iOS/iPhone Collection View (UICollectionViewController) Multiple Column Item (iPhone, iPad) |
iOS/iPhone Collection View (UICollectionViewController) Multiple Column Item (iPhone, iPad) ในการเขียน iOS บน iPhone และ iPad ที่จะแสดงข้อมูลในรูปแบบของ Column เช่น Gallery รูปภาพ เราไม่สามารถใช้ Table View ได้ เพระา Table View จะมอง 1 Rows คือ 1 Index ข้อมูลเท่านั้น แต่เราจะใช้ Object ที่มีชื่อว่า Collection View (UICollectionViewController) โดยจะทำงานรวมกับ Collection View Cell (UICollectionViewCell) การทำงานของ Collection View คือ จะอ่านข้อมูลที่อยู่ในรูปแบบของ Array ในรูปแบบต่าง ๆ และทำการ Loop แสดงรายการข้อมูลตาม Collection View Cell ที่ได้สร้างขึ้นมา โดยสามารถ Loop แสดงได้ทั้งแบบ แนวนอน และแนวตั้ง
iOS/iPhone Collection View (UICollectionViewController) Multiple Column Item
ในการสร้าง Collection View เราจะต้องออกแบบ Collection View Cell ซึ่งตัวนี้จะมาพร้อมกับ Collection View ที่ได้จากการสร้างบน Storyboard และเราจะต้องสร้าง Class ควบคุมการทำงานทั้ง Collection View และ Collection View Cell ดูตัวอย่างได้ดังนี้
