iOS/iPhone Edit Update Data on Web Server (URL,Website) |
iOS/iPhone Edit Update Data on Web Server (URL,Website) หลังจากบทความ iOS ก่อนหน้านี้เป็นการส่งข้อมูลเพื่อไปบันทึกข้อมูลลงไปใน Web Server สำหรับบทความนี้เราจะมาใช้การดึงข้อมูลที่อยู่บน Web Server มาแสดงบน Table View และสามารถคลิกแต่ล่ะรายการเพื่อที่จะแก้ไข หรือ Update รายการนั้น ๆ โดยเราจะยังใช้ PHP กับ MySQL และ JSON เป็นตัวสำหรับรับ-ส่งข้อมูลเช่นเดิม
iOS/iPhone Edit Update Data on Web Server (URL,Website)
หลักการนั้นเราจะสร้างไฟล์ php ขึ้นมา 3 ไฟล์ คือ (1) ไฟล์สำหรับแสดงข้อมูลทั้งหมด , (2) ไฟล์สำหรับแสดงแต่ล่ะรายการ และ (3) ไฟล์สำหรับทับทึการแก้ไข โดยจะได้นำความรู้จากบทความก่อน ๆ หน้านี้มา Apply ใช้กับตัวอย่างนี้ ซึ่งอาจจะมีความซับซ้อนพอสมควร แต่ก็ไม่ยากเกินไปที่จะทำความเข้าใจได้
Example การทำ App เพื่อแก้ไข (Edit/Update) ข้อมูลที่อยู่บน Web Server (PHP MySQL)
MySQL Database
CREATE TABLE `member` (
`MemberID` int(11) NOT NULL auto_increment,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
PRIMARY KEY (`MemberID`)
) ENGINE=MyISAM AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'Weerachai', '0819876107');
INSERT INTO `member` VALUES (2, 'Win', '021978032');
INSERT INTO `member` VALUES (3, 'Eak', '0876543210');
โครงสร้างของฐานข้อมูล MySQL Database
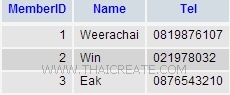
getData.php ไฟล์ php สำหรับแสดงข้อมูลทั้งหมด ซึ่งจะถูกเรียกใช้แสดงผลบน Table View
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM member WHERE 1 ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
getByMemberID.php ไฟล์ php สำหรับเมื่อคลิกเลือกแต่ล่ะรายการของ Cell บน Table View และแสดงข้อมูลสำหรับการแก้ไข
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
//$_POST["sMemberID"] = "1"; // for Sample
$strMemberID = $_POST["sMemberID"];
$strSQL = "SELECT * FROM member WHERE 1 AND MemberID = '".$strMemberID."' ";
$objQuery = mysql_query($strSQL);
$obResult = mysql_fetch_array($objQuery);
if($obResult)
{
$arr["MemberID"] = $obResult["MemberID"];
$arr["Name"] = $obResult["Name"];
$arr["Tel"] = $obResult["Tel"];
}
mysql_close($objConnect);
/*** return JSON by MemberID ***/
/* Eg :
{"MemberID":"1",
"Name":"Win",
"Tel":"0819876107",
*/
echo json_encode($arr);
?>

updateData.php ไฟล์ php สำหรับบันทึกการแก้ไขข้อมูล
<?php
/*** for Sample
$_POST["sMember"] = "1";
$_POST["sName"] = "a";
$_POST["sTel"] = "b";
*/
$strMemberID = $_POST["sMemberID"];
$strName = $_POST["sName"];
$strTel = $_POST["sTel"];
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
/*** Insert ***/
$strSQL = "UDPATE member SET
Name = '".$strName."',
Tel = '".$strTel."'
WHERE MemberID = '".$strMemberID."'
