iOS/iPhone Date Time and Date Format (NSDate, Objective-C) |
iOS/iPhone Date Time and Date Format (NSDate, Objective-C) บทความนี้จะเป็นการเขียน App บน iOS ด้วย Objective-C เกี่ยวกับ NSDate (วันที่ และเวลา) เช่น การอ่านค่าวันที่ของเครื่อง SmartPhone การแปลงวันที่จาก NSString และการอ่านข้อมูล เช่น วัน เดือน ปี พร้อม ๆ กับแสดงข้อความต่าง ๆ บน Label
iOS/iPhone Date Time and Date Format (NSDate, Objective-C)
บน Objective-C จะใช้ Class ของ NSDate ในการจัดการข้อมูลเกี่ยวกับวันที่ และจะมี Class อื่น ๆ มาเกี่ยวข้องเช่น NSTimeZone (กำหนดโซนประเทศ) , NSDateFormatter (กำหนดรูปแบบ Format), NSCalendar (อ่านข้อมูลในรูปแบบปฏิทิน) ลองมาดูตัวอย่างง่าย ๆ
NSDate อ่านข้อมูลปัจจุบันของเครื่อง
NSDateFormatter *formatter = [[NSDateFormatter alloc] init];
[formatter setDateFormat:@"YYYY-MM-dd HH:mm:SS"];
//Optionally for time zone converstions
[formatter setTimeZone:[NSTimeZone timeZoneWithName:@"Asia/Bangkok"]];
NSString *stringFromDate = [formatter stringFromDate:[NSDate date]];
lblDateTime.text = stringFromDate;
[formatter release];
NSDate อ่าน วัน เดือน ปี จากข้อความ NSString
NSString *currentDateString = @"2012-12-07 08:16:45";
NSDateFormatter *dateFormater = [[NSDateFormatter alloc] init];
[dateFormater setTimeZone:[NSTimeZone timeZoneWithName:@"Asia/Bangkok"]];
[dateFormater setDateFormat:@"YYYY-MM-dd HH:mm:ss"];
NSDate *currentDate = [dateFormater dateFromString:currentDateString];
NSCalendar* calendar = [NSCalendar currentCalendar];
NSDateComponents* compoNents = [calendar components:NSYearCalendarUnit|NSMonthCalendarUnit|NSDayCalendarUnit
|NSHourCalendarUnit|NSMinuteCalendarUnit|NSSecondCalendarUnit
fromDate:currentDate];
// Year
NSMutableString *yy = [NSString stringWithFormat:@"Year = %d",[compoNents year]];
lblYear.text = yy;
// Month
NSMutableString *mm = [NSString stringWithFormat:@"Month = %d",[compoNents month]];
lblMonth.text = mm;
// Day
NSMutableString *dd = [NSString stringWithFormat:@"Day = %d",[compoNents day]];
lblDay.text = dd;