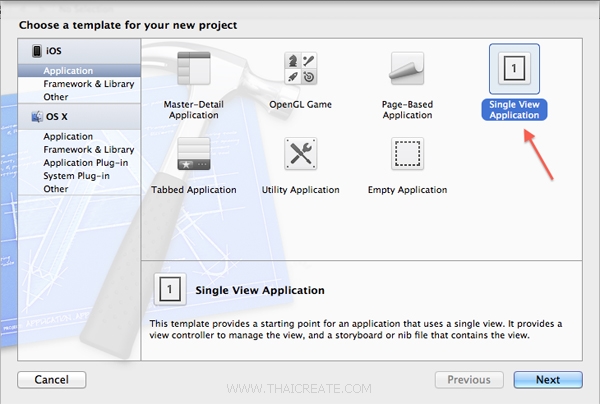
เริ่มต้นด้วยการสร้าง Project บน Xcode แบบ Single View Application
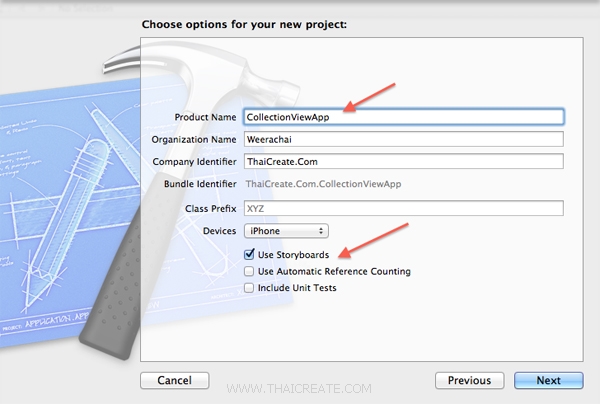
เลือกใช้ Use Storyboard
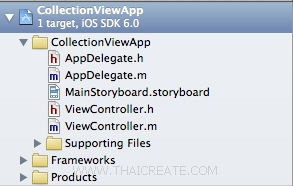
โครงสร้างไฟล์ที่ได้
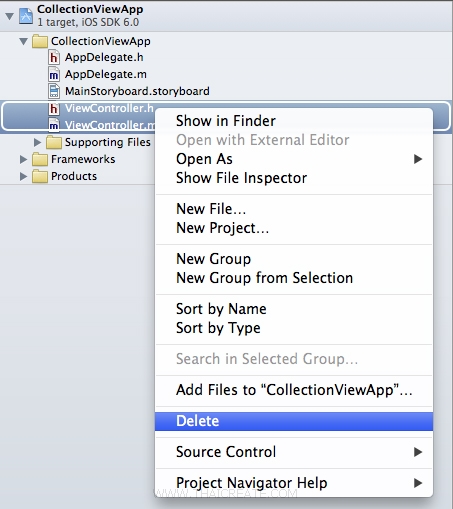
เพิ่อความไม่สับสนจะลบไฟล์ 2 ตัวนี้ทิ้ง ViewController.h และ ViewController.m
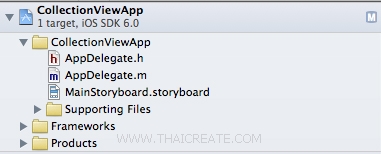
โครงสร้างไฟล์ปัจจุบัน
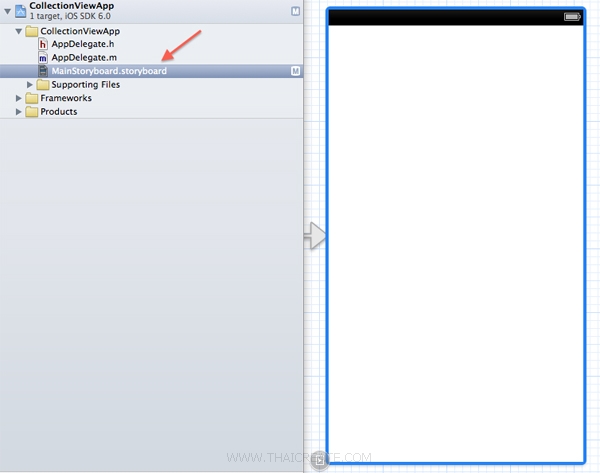
กลับมาที่ Storyboard ตอนนี้จะมี View ที่เป็น Default อยู่ 1 ตัว ให้ทำการลบ View นี้ทิ้งได้เลย
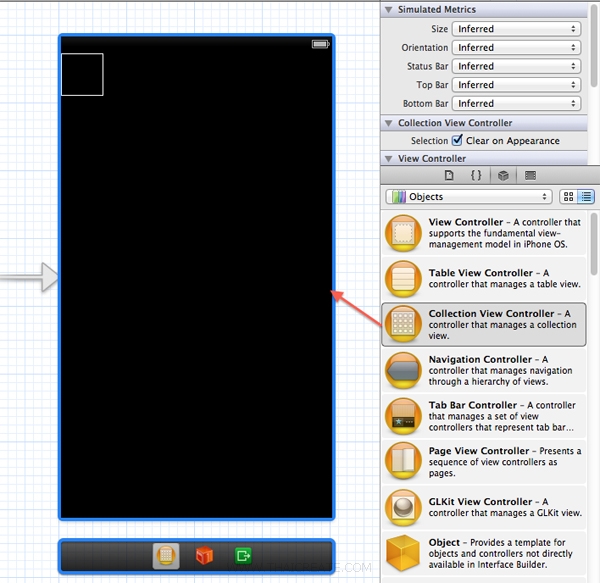
จากนั้นลาก Object ที่มีชื่อว่า Collection View Controller มาวางไว้ที่ Storyboard เราจะเห็นว่าตอนนี้มี Collection View และ Collection View Cell (กล่องเล็ก ๆ สีเหลี่ยม ได้ถูกสร้างมาให้พร้อมในทันที)
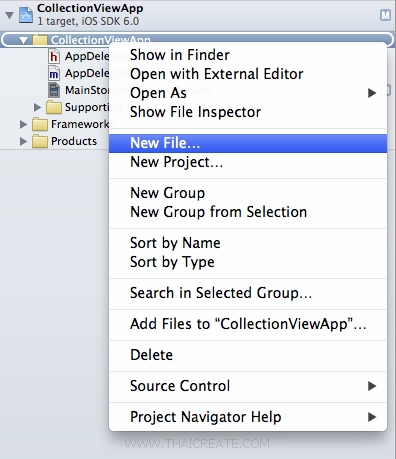
ทำการ New Files... ขึ้นมา