";
$objQuery = mysql_query($strSQL);
$arr = null;
if(!$objQuery)
{
$arr["Status"] = "0";
$arr["Message"] = "Update Data Failed";
}
else
{
$arr["Status"] = "1";
$arr["Message"] = "Update Data Successfully";
}
echo json_encode($arr);
?>
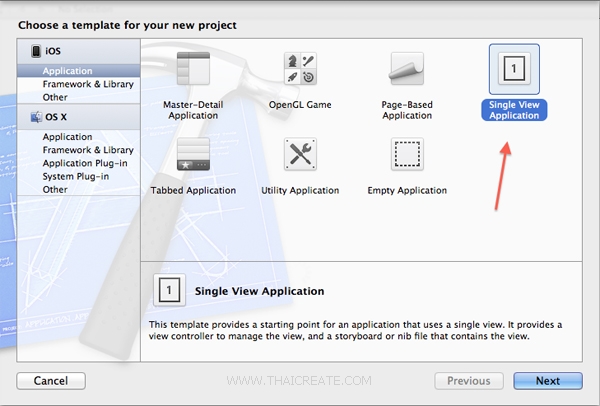
เริ่มต้นด้วยการสร้าง Application บน Xcode แบบ Single View Application
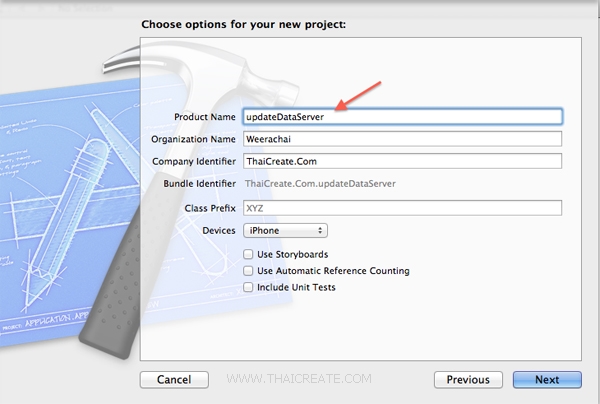
เลือกและไม่เลือกรายการดังรูป
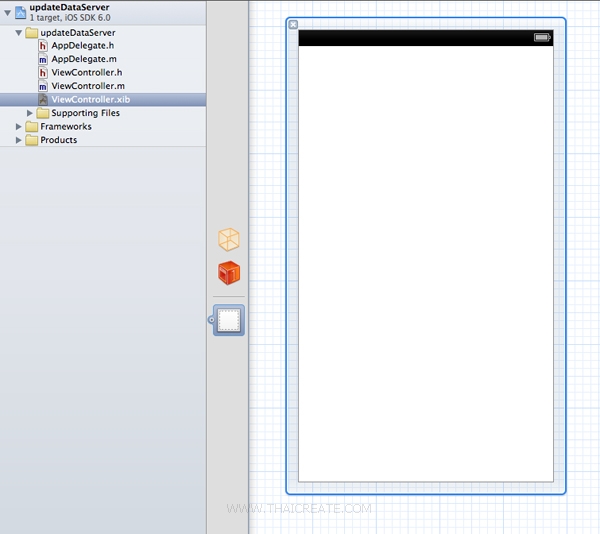
ตอนนี้หน้าจอ View แรกของเรา จะยังว่าง ๆ
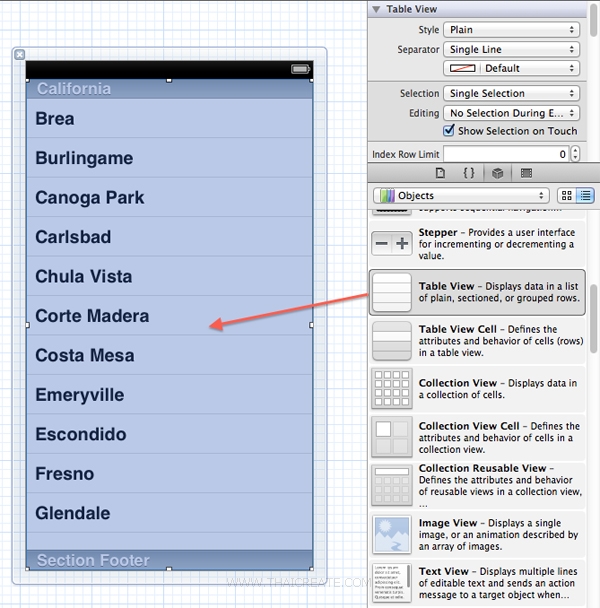
ให้คลิกที่ Table View แล้วลากไปยังหน้าจอ View
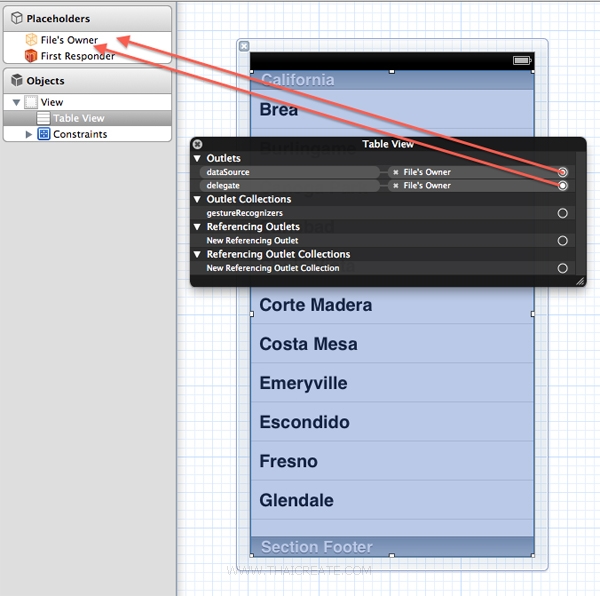
คลิกขวาที่ Table View ให้ทำการเชื่อม dataSource และ delegate กับ File's Owner
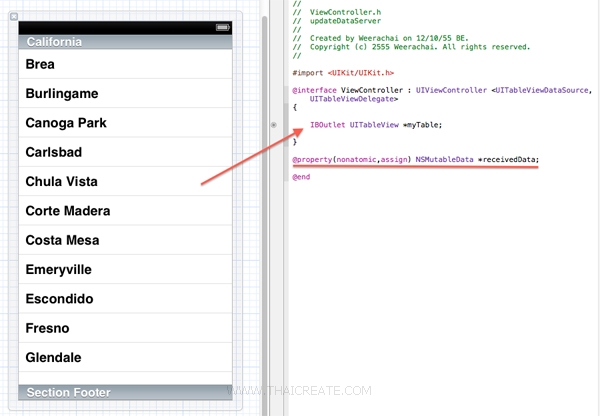
ใน Class ของ .h ให้เชื่อม IBOutlet ดังรูป จากนั้นเขียน Code ต่าง ๆ ดังนี้
ViewController.h
//
// ViewController.h
// updateDataServer
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController <UITableViewDataSource,UITableViewDelegate>
{
IBOutlet UITableView *myTable;
}
@property(nonatomic,assign) NSMutableData *receivedData;
@end
ViewController.m
//
// ViewController.m
// updateDataServer
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "ViewController.h"
#import "DetailViewController.h"
@interface ViewController ()
{
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *memberid;
NSString *name;
NSString *tel;
UIAlertView *loading;
}
@end
@implementation ViewController
@synthesize receivedData;
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
// Define keys
memberid = @"MemberID";
name = @"Name";
tel = @"Tel";
// Create array to hold dictionaries
myObject = [[NSMutableArray alloc] init];
NSURLRequest *theRequest =
[NSURLRequest requestWithURL:[NSURL URLWithString:@"https://www.thaicreate.com/url/getData.php"]
cachePolicy:NSURLRequestReloadIgnoringLocalCacheData
timeoutInterval:10.0];
NSURLConnection *theConnection=[[NSURLConnection alloc] initWithRequest:theRequest delegate:self];
// Loading...
