iOS/iPhone Collection View and Master Detail (Objective-C, iPhone, iPad) |
iOS/iPhone Collection View and Master Detail (Objective-C, iPhone, iPad) บทความนี้เป็นภาคต่อของ Collection View เป็นการแสดงข้อมูลแบบ Collection แบ่งออกเป็นหลาย ๆ Column และในตัวอย่างนี้จะมีการเพิ่มฟีเจอร์เป็นแบบ Master Detail คือสามารถคลิกในรายการของแต่ล่ะ Item และแสดงรายละเอียดต่าง ๆ ใน View ที่เป็น Detail หรือรายละเอียด
iOS/iPhone Collection View and Master Detail
สำหรับตัวอย่างนี้ เป็นการแสดง Master Detail จาก Collection View จะเป็นการเขียนต่อจากบทความที่แล้ว เพราะฉะนั้นถ้าจะให้เข้าใจ แนะนำให้ไปดูบทความก่อนหน้านี้ ตามลิ้งค์นี้
iOS/iPhone Collection View (UICollectionViewController) Multiple Column Item (iPhone, iPad)
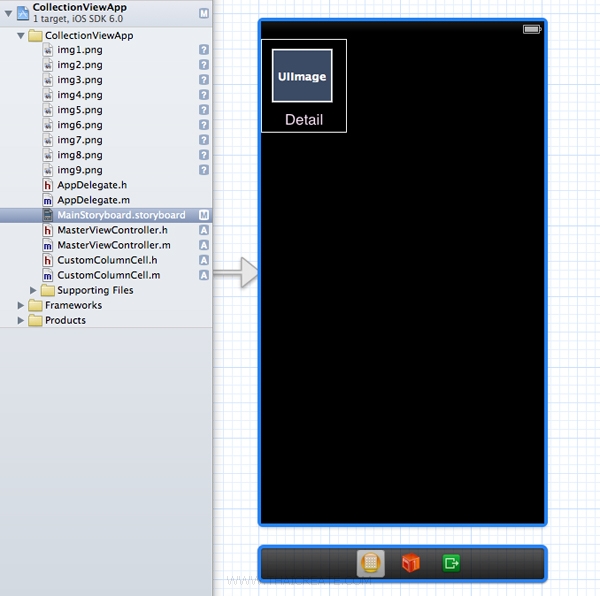
จกาตัวอย่างก่อนหน้านี้ เราได้ออกแบบ Collection View และ Collection View Cell บน Storyboard
