|
 |
|
Android อยากจะเพิ่มฟังชั่นให้ส่งค่าไป Select Database ก่อน แล้วดึงค่าที่ Select ออกมาแสดงครับ |
|
 |
|
|
 |
 |
|
อันนี้ตัวอย่างที่ผมเอามาดัดแปลงครับ
Code (Android)
package com.androidtutorialpoint.androidlogin;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.AsyncTask;
import android.os.Bundle;
import android.preference.PreferenceManager;
import android.support.v4.widget.SwipeRefreshLayout;
import android.util.Log;
import android.view.View;
import android.view.Window;
import android.widget.ListView;
import android.widget.ProgressBar;
import android.widget.TextView;
import android.widget.Toast;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.methods.HttpUriRequest;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.params.BasicHttpParams;
import org.apache.http.params.HttpConnectionParams;
import org.apache.http.params.HttpParams;
import org.apache.http.protocol.HTTP;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.List;
public class TopicActivity extends Activity {
String GetUser;
private SwipeRefreshLayout refresh;
private TextView userTextView;
private TextView idTextView;
ListView listCollege;
ProgressBar proCollageList;
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_topic);
refresh = (SwipeRefreshLayout)
findViewById(R.id.swipe_refresh_layout);
refresh.setOnRefreshListener(new SwipeRefreshLayout.OnRefreshListener() {
@Override
public void onRefresh() {
Intent intent = getIntent();
finish();
overridePendingTransition( 0, 0);
startActivity(intent);
overridePendingTransition( 0, 0);
}
});
Bundle bundle = getIntent().getExtras();
listCollege = (ListView)findViewById(R.id.listCollege);
proCollageList = (ProgressBar)findViewById(R.id.proCollageList);
final String user = bundle.getString("username");
final String id = bundle.getString("userid");
userTextView = (TextView) findViewById(R.id.username_text);
userTextView.setText(user);
idTextView = (TextView) findViewById(R.id.userid_text);
idTextView.setText(id);
SharedPreferences prefs = PreferenceManager.getDefaultSharedPreferences(this);
SharedPreferences.Editor editor = prefs.edit();
editor.putString("username", user);
editor.putString("userid", id);
editor.commit();
new GetHttpResponse(this).execute();
}
private class GetHttpResponse extends AsyncTask<Void, Void, Void>
{
private Context context;
String result;
List<cources> collegeList;
public GetHttpResponse(Context context)
{
this.context = context;
}
@Override
protected void onPreExecute()
{
super.onPreExecute();
}
@Override
protected Void doInBackground(Void... arg0)
{
HttpService httpService = new HttpService("https://thongkumdee.000webhostapp.com/courses.php");
try
{
httpService.ExecutePostRequest();
if(httpService.getResponseCode() == 200)
{
result = httpService.getResponse();
Log.d("Result", result);
if(result != null)
{
JSONArray jsonArray = null;
try {
jsonArray = new JSONArray(result);
JSONObject object;
JSONArray array;
cources college;
collegeList = new ArrayList<cources>();
for(int i=0; i<jsonArray.length(); i++)
{
college = new cources();
object = jsonArray.getJSONObject(i);
college.product_id = object.getString("sc_poker_product");
college.cources_description = object.getString("sc_product_story");
collegeList.add(college);
}
}
catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
else
{
Toast.makeText(context, httpService.getErrorMessage(), Toast.LENGTH_SHORT).show();
}
}
catch (Exception e)
{
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
@Override
protected void onPostExecute(Void result)
{
proCollageList.setVisibility(View.GONE);
listCollege.setVisibility(View.VISIBLE);
if(collegeList != null)
{
ListAdapter adapter = new ListAdapter(collegeList, context);
listCollege.setAdapter(adapter);
}
}
}
public class HttpService
{
private ArrayList<NameValuePair> params;
private ArrayList<NameValuePair> headers;
private String url;
private int responseCode;
private String message;
private String response;
public String getResponse()
{