[UIApplication sharedApplication].networkActivityIndicatorVisible = YES;
loading = [[UIAlertView alloc] initWithTitle:@"" message:@"Please Wait..." delegate:nil cancelButtonTitle:nil otherButtonTitles:nil];
UIActivityIndicatorView *progress= [[UIActivityIndicatorView alloc] initWithFrame:CGRectMake(125, 50, 30, 30)];
progress.activityIndicatorViewStyle = UIActivityIndicatorViewStyleWhiteLarge;
[loading addSubview:progress];
[progress startAnimating];
[progress release];
[loading show];
if (theConnection) {
self.receivedData = nil;
} else {
UIAlertView *connectFailMessage = [[UIAlertView alloc] initWithTitle:@"NSURLConnection " message:@"Failed in viewDidLoad" delegate: self cancelButtonTitle:@"Ok" otherButtonTitles: nil];
[connectFailMessage show];
[connectFailMessage release];
}
}
- (void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response
{
receivedData = [[NSMutableData alloc] init];
}
- (void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data
{
sleep(2);
[receivedData appendData:data];
}
- (void)connection:(NSURLConnection *)connection didFailWithError:(NSError *)error
{
[connection release];
[receivedData release];
// inform the user
UIAlertView *didFailWithErrorMessage = [[UIAlertView alloc] initWithTitle: @"NSURLConnection " message: @"didFailWithError" delegate: self cancelButtonTitle: @"Ok" otherButtonTitles: nil];
[didFailWithErrorMessage show];
[didFailWithErrorMessage release];
//inform the user
NSLog(@"Connection failed! Error - %@", [error localizedDescription]);
}
- (void)connectionDidFinishLoading:(NSURLConnection *)connection
{
[UIApplication sharedApplication].networkActivityIndicatorVisible = NO;
[loading dismissWithClickedButtonIndex:0 animated:YES];
if(receivedData)
{
//NSLog(@"%@",receivedData);
//NSString *dataString = [[NSString alloc] initWithData:receivedData encoding:NSASCIIStringEncoding];
//NSLog(@" abc = %@",dataString);
id jsonObjects = [NSJSONSerialization JSONObjectWithData:receivedData options:NSJSONReadingMutableContainers error:nil];
// values in foreach loop
for (NSDictionary *dataDict in jsonObjects) {
NSString *strMemberID = [dataDict objectForKey:@"MemberID"];
NSString *strName = [dataDict objectForKey:@"Name"];
NSString *strTel = [dataDict objectForKey:@"Tel"];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
strMemberID, memberid,
strName, name,
strTel, tel,
nil];
[myObject addObject:dict];
}
[myTable reloadData];
}
// release the connection, and the data object
[connection release];
[receivedData release];
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
int nbCount = [myObject count];
if (nbCount == 0)
return 1;
else
return [myObject count];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
// Use the default cell style.
cell = [[[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier] autorelease];