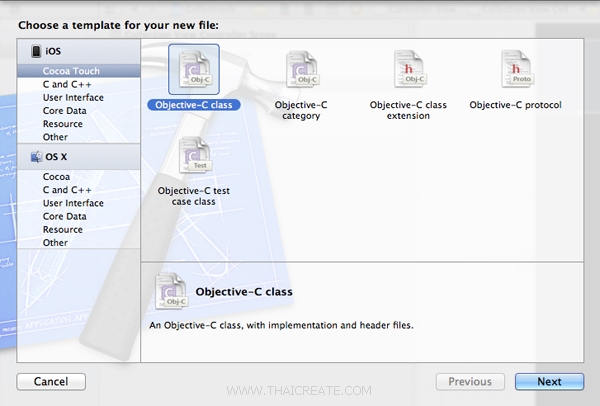
เลือก Objective-C class
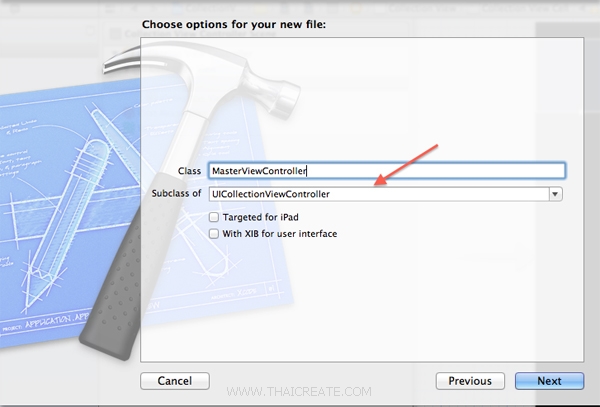
ตั้งชื่อเป็น MasterViewController และเลือก Subclass เป็น UICollectionViewController
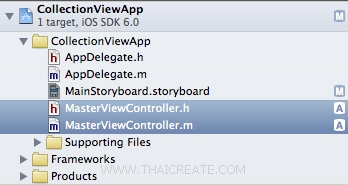
ได้ไฟล์ MasterViewController.h และ MasterViewController.m
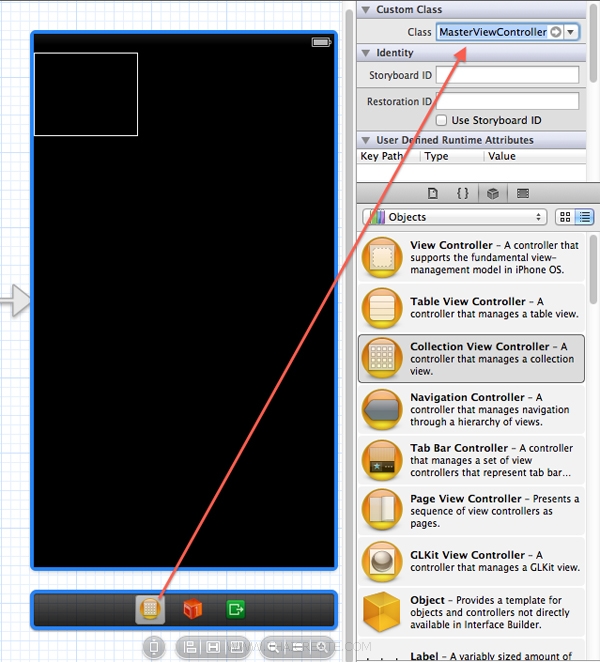
ทำการ Map Custom Class ของ Collection View กับ MasterViewController
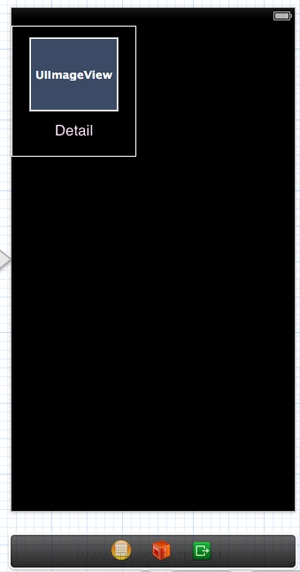
กลับมาที่ Collection View Cell ที่อยู่ภายใน Collection View ให้ลาก Object ของ Image และ Label ไปใส่ดังรูป
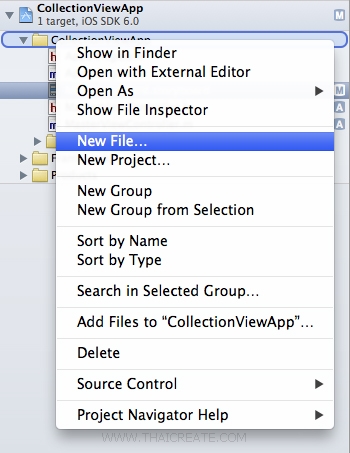
จากนั้นให้ทำการ New File.. ขึ้นมาใหม่
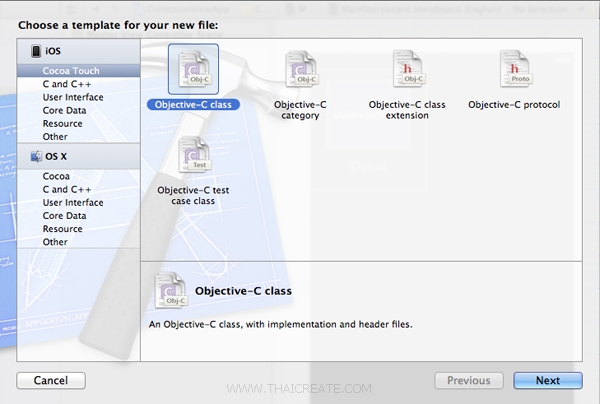
เลือกเป็น Objective-C class
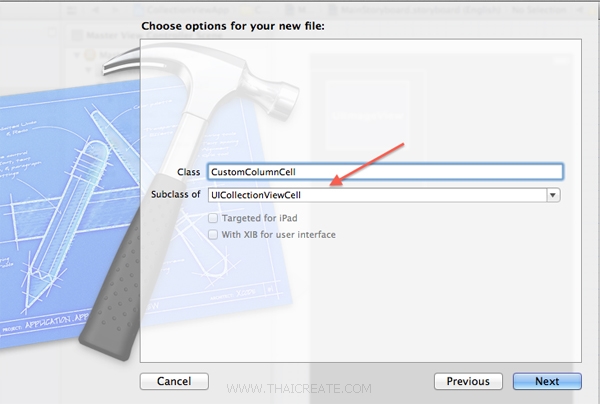
ตั้งชื่อเป็น CustomColumnCell และเลือก Subclass เป็น UICollectionViewCell

ได้ไฟล์ UICollectionViewCell.h และ UICollectionViewCell.m
โดยในไฟล์ UICollectionViewCell.h และ UICollectionViewCell.m ประกาศค่าต่าง ๆ ดังนี้
