|
 |
|
Andoid - ผมอยากจะคลิกตรงแผนที่ Google Maps แล้วเปิดแอป Google Maps ขึ้นมานะครับต้องทำไง |
|
 |
|
|
 |
 |
|
ผมอยากจะคลิกตรงแผนที่ Google Maps แล้วเปิดแอป Google Maps ขึ้นมานะครับต้องทำไง
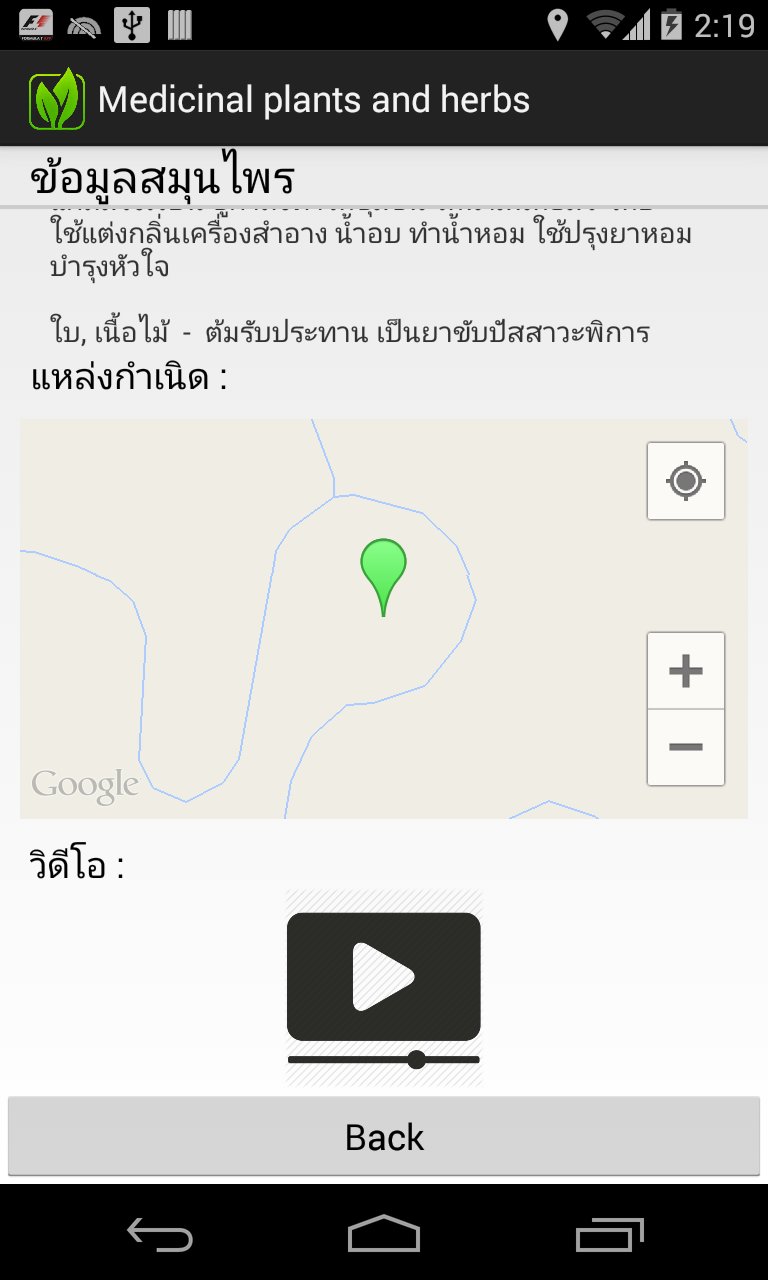
Code (Android-Java)
package com.example.last;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.BufferedHttpEntity;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONException;
import org.json.JSONObject;
import com.example.last.DetailActivity_th;
import com.example.last.R;
import com.google.android.gms.maps.CameraUpdateFactory;
import com.google.android.gms.maps.GoogleMap;
import com.google.android.gms.maps.SupportMapFragment;
import com.google.android.gms.maps.model.BitmapDescriptorFactory;
import com.google.android.gms.maps.model.LatLng;
import com.google.android.gms.maps.model.Marker;
import com.google.android.gms.maps.model.MarkerOptions;
import android.net.Uri;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.content.Intent;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.support.v4.app.FragmentActivity;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
public class DetailActivity_th<img> extends FragmentActivity {
private GoogleMap mGoogleMap;
private SupportMapFragment mSupportMapFragment;
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_detail_th);
setTitle("Medicinal plants and herbs");
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder()
.permitAll().build();
StrictMode.setThreadPolicy(policy);
}
showInfo();
// btnBack
final Button btnBack = (Button) findViewById(R.id.btnBack);
// Perform action on click
btnBack.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent newActivity = new Intent(DetailActivity_th.this,
Main_Th.class);
startActivity(newActivity);
}
});
}
public void showInfo() {
final TextView tMemberID = (TextView) findViewById(R.id.herb_name);
final TextView tUsername = (TextView) findViewById(R.id.name_sci);
final TextView tPassword = (TextView) findViewById(R.id.herb_gene);
final TextView tName = (TextView) findViewById(R.id.herb_fix);
final ImageView img = (ImageView) findViewById(R.id.imageView1);
// final ImageView img2 = (ImageView) findViewById(R.id.imageView2);
String ip = "10.60.7.62";
String urll = "http://"+ip+"/test/json/getByMemberID.php";
Intent intent = getIntent();
final String herb_id = intent.getStringExtra("herb_id");
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("txtKeyword", herb_id));
String resultServer = getHttpPost(urll, params);
String herb_name = "";
String name_sci = "";
String herb_gene = "";
String herb_fix = "";
String herb_pic = "";
JSONObject c;
try {
c = new JSONObject(resultServer);
herb_name = c.getString("herb_name");
name_sci = c.getString("name_sci");
herb_gene = c.getString("herb_gene");
herb_fix = c.getString("herb_fix");
herb_pic = c.getString("herb_pic");
final String herb_vdox = c.getString("herb_vdo");
final double herb_lat = c.getDouble("herb_lat");
final double herb_long = c.getDouble("herb_long");
final String latlong_detail = c.getString("latlong_detail");
final String detail_1 = c.getString("detail_1");
final ImageView vdoim = (ImageView) findViewById(R.id.imageView2);
vdoim.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent i = new Intent(Intent.ACTION_VIEW);
i.putExtra("force_fullscreen", true);
i.setData(Uri.parse("" + herb_vdox + ""));
