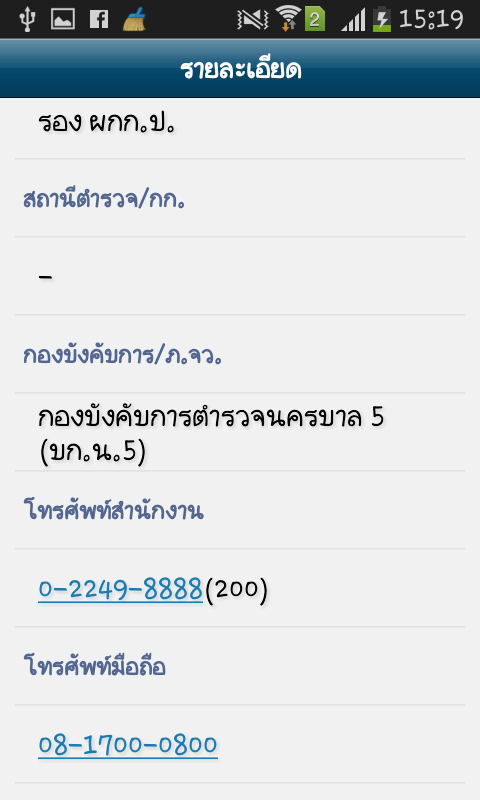
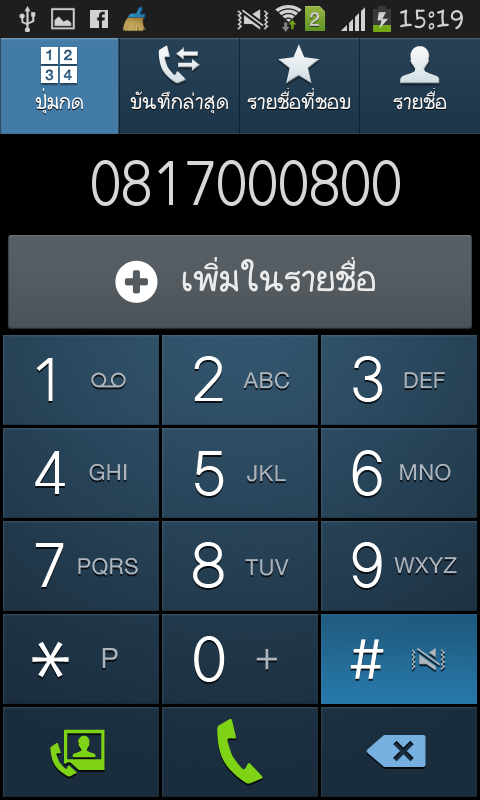
Code
package com.appTest.testapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.util.Log;
import android.view.View;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import android.content.DialogInterface.OnClickListener;
public class Search extends Activity {
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_search);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
final Button btn1 = (Button) findViewById(R.id.button1);
// Perform action on click
btn1.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
SearchData();
}
});
}
public void SearchData()
{
// listView1
final ListView lisView1 = (ListView)findViewById(R.id.listView1);
// editText1
final EditText inputText = (EditText)findViewById(R.id.editText1);
/**
* [{"CustomerID":"C001","Name":"Win Weerachai","Email":"[email protected]" ,"CountryCode":"TH","Budget":"1000000","Used":"600000"},
* {"CustomerID":"C002","Name":"John Smith","Email":"[email protected]" ,"CountryCode":"EN","Budget":"2000000","Used":"800000"},
* {"CustomerID":"C003","Name":"Jame Born","Email":"[email protected]" ,"CountryCode":"US","Budget":"3000000","Used":"600000"},
* {"CustomerID":"C004","Name":"Chalee Angel","Email":"[email protected]" ,"CountryCode":"US","Budget":"4000000","Used":"100000"}]
*/
String url = "http://www.autocontrol.in.th/policephone/getJSON.php";
//String url = "http://10.0.2.2/android/test.php";
// Paste Parameters
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("txtKeyword", inputText.getText().toString()));
try {
JSONArray data = new JSONArray(getJSONUrl(url,params));
final ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("ID", c.getString("ID"));
map.put("Position", c.getString("Position"));
map.put("Name", c.getString("Name"));
map.put("Tel", c.getString("Tel"));
map.put("Mobile", c.getString("Mobile"));
map.put("Station", c.getString("Station"));
map.put("Province", c.getString("Province"));
MyArrList.add(map);
}
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(Search.this, MyArrList, R.layout.activity_column,
new String[] {"Name", "Station"}, new int[] {R.id.ColName, R.id.ColStation});
lisView1.setAdapter(sAdap);
final AlertDialog.Builder viewDetail = new AlertDialog.Builder(this);
// OnClick Item
lisView1.setOnItemClickListener(new OnItemClickListener() {
public void onItemClick(AdapterView<?> myAdapter, View myView,
int position, long mylng) {
String strPosition = MyArrList.get(position).get("Position")
.toString();
String sName = MyArrList.get(position).get("Name")
.toString();
String strTel = MyArrList.get(position).get("Tel")
.toString();
String strMobile = MyArrList.get(position).get("Mobile")
.toString();
String strStation = MyArrList.get(position).get("Station")
.toString();
String strProvince = MyArrList.get(position).get("Province")
.toString();
viewDetail.setIcon(android.R.drawable.btn_star_big_on);
viewDetail.setTitle("รายละเอียด");
viewDetail.setMessage("ตำแหน่ง : " + strPosition + "\n"
+ "ชื่อ : " + sName + "\n"
+ "ที่ทำงาน : " + strTel + "\n"
+ "มือถือ : " + strMobile + "\n"
+ "ประจำ : " + strStation + "\n"
+ "จังหวัด : " + strProvince);
viewDetail.setPositiveButton("ตกลง",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
dialog.dismiss();
}
});
viewDetail.show();
}
});
} catch (JSONException e) {
e.printStackTrace();
}
}
public String getJSONUrl(String url,List<NameValuePair> params) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
try {
httpPost.setEntity(new UrlEncodedFormEntity(params,"utf-8"));
HttpResponse response = client.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content,"utf-8"));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download file..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
public void onBackPressed() {
AlertDialog.Builder dialog = new AlertDialog.Builder(this);
dialog.setTitle("ออก");
dialog.setIcon(R.drawable.ic_launcher);
dialog.setCancelable(true);
dialog.setMessage("คุณต้องการออกจากระบบใช่หรือไม่?");
dialog.setPositiveButton("ใช่", new OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
finish();
Intent intent = new Intent(Intent.ACTION_MAIN);
intent.addCategory(Intent.CATEGORY_HOME);
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
startActivity(intent);
}
});
dialog.setNegativeButton("ไม่", new OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
dialog.cancel();
}
});
dialog.show();
}
}
Tag : Mobile, Mobile
|
ประวัติการแก้ไข 2014-03-31 15:39:32 2014-03-31 19:42:19
|
 |
 |
 |
 |
Date :
2014-03-31 15:38:15 |
By :
jokerjoza555 |
View :
1272 |
Reply :
3 |
|
 |
 |
 |
 |
|
|
|
 |