UICollectionViewCell.h
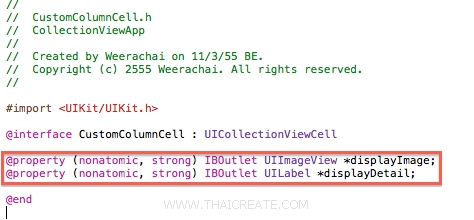
UICollectionViewCell.h
//
// CustomColumnCell.h
//
// Created by Weerachai on 11/3/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface CustomColumnCell : UICollectionViewCell
@property (nonatomic, strong) IBOutlet UIImageView *displayImage;
@property (nonatomic, strong) IBOutlet UILabel *displayDetail;
@end
UICollectionViewCell.m
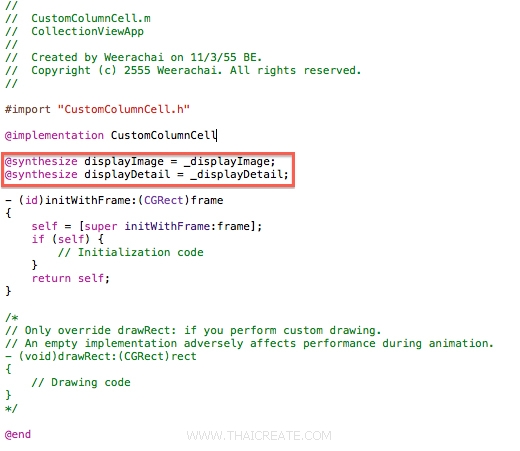
UICollectionViewCell.m
//
// CustomColumnCell.m
//
// Created by Weerachai on 11/3/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "CustomColumnCell.h"
@implementation CustomColumnCell
@synthesize displayImage = _displayImage;
@synthesize displayDetail = _displayDetail;
- (id)initWithFrame:(CGRect)frame
{
self = [super initWithFrame:frame];
if (self) {
// Initialization code
}
return self;
}
/*
// Only override drawRect: if you perform custom drawing.