}
int nbCount = [myObject count];
if (nbCount ==0)
cell.textLabel.text = @"Loading Data";
else
{
NSDictionary *tmpDict = [myObject objectAtIndex:indexPath.row];
// MemberID
NSMutableString *text;
text = [NSString stringWithFormat:@"MemberID : %@",[tmpDict objectForKey:memberid]];
// Name & Tel
NSMutableString *detail;
detail = [NSString stringWithFormat:@"Name : %@ , Tel : %@"
,[tmpDict objectForKey:name]
,[tmpDict objectForKey:tel]];
cell.textLabel.text = text;
cell.detailTextLabel.text= detail;
//[tmpDict objectForKey:memberid]
//[tmpDict objectForKey:name]
//[tmpDict objectForKey:tel]
}
return cell;
}
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
NSDictionary *tmpDict = [myObject objectAtIndex:indexPath.row];
DetailViewController *editForm = [[[DetailViewController alloc] initWithNibName:nil bundle:nil] autorelease];
editForm.sMemberID = [tmpDict objectForKey:memberid];
[self presentViewController:editForm animated:YES completion:NULL];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[myTable release];
[super dealloc];
}
@end

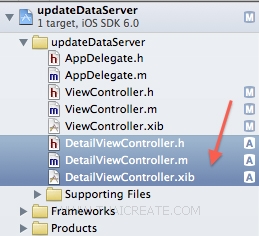
เพิ่มไฟล์ Interface ขึ้นมาอีก 1 ชุดชื่อว่า DetailViewController โดยใช้ Subclass ของ UIViewController ประกอบด้วย .xib , .h และ .m
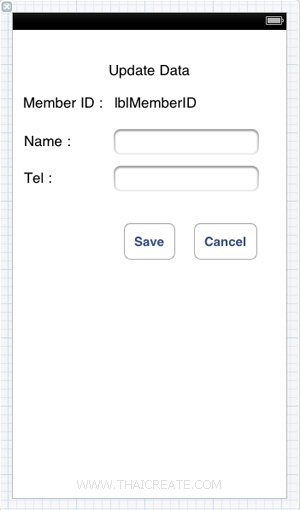
ออกแบบหน้าจอ View ดังรูป
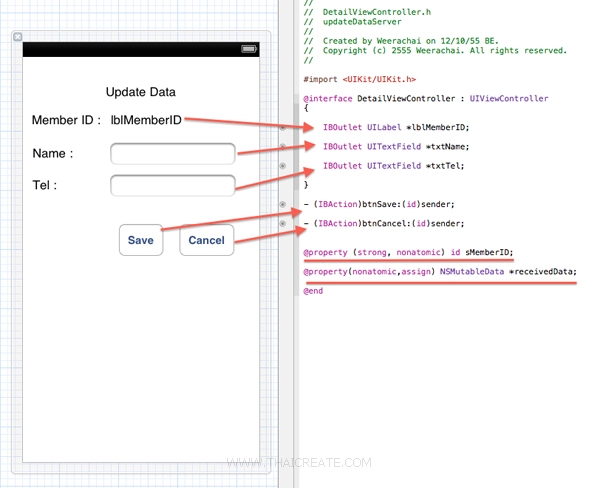
ใน Class ของ .h ให้เชื่อม IBOutlet และ IBAction ต่าง ๆ ดังรูป จากนั้นเขียน Code ดังนี้
DetailViewController.h
//
// DetailViewController.h
// updateDataServer
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface DetailViewController : UIViewController
{
IBOutlet UILabel *lblMemberID;
IBOutlet UITextField *txtName;
IBOutlet UITextField *txtTel;
}
- (IBAction)btnSave:(id)sender;
- (IBAction)btnCancel:(id)sender;
@property (strong, nonatomic) id sMemberID;
@property(nonatomic,assign) NSMutableData *receivedData;
@end
DetailViewController.m
//
// DetailViewController.m
// updateDataServer
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "DetailViewController.h"
#import "ViewController.h"
@interface DetailViewController ()
{
NSString *actionCommand;
}
@end
@implementation DetailViewController
@synthesize receivedData;
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view from its nib.
actionCommand = @"LOAD";
if([actionCommand isEqualToString:@"LOAD"] ){
//sMemberID=1
NSMutableString *post = [NSString stringWithFormat:@"sMemberID=%@",[self.sMemberID description]];
NSData *postData = [post dataUsingEncoding:NSASCIIStringEncoding allowLossyConversion:YES];
NSString *postLength = [NSString stringWithFormat:@"%d", [postData length]];
NSURL *url = [NSURL URLWithString:@"https://www.thaicreate.com/url/getByMemberID.php"];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:url
cachePolicy:NSURLRequestReloadIgnoringLocalCacheData