Example การใช้งาน NSDate ในรูปแบบต่าง ๆ แบบง่าย ๆ
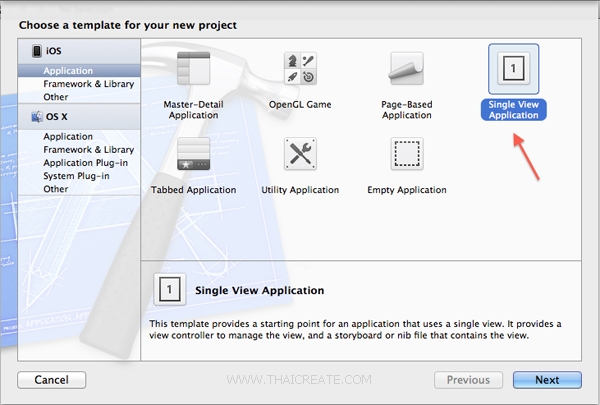
เริ่มต้นด้วยการสร้าง Application บน Xcode แบบ Single View Application แบบง่าย ๆ
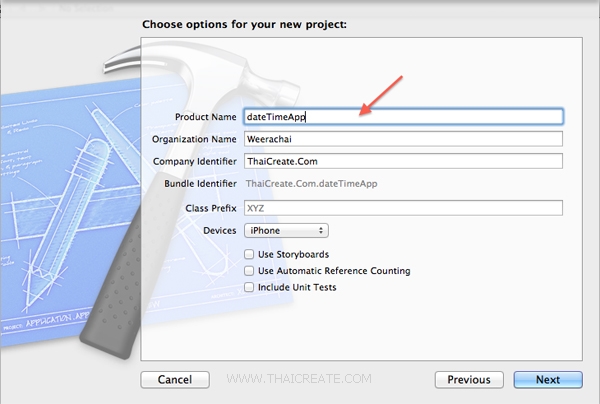
เลือกและไม่เลือกดังรูป
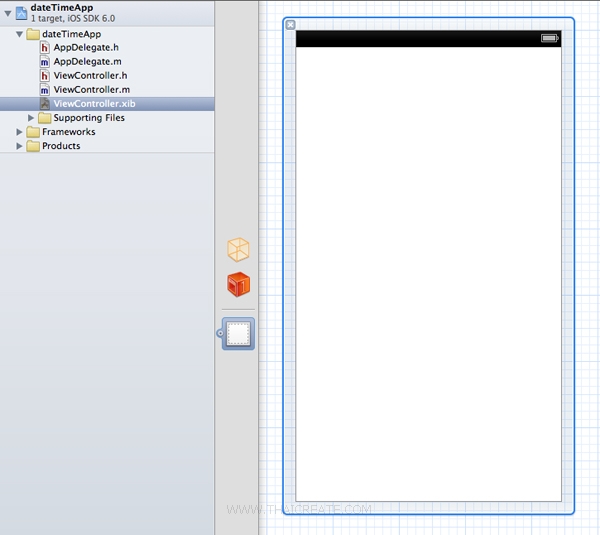
ตอนนี้หน้าจอ View ยังว่าง ๆ
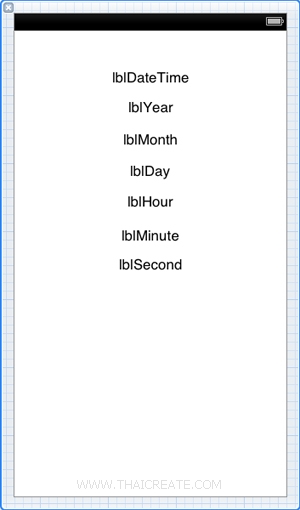
ออกแบบ Label สำหรับแสดงผลต่าง ๆ ดังรูป
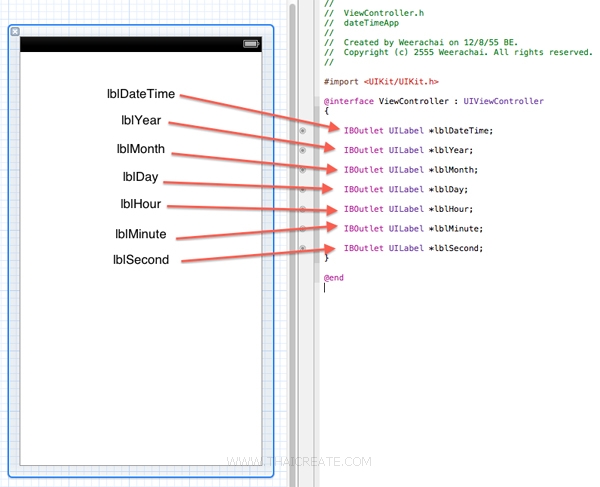
ใน Class ของ .h ให้ทำการเชื่อม IBOutlet ต่าง ๆ ดังรูป จากนั้นเขียน Code ทั้งหมดตามนี้
ViewController.h
//
// ViewController.h
// dateTimeApp
//
// Created by Weerachai on 12/8/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
{
IBOutlet UILabel *lblDateTime;
IBOutlet UILabel *lblYear;
IBOutlet UILabel *lblMonth;
IBOutlet UILabel *lblDay;
IBOutlet UILabel *lblHour;
IBOutlet UILabel *lblMinute;
IBOutlet UILabel *lblSecond;
}
@end
ViewController.m
//
// ViewController.m
// dateTimeApp
//
// Created by Weerachai on 12/8/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "ViewController.h"
@interface ViewController ()
@end
@implementation ViewController
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
NSDateFormatter *formatter = [[NSDateFormatter alloc] init];
[formatter setDateFormat:@"YYYY-MM-dd HH:mm:SS"];
//Optionally for time zone converstions
[formatter setTimeZone:[NSTimeZone timeZoneWithName:@"Asia/Bangkok"]];
NSString *stringFromDate = [formatter stringFromDate:[NSDate date]];
lblDateTime.text = stringFromDate;
[formatter release];
/*
// Get date by Current
NSDate *curentDate = [NSDate date];
NSCalendar* calendar = [NSCalendar currentCalendar];
NSDateComponents* compoNents = [calendar components:NSYearCalendarUnit|NSMonthCalendarUnit|NSDayCalendarUnit
|NSHourCalendarUnit|NSMinuteCalendarUnit|NSSecondCalendarUnit
fromDate:curentDate];
*/
// Get date by Sting
NSString *currentDateString = @"2012-12-07 08:16:45";
NSDateFormatter *dateFormater = [[NSDateFormatter alloc] init];
[dateFormater setTimeZone:[NSTimeZone timeZoneWithName:@"Asia/Bangkok"]];