startActivity(i);
}
});
if(herb_vdox.equals("")){
vdoim.setImageResource(R.drawable.no);
vdoim.setEnabled(false);
}
// if(!herb_name.equals(""))
// {
tMemberID.setText(herb_name);
tUsername.setText(name_sci);
tPassword.setText(herb_gene);
tName.setText(herb_fix);
// }
// else
// {
// tMemberID.setText("-");
// tUsername.setText("-");
// tPassword.setText("-");
// tName.setText("-");
// tEmail.setText("-");
// tTel.setText("-");
// }
// view/fragment matching
mSupportMapFragment = (SupportMapFragment) getSupportFragmentManager()
.findFragmentById(R.id.main_Support_map_fragment);
mGoogleMap = mSupportMapFragment.getMap();
// Set Google Map Location
mGoogleMap.setMyLocationEnabled(true);
mGoogleMap.setMapType(GoogleMap.MAP_TYPE_NORMAL);
// register event for google map
mGoogleMap
.setOnInfoWindowClickListener(new GoogleMap.OnInfoWindowClickListener() {
@Override
public void onInfoWindowClick(Marker marker) {
Toast.makeText(DetailActivity_th.this,
marker.getTitle(), Toast.LENGTH_SHORT)
.show();
// TODO Auto-generated method stub
}
});
// animate Google Map's camera
mGoogleMap.animateCamera(CameraUpdateFactory.newLatLngZoom(
new LatLng(herb_lat,
herb_long), 15));
addMarkerToGoogleMap(detail_1,latlong_detail, herb_lat,
herb_long);
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// ------------------------------------------------------
try {
URL url = new URL("http://" + ip + "/test/myfile/" + herb_pic);
// try this url =
// "http://0.tqn.com/d/webclipart/1/0/5/l/4/floral-icon-5.jpg"
HttpGet httpRequest = null;
httpRequest = new HttpGet(url.toURI());
HttpClient httpclient = new DefaultHttpClient();
HttpResponse response = (HttpResponse) httpclient
.execute(httpRequest);
HttpEntity entity = response.getEntity();
BufferedHttpEntity b_entity = new BufferedHttpEntity(entity);
InputStream input = b_entity.getContent();
Bitmap bitmap = BitmapFactory.decodeStream(input);
img.setImageBitmap(bitmap);
} catch (Exception ex) {
}
}
public String getHttpPost(String url, List<NameValuePair> params) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
try {
httpPost.setEntity(new UrlEncodedFormEntity(params));
HttpResponse response = client.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Status OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(
new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
private void addMarkerToGoogleMap(String title, String snippet, double lat,
double lng) {
if (null != mGoogleMap) {
MarkerOptions options = new MarkerOptions();
options.title(title);
options.snippet(snippet);
options.position(new LatLng(lat, lng));
options.icon(BitmapDescriptorFactory
.defaultMarker(BitmapDescriptorFactory.HUE_GREEN));
// add marker
mGoogleMap.addMarker(options);
}
}
}
Tag : Mobile, MySQL, Android, JAVA

|
|
 |
 |
 |
 |
Date :
2014-07-09 02:24:35 |
By :
madrid_utd |
View :
1153 |
Reply :
1 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (Android-Java)
Intent i;
PackageManager manager = getPackageManager();
try {
i = manager.getLaunchIntentForPackage("app package name");
if (i == null)
throw new PackageManager.NameNotFoundException();
i.addCategory(Intent.CATEGORY_LAUNCHER);
startActivity(i);
} catch (PackageManager.NameNotFoundException e) {
}
|
 |
 |
 |
 |
Date :
2014-07-09 17:23:22 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 02
|