// An empty implementation adversely affects performance during animation.
- (void)drawRect:(CGRect)rect
{
// Drawing code
}
*/
@end
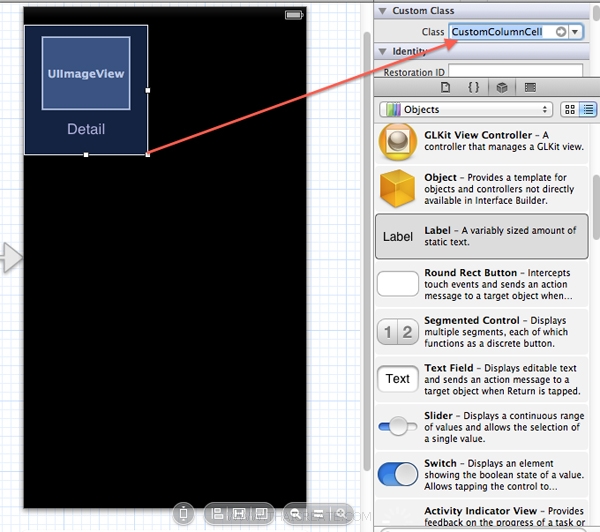
จากนั้นคลิกที่ Collection View Cell ให้กำหนด Custom Class เป็น CustomColumnCell
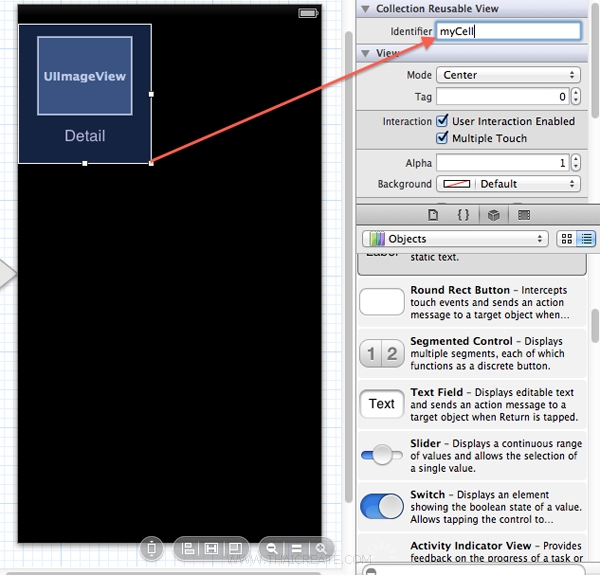
ตั้งชื่อ identifier ให้เป็น myCell ด้วย
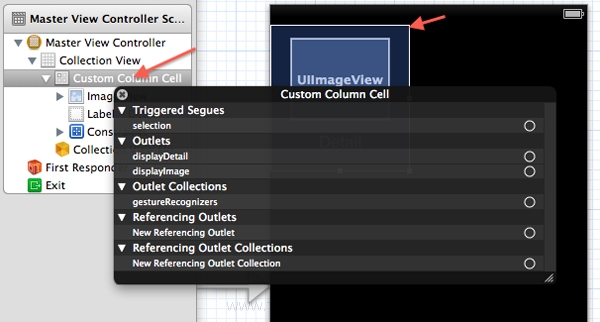
คลิกวาที่ Custom Column Cell หรือคลิกขวาที่กล่องของ Collection View Cell ซึ่งจะมีกล่องปรากฏดังรูป
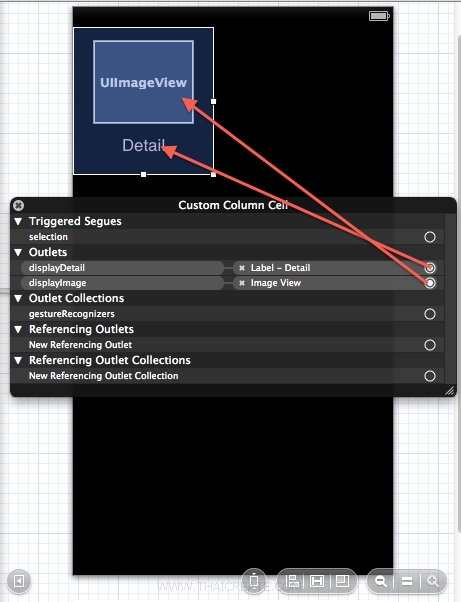
ทำการเชื่อม IBOutlet ของ Object ทั้ง Image และ Label จากนั้นก็จะเสร็จสิ้นขั้นตอนการสร้าง Collection View Cell
เนื่องจากตัวยอ่างนี้เราจะแสดงรูปภาพด้วย ก็ให้ Add เข้ามาใน Project
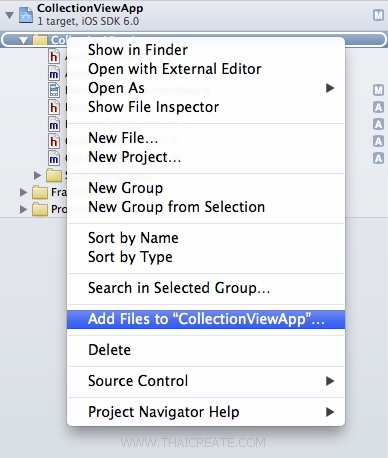
ทำการ Add Files.. เข้ามาใน Project
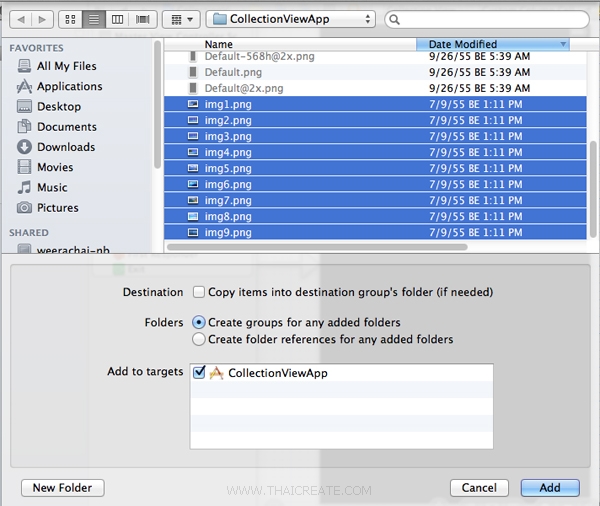
เลือกไฟล์รูปภาพ
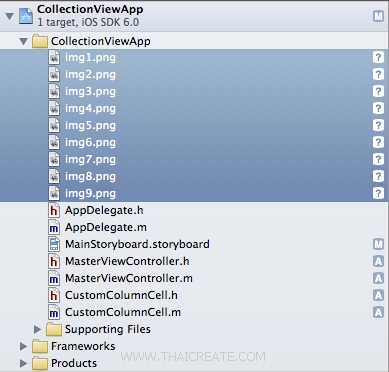
ได้ไฟล์รุปภาพเรียบร้อย ที่เหลือจะเป็น Code ของ MasterViewController.h และ MasterViewController.m สำหรับการเรียกข้อมูลมาแสดงบน Collection View
MasterViewController.h
//
// MasterViewController.h
//
// Created by Weerachai on 11/3/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface MasterViewController : UICollectionViewController
@end
MasterViewController.m
//
// MasterViewController.m
//
// Created by Weerachai on 11/3/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "MasterViewController.h"
#import "CustomColumnCell.h"
@interface MasterViewController ()
{
// A array Object
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *images;
NSString *detail;
}
@end
@implementation MasterViewController
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view.