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setValue:postLength forHTTPHeaderField:@"Content-Length"];
[request setValue:@"application/x-www-form-urlencoded" forHTTPHeaderField:@"Content-Type"];
[request setHTTPBody:postData];
NSURLConnection *theConnection=[[NSURLConnection alloc] initWithRequest:request delegate:self];
if (theConnection) {
self.receivedData = nil;
} else {
UIAlertView *connectFailMessage = [[UIAlertView alloc] initWithTitle:@"NSURLConnection " message:@"Failed in viewDidLoad" delegate: self cancelButtonTitle:@"Ok" otherButtonTitles: nil];
[connectFailMessage show];
[connectFailMessage release];
}
}
}
- (void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response
{
receivedData = [[NSMutableData alloc] init];
}
- (void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data
{
[receivedData appendData:data];
}
- (void)connection:(NSURLConnection *)connection didFailWithError:(NSError *)error
{
[connection release];
[receivedData release];
// inform the user
UIAlertView *didFailWithErrorMessage = [[UIAlertView alloc] initWithTitle: @"NSURLConnection " message: @"didFailWithError" delegate: self cancelButtonTitle: @"Ok" otherButtonTitles: nil];
[didFailWithErrorMessage show];
[didFailWithErrorMessage release];
//inform the user
NSLog(@"Connection failed! Error - %@", [error localizedDescription]);
}
- (void)connectionDidFinishLoading:(NSURLConnection *)connection
{
// Return Status E.g : { "MemberID":"1", "Name":"Win", "Tel":"0819876107" }
if(receivedData)
{
//NSLog(@"%@",receivedData);
//NSString *dataString = [[NSString alloc] initWithData:receivedData encoding:NSASCIIStringEncoding];
//NSLog(@"%@",dataString);
// for Load Data
if([actionCommand isEqualToString:@"LOAD"] ){
id jsonObjects = [NSJSONSerialization JSONObjectWithData:receivedData options:NSJSONReadingMutableContainers error:nil];
// value in key name
NSString *strMemberID = [jsonObjects objectForKey:@"MemberID"];
NSString *strName = [jsonObjects objectForKey:@"Name"];
NSString *strTel = [jsonObjects objectForKey:@"Tel"];
NSLog(@"MemberID = %@",strMemberID);
NSLog(@"Name = %@",strName);
NSLog(@"Tel = %@",strTel);
lblMemberID.text = strMemberID;
txtName.text = strName;
txtTel.text = strTel;
}
// for Save Data
if([actionCommand isEqualToString:@"SAVE"] ){
// Return Status E.g : { "Status":"1", "Message":"Update Data Successfully" }
// 0 = Error
// 1 = Completed
id jsonObjects = [NSJSONSerialization JSONObjectWithData:receivedData options:NSJSONReadingMutableContainers error:nil];
// value in key name
NSString *strStatus = [jsonObjects objectForKey:@"Status"];
NSString *strMessage = [jsonObjects objectForKey:@"Message"];
NSLog(@"Status = %@",strStatus);
NSLog(@"Message = %@",strMessage);
// Completed
if( [strStatus isEqualToString:@"1"] ){
UIAlertView *completed =[[UIAlertView alloc]
initWithTitle:@": -) Completed!"