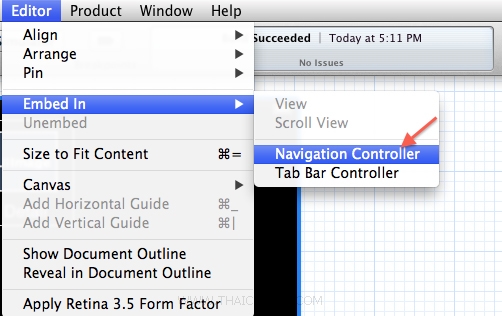
ให้เราสร้าง Navigation Controller ให้กับ Collection View โดยการเลือกที่ Editor -> Embed in -> Navigation Controller
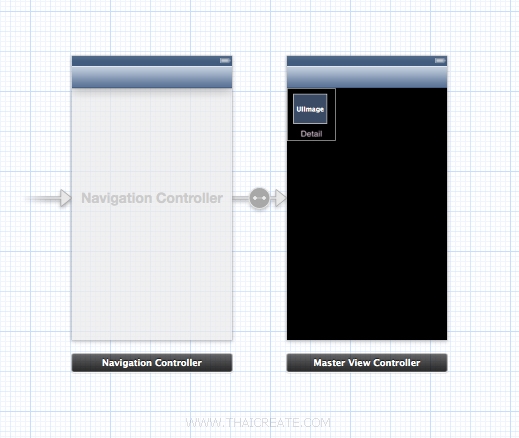
จะได้ Navigation Controller ดังรูป
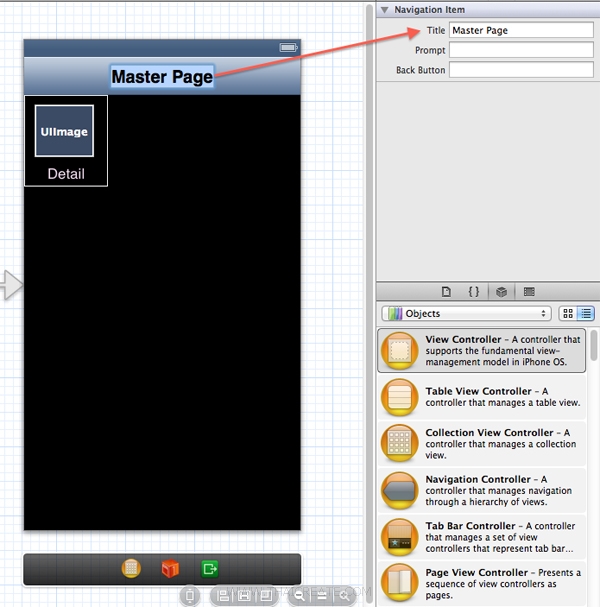
ใส่ชื่อ Title Bar ให้กับ View โดยตั้งชื่อเป็น Master Page
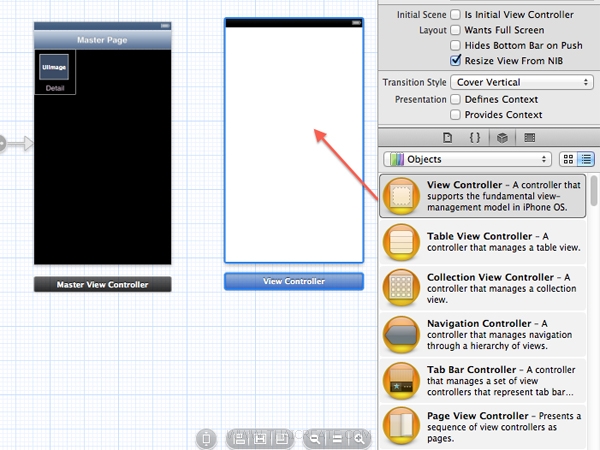
สร้าง View Controller ขึ้นมาอีก 1 ตัว เราจะเรียกว่า View 2
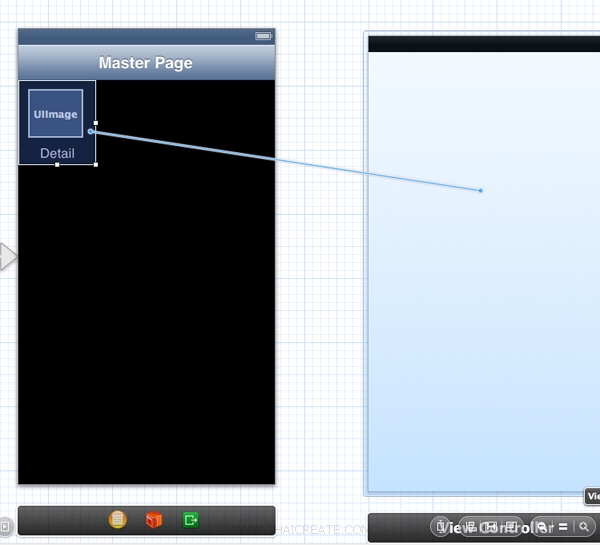
ทำการเชื่อมโยง Action จาก Collection View Cell มายัง View 2
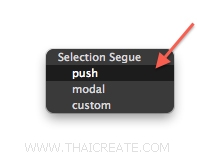
Selection Segue แบบ push
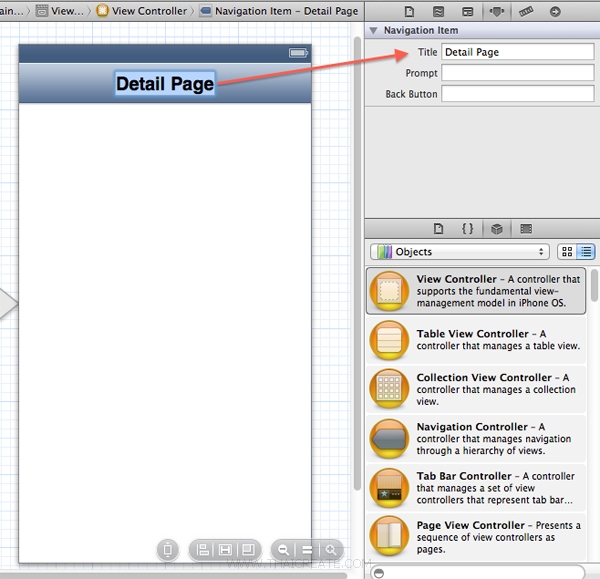
ใน View 2 จะได้ Navigation Controller เช่นเดียวกัน เปลี่ยนชื่อ Title ให้เป็น Detail Page
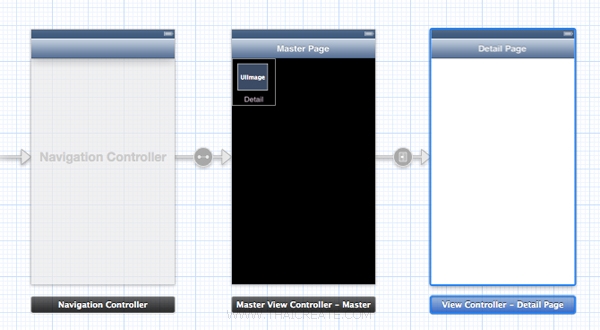
ตอนนี้บน Storyboard ทั้งหมดจะได้ดังรูป