return response;
}
public String getErrorMessage()
{
return message;
}
public int getResponseCode()
{
return responseCode;
}
public HttpService(String url)
{
this.url = url;
params = new ArrayList<NameValuePair>();
headers = new ArrayList<NameValuePair>();
}
public void AddParam(String name, String value)
{
params.add(new BasicNameValuePair(name, value));
}
public void AddHeader(String name, String value)
{
headers.add(new BasicNameValuePair(name, value));
}
public void ExecuteGetRequest() throws Exception
{
String combinedParams = "";
if(!params.isEmpty())
{
combinedParams += "?";
for(NameValuePair p : params)
{
String paramString = p.getName() + "=" + URLEncoder.encode(p.getValue(),"UTF-8");
if(combinedParams.length() > 1)
{
combinedParams += "&" + paramString;
}
else
{
combinedParams += paramString;
}
}
}
HttpGet request = new HttpGet(url + combinedParams);
for(NameValuePair h : headers)
{
request.addHeader(h.getName(), h.getValue());
}
executeRequest(request, url);
}
public void ExecutePostRequest() throws Exception
{
HttpPost request = new HttpPost(url);
for(NameValuePair h : headers)
{
request.addHeader(h.getName(), h.getValue());
}
if(!params.isEmpty())
{
request.setEntity(new UrlEncodedFormEntity(params, HTTP.UTF_8));
}
executeRequest(request, url);
}
private void executeRequest(HttpUriRequest request, String url)
{
HttpParams httpParameters = new BasicHttpParams();
int timeoutConnection = 10000;
HttpConnectionParams.setConnectionTimeout(httpParameters, timeoutConnection);
int timeoutSocket = 10000;
HttpConnectionParams.setSoTimeout(httpParameters, timeoutSocket);
HttpClient client = new DefaultHttpClient(httpParameters);
HttpResponse httpResponse;
try
{
httpResponse = client.execute(request);
responseCode = httpResponse.getStatusLine().getStatusCode();
message = httpResponse.getStatusLine().getReasonPhrase();
HttpEntity entity = httpResponse.getEntity();
if (entity != null)
{
InputStream instream = entity.getContent();
response = convertStreamToString(instream);
instream.close();
}
}
catch (ClientProtocolException e)
{
client.getConnectionManager().shutdown();
e.printStackTrace();
}
catch (IOException e)
{
client.getConnectionManager().shutdown();
e.printStackTrace();
}
}
private String convertStreamToString(InputStream is)
{
BufferedReader reader = new BufferedReader(new InputStreamReader(is));
StringBuilder sb = new StringBuilder();
String line = null;
try
{
while ((line = reader.readLine()) != null)
{
sb.append(line + "\n");
}
}
catch (IOException e)
{
e.printStackTrace();
}
finally
{
try
{
is.close();
}
catch (IOException e)
{
e.printStackTrace();
}
}
return sb.toString();
}
}
}
อันนี้เป็นส่วน PHP ที่ต่อกับ database
Code (PHP)
<?php
$hostname = "localhost";
$username = "id1479268_staporn";
$password = "054324696t";
$dbname = "id1479268_sc_db";
// Create connection
$conn = new mysqli($hostname, $username, $password, $dbname);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$id_user = 'us0005';
$sql = "SELECT * FROM sc_poker,sc_productbacklog WHERE sc_productbacklog.sc_product_id = sc_poker.sc_poker_product AND sc_poker_product NOT IN (SELECT sc_vote_product FROM sc_vote WHERE sc_vote_user = '$id_user')";
$result = $conn->query($sql);
if ($result->num_rows >0) {
// output data of each row
while($row[] = $result->fetch_assoc()) {
$tem = $row;
$json = json_encode($tem);
}
} else {
echo "0 results";
}
echo $json;
$conn->close();
?>
ที่ผมทำตอนนี้มันแสดงค่าได้อย่างเดียว แต่ผมต้องการให้ android มันส่งค่า $id_user ไป select ด้วย แล้วค่อยนำข้อมูลที่ select มาแสดง ผมต้องเพิ่มฟังชั่นยังไงครับ
Tag : Mobile, Android

|
|
 |
 |
 |
 |
Date :
2017-05-18 14:26:52 |
By :
Taiyark |
View :
963 |
Reply :
1 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ดูวิธีการส่งค่าไปดึงมาใช้งานครับ
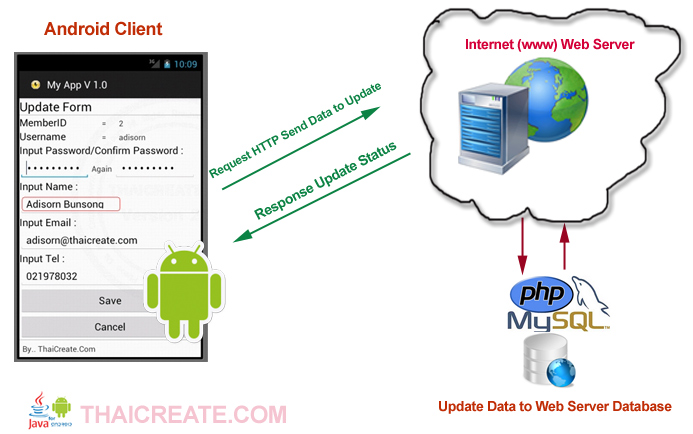
Android Edit/Update Data to Web Server Database (Web Server)
|
 |
 |
 |
 |
Date :
2017-05-19 10:49:22 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 04
|