// Define keys
images = @"Images";
detail = @"Detail";
// Create array to hold dictionaries
myObject = [[NSMutableArray alloc] init];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img1.png", images,
@"Picture 1", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img2.png", images,
@"Picture 2", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img3.png", images,
@"Picture 3", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img4.png", images,
@"Picture 4", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img5.png", images,
@"Picture 5", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img6.png", images,
@"Picture 6", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img7.png", images,
@"Picture 7", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img8.png", images,
@"Picture 8", detail,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"img9.png", images,
@"Picture 9", detail,
nil];
[myObject addObject:dict];
}
-(NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section
{
return myObject.count;
}
-(UICollectionViewCell *)collectionView:(UICollectionView *)collectionView
cellForItemAtIndexPath:(NSIndexPath *)indexPath
{
CustomColumnCell *myCell = [collectionView
dequeueReusableCellWithReuseIdentifier:@"myCell"
forIndexPath:indexPath];
NSDictionary *tmpDict = [myObject objectAtIndex:indexPath.row];
UIImage* theImage = [UIImage imageNamed:[tmpDict objectForKey:images]];
myCell.displayImage.image = theImage;
myCell.displayDetail.text= [tmpDict objectForKey:detail];
return myCell;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
@end
สำหรับ Code ลองไล่ดูครับ ไม่ยาก
Screenshot
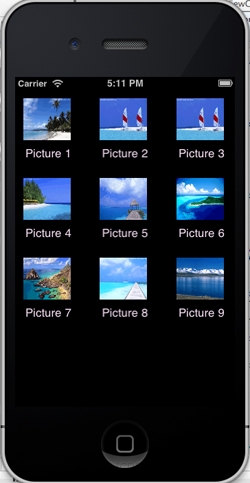
แสดงข้อมูลแบบ Column บน Collection View
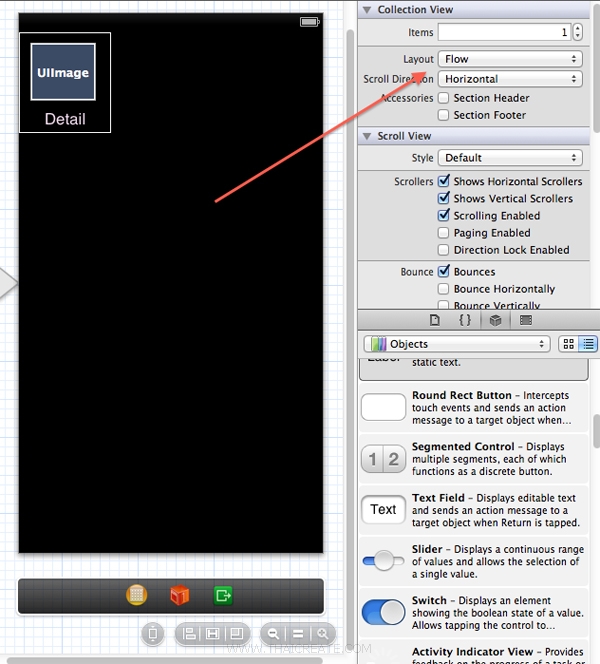
ในกรณีที่ต้องการปรับแต่งแบบแนวตั้ง Sroll Direction เป็นแบบ Horizontal
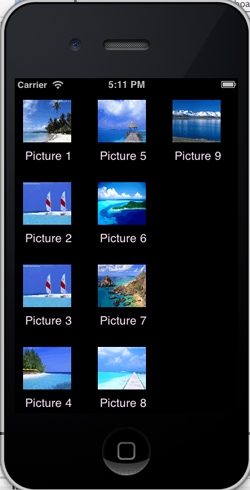
จัดเรียงข้อมูลแบบ แนวตั้ง
เพิ่มเติม
สำหรับการกำหนดจำนวน Column ในแต่ล่ะ Rows นั้น จะขึ้นอยู่กับขนาดความกว้างของ Collection View Cell
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-11-07 10:36:57 /
2017-03-25 23:55:31 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|
|