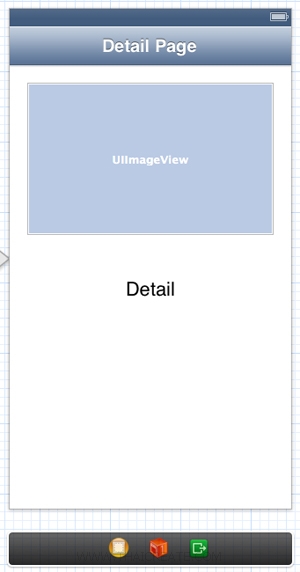
ทำการสร้าง Object ของ Image View และ Label บน View 2
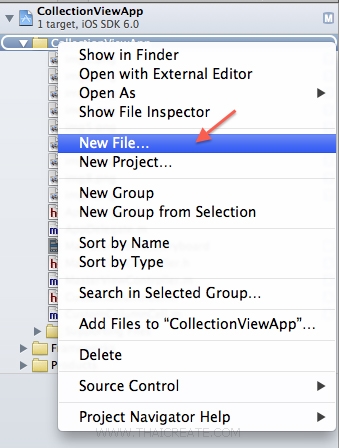
ให้ทำการ New File.. ขึ้นมา 1 ชุด เราจะสร้าง Class ให้กับ View 2
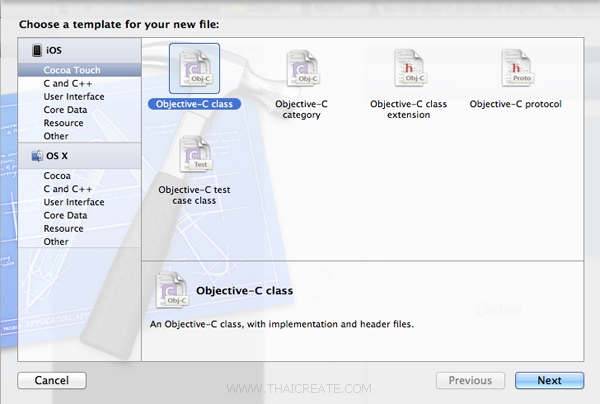
เลือกเป็นแบบ Objective-C class
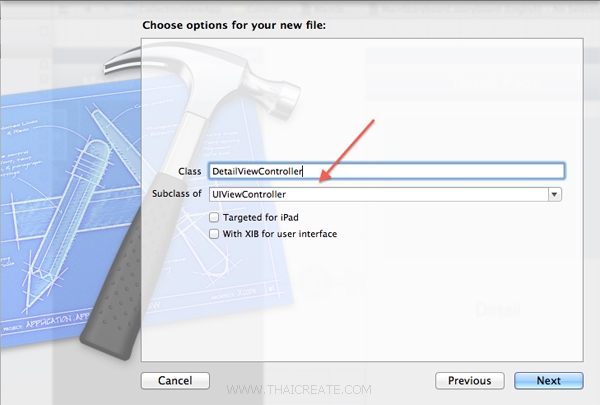
ตั้งชื่อเป็น DetailViewController และเลือก Subclass เป็น UIViewController
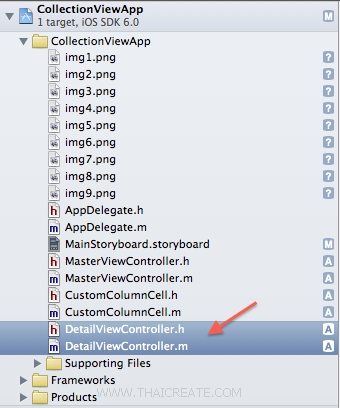
ได้ไฟล์ Class ขึ้นมา 1 ชุด
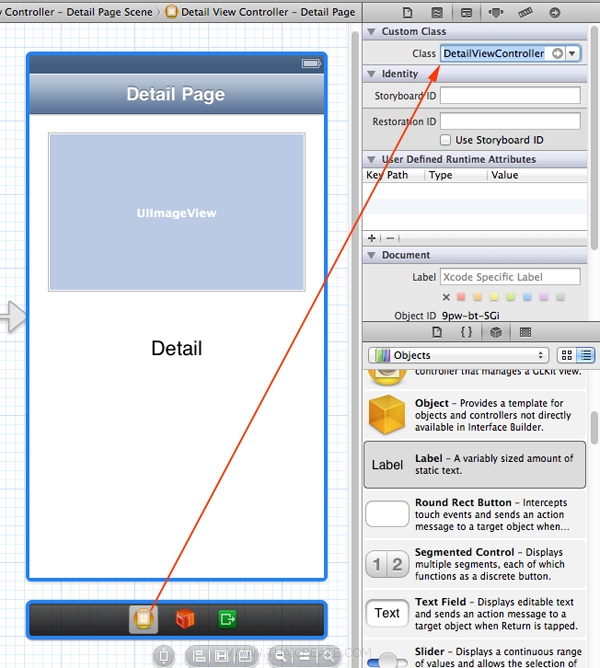
กำหนด Custom Class ของ View 2 เป็น DetailViewController
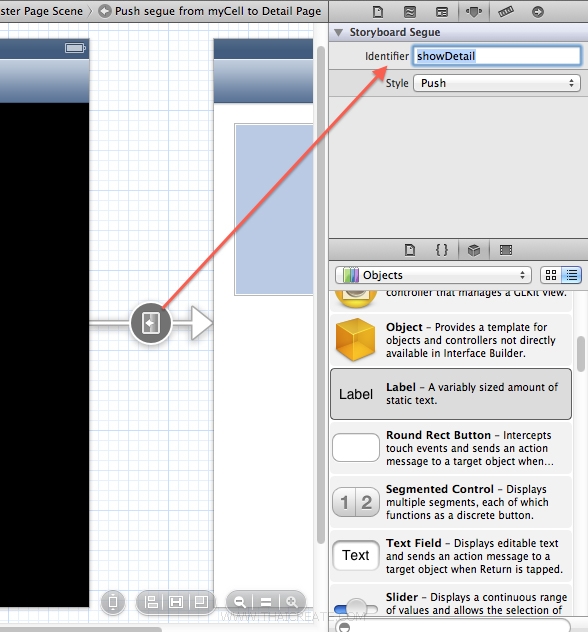
คลิกที่เส้นของ Segue ให้กำหนดชื่อ identifier เป็น showDetail
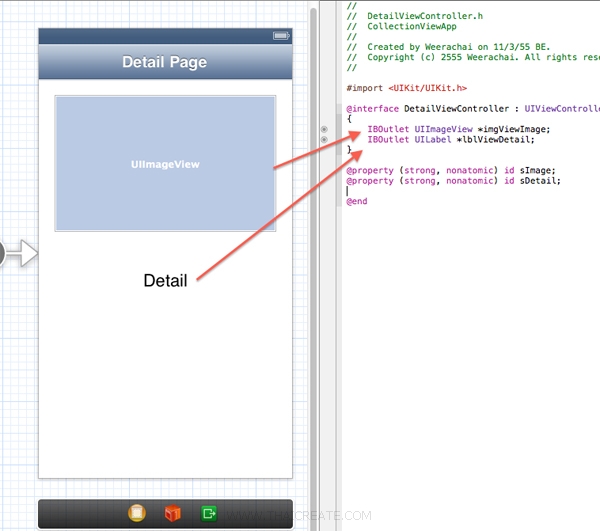
เสร็จแล้วให้ทำการสร้าง IBOutlet ดังรูป
ส่วนที่เหลือจะเป็น Code ทั้งหมด
CustomColumnCell.h
//
// CustomColumnCell.h
//
// Created by Weerachai on 11/3/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface CustomColumnCell : UICollectionViewCell
@property (nonatomic, strong) IBOutlet UIImageView *displayImage;
@property (nonatomic, strong) IBOutlet UILabel *displayDetail;
@end
CustomColumnCell.m
//
// CustomColumnCell.m
//
// Created by Weerachai on 11/3/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "CustomColumnCell.h"
@implementation CustomColumnCell
@synthesize displayImage = _displayImage;
@synthesize displayDetail = _displayDetail;
- (id)initWithFrame:(CGRect)frame
{
self = [super initWithFrame:frame];
if (self) {
// Initialization code
}
return self;
}
/*
// Only override drawRect: if you perform custom drawing.
// An empty implementation adversely affects performance during animation.