[dateFormater setDateFormat:@"YYYY-MM-dd HH:mm:ss"];
NSDate *currentDate = [dateFormater dateFromString:currentDateString];
NSCalendar* calendar = [NSCalendar currentCalendar];
NSDateComponents* compoNents = [calendar components:NSYearCalendarUnit|NSMonthCalendarUnit|NSDayCalendarUnit
|NSHourCalendarUnit|NSMinuteCalendarUnit|NSSecondCalendarUnit
fromDate:currentDate];
// Year
NSMutableString *yy = [NSString stringWithFormat:@"Year = %d",[compoNents year]];
lblYear.text = yy;
// Month
NSMutableString *mm = [NSString stringWithFormat:@"Month = %d",[compoNents month]];
lblMonth.text = mm;
// Day
NSMutableString *dd = [NSString stringWithFormat:@"Day = %d",[compoNents day]];
lblDay.text = dd;
// Hour
NSMutableString *h = [NSString stringWithFormat:@"Hour = %d",[compoNents hour]];
lblHour.text = h;
// Minute
NSMutableString *m = [NSString stringWithFormat:@"Minute = %d",[compoNents minute]];
lblMinute.text = m;
// Second
NSMutableString *s = [NSString stringWithFormat:@"Second = %d",[compoNents second]];
lblSecond.text = s;
/*
NSString* input = @"2012-12-07 08:16:45";
NSString* year = [input substringWithRange: NSMakeRange( 0, 4)];
NSString* month = [input substringWithRange: NSMakeRange( 5, 2)];
NSString* day = [input substringWithRange: NSMakeRange( 8, 2)];
NSString* time = [input substringWithRange: NSMakeRange(11, 8)];
NSString* output = [NSString stringWithFormat:@"%@.%@.%@ %@",day,month,year,time]
*/
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[lblDateTime release];
[lblYear release];
[lblMonth release];
[lblDay release];
[lblHour release];
[lblMinute release];
[lblSecond release];
[super dealloc];
}
@end
Screenshot
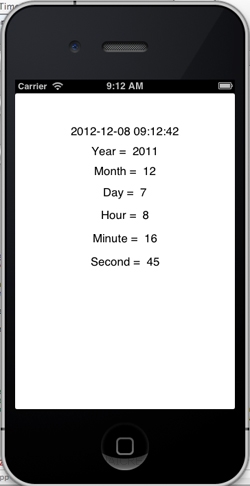
แสดงวันที่จาก NSDate และการแปลง NSString ให้เป็น NSDate และการตัดอ่านค่า วัน เดือน ปี ชม. นาที วินาที จาก String ข้อความ
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-12-12 14:06:34 /
2017-03-25 22:46:23 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|
|