message:strMessage delegate:self
cancelButtonTitle:@"OK" otherButtonTitles: nil];
[completed show];
}
else // Error
{
UIAlertView *error =[[UIAlertView alloc]
initWithTitle:@": ( Error!"
message:strMessage delegate:self
cancelButtonTitle:@"OK" otherButtonTitles: nil];
[error show];
}
}
}
// release the connection, and the data object
[connection release];
[receivedData release];
}
// Save Command
- (IBAction)btnSave:(id)sender {
actionCommand = @"SAVE";
//sMemberID=1&sName=Win&sTel=0819876107
NSMutableString *post = [NSString stringWithFormat:@"sMemberID=%@&sName=%@&sTel=%@",
[self.sMemberID description],[txtName text],[txtTel text]];
NSData *postData = [post dataUsingEncoding:NSASCIIStringEncoding allowLossyConversion:YES];
NSString *postLength = [NSString stringWithFormat:@"%d", [postData length]];
NSURL *url = [NSURL URLWithString:@"https://www.thaicreate.com/url/updateData.php"];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:url
cachePolicy:NSURLRequestReloadIgnoringLocalCacheData
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setValue:postLength forHTTPHeaderField:@"Content-Length"];
[request setValue:@"application/x-www-form-urlencoded" forHTTPHeaderField:@"Content-Type"];
[request setHTTPBody:postData];
NSURLConnection *theConnection=[[NSURLConnection alloc] initWithRequest:request delegate:self];
if (theConnection) {
self.receivedData = nil;
} else {
UIAlertView *connectFailMessage = [[UIAlertView alloc] initWithTitle:@"NSURLConnection " message:@"Failed in viewDidLoad" delegate: self cancelButtonTitle:@"Ok" otherButtonTitles: nil];
[connectFailMessage show];
[connectFailMessage release];
}
}
// Cancel Command
- (IBAction)btnCancel:(id)sender {
ViewController *main = [[[ViewController alloc] initWithNibName:nil bundle:nil] autorelease];
[self presentViewController:main animated:YES completion:NULL];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[txtName release];
[txtTel release];
[lblMemberID release];
[txtName release];
[super dealloc];
}
@end
สำหรับ Code ทั้งหมด ลองไล่ดูครับ ไม่ยาก เป็นการ Apply จากหลาย ๆ บทความก่อนหน้านี้
Screenshot
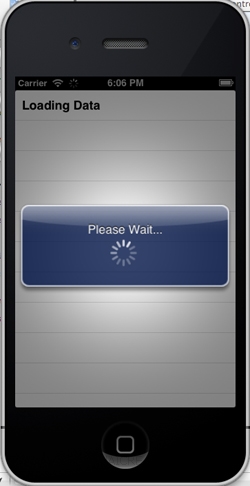
กำลังแสดงข้อมูลโดยจะแสดง Progress แบบ Icons หมุ่น ๆ
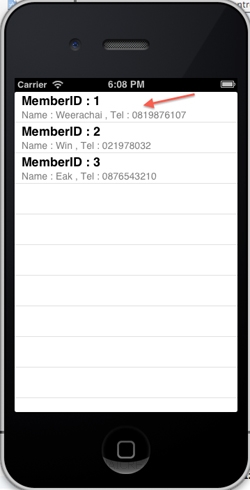
แสดงข้อมูลบน Table View ให้คลิกในแต่ล่ะ Cell
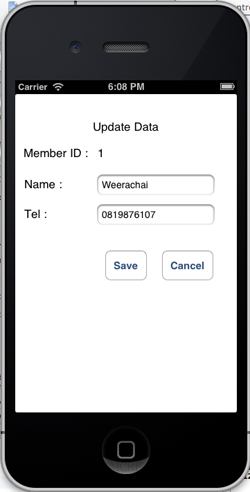
จะแสดงรายละเอียดเพื่อแก้ไข
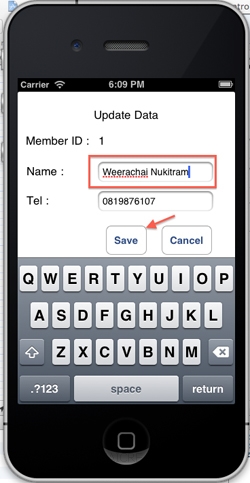
ทำการแก้ไขข้อมูล
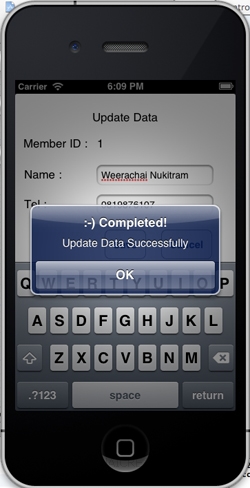
กำลังส่งข้อมูลไปแก้ไขบน Web Server และแจ้งสถานะหลังจากที่ได้ แก้ไข Update เรียบร้อยแล้ว
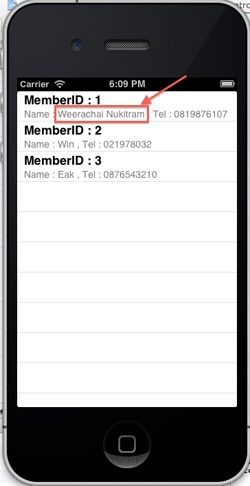
เมื่อกลับไปดูที่ MySQL Database บน Web Server ก็จะพบกว่าข้อมูลได้ถูกแก้ไขเรียบร้อยแล้ว
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2012-12-13 12:27:31 /
2017-03-26 08:53:39 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|
|