- (void)drawRect:(CGRect)rect
{
// Drawing code
}
*/
@end
MasterViewController.h
//
// MasterViewController.h
//
// Created by Weerachai on 11/3/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface MasterViewController : UICollectionViewController
@end
MasterViewController.m
//
// MasterViewController.m
//
// Created by Weerachai on 11/3/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "MasterViewController.h"
#import "CustomColumnCell.h"
#import "DetailViewController.h"
@interface MasterViewController ()
{
// A array Object
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *images;
NSString *detail;
}
@end
@implementation MasterViewController
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view.
// Define keys
images = @"Images";
detail = @"Detail";
myObject = [[NSMutableArray alloc] init];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img1.png", images,
@"Picture 1", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img2.png", images,
@"Picture 2", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img3.png", images,
@"Picture 3", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img4.png", images,
@"Picture 4", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img5.png", images,
@"Picture 5", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img6.png", images,
@"Picture 6", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img7.png", images,
@"Picture 7", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img8.png", images,
@"Picture 8", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img9.png", images,
@"Picture 9", detail,
nil];
[myObject addObject:dict];
}
-(NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section
{
return myObject.count;
}
-(UICollectionViewCell *)collectionView:(UICollectionView *)collectionView
cellForItemAtIndexPath:(NSIndexPath *)indexPath
{
CustomColumnCell *myCell = [collectionView
dequeueReusableCellWithReuseIdentifier:@"myCell"
forIndexPath:indexPath];
NSDictionary *tmpDict = [myObject objectAtIndex:indexPath.row];
UIImage* theImage = [UIImage imageNamed:[tmpDict objectForKey:images]];
myCell.displayImage.image = theImage;
myCell.displayDetail.text= [tmpDict objectForKey:detail];
return myCell;
}
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender
{
if ([[segue identifier] isEqualToString:@"showDetail"]) {
NSIndexPath *IndexPath = [[self.collectionView indexPathsForSelectedItems] objectAtIndex:0];
NSDictionary *tmpDict = [myObject objectAtIndex:IndexPath.row];
[[segue destinationViewController] setImageItem:[tmpDict objectForKey:images]];
[[segue destinationViewController] setDetailItem:[tmpDict objectForKey:detail]];
}
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
@end
DetailViewController.h
//
// DetailViewController.h
//
// Created by Weerachai on 11/3/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface DetailViewController : UIViewController
{
IBOutlet UIImageView *imgViewImage;
IBOutlet UILabel *lblViewDetail;
}
@property (strong, nonatomic) id sImage;
@property (strong, nonatomic) id sDetail;
-(void) setImageItem:(id) newImage;
-(void) setDetailItem:(id) newDetail;
@end
DetailViewController.m
//
// DetailViewController.m
//
// Created by Weerachai on 11/3/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "DetailViewController.h"
@interface DetailViewController ()
@end
@implementation DetailViewController
@synthesize sImage;
@synthesize sDetail;
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view.
UIImage* theImage = [UIImage imageNamed:[sImage description]];
imgViewImage.image = theImage;
lblViewDetail.text = [sDetail description];
}
- (void)setImageItem:(id)newImage
{
sImage = newImage;
}
- (void)setDetailItem:(id)newDetail
{
sDetail = newDetail;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[imgViewImage release];
[lblViewDetail release];
[super dealloc];
}
@end
สำหรับ Code ทั้งหมดเป็นการ Apply มาจากการส่งค่าระหว่าง View สามารถดูแล้วเข้าใจได้โดยไม่ยาก
iOS/iPhone Passing Data Between View (Objective-C,iPhone,iPad)
iOS/iPhone Storyboard and Passing Data Between View (Objective-C,iPhone)
Screenshot
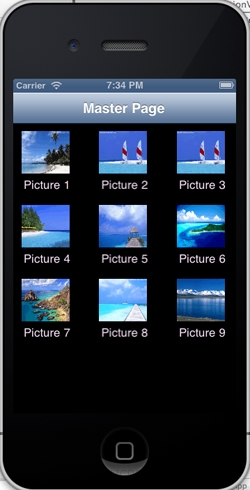
แสดงหน้าแรกของ App บน Collection View ซึ่งแบ่งเป็น Column ต่าง ๆ
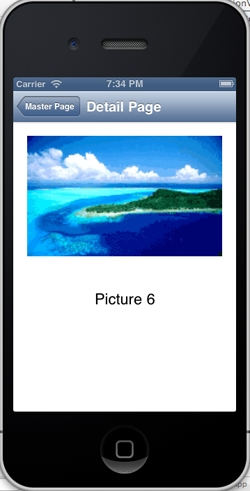
แสดง Detail ของแต่ล่ะ Item
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-11-07 10:37:09 /
2017-03-25 23:52:51 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|